flutter 填充
The developers at Flutter recently released version 1.20.0. This update features performance improvements, new widgets, added features, and more. For more specific information about the update, check out the official release notes here, here, and here.
Flutter的开发人员最近发布了1.20.0版本。 此更新具有性能改进,新的小部件,添加的功能等更多功能。 有关更新的更多特定信息,请在此处,此处和此处查看官方发行说明。
We’ll be talking about one newly added feature in this article: autofill. There’s a reason why autofill has been the most requested feature — forms are slow, annoying, and error-prone. Taking advantage of the autofill capabilities introduced in Flutter 1.20.0 will greatly improve user experience when using your app. This article will provide a comprehensive guide to implementing this feature along with detailed examples and sample code.
我们将在本文中讨论一个新添加的功能:自动填充。 自动填充一直是最需要的功能,这是有原因的-表单速度慢,烦人且容易出错。 利用Flutter 1.20.0中引入的自动填充功能,将在使用您的应用程序时极大地改善用户体验。 本文将提供有关实现此功能的全面指南,以及详细的示例和示例代码。
建立表格 (Creating a Form)
To begin adding this feature into your app, start by creating a simple form that asks for the user’s name, email address, and phone number (standard data that should be available on a user’s mobile device). Although the form we’ll be creating will be limited to these fields, the scope of autofill’s capabilities is much wider. Here’s a list of everything it supports:
要将此功能添加到您的应用中,请先创建一个简单的表格,询问用户的姓名,电子邮件地址和电话号码(用户移动设备上应提供的标准数据)。 尽管我们将要创建的表单将限于这些字段,但是自动填充功能的范围要广泛得多。 这是它支持的所有内容的列表:
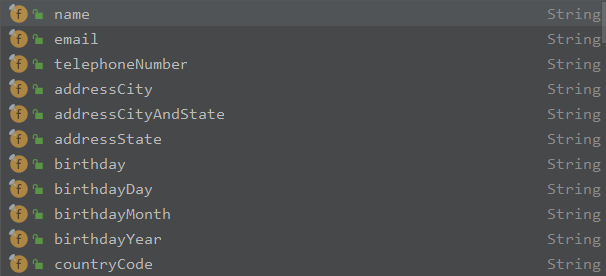
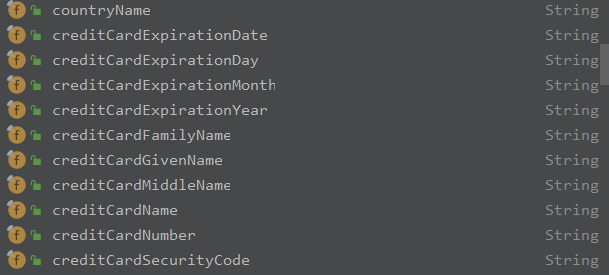