Currying function is a basic tool in functional programming.We can transform a function with multiple arguments into a sequence of nesting functions. It returns a new function that expects the next argument inline.
咖喱函数是函数编程中的基本工具,我们可以将具有多个参数的函数转换为嵌套函数序列。 它返回一个新函数,该函数需要内联下一个参数。
Let’s see a simple example. We’ll create a function ‘add’, which has three arguments and will return the sum of all arguments.
让我们看一个简单的例子。 我们将创建一个函数“ add”,该函数具有三个参数,并将返回所有参数的总和。
function add(a, b, c) {
return a + b + c;
}
add(1, 2, 3);
In above, we see a function with three arguments if we would like to change the numeric arguments we need to write a new function because:
在上面,我们看到一个带有三个参数的函数,如果我们想更改数字参数,我们需要编写一个新函数,因为:
- This function expects only three arguments 该函数只需要三个参数
- It is considered good practice to write functions with a minimal amount of arguments编写带有最少参数的函数被认为是一种好习惯
- We can’t call function add with two parameters or more than three parameters.我们不能使用两个或三个以上的参数来调用函数add。
If we want the function to be more flexible. We could use the currying function.
如果我们希望功能更加灵活。 我们可以使用currying函数。
function add(a) {
return function (b) {
return function (c) {
return a + b + c;
}
}
}
add(1)(2)(3); // 6
We used Currying functions:) It’s simple, right?
我们使用了Currying函数:)很简单,对吧?
Now, We want to write a function, which is more flexible. It will be a function which will not have specific arguments but return the sum of all arguments. We’ll use currying functions, of course.
现在,我们要编写一个更灵活的函数。 这将是一个没有特定参数的函数,但返回所有参数的总和。 当然,我们将使用currying函数。
function add(a) {
let sum = a;
const addSum = (b) => {
sum = sum + b;
return addSum ;
}
addSum .result = () => sum ;
return addSum ;
};
console.log('result ', add(2)(4).result()); // 6
console.log('result ', add(2)(4)(6).result()); // 12
console.log('result ', add(2)(4)(6)(9).result()); // 21
In the above snippet, We see a function ‘add’ with one parameter. This parameter assigns to variable ‘sum’. Variable sum will be the total sum of all parameters. According to the logic of the currying function we created another function ‘addSum’ with arguments ‘b’. This function adds the current sum to value b and returns itself.
在上面的代码片段中,我们看到了一个带有一个参数的函数“ add”。 此参数分配给变量“ sum”。 可变总和将是所有参数的总和。 根据currying函数的逻辑,我们创建了另一个参数为'b'的函数'addSum'。 此函数将当前总和加到值b上并返回自身。
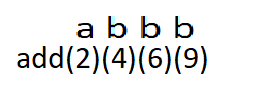
The completed ‘add property’ result. This property displays the result of the sum.
完成的“添加属性”结果。 此属性显示总和的结果。
何时以及为何使用Currying? (When and Why use Currying?)
Currying using when we want to have pre-configured behave our program. Also helps us avoid often call function with same parameter. Example:
当我们想要进行预配置时使用currying来表现我们的程序。 还可以帮助我们避免经常调用具有相同参数的函数。 例:
function add(2) {
return function (b) {
return 2 + b;
}
}
More advance examples find here. Currying can seems difficult but I hope that explained what is currying and you will not be have problem with Currying functions in JavaScript.
在这里可以找到更多高级示例。 咖喱化似乎很困难,但我希望能解释出什么是咖喱味,并且您不会对JavaScript中的咖喱化功能有任何疑问。
翻译自: https://medium.com/tomaszwlodarczyk/what-is-a-currying-function-a99c5ada57cf