vue导出js中的函数
In JavaScript, we have got four major things to do:-
在JavaScript中,我们要做四件事:
- To Store values. 存储值。
- To Store a series of steps. 存储一系列步骤。
- To make decisions. 做出决定。
To Repeat the steps. (loops)
要重复这些步骤。 (循环)
In this article, we will mainly focus on “storing a series of steps”. And to do that we need to learn about function because, in js, function helps us to store a series of steps and execute those steps/codes whenever required by calling that function.
在本文中,我们将主要集中于“存储一系列步骤”。 为此,我们需要学习函数,因为在js中,函数可帮助我们存储一系列步骤,并在需要时通过调用该函数执行这些步骤/代码。
Now coming to the syntax of the function, we have got several ways to define a function but the most basic way is to “declare a function” which is termed as function declaration.
现在来看函数的语法,我们有几种定义函数的方法,但是最基本的方法是“声明一个函数”,称为函数声明。
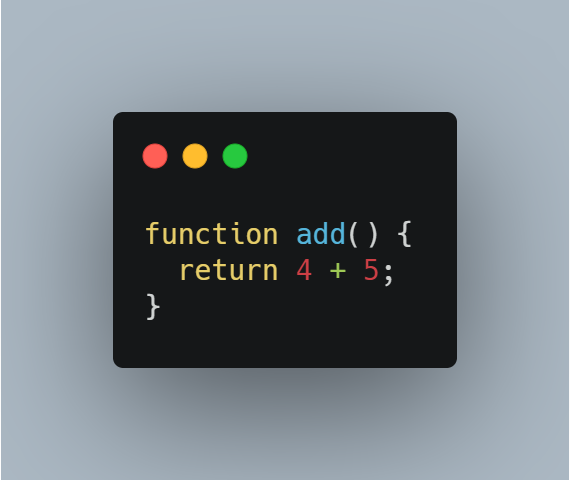
The snippet above shows the most basic way to declare a function.
上面的代码段显示了声明函数的最基本方法。
So, this is the first step in writing a function and the next step is to “call the function” and to do that we need to just write the name of the function i.e “add” and put the parenthesis (()) after that.
因此,这是编写函数的第一步,而下一步是“调用函数” ,为此,我们只需要写函数名即“ add ”,然后在其后加上括号( () ) 。
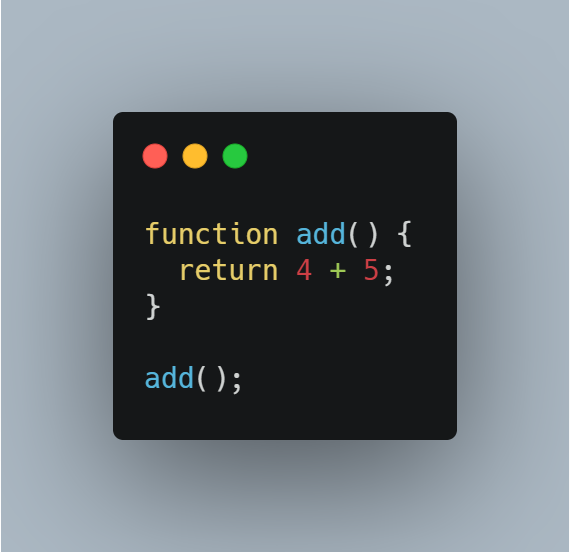
With that, we have seen how to declare a function and then “give a call to that”. One more important thing to note here is that we can call this function anywhere we want, like whenever we call this function we will get the code executed present between the curly braces.
至此,我们已经看到了如何声明一个函数,然后“对其进行调用”。 这里要注意的另一件重要事情是,我们可以在任何需要的地方调用此函数,就像每当调用此函数时,我们都会在花括号之间得到执行的代码。
功能类型 (Types of functions)
function expression
功能表达
Above we have seen the most basic type of function which is function declaration. Now we will see the second type i.e. function expression.
上面我们看到了最基本的函数类型,即函数声明。 现在我们将看到第二种类型,即函数表达式。
So, to define a variable using let, var or const we need these keywords, then the name of the variable and then the assignment operator and then the value. Like this:-
因此,要使用let , var或const定义变量,我们需要这些关键字,然后是变量的名称,然后是赋值运算符,然后是值。 像这样:-
let user = "sushant";
Now, to the right of assignment operator(=) we can only have string, number, boolean, null, undefined and object and any value other than that will be treated as an invalid value.
现在,在赋值运算符(=)的右边,我们只能有字符串,数字,布尔值,null,未定义和对象,除此以外的任何值都将被视为无效值。
So, in JS, functions are treated as an object. So, we can define it to the right of assignment operator like this:-
因此,在JS中,函数被视为对象。 因此,我们可以在赋值运算符的右侧定义它,如下所示:-
let user = function user() {
return "riya";
}
user();
So, this is known as a function expression.
因此,这称为函数表达式。
2. Anonymous function expression
2.匿名函数表达式
An anonymous function expression is almost similar to function expression, the only difference is that, in this, we omit the name of the function, like this:-
匿名函数表达式几乎类似于函数表达式,唯一的区别是,在此,我们省略了函数名称,如下所示:
let user = function () { //name 'user' is omitted
return "riya";
}
user();
3. Arrow function
3.箭头功能
For an arrow function, we can say that it’s a kind of a short form of anonymous function expression. In writing arrow function, we omit the word function from it and just use parenthesis and after parenthesis add the symbol “=>”, like this:-
对于箭头函数,可以说这是匿名函数表达式的一种简短形式。 在编写箭头函数时,我们从中省略单词function ,仅使用括号,并在括号后添加符号“ => ”,如下所示:-
let user = () => {
return "riya";
}
user();
Now, if there is only one line in curly braces then we can omit these curly braces and the keyword “return” too:-
现在,如果花括号中只有一行,那么我们可以省略这些花括号,而关键字“ return ”也可以省略:
let user = () => "riya";user();
带参数的功能 (Function with arguments)
Now that we have discussed the most basic part of functions and almost all types of functions, we will learn about function with arguments.
既然我们已经讨论了函数的最基本部分以及几乎所有类型的函数,我们将学习带参数的函数。
Sometimes what happens is that we need to get different “return” value but the steps should be the same. For example:- we need to do the same operation, say multiplication, but with different operands, in that case we need to write the function again and again. So, here we use functions with arguments, Like this: -
有时发生的事情是我们需要获得不同的“返回”值,但步骤应该相同。 例如:-我们需要执行相同的运算(例如乘法),但使用不同的操作数,在这种情况下,我们需要一次又一次地编写函数。 所以,在这里我们使用功能与参数,像这样: -
function mul(a, b) {
return a * b;
};
console.log(mul(20, 30));
console.log(mul(10, 15));
Hence, in this case, we don’t need to write the code, again and again, we just need to change the arguments & we will get the result.
因此,在这种情况下,我们不需要一遍又一遍地编写代码,只需更改参数即可得到结果。
So, that was all about functions with arguments.
因此,这全都与带有参数的函数有关。
Tbasics of functions are left, that is, about “return” keyword and “function with default parameter”. We may discuss these in future.
剩下的函数基本知识,即“ return ”关键字和“带有默认参数的函数”。 我们将来可能会讨论这些。
Thank you.
谢谢。
翻译自: https://medium.com/@sushantsareen456/functions-in-js-a2beb4a59198
vue导出js中的函数