In the previous article, we discussed how to set up instrumented tests for our Android app. In this tutorial we will add some new functionality to our app and write unit tests to test that behavior.
在上一篇文章中,我们讨论了如何为我们的Android应用设置检测测试。 在本教程中,我们将向我们的应用程序添加一些新功能,并编写单元测试以测试该行为。
You can check out the previous post here
您可以在此处查看以前的帖子
You can check out the beginning code for this article here
您可以在此处查看本文的开始代码
In our app we have a simple TextView which shows a string present in app reources. We will now add a new TextView that will show the number of words shown in the above mentioned simple TextView.
在我们的应用程序中,我们有一个简单的TextView,它显示了应用程序资源中存在的字符串。 现在,我们将添加一个新的TextView,它将显示上述简单TextView中显示的单词数。
Lets add another TextView to our MainActivity’s layout file.
让我们向MainActivity的布局文件添加另一个TextView。
<TextView
android:id="@+id/text_container"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/string_to_be_tested"
/>
<!--Add new textView here-->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/word_count"
/>
Now we will add some code to count the number of words in text_container.
现在,我们将添加一些代码来计算text_container中的单词数。
Create a new class StringUtil that will contain the logic of our word counting algorithm.
创建一个新的StringUtil类,其中将包含我们的单词计数算法的逻辑。
package com.sample.androidtesting
class StringUtil {
companion object {
fun countNoOfWords(text: CharSequence?): Int {
val pattern = "\\s".toPattern()
//If text is null or empty/contains only white space characters, //return 0
if (text == null || text.trim().isEmpty())
return 0
var noOfWords = 0
//Split text using split() function and count the segments that //are not empty. for (word in text.split(pattern.toRegex())) {
if (word.isNotEmpty()) {
noOfWords += 1
}
}
return noOfWords
}
}
}
You can find the code for this class here.
您可以在 此处 找到此类的代码 。
Now we need to call this function from our MainActivity with text shown in text_container as a parameter.
现在,我们需要从MainActivity调用此函数, 并将text_container中显示的文本作为参数。
Our MainActivity will look like this
我们的MainActivity将如下所示
package com.sample.androidtesting
import android.os.Bundle
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
private val textContent by lazy<TextView> { findViewById(R.id.text_container) }private val wordCount by lazy<TextView> { findViewById(R.id.word_count) }override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val text = textContent.textwordCount.text = StringUtil.countNoOfWords(text).toString()
}
}
Code for MainActivity can be found here.
MainActivity的代码可以在 这里 找到 。
Now we want to add unit tests to test countNoOfWords() function. Think of all the possible inputs that this function might receive from MainActivity. We need to make sure that the result returned by this function in all these test cases is as per the defined behavior i.e. “Return the number of words in text supplied as function parameter”.
现在我们要添加单元测试以测试countNoOfWords()函数。 考虑一下此函数可能从MainActivity接收的所有可能的输入。 我们需要确保在所有这些测试用例中此函数返回的结果均符合定义的行为,即“ 返回作为函数参数提供的文本中的单词数 ”。
Unit tests can be run locally and do not require physical device or emulators so you can write as much test cases as you need to.
单元测试可以在本地运行,不需要物理设备或仿真器,因此您可以编写所需数量的测试用例。
To add unit tests we need to create a new class in the test folder. In android studio find the test folder here
要添加单元测试,我们需要在测试文件夹中创建一个新类。 在android studio中找到测试文件夹
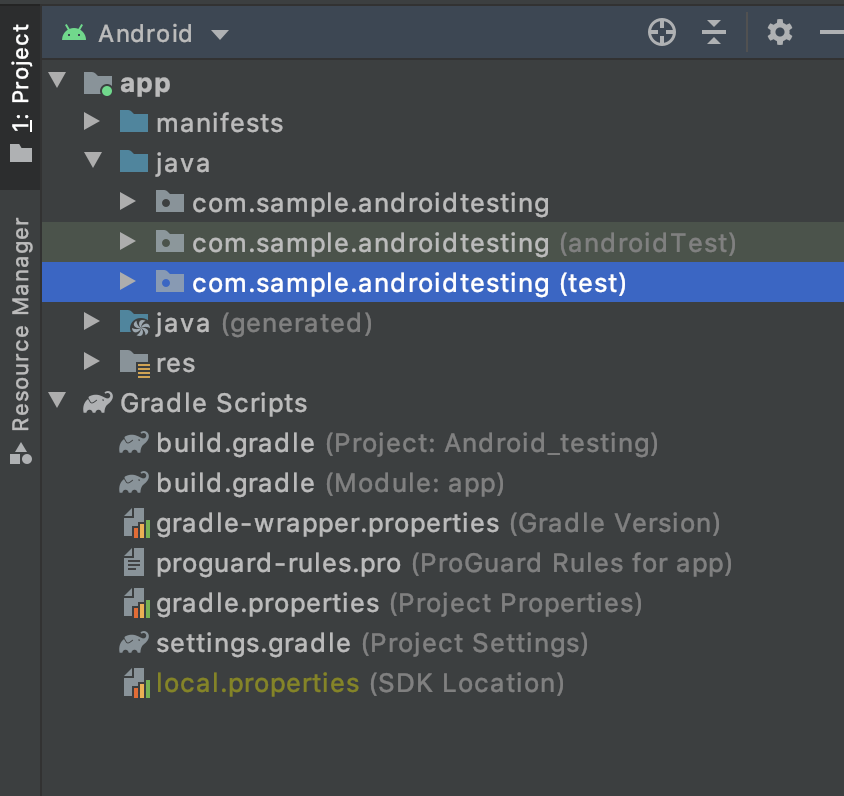
Since we are testing StringUtil class lets name our class StringutilUnitTest.
由于我们正在测试StringUtil类,因此让我们将类命名为StringutilUnitTest。
Now we need to add our testing code.
现在我们需要添加测试代码。
class StringUtilUnitTest{//Test function with null input
@Test
fun checkCountOfWordsForNullString(){
}//Test function with empty string input
@Test
fun checkCountOfWordsForEmptyString(){
}
//Test function with string which contains only white space
@Test
fun checkCountOfWordsForStringWithWhiteSpaces(){
}
//Test function with a single word and lots of white spaces
@Test
fun checkCountOfWordsForStringWithWhiteSpacesAndSingleWord(){
}//Test function with string that contains 4 words
@Test
fun checkCountOfWordsForString_with4words(){
}
}
Note: I have added test cases for all the use cases I could think of. But this is not an exhaustive list. There can be other use cases too.
注意:我为我能想到的所有用例添加了测试用例。 但这不是详尽的清单。 也可能有其他用例。
Let’s examine each test function separately and add code that will perform testing.
让我们分别检查每个测试功能,并添加将执行测试的代码。
//Test function with null input
@Test
fun checkCountOfWordsForNullString(){
val stringTobeTested: String? = null
assertThat(StringUtil.countNoOfWords(stringTobeTested), `is`(0))
}
In this method we will call countNoOfWords() with null input. assertThat Asserts that code (StringUtil.countNoOfWords(stringTobeTested)) satisfies the condition specified by matcher code (`is`(0)).
在此方法中,我们将使用空输入调用countNoOfWords() 。 assertThat断言该代码(StringUtil.countNoOfWords(stringTobeTested))满足匹配器代码( `is`(0) )指定的条件。
//Test function with empty string input
@Test
fun checkCountOfWordsForEmptyString(){
val stringTobeTested = ""
assertThat(StringUtil.countNoOfWords(stringTobeTested), `is`(0))
}
In this test case, we have called countNoOfWords() with and empty stirng and we expect it to return 0 as result.
在此测试用例中,我们使用并清空了stng调用了countNoOfWords() ,并期望它返回0作为结果。
//Test function with string which contains only white space
@Test
fun checkCountOfWordsForStringWithWhiteSpaces(){
val stringTobeTested = " "
assertThat(StringUtil.countNoOfWords(stringTobeTested), `is`(0))
}
In this case we have called countNoOfWords() a string that only contains a white space and we expect our function to return 0 as result.
在这种情况下,我们将countNoOfWords()称为仅包含空格的字符串,并且我们希望函数返回0作为结果。
//Test function with a single word and lots of white spaces
@Test
fun checkCountOfWordsForStringWithWhiteSpacesAndSingleWord(){
val stringTobeTested = " Buyakasha "
assertThat(StringUtil.countNoOfWords(stringTobeTested), `is`(1))
}
In this case we have countNoOfWords() with a String that contains a single word and white spaces. We expect our function to return 1 as result.
在这种情况下,我们有countNoOfWords() ,其String包含单个单词和空格。 我们希望我们的函数返回1作为结果。
//Test function with string that contains 4 words
@Test
fun checkCountOfWordsForString_with4words(){
val stringTobeTested = " Buyakasha wazza my amigos"
assertThat(StringUtil.countNoOfWords(stringTobeTested), `is`(4))
}
In this case we have called countNoOfWords() with a string and expect it to return 4 as result.
在这种情况下,我们已使用字符串调用countNoOfWords()并期望其返回4作为结果。
That’s it. We can now run our tests and all tests should pass. As an exercise you can add test cases with input strings that contain punctuation marks. (They will fail).
而已。 现在,我们可以运行测试,所有测试都应该通过。 作为练习,您可以使用包含标点符号的输入字符串添加测试用例。 (它们将失败)。
For completion, I have also added instrumented test for our new TextView. You can check out the new instrumented test here.
为了完成,我还为我们的新TextView添加了测试工具。 您可以 在此处 签出新的仪器化测试 。
You can find the complete code for this article here
您可以在此处找到本文的完整代码
翻译自: https://medium.com/swlh/getting-started-with-android-testing-part-2-f7f4b4c67454