In this digital era, security has always been a main concern for developers as well as the end users. As smartphones users are increasing day by day, more and more smartphone applications are being developed. According to Statista,
在这个数字时代,安全一直是开发人员和最终用户的主要关注点。 随着智能手机用户的日益增加,正在开发越来越多的智能手机应用程序。 根据Statista,
Over 2 million apps are available for download at Google Play Store and around 1.83 million at Apple App Store.
在Google Play商店中可以下载超过200万个应用程序,在Apple App Store中可以下载大约183万个应用程序。
Most of these apps require user identification for privacy and security. Therefore, first goal of any application that require security is to provide Authentication.
这些应用大多数都需要用户标识才能保护隐私和安全。 因此,任何需要安全性的应用程序的首要目标是提供身份验证。
Firebase Authentication is one of the most commonly used authentication service. So in this article, we are going to learn how we can utilize this service to provide authentication to user on android application. At the end of this article you will be able to
Firebase身份验证是最常用的身份验证服务之一。 因此,在本文中,我们将学习如何利用此服务为android应用程序上的用户提供身份验证。 在本文结尾,您将能够
Implement Email/Password sign-in method
实施电子邮件/密码登录方法
- Work with security measures like Email Verification and Password reset 使用安全措施,例如电子邮件验证和密码重置
If you want to learn how to setup Firebase Authentication for your android project you can check it out here.
如果您想学习如何为您的android项目设置Firebase身份验证,可以在此处查看 。
电子邮件密码验证 (Email-Password Authentication)
We can add this authentication functionality to our app in two steps,
我们可以分两个步骤将此身份验证功能添加到我们的应用中,
- Enable Email/Password on Firebase Console 在Firebase控制台上启用电子邮件/密码
- Code 码
在Firebase控制台上启用电子邮件/密码 (Enable Email/Password on Firebase Console)
In order to enable email and password authentication for your android project, you need to open Firebase Console on your browser and select your android project if already created or create it (know how to create). After selecting your project, you need to choose Authentication from left sidebar in your Firebase Console homepage.
为了为您的android项目启用电子邮件和密码身份验证,您需要在浏览器中打开Firebase Console并选择您的android项目(如果已创建)或创建它( 知道如何创建 )。 选择项目后,您需要在Firebase控制台主页的左侧栏中选择“身份验证”。
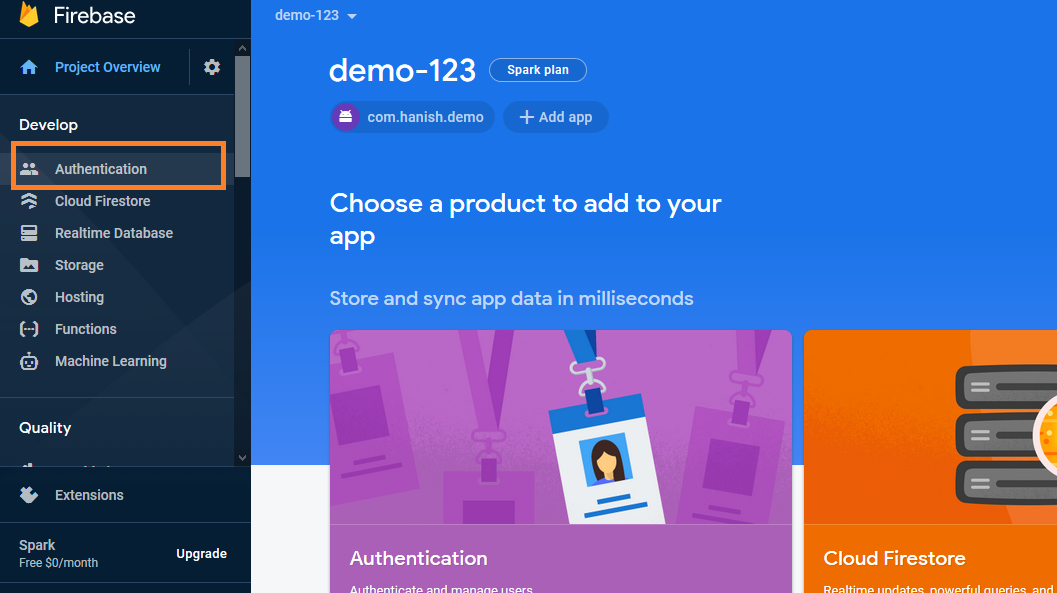
Once you are in Authentication page, click on Sign-in method. Here you will see many sign in methods, however we are going to focus only on Email/password method. Lets enable this method and Save the changes.
进入“身份验证”页面后,单击登录方法。 在这里,您会看到许多登录方法,但是我们将仅关注电子邮件/密码方法。 让我们启用此方法并保存更改。

码 (Code)
We are now ready to write some code. Firebase provides FirebaseAuth class which lets us handle all the authentication related code. So we start by creating a instance of FirebaseAuth.
现在我们准备编写一些代码。 Firebase提供了FirebaseAuth类,它使我们可以处理所有与身份验证相关的代码。 因此,我们首先创建一个FirebaseAuth实例。
.
.
private FirebaseAuth mAuth;
.
.@Overrideprotected void onCreate(Bundle savedInstanceState) {
.
.mAuth = FirebaseAuth.getInstance();
..
}
We use getInstance() method to get instance of FirebaseAuth object. From here we will divide code in four different sections
我们使用getInstance()方法获取FirebaseAuth对象的实例。 从这里我们将代码分为四个不同的部分
- Registration 注册
- Verification Email 验证邮件
- Sign In 登入
- Reset Password 重设密码
- **Bonus Tip** **奖金提示**
注册 (Registration)
First step is to register user using her/his email and password. We use createUserWithEmailAndPassword() method which takes email and password as parameters. We add a onCompleteListener that will be triggered once this method is completed. Inside this listener, we will check if the desired task is successful or not. If task i.e user registration is successful then we will send a verification mail to user.
第一步是使用她/他的电子邮件和密码注册用户。 我们使用createUserWithEmailAndPassword()方法,该方法将电子邮件和密码作为参数。 我们添加一个onCompleteListener ,此方法完成后将被触发。 在此侦听器内部,我们将检查所需任务是否成功。 如果任务即用户注册成功,那么我们将向用户发送验证邮件。
private void registerNewUser(){ ........ mAuth.createUserWithEmailAndPassword(mEmail, mPassword)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>()
{
@Overridepublic void onComplete(@NonNull Task<AuthResult> task) {if (task.isSuccessful()) {
FirebaseUser user = mAuth.getCurrentUser(); .... sendVerificationEmail(); ....... Toast.makeText(MainActivity.this, "Verify your email",
Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(MainActivity.this, "Authentication failed.",Toast.LENGTH_SHORT).show(); .....
}
}
});
}
验证邮件 (Verification Mail)
To send verification mail, we will retrieve current user using getCurrentUser() method on instance of FirebaseAuth. Notice the user returned by getCurrentUser() is of type FirebaseUser. Then we call sendEmailVerification() method on user and once that is successfully completed we will signOut() user so that user can sign in again.
要发送验证邮件,我们将在FirebaseAuth实例上使用getCurrentUser()方法检索当前用户。 请注意,由getCurrentUser()返回的用户的类型为FirebaseUser。 然后,我们对用户调用sendEmailVerification()方法,一旦成功完成,我们将对用户进行signOut()以便用户可以再次登录。
private void sendVerificationEmail() { FirebaseUser user = mAuth.getCurrentUser();
user.sendEmailVerification()
.addOnCompleteListener(new OnCompleteListener<Void>()
{
@Overridepublic void onComplete(@NonNull Task<Void> task) {if (task.isSuccessful())
{
FirebaseAuth.getInstance().signOut();
} else {// this might be case when email is not received then you can show a button which will trigger this function to send Verification Mail again}
}
}
);
}
登入 (Sign In)
Before we sign in, we need to check whether user have verified the email using verification email that we sent. In order to check that we use isEmailVerified() method on FirebaseUser instance. We call this method during sign in process
登录之前,我们需要检查用户是否已使用我们发送的验证电子邮件验证了电子邮件。 为了检查我们在FirebaseUser实例上使用isEmailVerified()方法。 我们在登录过程中称此方法
// to check if Email is verified by user or notprivate boolean checkifEmailVerified() {return mAuth.getCurrentUser().isEmailVerified();
}
We sign in user using signInWithEmailAndPassword() method on FirebaseAuth instance. If this task is completed successfully then we retrieve current user again and check if user email address is verified or not. If it is verified then we redirect user to Homepage otherwise we log out.
我们在FirebaseAuth实例上使用signInWithEmailAndPassword()方法登录用户。 如果此任务成功完成,那么我们将再次检索当前用户,并检查用户电子邮件地址是否已验证。 如果验证通过,则我们会将用户重定向到首页,否则我们将注销。
private void signInUser() {
.....mAuth.signInWithEmailAndPassword(mEmail, mPassword)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>()
{
@Overridepublic void onComplete(@NonNull Task<AuthResult> task) {if (task.isSuccessful()) {
FirebaseUser user = mAuth.getCurrentUser();
if(checkifEmailVerified()){
.......
//REDIRECT TO HOMEPAGE
.....
}else{
mAuth.signOut(); Toast.makeText(MainActivity.this, "Verify your email first.",Toast.LENGTH_SHORT).show();
} else { ......
}// ...}
});
}
重设密码 (Reset Password)
Firebase also provide a simple method to deal with the situation if user forgets password. It provides a method called sendPasswordResetEmail() to send a password reset email. It takes user email address as input.
如果用户忘记密码,Firebase还提供了一种简单的方法来处理这种情况。 它提供了一种称为sendPasswordResetEmail()的方法来发送密码重置电子邮件。 它以用户电子邮件地址作为输入。
private void sendResetPasswordEmail()
{
mAuth.sendPasswordResetEmail(email.getText().toString())
.addOnCompleteListener(new OnCompleteListener<Void>()
{
@Overridepublic void onComplete(@NonNull Task<Void> task) {if (task.isSuccessful())
{
Toast.makeText(MainActivity.this, "Password reset mail is sent.",Toast.LENGTH_SHORT).show();
} else {
}
}
})
.addOnFailureListener(new OnFailureListener() {
@Overridepublic void onFailure(@NonNull Exception e) {
Toast.makeText(MainActivity.this, "There is no id registered with this email address.",Toast.LENGTH_SHORT).show();
}
});
}
**奖金提示** (**Bonus Tip**)
Well, it’s not that much of a pro tip or some extraordinary tip, but it is very important for correct authentication flow. While creating a new user or signing in a user, it is necessary to validate email and password with proper constraints. For password, we simply check if password is non-empty and have characters more than 6. For email, we used Pattern class which uses regular expression to check if provided email id is in valid format or not.
好吧,这不是很多专业技巧或非凡技巧,但对于正确的身份验证流程而言,这非常重要。 在创建新用户或登录用户时,有必要在适当的约束下验证电子邮件和密码。 对于密码,我们只需检查密码是否为非空且字符数大于6。对于电子邮件,我们使用了Pattern类,该类使用正则表达式来检查提供的电子邮件ID是否为有效格式。
Remember, this do not validate whether this email id actually exist or not.
请记住,这不会验证此电子邮件ID是否实际存在。
That is why we verify email address before we allow user to sign in with email address.
这就是为什么我们在允许用户使用电子邮件地址登录之前验证电子邮件地址的原因。
String mEmail = emailEditText.getText().toString().trim();
String mPassword = passwordEditText.getText().toString().trim();if (mEmail.isEmpty()) {emailEditText.setError("Email Required");emailEditText.requestFocus();return;
}if (!Patterns.EMAIL_ADDRESS.matcher(mEmail).matches()) {emailEditText.setError("Valid Email Required");emailEditText.requestFocus();return;
}if (mPassword.isEmpty() || password.length() < = 6) {passwordEditText.setError("Minimum 7 characters required");passwordEditText.requestFocus();return;
}
You can add more validation steps before you use email and password for registration or sign in process. Usually we try to strengthen password by introducing constraints like at least a lowercase and uppercase character, on symbol, one numeric character etc.
在使用电子邮件和密码进行注册或登录之前,您可以添加更多验证步骤。 通常,我们尝试通过在符号,一个数字字符等上引入至少像小写和大写字符这样的约束来增强密码。
We have covered all the steps along with a “Bonus Tip” and come to the end of this article. Using this authentication flow, I believe you can make your application more secure and professional. If you want to see the full code, then you can check out Github repo for this project here. Thank you for reading. Happy Coding !
我们已经涵盖了所有步骤以及“奖金技巧”,并且到了本文的结尾。 相信使用此身份验证流程,您可以使您的应用程序更安全,更专业。 如果您想查看完整的代码,则可以在此处查看该项目的Github存储库。 感谢您的阅读。 编码愉快!
翻译自: https://medium.com/swlh/this-is-how-you-should-use-firebase-authentication-in-android-254847b8c9bd