面向对象编程基础
The information in this article is the result of my understanding of what is described in the book “Object Oriented Thought Process” by Matt Weisfeld.
本文中的信息是我对Matt Weisfeld的“面向对象的思想过程”一书中所描述内容的理解的结果。
Hey there! I hope that this article will help you to understand main OOP concepts easily and quickly. First of all, let’s define the meaning of OOP.
^ h安永那里! 我希望本文将帮助您轻松快速地理解主要的OOP概念。 首先,让我们定义OOP的含义。
什么是物体? (What is an object?)
So… Let’s figure out what is object, data and behavior that I mentioned above.
所以……让我们弄清楚我上面提到的对象,数据和行为。
Object — is a building block of object-oriented program. We can say that object-oriented program is a set of interacting objects.
对象—是面向对象程序的构建块。 可以说面向对象程序是一组交互对象。
Data — is an information that describe the object. For example, the data of the object “Human” are eye color or age.
数据-是描述对象的信息。 例如,对象“人类”的数据是眼睛的颜色或年龄。
Behavior — are acts that the object can do. E.g. the object ‘Human’ can breathe, move ,laugh, cry and etc.
行为-对象可以执行的行为。 例如,“人类”物体可以呼吸,移动,笑,哭泣等。
When we understood what is object and what it consists of, the question arises how to describe such an object in a program. Here the class comes to the rescue.
当我们了解什么是对象及其由什么组成时,就会出现一个问题,即如何在程序中描述这样的对象。 在这里,全班来了。
什么是课程? (What is a class?)
Let’s imagine a situation where you decide to build a car. In order to assemble the car, you must use the car drawings. The same situation with any object in any object-oriented program. To create an object, we must use a class (object’s drawing).
让我们想象一下您决定制造汽车的情况。 为了组装汽车,必须使用汽车图纸。 在任何面向对象的程序中,任何对象的情况都相同。 要创建一个对象,我们必须使用一个类(对象的图形)。
One more example, in order to bake round cookies (objects), you need to have a special round shape (class).
再举一个例子,为了烤制圆形饼干(对象),您需要具有特殊的圆形(类)。
You can think of classes as templates of concrete objects.
您可以将类视为具体对象的模板。
课堂上如何描述数据和行为? (How are data and behavior described in the class?)
To describe object’s data in a class, the concept is used — attributes. Any class must define attributes that preserve the state of every object that will be instantiated based on that class.
为了在类中描述对象的数据,使用了概念-属性。 任何类都必须定义属性,以保留将基于该类实例化的每个对象的状态。
In turn, the class methods implement the desired behavior of the object. They can be public (so called interfaces — other objects have access to use it) and private (so called implementations — can be used only inside the class).
反过来,类方法实现对象的所需行为。 它们可以是公共的(所谓的接口-其他对象可以使用它)和私有的(所谓的实现-只能在类内部使用)。
Finally, having analysed the basic definitions, we can move on to OOP concepts. This paradigm based on 3 concepts: encapsulation, inheritance and polymorphism. Let me explain them to you.
最后,在分析了基本定义之后,我们可以继续介绍OOP概念。 该范式基于3个概念:封装,继承和多态。 让我向您解释。
封装形式 (Encapsulation)
One of the main advantages of using objects is that the object does NOT need to expose all of its attributes and behavior. With good object-oriented design, an object should only show the interfaces that other objects need to interact with it. Details (such as attributes or private methods/implementations) not related to the use of the object should be hidden from all other objects.
对使用对象的主要优点是对象并不需要公开其所有属性和行为。 通过良好的面向对象设计,一个对象应该仅显示其他对象需要与其交互的接口。 与该对象的使用无关的细节(例如属性或私有方法/实现)应从所有其他对象中隐藏。
Interfaces — define the basic means of communication between objects.
接口-定义对象之间通信的基本方法。
Implementation — how exactly an action is performed (an algorithm that is not of interest to another objects).
实现-执行动作的精确度(另一对象不感兴趣的算法)。
It’ll be clear on example. I have an electronic device that needs to be charged. In my flat there is a power socket that allow me to charge the device. The socket itself does not produce electricity, but get it from the power plant. In this situation the socket is an interface, that I can use, but I’m not interested in knowing the exact source of the electricity (such as hydroelectric power plant or nuclear power plant). So, I’m not interested in concrete implementation of producing electricity. The main thing that if I use the socket, it will provide me what I need, no more and no less.
例子很清楚。 我有一个需要充电的电子设备。 我的公寓中有一个电源插座,可让我为设备充电。 插座本身不产生电能,而是从发电厂获取电能。 在这种情况下,套接字是我可以使用的接口,但是我对知道确切的电力来源(例如水力发电厂或核电厂)不感兴趣。 因此,我对发电的具体实施不感兴趣。 最主要的是,如果我使用插座,它将为我提供我所需要的东西,没有更多也没有更少。
遗产 (Inheritance)
Inheritance allows a class to inherit the attributes and methods of another class. This makes it possible to create completely new classes by abstracting out common attributes and behavior.
继承允许一个类继承另一个类的属性和方法。 通过抽象出通用属性和行为,可以创建全新的类。
One of the main design tasks in object-oriented programming is to highlight the commonality of the various classes.
面向对象编程中的主要设计任务之一是强调各种类的通用性。
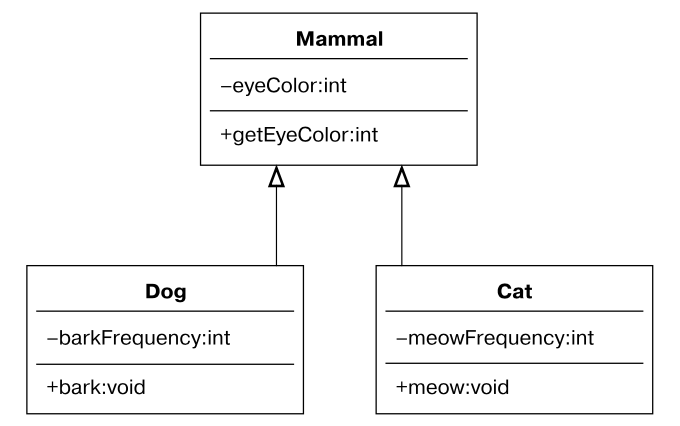
On the (pic. 1) we can see the example of inheritance. There is a superclass that called “Mammal” with eyeColor
attribute and getEyeColor
method. Also there are 2 subclasses, that also have their own attributes and methods, but the main thing that both of them have access to ‘Mammal’s’ attribute and method. They inherit eyeColor
attribute and getEyeColor
method.
在(图1)上,我们可以看到继承的示例。 有一个超类,它具有eyeColor
属性和getEyeColor
方法,称为“哺乳动物”。 也有2个子类,它们也具有自己的属性和方法,但是最重要的是,它们两个都可以访问“哺乳动物”的属性和方法。 它们继承了eyeColor
属性和getEyeColor
方法。
多态性 (Polymorphism)
Polymorphism is a Greek word that literally means plurality of forms. Essentially means replacing the implementation of the parent class with the implementation from the child class.
多态性是一个希腊词,字面意思是多种形式。 本质上意味着用子类的实现替换父类的实现。
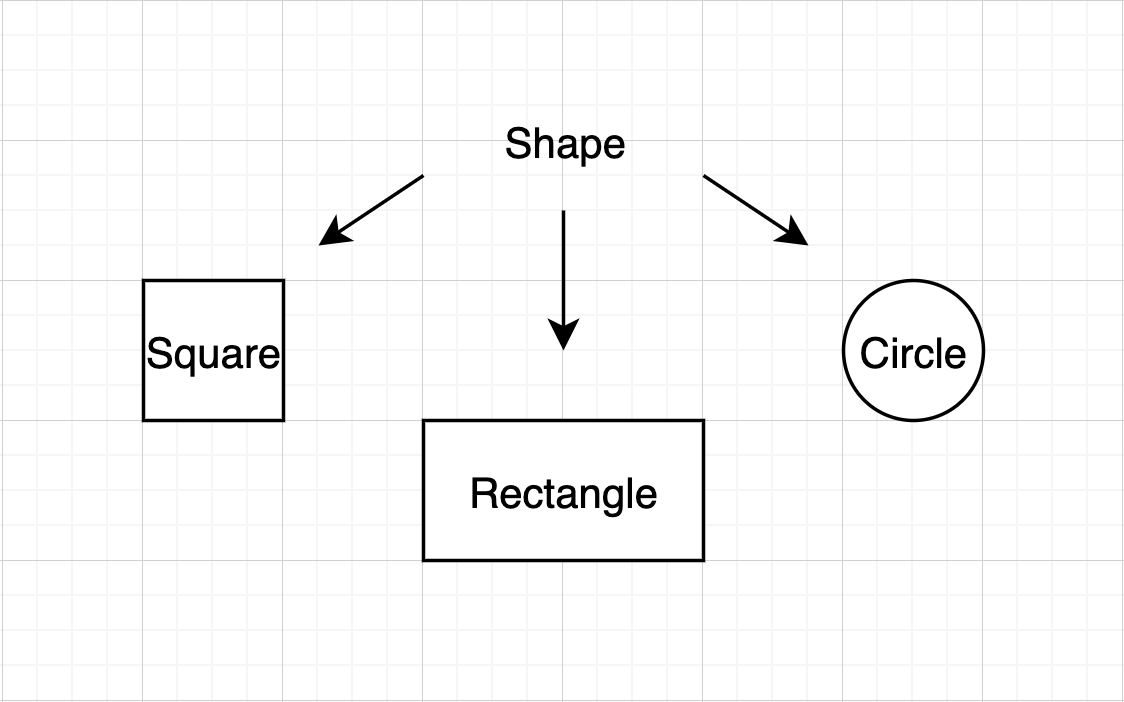
For example, we have a superclass “Shape” and its subclasses. The Shape class has a draw
method. All subclasses inherit this method, but as we know these shapes are drawn differently. So, we need to implement the draw
method for each subclass separately. This step is called “overriding” the method. And this is the main idea of polymorphism — to be able to create many implementations of the same method (and not just a method).
例如,我们有一个超类“ Shape”及其子类。 Shape类具有draw
方法。 所有子类均继承此方法,但众所周知,这些形状的绘制方式有所不同。 因此,我们需要分别为每个子类实现draw
方法。 此步骤称为“覆盖”方法。 这是多态性的主要思想-能够创建同一方法(而不仅仅是一个方法)的许多实现。
奖金。 附加概念-组成 (Bonus. Additional concept — composition)
Objects are often formed or composed of other objects — this is composition.
对象通常由其他对象形成或组成-这就是composition 。
For example, the computer is an object, but it consists of other objects like RAM, monitor, graphics card and etc. Also these objects consist of smaller objects. And so on…
例如,计算机是一个对象,但是它由RAM,监视器,图形卡等其他对象组成。这些对象也由较小的对象组成。 等等…
概要 (Summary)
Encapsulation. Encapsulating data and behavior in a single object is paramount in object-oriented development. One object will contain both its data and behavior, and will be able to hide what it needs from other objects.
封装。 在面向对象的开发中,将数据和行为封装在单个对象中至关重要。 一个对象将同时包含其数据和行为,并且能够从其他对象中隐藏所需的内容。
Inheritance. A class can inherit from another class and take advantage of the attributes and methods defined by the superclass.
继承。 一个类可以继承另一个类,并利用超类定义的属性和方法。
Polymorphism. Indicates that similar objects are capable of implementing the same method in different ways. For example, you might have a system with many shapes.
多态性。 表示相似的对象能够以不同的方式实现相同的方法。 例如,您可能有一个具有多种形状的系统。
Composition. Means that the object is formed from other objects.
组成。 表示该对象是由其他对象形成的。
翻译自: https://medium.com/swlh/object-oriented-programming-f9fd0adfed88
面向对象编程基础