编程语言语法汇总
编程辅导 (PROGRAMMING TUTORIAL)
We love to criticize programming languages.
我们喜欢批评编程语言。
We also love to quote Bjarne Stroustrup:
我们也喜欢引用Bjarne Stroustrup的话:
“There are only two kinds of languages: the ones people complain about and the ones nobody uses.”
“只有两种语言:人们抱怨的语言和没人使用的语言。”
So today I decided to flip things around and talk about pieces of syntax that I appreciate in some of the various programming languages I have used.
因此,今天我决定进行一些讨论,并讨论一些我在使用的各种编程语言中所欣赏的语法。
This is by no means an objective compilation and is meant to be a fun quick read.
这绝不是一个客观的汇编,而是一个有趣的快速阅读。
I must also note that I’m far from proficient in most of these languages. Focusing on that many languages would probably be very counter-productive.
我还必须指出,我对大多数这些语言都不精通。 专注于许多种语言可能会适得其反。
Nevertheless, I’ve at least dabbled with all of them. And so, here’s my list:
不过,我至少已经涉猎了所有这些。 因此,这是我的清单:
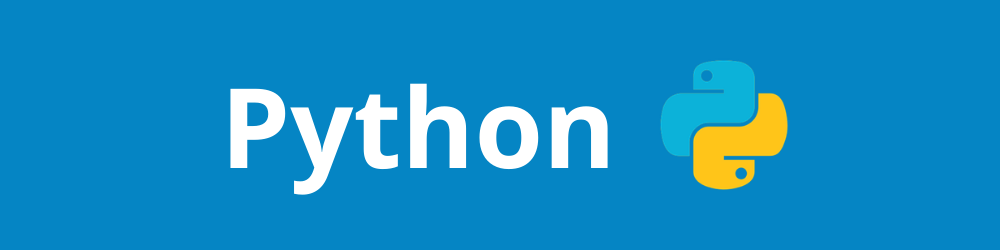
清单理解 (List Comprehension)
def squares(limit):
return [num*num for num in range(0, limit)]
Python syntax has a lot of gems one could pick from, but list comprehension is just something from heaven.
Python语法有很多宝石可以选择,但是列表理解只是天堂。
It’s fast, it’s concise, and it’s actually quite readable. Plus it lets you solve Leetcode problems with one-liners. Absolute beauty.
它快速,简洁,而且实际上可读性强。 此外,它还使您可以使用单线解决Leetcode问题。 绝对美丽。
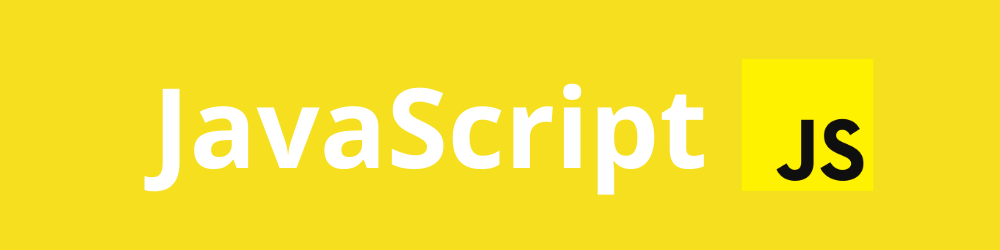
点差算子 (Spread Operator)
let nums1 = [1,2,3]
let nums2 = [4,5,6]let nums = [...nums1, ...nums2]
Introduced with ES6, the JavaScript spread operator is just so versatile and clean that it had to be on this list.
ES6引入了JavaScript传播运算符,它是如此的通用和简洁,因此必须列入此列表。
Want to concatenate arrays? Check.
要串联数组吗? 检查一下
let nums = [...nums1, ...nums2]
Want to copy/unpack an array? Check.
是否要复制/解压缩数组? 检查一下
let nums = [...nums1]
Want to append multiple items? Check.
要附加多个项目? 检查一下
nums = [...nums, 6, 7, 8, 9, 10]
And there are many other uses for it that I won’t mention here.
而且还有许多其他用途,我在这里不会提及。
In short, it’s neat and useful, so that earns my JS syntax prize.
简而言之,它既简洁又有用,因此赢得了我的JS语法奖。
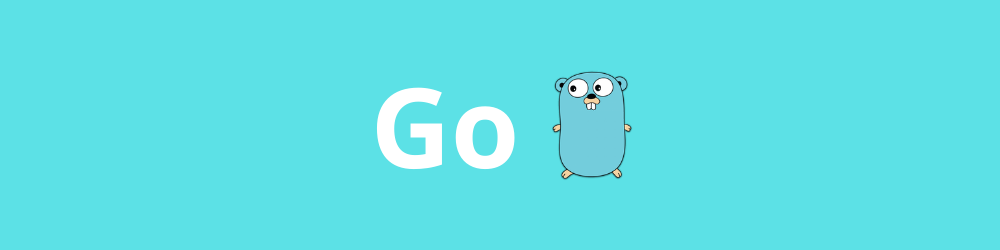
Goroutines (Goroutines)
go doSomething()
Goroutines are lightweight threads in Go. And to create one, all you need to do is add go
in front of a function call.
Goroutines是Go中的轻量级线程。 而要创建一个,只需在函数调用之前添加go
。
I feel like concurrency has never been so simple.
我觉得并发从未如此简单。
Here’s a quick example for those not familiar with it. The following snippet:
对于那些不熟悉它的人,这是一个简单的例子。 以下代码段:
fmt.Print("Hello")
go func() {
doSomethingSlow()
fmt.Print("world!")
}()
fmt.Print(" there ")
Prints:
印刷品:
Hello there world!
By adding go
in front of the call to the closure (anonymous function) we make sure that it it is non-blocking.
通过在闭包(匿名函数)的调用前添加go
,我们可以确保它是非阻塞的。
Very cool stuff indeed!
确实很酷的东西!
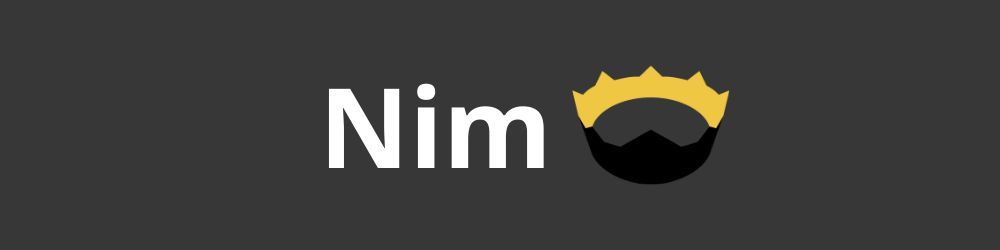
案例与下划线的冷漠 (Case & Underscore Indifference)
proc my_func(s: string) =
echo smyFunc("hello")
Nim is, according to their website, a statically typed compiled systems programming language. And, according to me, you probably never heard of it.
根据他们的网站 ,Nim是一种静态类型的编译系统编程语言。 而且,据我看来,您可能从未听说过。
If you haven’t heard of Nim, I encourage you to check it out, because it’s actually a really cool language. In fact, some people even claim it could work well as Python substitute.
如果您还没有听说过Nim,我鼓励您检查一下它,因为它实际上是一种非常酷的语言。 实际上,甚至有人声称它可以很好地代替Python。
Either way, while the example above doesn’t show it too much, Nim’s syntax is often very similar to Python’s.
无论哪种方式,虽然上面的示例并没有太多说明,但Nim的语法通常与Python非常相似。
As such, this example is not actually what I think is necessarily the best piece of syntax in Nim, since I would probably pick something inherited from Python, but rather something that I find quite interesting.
因此,这个示例实际上并不是我认为一定是Nim中最好的语法,因为我可能会选择从Python继承的东西,而是我觉得很有趣的东西。
I have very little experience with Nim, but one of the first things I learned is that it is case and underscore-insensitive (except for the first character).
我对Nim的经验很少,但是我学到的第一件事是,它不区分大小写和下划线(除了第一个字符)。
Thus, HelloWorld
and helloWorld
are different, but helloWorld
, helloworld
, and hello_world
are all the same.
因此, HelloWorld
和helloWorld
不同,但是helloWorld
, helloworld
和hello_world
都相同。
At first I thought this could be problematic, but the Docs explains that this is helpful when using libraries that made use of a different style to yours, for example.
起初我以为这可能会有问题,但是文档解释说,例如,在使用使用与您的样式不同的库时,这很有用。
Since your own code should be consistent with itself, you most likely wouldn’t use camelCase
and snake_case
together anyway. However, this could be useful if, for instance, you want to port a library and keep the same names for the methods while being able to make use of your own style to call them.
由于您自己的代码应与其自身保持一致,因此您极有可能不会一起使用camelCase
和snake_case
。 但是,例如,当您想要移植一个库并为方法保留相同的名称,同时又能够使用自己的样式来调用它们时,这可能会很有用。
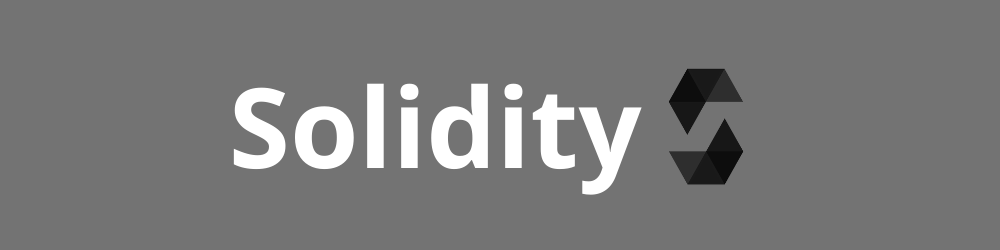
在线组装 (In-line Assembly)
function getTokenAddress() external view returns(address) {
address token;
assembly {
token := sload(0xffffffffffffffffffffffffffffffffffffffff)
}
return token
}
Solidity is the main language for writing smart contracts on the Ethereum blockchain.
坚固性是在以太坊区块链上编写智能合约的主要语言。
A big part of writing smart contracts is optimizing the code, since every operation on the Ethereum blockchain has an associated cost.
编写智能合约的很大一部分是优化代码,因为以太坊区块链上的每项操作都有相关的成本。
As such, I find the ability to add in-line Solidity assembly right there with your code extremely powerful, as it lets you get a little closer to the Ethereum Virtual Machine for optimizations where necessary.
因此,我发现使用代码在其中添加内联Solidity程序集的功能非常强大,因为它使您可以更靠近以太坊虚拟机进行必要的优化。
I also think it fits in very nicely within the assembly
block.
我也认为它非常适合assembly
块。
And, last but not least, it makes proxies possible, which is just awesome.
最后但并非最不重要的一点是,它使代理成为可能,这真是太棒了。
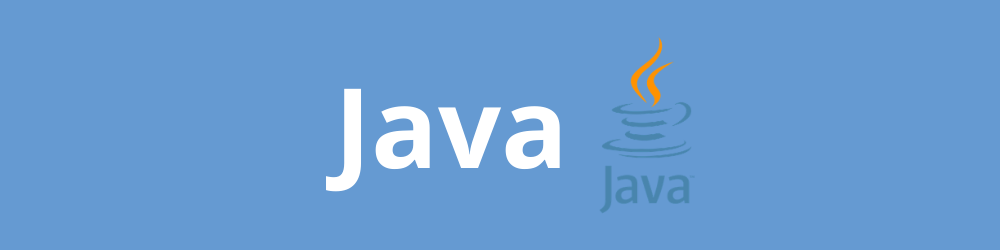
每次循环 (For-Each Loop)
for (int num : nums) {
doSomething(num);
}
In a language generally considered verbose, the for-each loop in Java is a breath of fresh air. I think it looks pretty clean and is quite readable (although not quite Python num in nums
readable).
在通常被认为是冗长的语言中,Java中的for-each循环是一口新鲜空气。 我认为它看起来很干净而且可读性很好(尽管可读性不是很好的Python num in nums
)。
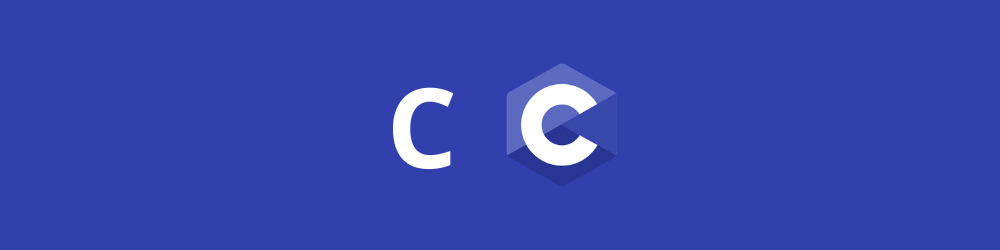
巨集 (Macros)
#define MAX_VALUE 10
I got introduced to C-style macros when building my first Arduino project and for a while had no clue exactly what they did.
在构建我的第一个Arduino项目时,我被引入了C风格的宏,有一阵子不知道它们到底做了什么。
Nowadays, I have a better idea of what macros are and am quite happy with the way they are declared in C.
如今,我对宏是什么有了更好的了解,并且对在C中声明宏的方式感到非常满意。
Not hating on C by any means, but, like Java, there’s little about the actual syntax that stands out, so these last two ones are a little meh, unfortunately.
绝不讨厌C,但是像Java一样,实际的语法几乎没有什么特色,因此不幸的是,最后两个语法有点差强人意 。
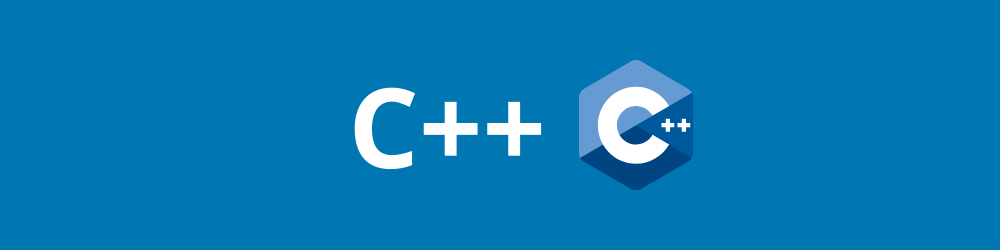
“使用名称空间” (‘using namespace’)
using namespace std;
Sorry :(
对不起:(
And that’s it! So, what are your favorite pieces of syntax?
就是这样! 那么, 您最喜欢的语法是什么?
作者注✍️ (Author’s Note ✍️)
Thanks for reading! If you believe this article was useful, feel free to support me with some claps 👏👏.
谢谢阅读! 如果您认为本文有用,请随时鼓掌支持我。
And remember: We’re talking about syntax here — not features of languages.
请记住:我们在这里谈论的是语法 -并不是语言的功能。
This article is part of my series of Programming Tutorials. Here are some of the other tutorials on the list:
本文是我的一系列编程教程的一部分。 这是列表中的其他一些教程:
编程语言语法汇总