js 子节点父节点兄弟节点
Not too long ago, JavaScript code could only be executed on the browser. As Javascript evolved through the years and became a more powerful language, it was still only used as a front end language, and the other languages had to be used for the back end side. But thank to Node.js which was invented in 2009, by Ryan Dahl; it quickly received a wide range of interest and it is currently still popular. Node.js is used by top companies like: Netflix, Uber or eBay.
不久之前,JavaScript代码只能在浏览器上执行。 随着Java多年来的发展并成为一种功能更强大的语言,它仍然仅用作前端语言,而其他语言则必须用作后端。 但是要感谢Ryan Dahl在2009年发明的Node.js。 它很快引起了广泛的兴趣,并且目前仍然很流行。 像Netflix,Uber或eBay这样的顶级公司都使用Node.js。
Node.js an asynchronous event-driven JavaScript runtime. But what does this exactly mean? JavaScript and Node.js run on the V8 Javascript runtime engine. It converts code as it is run. It forms high-level code and compiles to faster machine process where computer can execute without interpreting.
Node.js是异步事件驱动JavaScript运行时。 但这到底是什么意思? JavaScript和Node.js在V8 Javascript运行时引擎上运行。 它在运行时转换代码。 它形成高级代码,并编译为更快的计算机处理程序,使计算机无需解释即可执行。
什么是节点REPL (What is Node REPL)
Abbreviation stands for ReadEvalPrintLoop, it means that Node.js is a program that cycles between different states. REPL comes in the package when Node.js is downloaded.
缩写代表ReadEvalPrintLoop,它表示Node.js是一个在不同状态之间循环的程序。 下载Node.js时,REPL包含在软件包中。
Read: as name indicated it a stated where it reads the input.
读取:如其名称所示,说明读取输入的位置。
Eval: where the input is evaluated.
评估:评估输入的位置。
Print: evaluation is printed.
打印:打印评估结果。
Loop: process of looping.
循环:循环过程。
使用Node运行程序 (Running a Program with Node)
Instead of using console.log in a browser you can run JavaScript on computer, as below example shows, you can type in your terminal node and name of the file you need, in this case is App.js and the outcome will be Hello World.
可以在计算机上运行JavaScript,而不是在浏览器中使用console.log ,如以下示例所示,您可以键入终端节点和所需文件的名称,在这种情况下为App.js ,结果将为Hello World。 。
// Inside App.js
console.log('Hello World');
//Hello World
模块化 (Modularity)
Is a technique where diffrent parts of a program are in their individual files instead of in one huge file where code may become confusing, and when they come together they create one cohesive unit. All those parts are combined together using require() function.
一种技术,其中程序的不同部分位于它们的单独文件中,而不是在一个大文件中,在这些文件中代码可能会造成混乱,并且当它们组合在一起时,它们会创建一个内聚的单元。 所有这些部分都使用require()函数组合在一起。
// week.js
module.exports = class Week {
constructor(day) {
this.day = day;
}
praise() {
return `Wow, today is ${this.name}!`;
}
};
// app.js
let Week = require('./week.js');
const weekDay = new Week('Saturday');
console.log(weekDay.praise());
In above example we will take a look at two files week.js and app.js. In week.js we are adding Week class a value of module exports. If you wonder what that is, I will tell you it is an object that exists in every Node environment. What this object does is that it holds the content of that file, and it can be required to a diffrent file. So, if you look on line thirteen, in app.js, we have assigned variable Week to the module.exports object of week.js file by invoking the require(). In function, we pass the path to the module; in this case it is (‘./week.js’).
在上面的示例中,我们将每周查看两个文件。 js和app.js。 在week.js中,我们为Week类添加了模块导出的值。 如果您想知道它是什么,我将告诉您它是每个Node环境中都存在的对象。 该对象的作用是保存该文件的内容,并且可能需要其他文件。 因此,如果您在app.js的第十三行中,我们通过调用require()将变量Week分配给week.js文件的module.exports对象。 在功能上,我们将路径传递给模块; 在这种情况下,它是('./week.js') 。
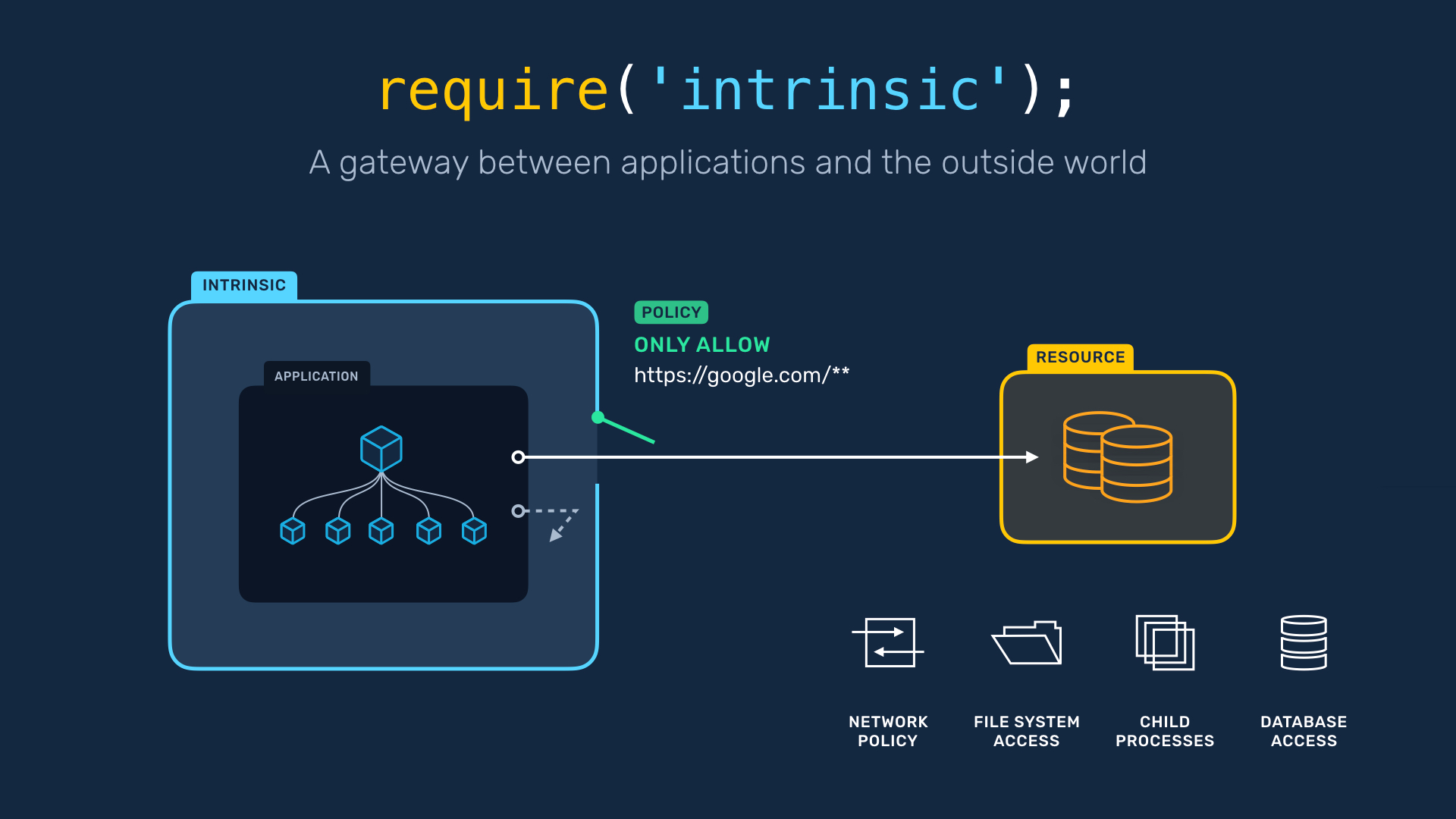
节点包管理器 (Node Package Manager)
NPM organizes modules so they can be easy found by Node, which also manages dependencies. NPM itself consists of three components: website, registry and CLI. You can locally install your npm, by running the below command. One popular package is nodemon. It is very useful tool the watches all the files in the project you working on and resets every time you make changes in the file.
NPM组织模块,以便Node可以轻松找到它们,后者还管理依赖项。 NPM本身包含三个组件:网站,注册表和CLI。 您可以通过运行以下命令在本地安装npm。 一种流行的软件包是nodemon。 这是一个非常有用的工具, 它可以监视您正在处理的项目中的所有文件,并在每次更改文件时将其重置。
$ npm install [package-name]
When npm is installed you will have node_modules and package.json in your folder. Below is an example how package.json looks like.
安装npm后,您的文件夹中将包含node_modules和package.json 。 以下是package.json的示例。
{
"name": "Project name",
"version": "1.0.0",
"description": "This is a basic description",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "Amy XXX",
"license": "XX",
"dependencies": {
"config": "^3.1.0",
"express": "^4.17.1",
"socket.io": "^2.2.0",
"winston": "^3.2.1"
}
}
事件驱动架构 (Event-Driven Architecture)
We often hear that Node is event driven. What does this exactly mean? Node facilitates EventEmitter module that communicates between objects in Node. We can access events using requiring.
我们经常听到Node是事件驱动的。 这到底是什么意思? Node简化了EventEmitter模块,该模块在Node中的对象之间进行通信。 我们可以使用require访问事件。
// Require in the 'events' core module
let events = require('events');
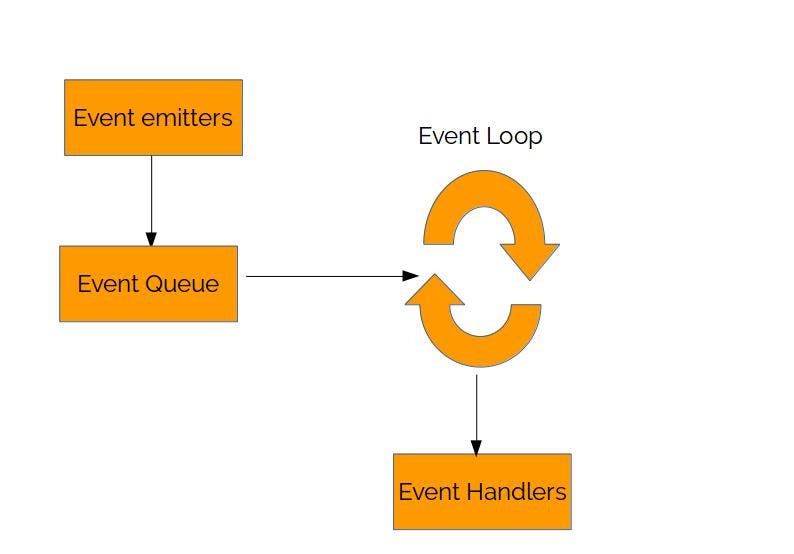
As you can see, it seems that Node.js is one very powerful tool with a very rich ecosystem, ready to use and to create. And can be used to build many different things like:
如您所见,Node.js似乎是一个非常强大的工具,具有非常丰富的生态系统,可以立即使用和创建。 可以用来构建许多不同的东西,例如:
SPAs, single page apps, due to its asynchronous data flow and event loop it captures simultaneously user’s requests and updates.
SPA,单页应用程序,由于其异步数据流和事件循环,因此可以同时捕获用户的请求和更新。
RTAs, real-time app, like Google Doc, Slack are also asynchronies event-driven which play huge roles.
RTA,实时应用程序(例如Google Doc,Slack)也是异步事件驱动的,它们扮演着重要的角色。
Node.js is a very powerful tool and it is not going anywhere, considering it is still very popular.
考虑到Node.js仍然很受欢迎,它是一个非常强大的工具,它无处不在。
Thank you for reading.
感谢您的阅读。
翻译自: https://medium.com/@saohlsen/introduction-to-node-js-4222669a9cf7
js 子节点父节点兄弟节点