So you’re like me, and you’ve started using Javascript on the front end while using some sort of JSON formatted data on the backend. That awesome! But as awesome as it is, this new concept comes with a few techniques that you’re going to want to wrap your head around.
因此,您就像我一样,并且开始在前端使用Javascript,而在后端使用某种JSON格式的数据。 太棒了! 但是,尽管它真棒,但是这个新概念还附带了一些您想将自己的头脑弄清楚的技术。
In order to retrieve data from the backend, you have to ‘fetch’ that data from the database. There are also a few different types of fetches you’’l need to be familiar with so let’s start with the most basic:
为了从后端检索数据,您必须从数据库中“获取”该数据。 您还需要熟悉几种不同类型的提取,所以让我们从最基本的开始:
In order to make any request using fetch, you’ll need some sort of base URL to plug in as the first argument, like so:
为了使用fetch发出任何请求,您需要某种基本URL作为第一个参数插入,如下所示:

The code above will return to you a Promise. A promise is an object that represents the data sent back from the server. It is not, however, the actual data. Instead, we have to next call a ‘then()’ method to actually take out the content from the server’s response. This method takes in a function as its argument and must return the content of the server’s response. Take this fetch, for instance:
上面的代码将向您返回一个承诺。 Promise是一个对象, 代表从服务器发送回的数据。 但是,它不是实际数据。 相反,我们必须接下来调用“ then()”方法,以从服务器的响应中实际取出内容。 此方法接受一个函数作为其参数,并且必须返回服务器响应的内容。 以这个获取为例:
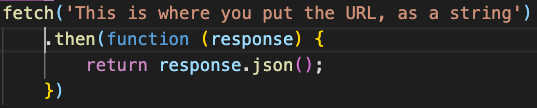
This can also be written as an arrow function, like so:
也可以将其编写为箭头函数,如下所示:

Finally, we add call another ‘then()’ method in order to actually do something with our newly formatted data. A great first step would be to log your data to the console, in order to see what you have and think about how to handle it. Here you have it… A full-fledged fetch from a server of your choosing!
最后,我们添加调用另一个“那么()”方法,以实际做我们的新格式化的数据进行处理。 一个伟大的第一步是将您的数据记录到控制台,以便查看您拥有的内容并考虑如何处理它。 在这里,您已经拥有了……从您选择的服务器中获取完整信息!
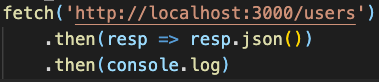
It just so happens that the code we just wrote is everything we need for a ‘GET’ request from a server’s index page of users (or database of your choice). We could refactor this code to receive a single user’s data by adding their database ID number to the end of our base URL like so:
碰巧的是,我们刚刚编写的代码就是我们从服务器的用户索引页(或您选择的数据库)中获取“ GET”请求所需的一切。 我们可以通过将其数据库ID号添加到基本URL的末尾来重构此代码以接收单个用户的数据,如下所示:

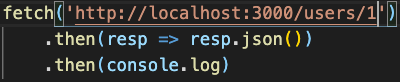
Next up…
接下来...
“ POST”请求 (The ‘POST’ Request)
POST requests are similar to GET requests in that they require a base URL as an argument. They also, however, require ‘options’ as another argument. These ‘options’ are actually a single object that include keys for ‘method,’ ‘headers,’ and ‘body’ of the request. Our options might look something like the following. Pay close attention to what are written as strings here:
POST请求与GET请求相似,因为它们需要基本URL作为参数。 但是,它们还要求“选项”作为另一个论点。 这些“选项”实际上是单个对象,其中包含请求的“方法”,“标题”和“正文”的键。 我们的选项可能类似于以下内容。 请密切注意此处写为字符串的内容:
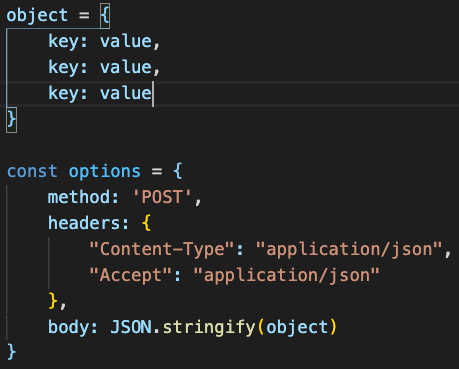
Notice how the ‘body’ key’s value takes in an object that we have previously created. This must be passed in as an argument for JSON.stringify() in order for the object to successfully be ‘POST’ed to the database.
请注意,“ body”键的值如何接收我们先前创建的对象。 必须将它作为JSON.stringify()的参数传入,以便将对象成功地“ POST”到数据库。
A full POST request will look something like this:
完整的POST请求如下所示:
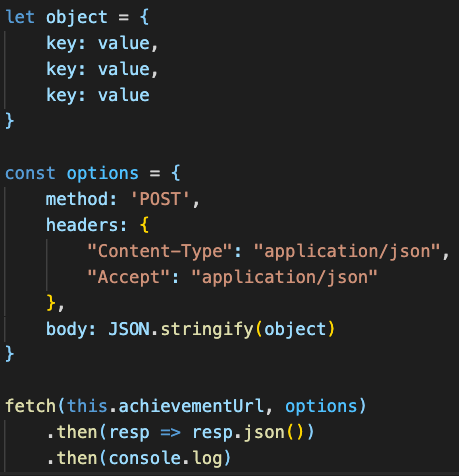
“ PATCH”请求 (The ‘PATCH’ Request)
A PATCH request is very similar to its predecessor, POST, in that it also requires an ‘options’ object as a second argument. The first argument however takes a URL that is, again, appended the ID of the database object you are trying to PATCH.
PATCH请求与其前身POST非常相似,因为它还需要一个“选项”对象作为第二个参数。 但是,第一个参数采用一个URL,该URL再次附加了您要修补的数据库对象的ID。
Let’s look at the ‘options’ for this fetch. I might have a User whose object looks like this:
让我们看一下此获取的“选项”。 我可能有一个用户,其对象如下所示:
{firstName: "Ian", lastName: "Rose"}
The last name is obviously misspelled. To fix this in the database, I would make a PATCH request and place the correct object into JSON.stringify() in the body, like so:
姓显然拼写错误。 要在数据库中修复此问题,我将发出PATCH请求并将正确的对象放入正文中的JSON.stringify()中,如下所示:
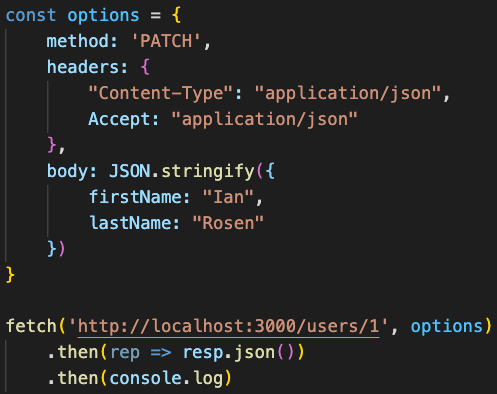
Last but not least…
最后但并非最不重要的…
“删除”请求 (The ‘DELETE’ request)
The DELETE request is quite possibly the simplest fetch out of the four that we are going over here. Much like the PATCH request, the DELETE takes an argument of a URL appended by the ID of the object you would like to remove from the database. Unlike PATCH, the DELETE request does not take any ‘options’ past the ‘method,’ nor does it require any ‘then()’ methods. Since you are deleting an object from the database, what do you really need to render upon completion of the object’s deletion? A DELETE request, simply put, should look something like the following. Keep in mind, that since the ‘options ’are shortened, we are placing the ‘options’ object directly into the parameters of the fetch, instead of first storing it in a variable…
DELETE请求很可能是我们在这里要进行的四个操作中最简单的操作。 与PATCH请求非常相似,DELETE接受URL的参数,该参数后附加您要从数据库中删除的对象的ID。 与PATCH不同,DELETE请求不会在“方法”之后接受任何“选项”,也不需要任何“ then()”方法。 由于要从数据库中删除对象,因此完成对象删除后,您真正需要渲染什么? 简而言之,DELETE请求应类似于以下内容。 请记住,由于缩短了“ options”,我们将“ options”对象直接放入提取的参数中,而不是先将其存储在变量中……
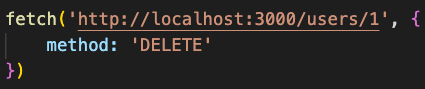