rails中ar
Building an application in Rails is one of the easiest ways to spin up a powerful, fully functioning web app. Recently I was tasked with just that, and while I’m not going to go into great detail about my project and the breakdown of individual parts, I do want to share something I felt was very useful to me in my first Rails build.
在Rails中构建应用程序是启动功能强大且功能齐全的Web应用程序的最简单方法之一。 最近,我的任务是这样做的,尽管我不打算详细介绍我的项目和各个部分的故障,但我确实想分享一些我认为对我的第一个Rails构建非常有用的东西。
One of the golden rules of web development and programming is DRY (Don’t Repeat Yourself). When building my application I found myself repeating a lot of the same code, specifically forms used to create and edit my models. I knew this was an issue that I could solve, but didn’t have the knowledge necessary to solve. When the idea of the same (literally, the same) being used form could be used to both create and edit the models, it was hard to wrap my head around. After some tinkering and getting used to a bit of a strange syntax, the idea became blatantly obvious and almost trivial.
Web开发和编程的黄金法则之一是DRY(不要重复自己)。 在构建应用程序时,我发现自己重复了很多相同的代码,特别是用于创建和编辑模型的表单。 我知道这是我可以解决的问题,但是没有解决该问题所必需的知识。 当可以使用相同形式(实际上是相同形式)的想法来创建和编辑模型时,很难把我的头缠起来。 经过一番修补并习惯了一些奇怪的语法之后,这个想法变得显而易见,几乎是微不足道的。
Take for example this form for my model called instrument.
例如,对于我称为模型的模型的这种形式instrument.
Without dissecting the code here too much, I just mainly want to portray that there’s a lot of tedious code here. The ERB tags alone give bad memories. And to exacerbate this even more, 4 of my models will have forms similar for creation. Add on the forms needed for edit
functionality, and we have two nearly identical forms for each model in the application. Not very DRY if you ask me. This is ON TOP OF any page with an index for displaying all of the instruments in the database. In case it’s not obvious, the first version of the app was functional in a browser, but a complete trash bin of repeated code on the editor side. This is where partials can and most likely will be a life saver.
在这里不对代码进行过多的剖析,我主要想描述一下这里有很多乏味的代码。 仅ERB标签会给您带来不好的回忆。 为了进一步加剧这种情况,我的4个模型将具有相似的创建形式。 加上edit
功能所需的表格,我们在应用程序中的每个模型都有两个几乎相同的表格。 如果你问我,不是很干。 这是任何具有索引的页面的顶部,该索引用于显示数据库中的所有工具。 如果不是很明显,该应用程序的第一个版本可以在浏览器中运行,但是在编辑器端是一个完整的重复代码垃圾箱。 这是局部零件可以而且很可能将挽救生命的地方。
With a partial for the instrument form, my edit view when from this:
对于部分的工具表格,我的编辑视图如下所示:
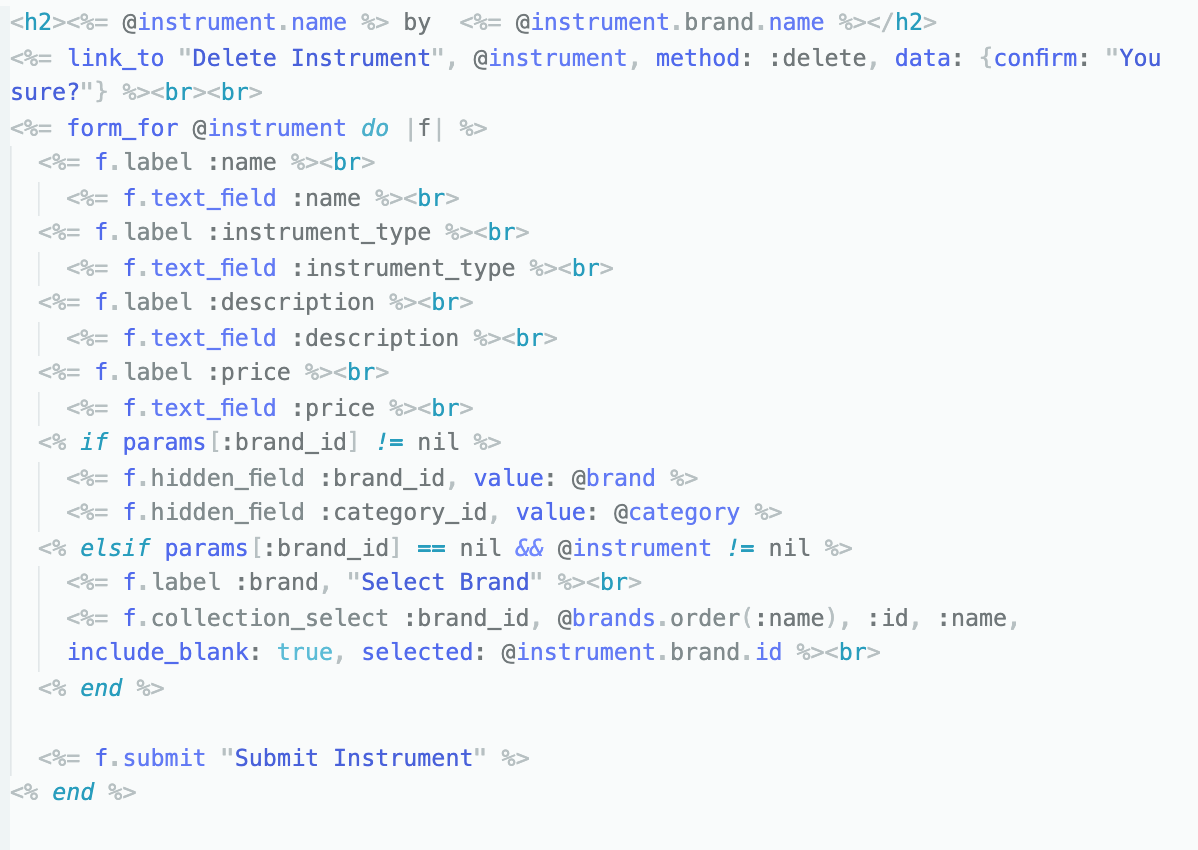
edit view before partial
edit view before partial
To this:
对此:

The code that seemed to disappear didn’t get deleted, it just got moved. And thanks to the magic of Rails, as long as the partial view file in in the root of the views directory, Rails can find it with very little effort. Creating the partial view file is as simple as creating a file in the root of the views directory with the name of whatever you put after the forward slash above, plus an underscore in front. In my example, the partial being rendered here in inside of file /app/views/_instrument.html.erb
.We’re almost there, but you might still be uncertain as to how exactly we use the same form for two different actions. To achieve this, we just have to make sure that our instance variable in the controller is accurate to what we need. In my example, the instance variable @instrument
in the new
method is set to Instrument.new
. In the edit method however, a helper method is called that sets @instrument
equal the instrument in the database with the corresponding id
based on the params hash. Both views will have the same line of code: <%= render partial: ‘/instruments’, locals: { record: @instrument }%>
. I chose to use the same instance variable name for both, so that both methods could use the same partial, with the same instance method name. But depending on what route the user takes to get to the partial, the instance variable will have a different definition (@instrument = Instrument.new
vs. @instrument = Instrument.find_by(id: params[:id])
).
似乎消失的代码没有被删除,只是被移动了。 而且,由于Rails的神奇之处,只要将部分视图文件放在views目录的根目录中,Rails就能毫不费力地找到它。 创建部分视图文件就像在views目录的根目录中创建文件一样简单,该文件名的名称应为您在上面的正斜杠后加上任何下划线的名称。 在我的示例中,部分渲染在文件/app/views/_instrument.html.erb
内部。我们已经差不多了,但是您仍然不确定我们对两个不同动作使用相同形式的精确程度。 为此,我们只需要确保控制器中的实例变量与我们所需要的准确即可。 在我的示例中, new
方法中的实例变量@instrument
设置为Instrument.new
。 但是,在edit方法中,调用了一个辅助方法,该方法根据参数散列将@instrument
设置@instrument
等于数据库中具有相同id
的乐器。 这两个视图将具有相同的代码行: <%= render partial: '/instruments', locals: { record: @instrument }%>
。 我选择对两者使用相同的实例变量名称,以便两个方法可以使用具有相同实例方法名称的相同局部变量。 但是根据用户到达部分变量的路线,实例变量将具有不同的定义( @instrument = Instrument.new
与@instrument = Instrument.find_by(id: params[:id])
)。
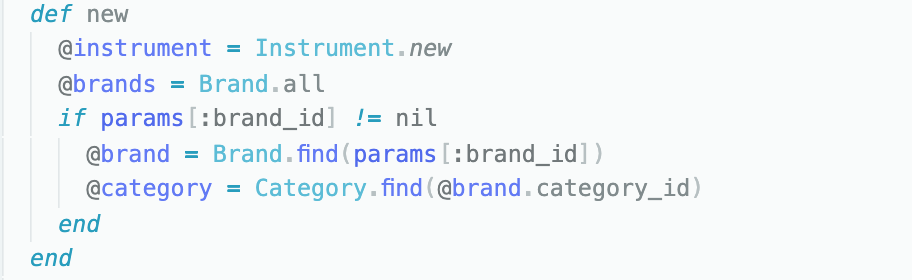
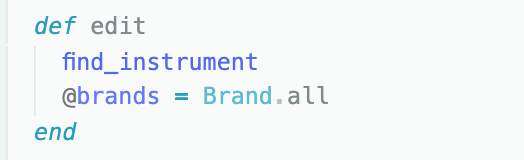

The main benefit I found from this, is that if I need to add or remove or change an attribute for a model, and I want a user to be able to specify what this attribute is upon creation or edit it later, I only have to change it in one place. This is a simple concept but one that is often taken for granted. When working with a full scale Rails app, the amount of different view and controller files that are relevant to one facet of the app can be daunting, and reducing the amount of travel needed to make a change can save time and ensure continuity in the application.
我从中发现的主要好处是,如果我需要添加或删除或更改模型的属性,并且希望用户能够在创建或以后编辑时指定该属性,则只需要一处更改。 这是一个简单的概念,但通常被认为是理所当然的。 使用全尺寸Rails应用程序时,与该应用程序一个方面相关的不同视图和控制器文件的数量可能会令人望而生畏,减少进行更改所需的旅行数量可以节省时间并确保应用程序的连续性。
翻译自: https://levelup.gitconnected.com/utilizing-partials-in-your-rails-application-7a6806cfaaa4
rails中ar