react 状态管理工具
React is one of the most loved frontend libraries in the developer community. Together with React, the terms like Virtual DOM, Functional Components, State Management, and Higher-Order Components have emerged. Among these terms, State Management plays a vital role.
React是开发人员社区中最受欢迎的前端库之一。 与React一起,出现了诸如虚拟DOM,功能组件,状态管理和高阶组件之类的术语。 在这些术语中,国家管理起着至关重要的作用。
State Management is one of the key factors that need to be considered before starting a React project. Developers use famous patterns and libraries such as Flux, Redux, and Mobx to manage state in React Apps. However, they add complexity and boilerplate codes into the applications.
状态管理是启动React项目之前需要考虑的关键因素之一。 开发人员使用Flux,Redux和Mobx等著名的模式和库来管理React Apps中的状态。 但是,它们在应用程序中增加了复杂性和样板代码。
In this article, let’s discuss how React Query addresses the above-mentioned issue by building a small pokemon application and diving into its key concepts.
在本文中,我们将讨论React Query如何通过构建一个小型神奇宝贝应用程序并深入研究其关键概念来解决上述问题。
Tip: Share your reusable components between projects using Bit (Github). Bit makes it simple to share, document, and organize independent components from any project.
提示:使用Bit ( Github )在项目之间共享可重用组件。 Bit使共享,记录和组织来自任何项目的独立组件变得简单。
Use it to maximize code reuse, collaborate on independent components, and build apps that scale.
使用它可以最大程度地重复使用代码,在独立组件上进行协作以及构建可扩展的应用程序。
Bit supports Node, TypeScript, React, Vue, Angular, and more.
Bit支持Node,TypeScript,React,Vue,Angular等。
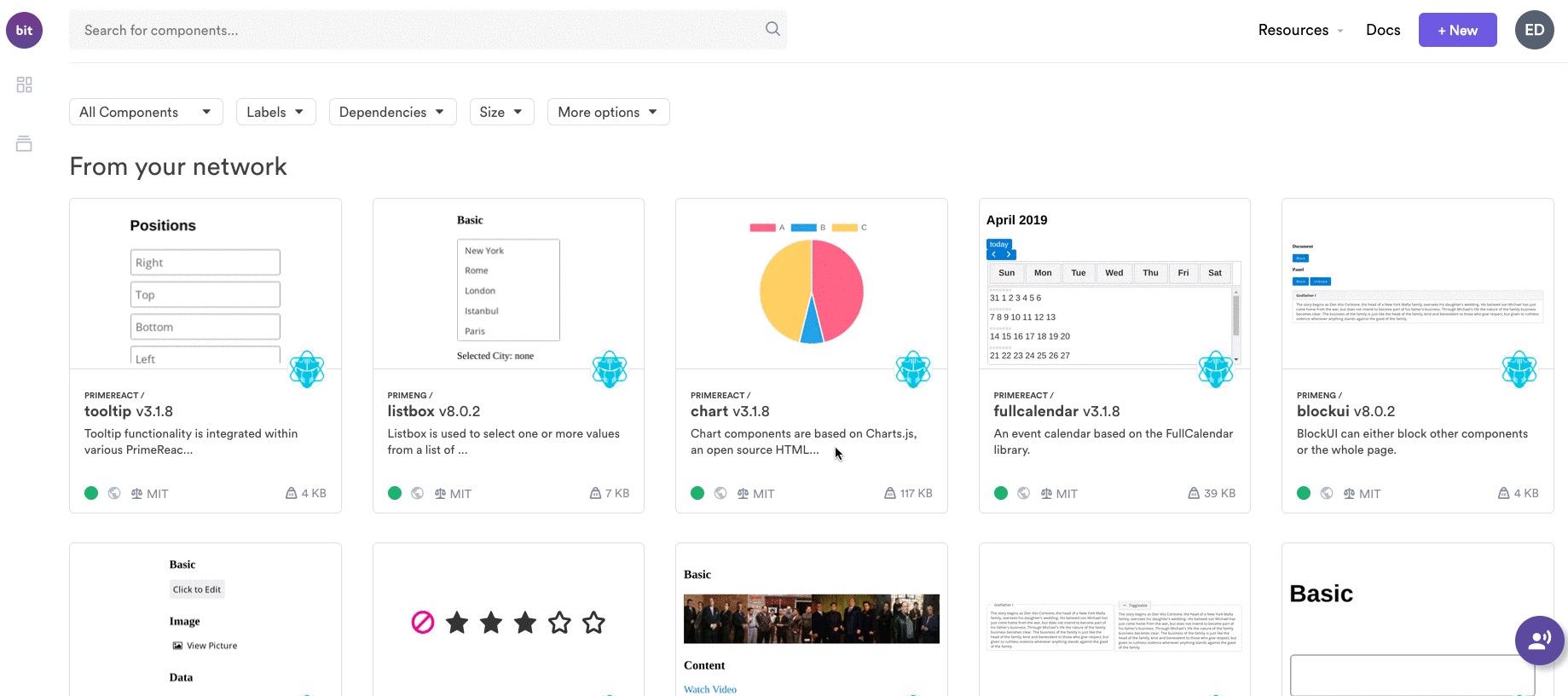
什么是React Query?(What is React Query?)
React Query is one of the state management tools which takes a different approach from Flux, Redux, and Mobx. It introduces the key concepts of Client State and Server State. This makes React Query one of the best libraries to manage state since all other state management patterns address only the client state and find it difficult to handle the server state that needs to be fetched, listened, or subscribed.
React Query是状态管理工具之一,它采用与Flux,Redux和Mobx不同的方法。 它介绍了客户端状态和服务器状态的关键概念。 这个 使React Query成为管理状态的最佳库之一,因为所有其他状态管理模式都仅处理客户端状态,并发现难以处理需要获取,监听或订阅的服务器状态。
In addition to handling server state, it works amazingly well, with zero-configurations, and can be customized to your liking as your application grows.
除了处理服务器状态外,它还具有零配置,效果非常好,并且可以根据您的应用程序的增长对其进行自定义。
Let me show that using a few examples.
让我使用一些示例进行说明。
安装React查询 (Installing React Query)
First of all, let's install react query inside a React project
首先,让我们在React项目中安装react查询
npm install react-query react-query-devtools axios --save
OR
要么
yarn add react-query react-query-devtools axios
配置开发工具 (Configuring Dev Tools)
React Query also has its own dev tools which help us to visualize the inner workings of React Query. Let's configure the React Query devtools in App.js file
React Query也有自己的开发工具,可帮助我们可视化React Query的内部工作原理。 让我们在App.js文件中配置React Query devtools
import { ReactQueryDevtools } from 'react-query-devtools'function App() {
return (
<>
{/* The rest of your application components */}
<ReactQueryDevtools initialIsOpen={false} />
</>
)
}
When we configure the React Query devtools you can see the React Query logo on the left bottom of your application like this
当我们配置React Query devtools时,您可以在应用程序的左下方看到React Query徽标,如下所示
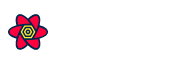
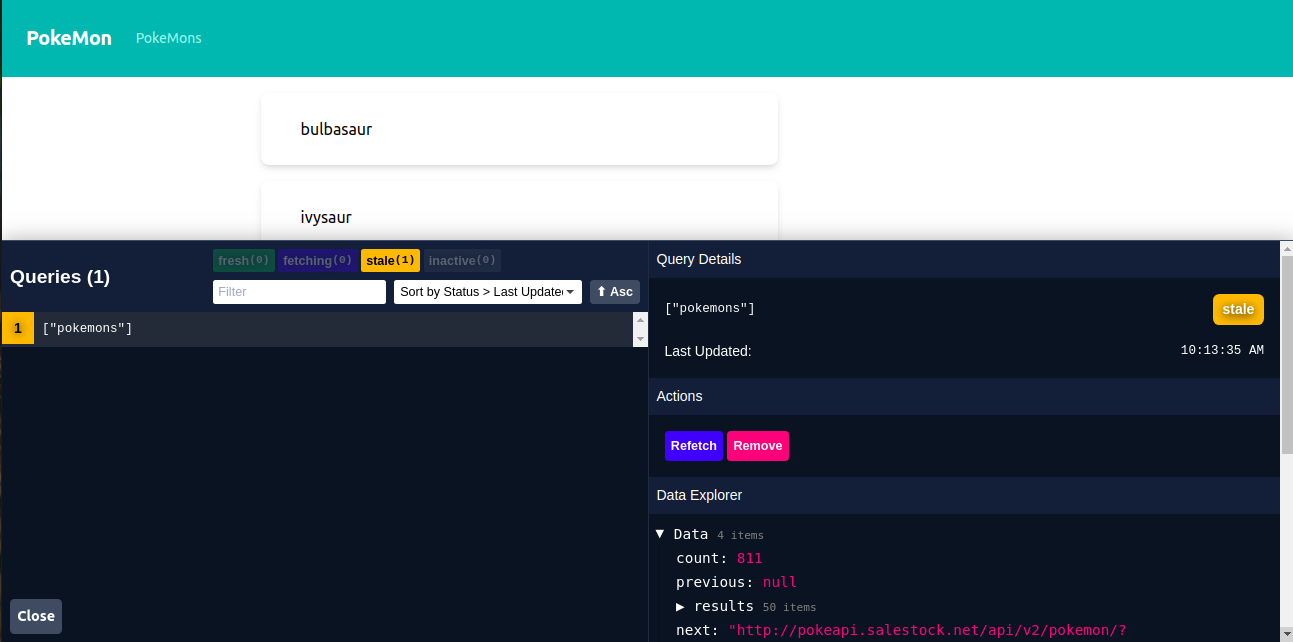
Devtools help us to see how the data flow happens inside the application just like redux devtools. This really helps to reduce the time in debugging the app.
Devtools可以像redux devtools一样帮助我们了解数据流如何在应用程序内部发生。 这确实有助于减少调试应用程序的时间。
Just like GraphQL, React Query is also based on similar core concepts like
就像GraphQL一样,React Query也基于类似的核心概念,例如
- Queries查询
- Mutations变异
- Query Invalidation查询无效
使用查询获取口袋妖怪(Fetching Pokemons using Queries)
In this example, let’s use the Pokemon API. We will start with useQuery which takes a unique key and a function which is responsible for fetching data
在此示例中,让我们使用Pokemon API。 我们将从useQuery开始,它具有一个唯一的键和一个负责获取数据的函数
import React from "react";
import axios from "axios";
import { useQuery } from "react-query";
import Card from "./Card";const fetchPokemons = async () => {
const { data } = await axios.get("http://pokeapi.salestock.net/api/v2/pokemon/?limit=50");
return data;
};function Main() {
const { data, status } = useQuery("pokemons", fetchPokemons);const PokemonCard = (pokemons) => {
return pokemons.results.map((pokemon) => {
return <Card key={pokemon.name} name={pokemon.name}></Card>;
});
};return (
<div>
{status === "loading" && <div>Loading...</div>}
{status === "error" && <div>Error fetching pokemons</div>}
{status === "success" && <div>{PokemonCard(data)}</div>}
</div>
);
}export default Main;
The above code will render a view as below
上面的代码将呈现如下视图
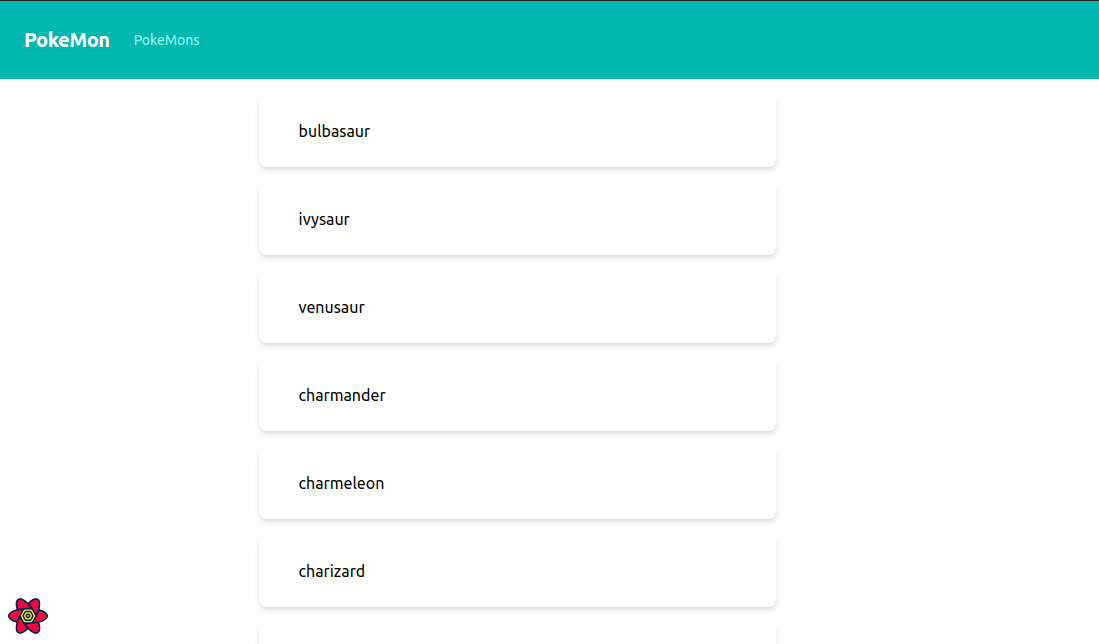
React查询缓存(React Query Caching)
As you can see the useQuery returns the data and status which can be used to display Loaders, Error messages, and the actual Data. By default, the React Query will only request data when it is stale or old.
如您所见, useQuery返回数据和状态,这些数据和状态可用于显示加载程序,错误消息和实际数据。 默认情况下,React Query仅在陈旧或过时才请求数据。
React Query caches the data so that it won't render the components unless there is a change. We can also use some special config with useQuery in order to refresh data in the background.
React Query缓存数据,以便除非进行更改,否则它不会呈现组件。 我们还可以在useQuery中使用一些特殊的配置,以便在后台刷新数据。
const { data, status } = useQuery("pokemons", fetchPokemons,{staleTime:5000,cacheTime:10});
The above config will make React Query fetch data every 5 seconds in the background. We can also set a cacheTime and a retryTime which defines the time that the browser should keep the cache and the number of attempts that it should fetch data on fails.
上面的配置将使React Query每5秒钟在后台获取一次数据。 我们还可以设置cacheTime和retryTime ,它们定义浏览器应保留高速缓存的时间以及应获取数据的尝试次数失败。
使用查询无效重置缓存 (Resetting cache with Query Invalidation)
React Query will fetch data once the data/cache is stale. It happens when the default staleTime is passed. You can also programmatically invalidate the cache so that React Query will refresh the data.
一旦数据/缓存过时,React Query将获取数据。 通过默认的staleTime时会发生。 您还可以通过编程使缓存无效,以便React Query刷新数据。
In order to do that, use queryCache. It is a utility instance that contains many functions that can be used to further manipulate queries and invalidate the cache.
为此,请使用queryCache。 它是一个实用程序实例,其中包含许多可用于进一步处理查询并使缓存无效的功能。
queryCache.invalidateQueries("pokemons")
React查询变量 (React Query Variables)
We can also pass variables to the query. For that, we need to pass them down like an array.
我们还可以将变量传递给查询。 为此,我们需要像数组一样将它们向下传递。
const { data, status } = useQuery(["pokemons",75], fetchPokemons);
The first element will be the key and the rest of the elements are variables. in order to use the variable let's do some modifications to our fetchPokemons function
第一个元素是键,其余元素是变量。 为了使用变量,让我们对fetchPokemons函数进行一些修改
const fetchPokemons = async (key,limit) => {
const { data } = await axios.get(`http://pokeapi.salestock.net/api/v2/pokemon/?limit=${limit}`);
return data;
};
玩变异 (Playing with Mutations)
Mutations are typically used to create/update/delete data or perform server side-effects. React Query provides useMutation hook in order to perform mutations. Let's create a mutation to create a pokemon
突变通常用于创建/更新/删除数据或执行服务器副作用。 React Query提供useMutation钩子以执行变异。 让我们创建一个突变来创建宠物小精灵
import React from "react";
import { useQuery } from "react-query";function Pokemon() {
const [name, setName] = useState("");
const [mutateCreate, { error, reset }] = useMutation(text => axios.post('/api/data', { text }),
{ onSuccess: () => {
setName('')
},
});return (
<div>
<form onSubmit={e=>{
e.preventDefault()
mutateCreate(name)
}>
{error && <h5 onClick={() => reset()}>{error}</h5>}
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<br />
<button type="submit">Create Pokemon</button>
</form>
</div>
);
}export default Pokemon;
In this example, when we add a new pokemon name and click on Create Pokemon button it will do the mutation and retrieve data. If the mutation failed the error will be displayed. The error and data state can be cleared by using the reset function which will reset the mutation. The onSuccess function can be used to clear the state of the input or the name.
在此示例中,当我们添加新的神奇宝贝名称并单击“创建神奇宝贝”按钮时,它将进行突变并检索数据。 如果突变失败,将显示错误。 可以通过使用重置功能来清除错误和数据状态,该功能将重置突变。 onSuccess函数可用于清除输入或名称的状态。
A mutation has more properties like onSuccess, isIdle, isLoading, isError, isSuccess. These can be used to handle errors and display relevant information for different states of the mutation.
变异具有更多属性,例如onSuccess,isIdle , isLoading , isError , isSuccess。 这些 可用于处理错误并显示有关突变不同状态的相关信息。
结论 (Conclusion)
In conclusion, React Query is one of the best ways of fetching, caching, and updating asynchronous data. We just need to tell the library where do you need to fetch the data, and it will handle caching, background updates, and refresh data without any extra code or configuration.
总之,React Query是获取,缓存和更新异步数据的最佳方法之一。 我们只需要告诉库您需要在哪里获取数据,它就可以处理缓存,后台更新和刷新数据,而无需任何额外的代码或配置。
It also provides some hook or events for mutation and queries to handle errors and other states of side effects which removes the need for useState
and useEffect
hooks and replace them with a few lines of React Query logic.
它还为突变和查询提供了一些挂钩或事件,以处理错误和其他副作用状态,从而消除了对useState
和useEffect
挂钩的需求,并用几行React Query逻辑替换了它们。
React Query deserves more attention in the React community due to these unique features and React Query has the potential to compete with Redux, Context, etc. You can find the code example in the following link.
由于这些独特的功能,React Query在React社区中应引起更多关注,React Query具有与Redux , Context等竞争的潜力。您可以在以下链接中找到代码示例。
Thanks for reading and if you have any questions, let me know in the comments below :)
感谢您的阅读,如果您有任何疑问,请在下面的评论中让我知道:)
Cheers!
干杯!
学到更多 (Learn More)
翻译自: https://blog.bitsrc.io/react-query-an-underrated-state-management-tool-5618b7b8cb36
react 状态管理工具