The call back function is a core concept of asynchronous JS development. To better follow and understand the concept I suggest you code along with this blog so you can test your functions using the example provided and straight your knowledge.
回调函数是异步JS开发的核心概念。 为了更好地理解和理解这一概念,我建议您在本博客中进行编码,以便您可以使用提供的示例来测试功能并掌握相关知识。
Let’ start with a classic example of a call back function.
让我们从回调函数的经典示例开始。
This function takes two arguments, the first is a function and the second is the amount of time in a millisecond to wait before the function get called. In this example, the callback function is the one we defined and passed as an argument to set time out.
此函数有两个参数,第一个是函数,第二个是调用该函数之前要等待的时间(以毫秒为单位)。 在此示例中,回调函数是我们定义的函数,并作为参数设置超时时间。
() =>{console.log('two seconds has passed')}
The call back function is just a function provided as an argument inside another function with the intention to call it later in the future. The above example uses setTimeout with the intention to call the inside function after that time has passed, in this case after 2 seconds.
回调函数只是在另一个函数中作为参数提供的函数,意在将来稍后调用它。 上面的示例使用setTimeout,目的是在该时间过去之后(在本例中为2秒之后),调用内部函数。
Point of confusion
困惑点
A lot of people thinks that every callback pattern used is an asynchrony. This is not true because in the above example setTimeout is a node API asynchronous function, but also forEach and filter are using the callback pattern but nothing asynchronous happen inside these methods
许多人认为使用的每个回调模式都是异步的。 这是不正确的,因为在上面的示例中setTimeout是节点API异步函数,而且forEach和filter正在使用回调模式,但是这些方法内部没有异步发生
Example of a callback non-asynchronous:
非异步回调的示例:
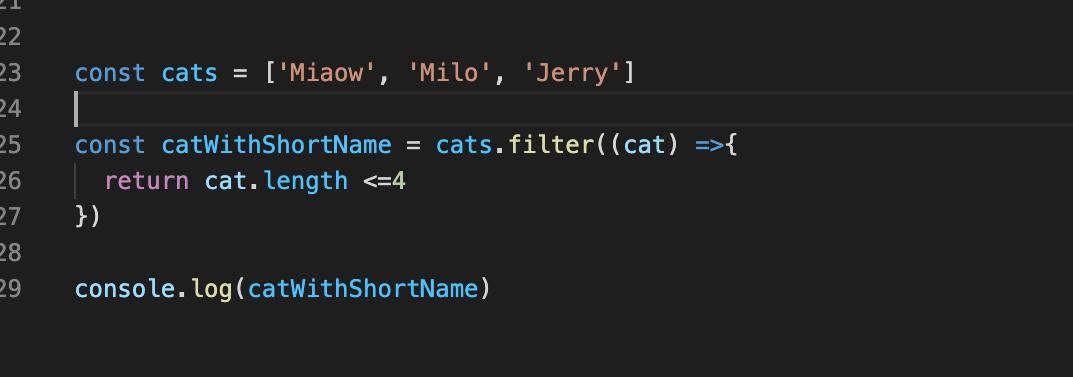
To make more clear our concept we can see the filter method in action here. We created an array of cat’s names and we filtered the cat that has the shorter name. In this example, we are using the callback pattern as the previous one but there is nothing asynchronous happening inside this method, is not interacting with a native node API and it’s just a standard javascript synchronous code.
为了更清楚地了解我们的概念,我们可以在这里看到实际的过滤方法。 我们创建了一个猫名数组,并过滤了名称较短的猫。 在此示例中,我们将回调模式用作上一个模式,但是此方法内部没有异步发生,没有与本机节点API进行交互,而只是标准JavaScript同步代码。
The benefit of defining your own callback function
定义自己的回调函数的好处
The question is why are so important to use the call back function and how this can help us to improve our code. Imagine we have a function “geocode()” that takes as argument a location and give us back some coordinates. Assuming we want to use this function in multiple files in our application let see how the structure of this function using a callback and a non-call back function can impact our functionality.
问题是,为什么使用回调函数如此重要,以及这如何帮助我们改进代码。 想象一下,我们有一个函数“ geocode()”,该函数以一个位置作为参数并返回一些坐标。 假设我们要在应用程序的多个文件中使用此函数,请查看使用回调和非回调函数的此函数的结构如何影响我们的功能。
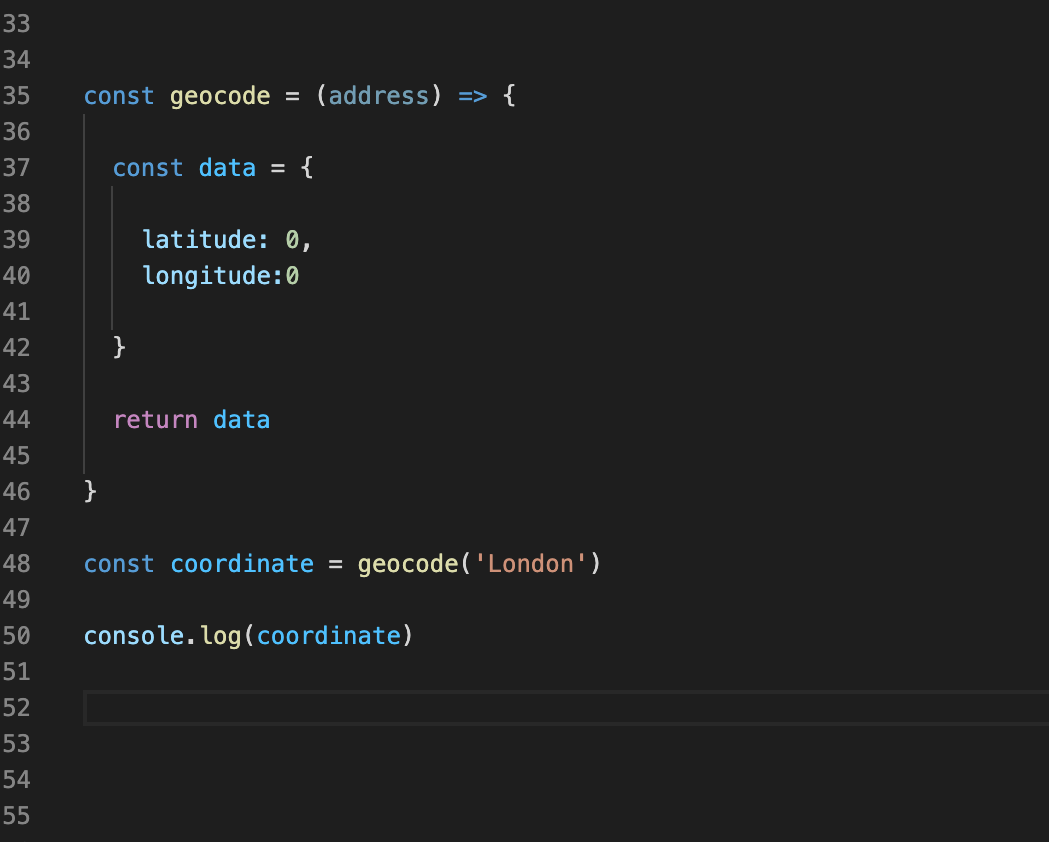
In this example, we can see that our method will work fine and give as back what we need but it does not perform any asynchronies logic inside. If we would like to implement some delay in the response, for example, using a setTimeout inside this function let’s see what would happen:
在此示例中,我们可以看到我们的方法可以正常工作并返回所需的信息,但它内部不执行任何异步逻辑。 如果我们想在响应中实现一些延迟,例如,在此函数内使用setTimeout,我们将看到会发生什么:
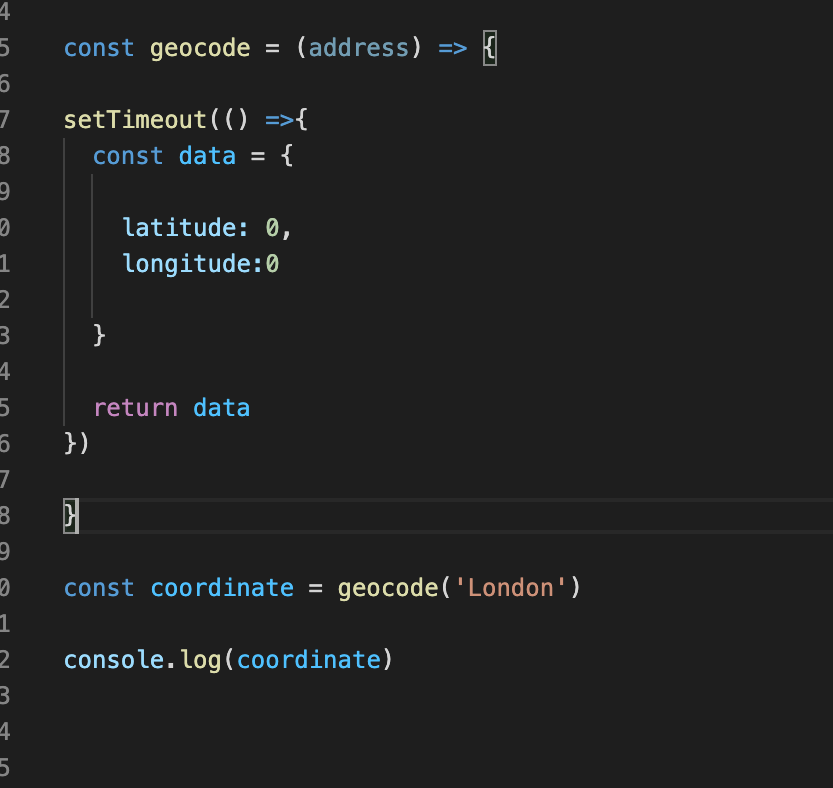
If you run the above function your return value will be undefined. The problem is that the main function does not return anything and even if the setTimeout has a return value the main one run immediately and has a return value of undefined because the data returned are inside a nested function. This is why the return pattern is not going to work when we try to do asynchronous action inside the main function.
如果运行上述函数,则返回值将是不确定的。 问题在于主函数不会返回任何内容,即使setTimeout具有返回值,主函数也会立即运行并且返回值未定义,因为返回的数据在嵌套函数内部。 这就是为什么当我们尝试在主函数中执行异步操作时返回模式将不起作用的原因。
Using a callback function as an argument
使用回调函数作为参数
let’s see how this function would perform with a call back function passed as an argument:
让我们看一下该函数与作为参数传递的回调函数一起执行的情况:
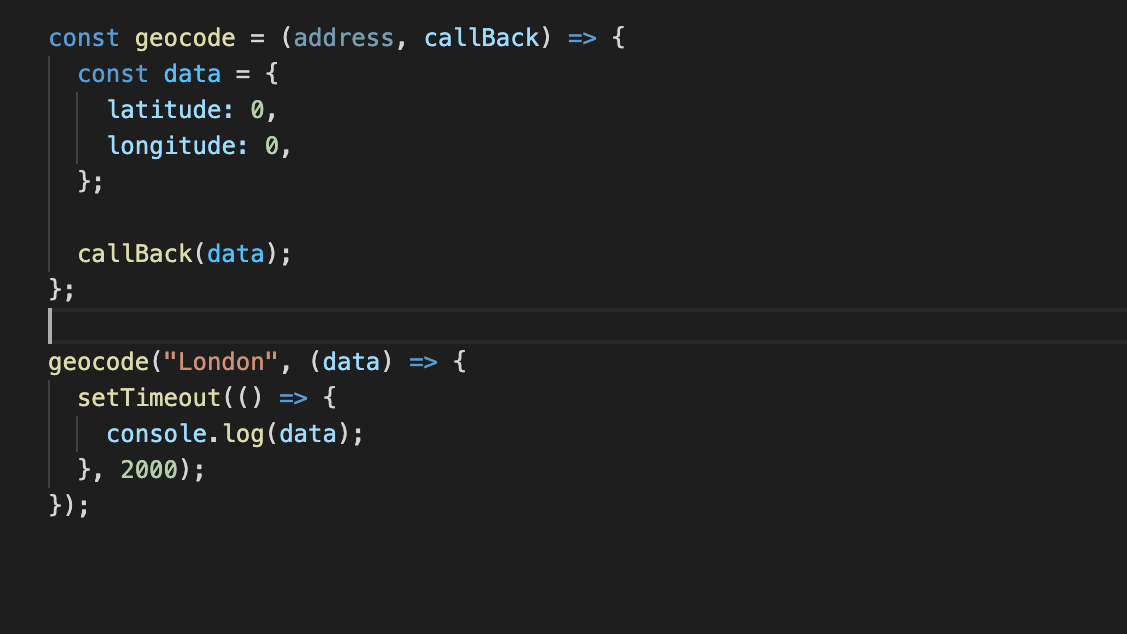
In the above example we passed a call back function as the second argument, and instead, to give a return value we passed the data inside the call back function. When we call the function in our app this will perform the Asychrones operation that allows us to get the data we want and perform later on a different operation, for example, call setTimeout and display the data after 2 seconds.
在上面的示例中,我们传递了一个回调函数作为第二个参数,相反,为了提供返回值,我们在回调函数内部传递了数据。 当我们在应用程序中调用该函数时,它将执行Asychrones操作,该操作使我们能够获取所需的数据,并稍后在其他操作上执行,例如,调用setTimeout并在2秒后显示数据。
The callback pattern gives us more control on the return value of the function called, and allow us to perform different operation every time we call the function without to be restricted on the return value defined inside the main function. If we want to just perform different operation on the return value we can change it every time we called inside a different file.
回调模式使我们能够更好地控制所调用函数的返回值,并允许我们每次调用函数时都执行不同的操作,而不受限于在主函数内部定义的返回值。 如果我们只想对返回值执行不同的操作,则可以在每次在不同文件中调用时更改它。
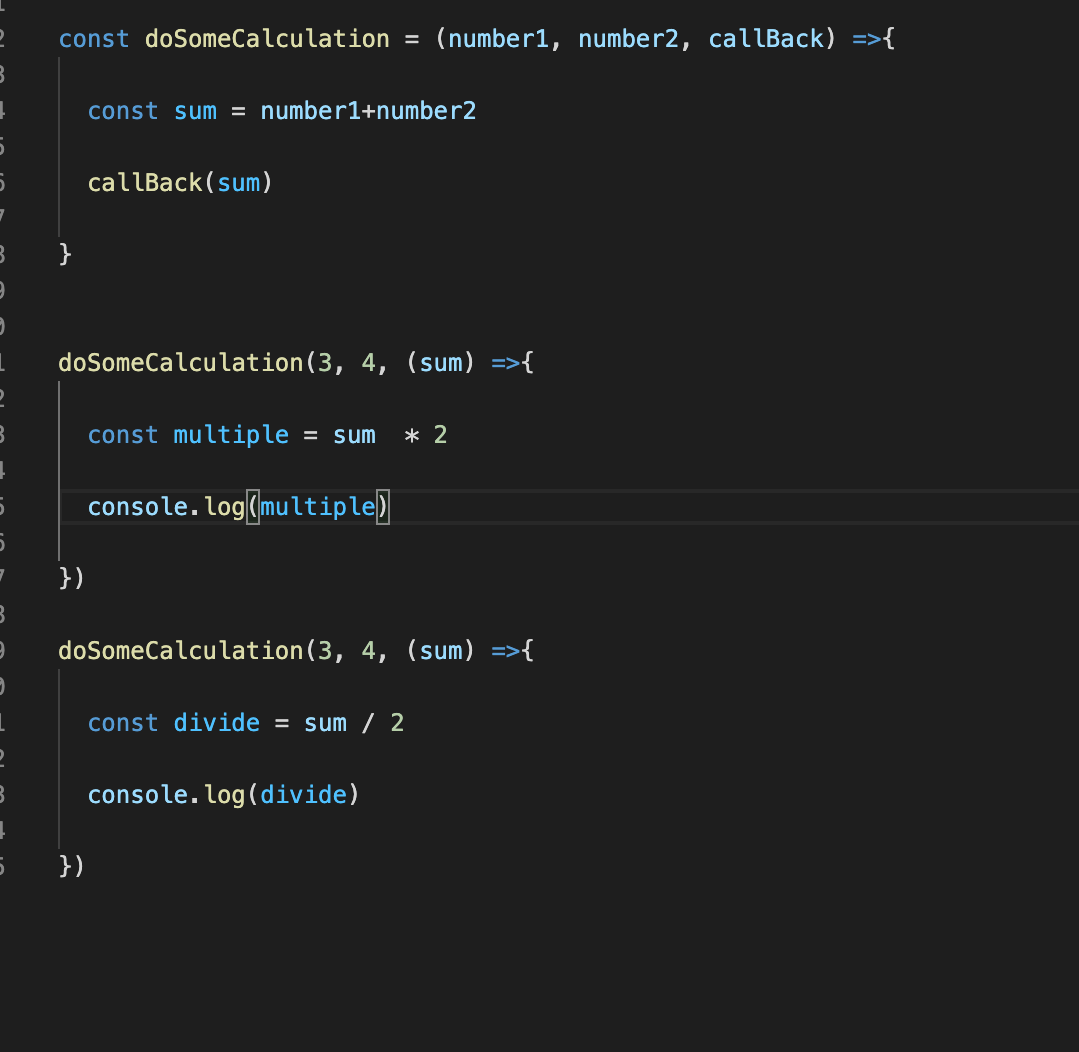
In the above example, we defined a function “doSomeCalculation()”, that takes two number and a call back function as arguments. When we call the function below we can perform a different operation with the return value, for example, multiply or divide with a different value every time, without being restricted in defining the operation inside the main function.
在上面的示例中,我们定义了一个函数“ doSomeCalculation()”,该函数以两个数字和一个回调函数作为参数。 当我们调用下面的函数时,我们可以对返回值执行不同的操作,例如,每次都用不同的值乘或除,而不受限于在主函数内部定义操作。
I hope this blog helped you to have a more clear idea of why and how we use the call back function in our code.
我希望这个博客可以帮助您更清楚地了解为什么以及如何在代码中使用回调函数。
翻译自: https://medium.com/@musta7188/call-back-function-javascript-fa32cc7890b2