java中字符串常量池
重点(Top highlight)
Java中的字符串池是什么?(What is String Pool in Java?)
It is a Pool of strings stored in heap memory. All the strings created are stored in this pool if they do not already exist in the pool.
它是存储在堆内存中的字符串池。 如果尚未创建的所有字符串都存储在该池中存在在游泳池。
String class is immutable class and hence all string objects created using literals or new operators cannot be changed/modified .
字符串类是不可变的类,因此不能更改/修改使用文字或新运算符创建的所有字符串对象。
字符串对象以两种方式创建: (String objects are created in two ways :)
1.使用双引号[使用字符串文字时] (1. Using double quotes [when String literals are used ])
String name1 = “Java”;
The above statement creates a string object and places it in a string pool if it is not already present in the string pool and a reference is assigned to name1.
上面的语句创建一个字符串对象,并将其放置在字符串池中(如果字符串池中尚不存在该对象,并将引用分配给name1)。
In this case, only one object is created.
在这种情况下,仅创建一个对象。
2. Using the ‘new’ keyword :
2.使用'new'关键字:
String name2 = new String(“newJava”);
The above statement creates a string object in heap memory and checks whether it is present in the string pool or not. If the “newJava” is not present in the string pool then it will place this string in the string pool else it will skip it.
上面的语句在堆内存中创建一个字符串对象,并检查它是否存在于字符串池中。 如果字符串池中不存在“ newJava ”,则它将把该字符串放在字符串池中,否则它将跳过它。
In this case, two objects are created. One in heap memory and the other in the String pool.
在这种情况下,将创建两个对象。 一个在堆内存中,另一个在字符串池中。
Let’s take another example and understand it programmatically and diagrammatically.
让我们再举一个例子,以编程方式和图表方式理解它。
public class Main {
public static void main(String[] args) {
/*
creates string object in string Pool
*/
String name1 = "Java";
/*
Does not create string object in string pool as this "Java"
literal already present there
*/
String name2 = "Java";
/*
name3 and name1 are pointing to same string literal "Java"
*/
String name3 = name1;
/*
creates new object in heap and does not create new object
in string pool as it already present there
*/
String name4 = new String("Java");
/*
creates new object in heap and does not create new object
in string pool as it already present there
*/
String name5 = new String("Java");
System.out.println(name1 == name2);//prints true
System.out.println(name1 == name3);//prints true
System.out.println(name2 == name3);//prints true
System.out.println(name1 == name4);//prints false
System.out.println(name1 == name5);//prints false
System.out.println(name4 == name5);//prints false
}
}
输出:(OUTPUT :)
truetruetruefalsefalsefalse
上面的代码以图表形式转换为: (Diagrammatically the above code translates to :)
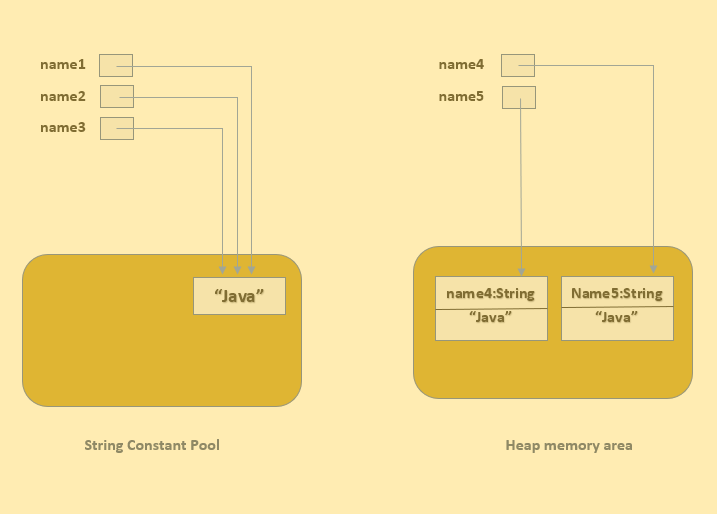
注意 :(Note :)
String literals are stored in a String constant pool. Strings with same content share this common memory area to reduce memory usage . String objects created with new operator are stored in the heap memory area and no sharing of storage for the same contents of different objects.
字符串文字存储在字符串常量池中。 具有相同内容的字符串共享此公共内存区域以减少内存使用。 使用new运算符创建的字符串对象存储在堆内存区域中,不同对象的相同内容不会共享存储。
在上面的程序中创建了多少个对象? (How many objects are created in the above program?)
The answer for this question will be 3.
这个问题的答案将是3 。
As you can see that there is one object in string constant pool which is created when we used string literal for name1 as at that time there wasn’t this ”Java” string present in string constant pool and for the next string that is name2, the same string already present so no new object created instead the same reference returned for this string and for name3, same reference of name1 would be assigned.
如您所见,在字符串常量池中有一个对象是在我们为name1使用字符串文字时创建的,因为那时在字符串常量池中以及下一个名为name2的字符串中都没有此“ Java”字符串,相同的字符串已经存在所以没有新的对象,而不是创建相同的参考此字符串和NAME3返回,同样参考NAME1将被分配。
Two objects are there in heap memory for each new operator and no objects would be created in string constant pool as this ”Java” string already present in string pool constant.
每个新运算符的堆内存中都有两个对象,并且不会在字符串常量池中创建任何对象,因为此“ Java”字符串已经存在于字符串池常量中。
我们如何检查相同或不同的字符串内容,以及两个字符串是否指向同一对象? (How can we check string contents that are the same or not and two strings points to the same object or not?)
A string function equals(Object obj) can be used to compare the two strings contents and == operator can be used to compare whether two string references are the same or not.
字符串函数equals(Object obj)可用于比较两个字符串的内容, ==运算符可用于比较两个字符串引用是否相同。
范例: (Example :)
String string1 = new String(“Hey Java”);String string2 = “Hey Java”;System.out.println(string1.equals(string2));//prints trueSystem.out.println(string1 == string2);//prints false
string1.equals(string2) checked the content of two string objects and hence returned true as they have same value “Hey Java” whereas string1 == string2 returned false as string1 is referring object in heap and string2 referring object from string constant pool.
string1.equals(string2)检查了两个字符串对象的内容,因此返回true,因为它们具有相同的值“ Hey Java”,而string1 == string2返回false,因为string1引用堆中的对象,而string2引用字符串常量池中的对象。
While using equals() function for object’s content comparison make sure that reference through which you call equals() method is not null otherwise you will get NullPointerException .
在使用equals()函数进行对象的内容比较时,请确保通过其调用equals()方法的引用不为null,否则将获得NullPointerException 。
intern()方法有什么作用? (What does the intern() method do?)
intern() returns a canonical representation for the string object.When the intern method is invoked, if the string pool already contains a string equal to this String object(the object with which intern method will be called) as determined by the equals(Object) method, then the string from the pool is returned. Otherwise, this String object is added to the pool, and a reference to this String object is returned.
intern()返回字符串对象的规范表示形式。调用intern方法时,如果字符串池中已经包含等于此String对象(将调用其intern方法的对象)的字符串,则由equals(Object )方法,然后返回池中的字符串。 否则,将此String对象添加到池中,并返回对此String对象的引用。
Example :
范例:
public class Main {
public static void main(String[] args) {
String string1 = "Java";
String string2 = string1.intern();
System.out.println(string1 == string2);
}
}
输出:(OUTPUT :)
true
In the above example at line number 6 “Java” was not present in the string pool and hence string object is created in the string pool and the reference of this object is returned to string1 variable. At line number 7 when string1.intern() is called it looked into the string pool and there was already a string present and hence the reference of that string object is returned to string2. Hence the output of the program is true.
在上面的示例中,第6行在字符串池中不存在“ Java ”,因此在字符串池中创建了字符串对象,并且该对象的引用返回给string1变量。 在第7行,当调用string1.intern()时,它查看字符串池,并且已经存在一个字符串,因此对该字符串对象的引用将返回给string2 。 因此,程序的输出为true 。
Let’s stop here for this article. I hope you have understood the string pool and how objects are created using string literals and the new operator.
让我们在这里停止。 我希望您了解字符串池以及如何使用字符串文字和new运算符创建对象。
觉得这篇文章有用吗? 关注我,获取更多此类翔实的文章。 (Found this article useful ?? follow me for more such informative articles.)
您可以查看其他可能对您有所帮助的Java文章。 (You can check out my other Java articles which might help you.)
翻译自: https://medium.com/javarevisited/what-does-string-pool-mean-in-java-414c725fbd59
java中字符串常量池