pythonic
Python is an object-oriented, as well as the scripting programming language, It is an interpreted, powerful, and syntactically easy language.
Python是一种面向对象的语言,也是一种脚本编程语言,它是一种解释性,功能强大且在语法上易于使用的语言。
It is known to everyone that Python gives an easy syntax for complicated coding logic, which enhances the productivity of Python programmers.
众所周知,Python为复杂的编码逻辑提供了一种简单的语法,从而提高了Python程序员的工作效率。
This is true that every programmer has their thought process and way of thinking to build the code logic, If we give one problem statement to many programmers then It is for sure that we will get many different solutions for the same problem.
每个程序员都有自己的思维过程和思维方式来构建代码逻辑,这是事实。如果我们向许多程序员给出问题陈述,那么可以肯定的是,对于同一问题,我们将获得许多不同的解决方案。
I observed it happens more with Python coding because of Python built-in features and its different modules which hides all the complexity and gives an easy solution. so more ways will be available to write the same code logic but only one way will be obvious and perfect for the specific problem.
我观察到,由于Python的内置功能及其不同的模块,这种情况在Python编码中发生的更多,从而隐藏了所有复杂性并提供了简单的解决方案。 因此将有更多方法可以编写相同的代码逻辑,但是只有一种方法对于特定问题是显而易见的和完美的。
The below lines are from Zen of python and I like this one more.
以下几行来自python的Zen,我更喜欢这一行。
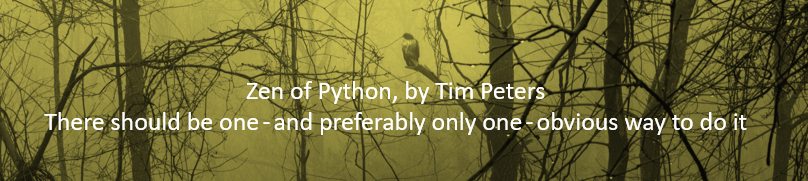
The more obvious way is something like you should write the code in that way instead of any other. For Python coding, the most obvious way is nothing but the Pythonic way.
更明显的方法是您应该以这种方式而不是其他方式编写代码。 对于Python编码,最明显的方法就是Pythonic方法。
If the code is not written in a pythonic way it means we don’t use Python’s capability and its features with our programming skills. It looks unfair with Python language that we write the Python code which is not Pythonic.
如果代码不是以pythonic方式编写的,则意味着我们不将Python的功能及其功能与我们的编程技能结合使用。 使用Python语言看起来不公平,因为我们编写的不是Python语言的Python代码。
什么是Python方式: (What is a Pythonic way:)
The Pythonic way is described as an easy way of writing code by so many programmers. I am just adding my thoughts on the same.
许多程序员将Pythonic方法描述为一种编写代码的简便方法。 我只是在加我的想法。
The Pythonic way is using the capability and Its features in Python’s style of coding.
Python的方式是以Python的编码风格使用该功能及其功能。
Python has a set of guidelines given with PEP 8, It is most important to look at the PEP 8 guidelines and what PEP 8 recommends for coding in Python.
Python具有PEP 8附带的一组准则。最重要的是要查看PEP 8准则以及PEP 8建议的Python编码建议。
尝试“ this”:打开Terminal> Go Python shell>编写“ import this”> Enter (Try “this”: Open Terminal > Go Python shell> write “import this” > Enter)
It will show the zen of python by Tim Peters, please read It, You will get an idea of why PEP 8 is important for learning more Pythonic coding styles.
它将显示蒂姆·彼得斯(Tim Peters)的python禅,请仔细阅读,您将了解为什么PEP 8对于学习更多Pythonic编码样式很重要。
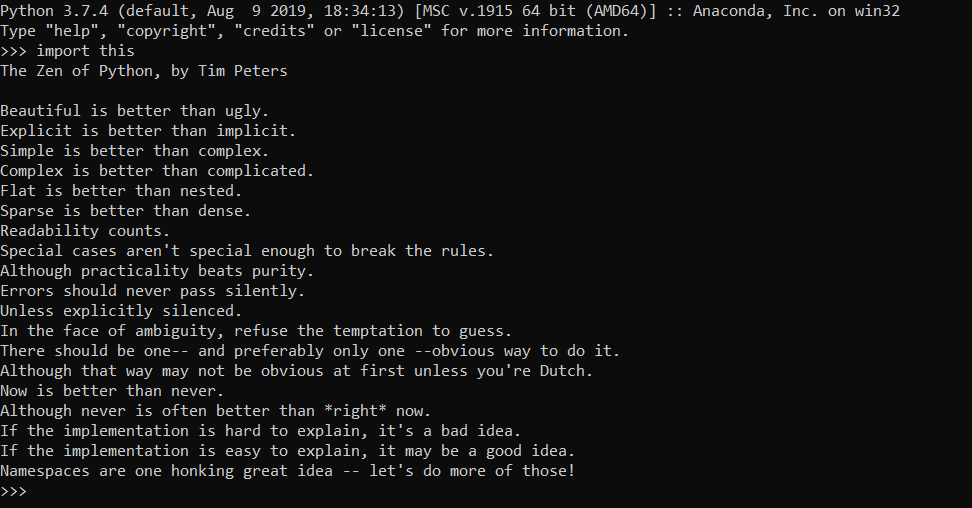
The above picture shows the output of “import this”, The Zen of Python, All are not just for Python it’s goes with all programming languages.
上图显示了“ import this”(Python的Zen)的输出,这不仅适用于Python,而且适用于所有编程语言。
Here are the PEP 8 guides please go through without failing!!!
这是PEP 8指南,请务必通过检查!!!
通过示例学习Pythonic: (Learning Pythonic way by example:)
This example might be giving you the possible thought process for you to write the Python code in a Pythonic way, I am using a simple logical programming example, many beginners may face this as one of the logical questions in their first job interview. For this programming example, we can write different solutions and can see which solution is more pythonic.
这个示例可能为您提供了一种可能的思路,使您可以用Python的方式编写Python代码。我使用的是一个简单的逻辑编程示例,许多初学者可能在初次面试时就将其作为逻辑问题之一。 对于此编程示例,我们可以编写不同的解决方案,并查看哪种解决方案更适合pythonic。
问题陈述:在未排序的列表中找到最大的数字。 (Problem statement: Find the biggest number in an unsorted list.)
解决方案1:搜索方式 (Solution#1: The searching way)
numbers = [1, 5, 9, 7, 7, 8, 4, 10, 4, 12, 16, 7, 89, 27, 67]
def get_max(l):
m = l[0]
for x in l:
if x > m:
m = x
return m
print(get_max(numbers))
This is a generic logic that can be implemented not only for Python but in any other programming language, and we have skipped using any built-in function provided by Python.
这是一种通用逻辑,不仅可以在Python中实现,而且可以在任何其他编程语言中实现,并且我们已经跳过了使用Python提供的任何内置函数的操作。
解决方案2:排序方式 (Solution#2: The sorting way)
The one possible solution for the above problem is “sorting of the list” and get the first or last number from the list according to the sorting order.
解决上述问题的一种可能的方法是“对列表进行排序”,并根据排序顺序从列表中获取第一个或最后一个数字。
numbers = [1, 5, 9, 7, 7, 8, 4, 10, 4, 12, 16, 7, 89, 27, 67]
# sorting in ascending order
numbers.sort()
# last number accessing
print(numbers[len(numbers) - 1])
Sorting even can give a little more brainstorming to programming beginners if it is not done by list.sort() method.
如果未通过list.sort()方法进行排序,则甚至可以对编程初学者进行更多的头脑风暴。
解决方案#3 Python方式 (Solution#3 The Pythonic way)
numbers = [1, 5, 9, 7, 7, 8, 4, 10, 4, 12, 16, 7, 89, 27, 67]
print(max(numbers))
Here max() is a built-in function that takes care of all logic and gives the max number from an iterator, So if we have this function in Python we can finish the logic in two lines.
这里的max()是一个内置函数,它处理所有逻辑并从迭代器中提供最大数目,因此,如果我们在Python中具有此函数,则可以分两行完成逻辑。
Yes, The pythonic way provides the solutions with hiding the complexity of internal logic. It is my opinion that if you are beginners of programming then for opting python as the first programing language is good no doubt.
是的,pythonic方式为解决方案提供了隐藏内部逻辑的复杂性。 我认为,如果您是编程的初学者,那么毫无疑问选择python作为第一种编程语言是不错的选择。
I have two points as suggestions!!!
我有两点建议!!!
If you are learning your first programming language: It is good to start with logical brainstorming and end with using Python power with learning Pythonic way. It is really important for only those who are learning Python or any other language as the first programing language.
如果您正在学习第一门编程语言:最好从逻辑头脑风暴开始,最后以学习Python语言方式使用Python功能结束。 对于只有那些学习Python或其他任何语言作为第一门编程语言的人来说,这确实很重要。
If you already practiced any programming language: giving up on generic logic and direct looking for a pythonic way is a good approach.
如果您已经练习过任何编程语言:放弃通用逻辑并直接寻找pythonic方式就是一个好方法。
The Pythonic way approach is a real use of Python capability and even It will make you aware of many Python concepts, built-in functions, and modules that you might not use so far.
Python方式是对Python功能的真正使用,甚至可以使您了解到目前为止可能尚未使用的许多Python概念,内置函数和模块。
Leaning the Pythonic way does not require more and more writing code, It is exploring the Python features and modules and using them.
靠Python的方式不需要越来越多的编写代码,它正在探索Python的功能和模块并使用它们。
I have collected a few Python topics with the code examples, these examples are might look very simple problems but It might be a small code of your thousands of lines of software code.
我已经用代码示例收集了一些Python主题,这些示例可能看起来很简单,但可能只是数千行软件代码中的一小部分。
Here is the blog where I collected code examples to learn The Pythonic way please go through and do hands-on and most important don’t wait to read the PEP 8 guidelines.
这是我收集代码示例以学习Python风格的博客,请逐步进行动手操作,最重要的是不要等待阅读PEP 8指南。
Happy coding!! Thanks.
编码愉快!! 谢谢。
pythonic