aws dynamodb
In this tutorial, I will walk you through how to set up endpoints using API Gateway to trigger Lambda functions which interact with your DynamoDB database using the boto3 Python library. I will upload a future tutorial explaining how to write a Lambda function to communicate with RDS instead of DynamoDB using pymysql and Lambda layers.
在本教程中,我将指导您如何使用API Gateway设置端点以触发Lambda函数,这些函数使用boto3 Python库与DynamoDB数据库进行交互。 我将上传一个未来的教程,说明如何使用pymysql和Lambda层编写Lambda函数以与RDS进行通信,而不是DynamoDB。
1. AWS账户设置 (1. AWS Account Setup)
If you already have an AWS account setup, please skip ahead to section 2. If you do not, you can sign up for an account here. You will be required to enter payment details but you get 12 months of free tier access when signing up and nothing in this tutorial will exceed free tier limits.
如果您已经设置了AWS账户,请跳至第2部分。如果没有,请在此处注册一个账户。 您将需要输入付款明细,但注册时可获得12个月的免费套餐访问权限,本教程中的任何内容都不会超出免费套餐限制。
Note: If you have exceeded your free tier usage for your account already, you may be charged for the usage of the AWS services described in this tutorial.
注意:如果您的帐户已超过免费套餐使用量,则可能会因使用本教程中所述的AWS服务而向您收费。
2.设置DynamoDB (2. Set up DynamoDB)
After logging in to the AWS Console, search for DynamoDB from the list of services. Click “Create table” in the Getting Started screen.
登录到AWS控制台后,从服务列表中搜索DynamoDB。 在“入门”屏幕中,单击“创建表”。
Note: DynamoDB instances are specific to the AWS region selected in the top right of the AWS console window. Ensure you have the correct region selected before creating your table (this is typically the region that is geographically closest to you).
注意: DynamoDB实例特定于在AWS控制台窗口右上方选择的AWS区域。 在创建表之前,请确保选择了正确的区域(这通常是地理上距离您最近的区域)。
In the Create DynamoDB table window, enter a name for your table and a primary key. You can also optionally specify a sort key if you want a composite primary key for your table. In this example, I have created a Playlist table with SongName as the primary key and Artist as the sort key. You can accept the default settings.
在“创建DynamoDB表”窗口中,输入表的名称和主键。 如果要为表使用复合主键,则还可以选择指定排序键。 在此示例中,我创建了一个播放列表表,其中SongName作为主键,Artist作为排序键。 您可以接受默认设置。

Once your table is created, select the Items tab for your table and create a few items to be stored in your database table. When creating an item in your table, you can also specify more fields on the item in addition to the two key fields. In this example, some of the items additionally specify the Album field. You have now successfully created your DynamoDB table which we can query from your Lambda function.
创建表后,选择表的“项目”选项卡,然后创建一些要存储在数据库表中的项目。 在表中创建项目时,除了两个关键字段之外,您还可以在该项目上指定更多字段。 在此示例中,某些项目还指定了“相册”字段。 您现在已经成功创建了DynamoDB表,我们可以从Lambda函数中查询该表。
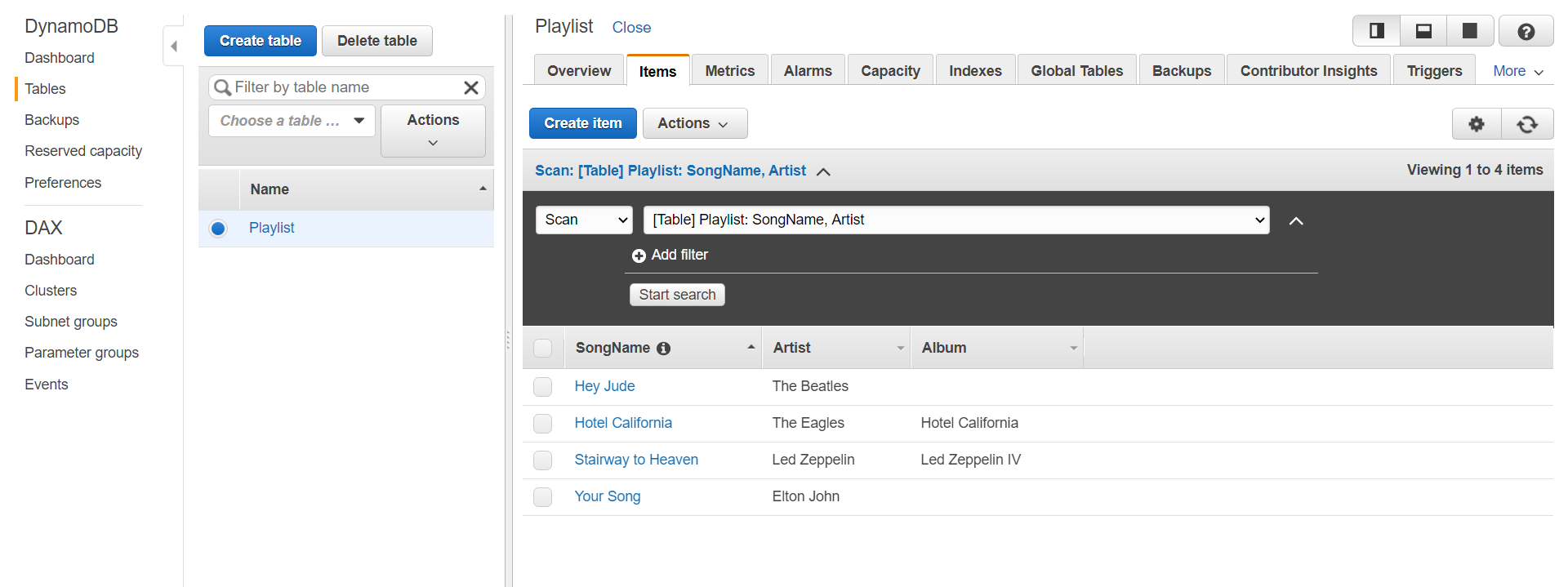
3.创建您的Lambda函数 (3. Create your Lambda function)
Now it’s time to set up your Lambda function. Open the Lambda service and ensure the Functions dashboard is selected. I recommend creating your Lambda function in the same region as the DynamoDB you have already created.
现在是时候设置Lambda函数了。 打开Lambda服务,并确保选择了功能仪表板。 我建议在与已经创建的DynamoDB相同的区域中创建Lambda函数。
Select “Create function” and give your function a name, I have called the function ‘get-playlist’ in this tutorial. Use Python 3.8 as the Runtime and leave “Create a new role with basic Lambda permissions” as the Execution role. Create your function.
选择“创建函数”并为您的函数命名,在本教程中,我将函数称为“获取播放列表”。 使用Python 3.8作为运行时,并保留“使用Lambda基本权限创建新角色”作为执行角色。 创建您的功能。

Add the following as your function code and Save. When the function is executed, the lambda_handler method is performed and returns a HTTP response which will be consumed by API Gateway. The code imports the boto3 library and creates the dynamodb resource, the scan() function is then called on the Playlist table to return all data from the table and sets the list of items in the table as the HTTP response body.
将以下内容添加为您的功能代码并保存。 执行该函数时,将执行lambda_handler方法并返回HTTP响应,该响应将被API Gateway占用。 该代码导入boto3库并创建dynamodb资源,然后在播放列表表上调用scan()函数以返回表中的所有数据,并将表中的项目列表设置为HTTP响应主体。
import json
import boto3dynamodb = boto3.resource('dynamodb')
def lambda_handler(event, context):
table = dynamodb.Table('Playlist')
body = table.scan()
items = body['Items'] return {
'statusCode': 200,
'headers': {
'Access-Control-Allow-Origin': '*',
'Access-Control-Allow-Headers': 'Content-Type,X-Amz-Date,Authorization,X-Api-Key,X-Amz-Security-Token',
'Access-Control-Allow-Credentials': 'true',
'Content-Type': 'application/json'
},
'body': json.dumps(items)
}
The Lambda function will not work just yet, we must give the function permissions to perform actions on our DynamoDB table. In your Lambda function, select the Permissions tab. You will see a default Execution role has been created for you. Click on the Role name to open the role in the IAM console. In the IAM console, attach the ‘AmazonDynamoDBFullAccess’ policy to your role and save.
Lambda函数目前还无法正常工作,我们必须授予该函数权限才能在DynamoDB表上执行操作。 在您的Lambda函数中,选择“权限”选项卡。 您将看到已为您创建了默认的执行角色。 单击角色名称以在IAM控制台中打开该角色。 在IAM控制台中,将“ AmazonDynamoDBFullAccess”策略附加到您的角色并保存。
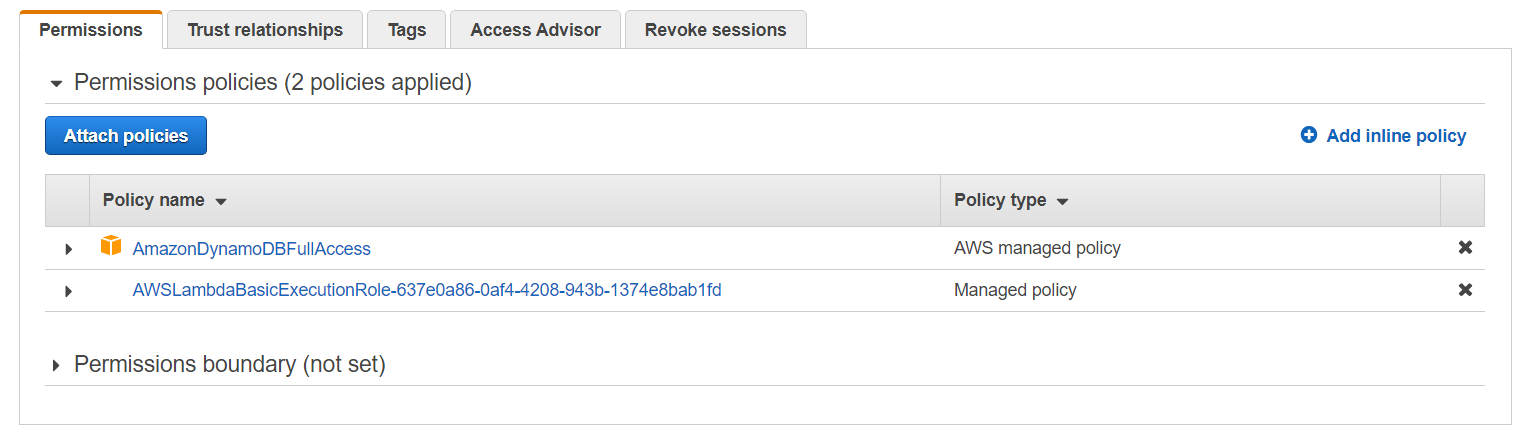
Now you can test your Lambda function. In the top right of your Lambda function console window, select the ‘Select a test event’ drop down menu and click ‘Configure test events’. AWS comes with many test event templates already configured, select the Amazon API Gateway AWS Proxy template and give your test event a name and save. Click on the ‘Test’ button and verify that your function executes successfully.
现在,您可以测试Lambda函数。 在Lambda功能控制台窗口的右上角,选择“选择测试事件”下拉菜单,然后单击“配置测试事件”。 AWS附带了许多已配置的测试事件模板,请选择Amazon API Gateway AWS Proxy模板,并为您的测试事件命名并保存。 单击“测试”按钮,并验证您的功能是否成功执行。

We are almost finished with our Lambda function set up. The last thing to do is set up the API Gateway trigger.
我们的Lambda函数设置几乎完成。 最后要做的是设置API网关触发器。
4. API网关触发器 (4. API Gateway Trigger)
In the Designer section of the Configuration tab of your Lambda function, click the ‘Add Trigger’ button. Set the Trigger configuration as shown below. The Security option can be changed from API key to Open or IAM depending on your desired security level, but I am choosing API key for this example. This will generate an API key which will be used when calling the API endpoint. Click ‘Add’ to add this trigger for your Lambda function. This will automatically create an API for you in the API Gateway console.
在Lambda函数的“配置”选项卡的“设计器”部分中,单击“添加触发器”按钮。 如下所示设置触发器配置。 可以根据需要的安全级别将“安全性”选项从“ API密钥”更改为“打开”或“ IAM”,但是在此示例中,我选择的是API密钥。 这将生成一个API密钥,该密钥将在调用API端点时使用。 单击“添加”为您的Lambda函数添加此触发器。 这将在API Gateway控制台中自动为您创建一个API。
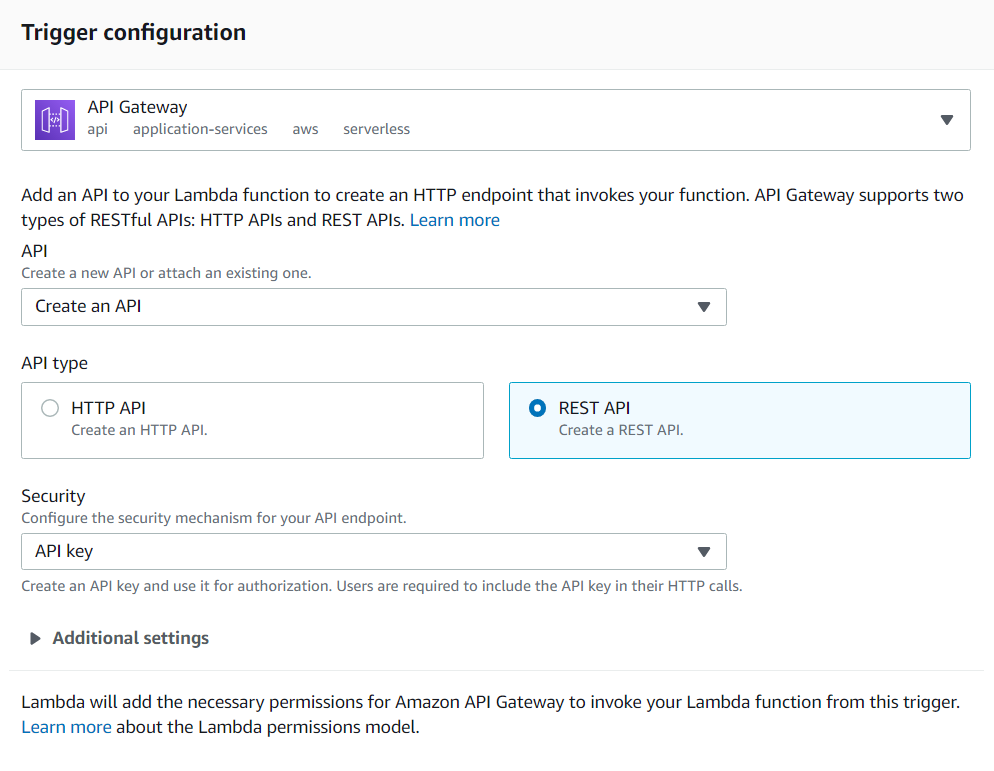
Navigate to API Gateway in the AWS console and you will see an API created for your Lambda function.
导航到AWS控制台中的API Gateway,您将看到为Lambda函数创建的API。

Select your API from the list and click on ANY under the Resource path, which is ‘/get-playlist’ in this case. This will open Method Execution information in the right of your window.
从列表中选择您的API,然后单击“资源”路径下的“ ANY”(在本例中为“ / get-playlist”)。 这将在窗口右侧打开方法执行信息。

Click on the TEST lightning bolt icon, select GET for the Method and click TEST. You will now see the contents of your Playlist DynamoDB table.
单击“测试”闪电图标,为“方法”选择“获取”,然后单击“测试”。 现在,您将看到播放列表DynamoDB表的内容。
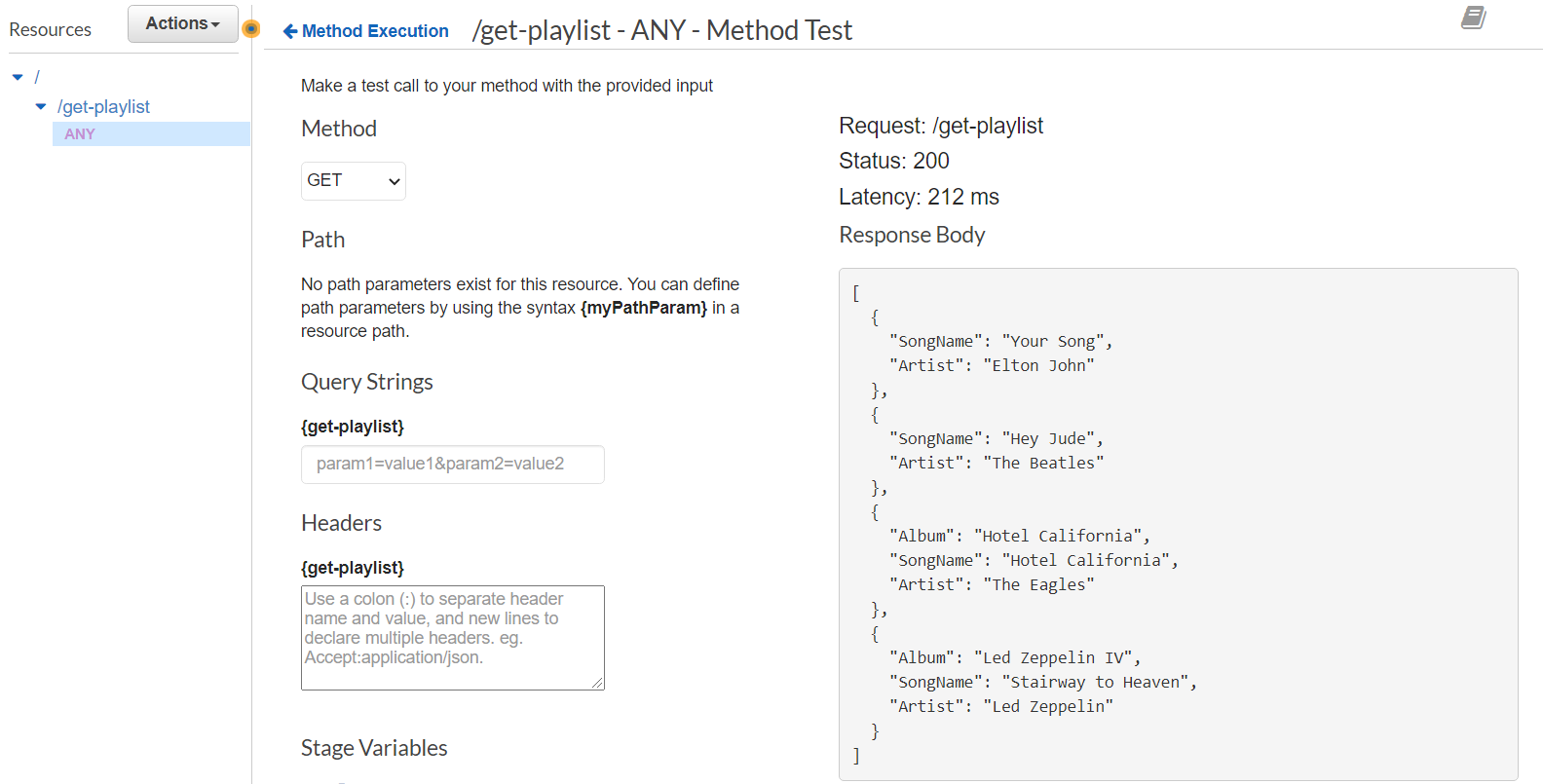
If you want to deploy your API endpoint so that it can be invoked from elsewhere, such as your front-end application, select the ‘/get-playlist’ resource and under Actions, select Deploy API. Choose the default Deployment stage and click Deploy. Once deployed, select the GET method under the ‘/get-playlist’ resource, this will give you the URL which can be used to invoke your API.
如果要部署API端点以便可以从其他地方(例如前端应用程序)调用它,请选择“ / get-playlist”资源,然后在“操作”下选择“部署API”。 选择默认的部署阶段,然后单击部署。 部署后,在“ / get-playlist”资源下选择GET方法,这将为您提供可用于调用API的URL。

Since we set the Security setting for our API to be API key, we will need to add the x-api-key header to our HTTP request when invoking the URL. This can be found by selecting API Keys from the menu on the left side of the page and choosing your API from the list. The Show button will reveal your API key.
由于我们将API的“安全性”设置设置为API密钥,因此在调用URL时,我们需要将x-api-key标头添加到HTTP请求中。 可以通过从页面左侧的菜单中选择“ API密钥”并从列表中选择您的API来找到。 显示按钮将显示您的API密钥。
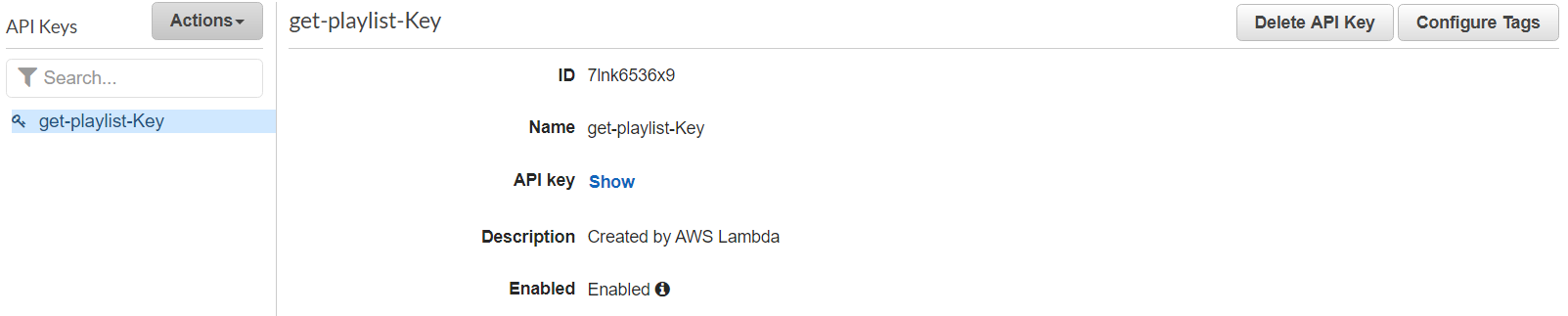
This can be tested using a tool such as Postman to invoke the API. Below is an example of calling the API endpoint using Postman with the x-api-key header added to the GET request. Postman adds some of the headers shown by default, enable the ‘Show/Hide Auto-Generated Headers’ option in Postman to show all the headers below.
可以使用诸如Postman之类的工具调用API进行测试。 以下是使用Postman调用API端点的示例,其中x-api-key标头已添加到GET请求中。 Postman添加默认显示的一些标题,启用Postman中的“显示/隐藏自动生成的标题”选项以显示下面的所有标题。
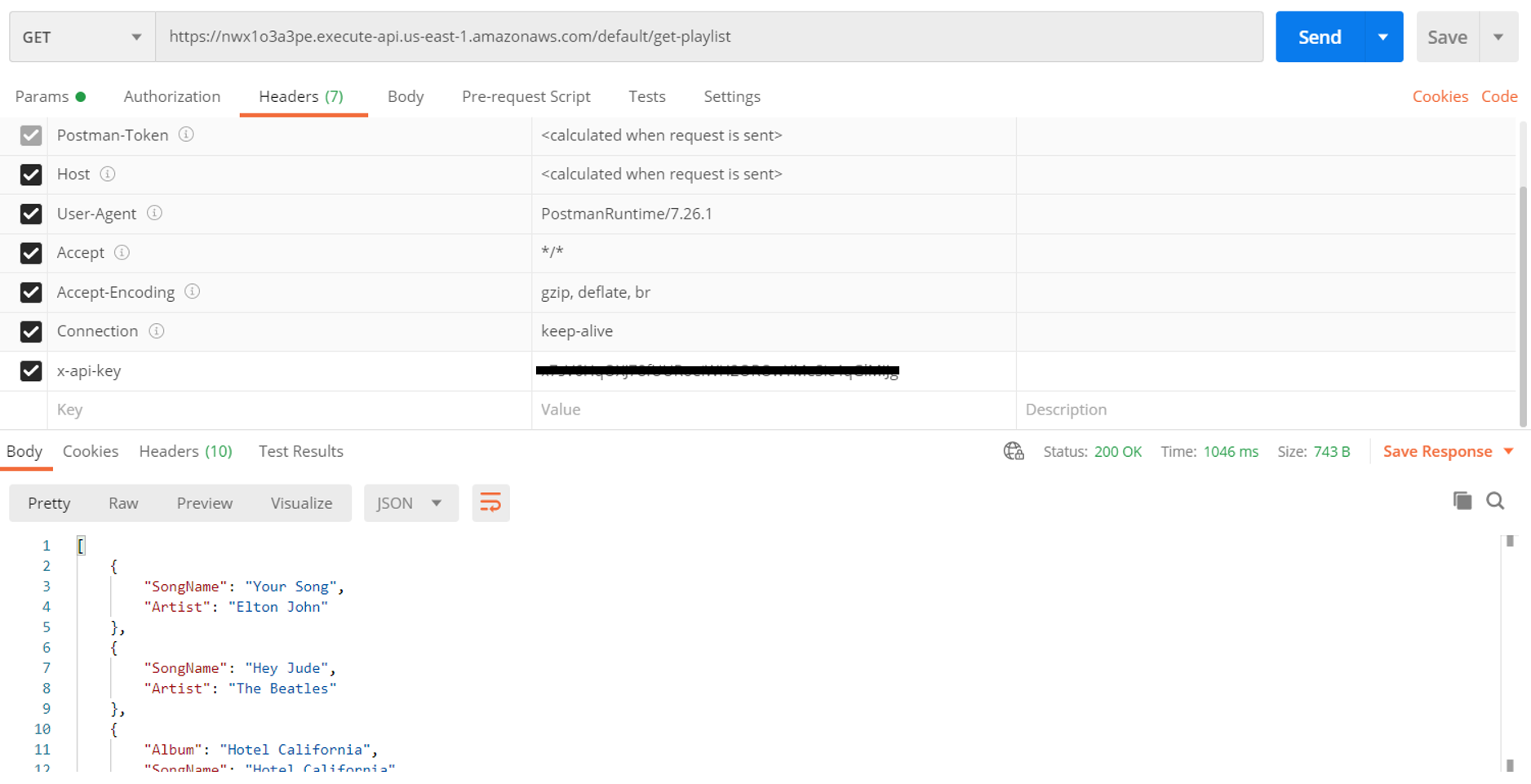
5.结论 (5. Conclusion)
We have now successfully created a simple DynamoDB database and set up an endpoint using API gateway which can be invoked to get the contents of our database.
现在,我们已经成功创建了一个简单的DynamoDB数据库,并使用API网关设置了端点,可以调用该端点来获取数据库的内容。
翻译自: https://medium.com/@odwyerc4/aws-api-gateway-lambda-dynamodb-71bb930abe51
aws dynamodb