com 初探
连接服务方面的挑战 (Challenges in connecting services)
When I think about my recent projects, I probably spent half of my time coding new services and the other half in connecting services. Service A calling Service B, or Service C calling an external service and using the result to feed into another Service D.
当我考虑我最近的项目时,我可能花费了一半时间来编写新服务,另一半用于连接服务。 服务A调用服务B,或服务C调用外部服务,并使用结果馈入另一个服务D。
Connecting services is one of those things that ‘should be easy’ but in reality, it takes a lot of time and effort. You need to figure out a common connection format for services to use, make the connection, parse the results, and pass the results on. I’m not even mentioning error handling, retries and all those production readiness type features that you ultimately need to do.
连接服务是“应该很容易”的事情之一,但实际上,这需要很多时间和精力。 您需要确定服务使用的通用连接格式,进行连接,解析结果并将结果继续传递。 我什至没有提到错误处理,重试以及最终需要做的所有生产准备类型功能。
That’s why I got really excited when I saw the following tweet from Filip Knapik talking about the beta availability of a new product called Workflows:
这就是为什么当我看到Filip Knapik的以下推文谈论一种名为Workflows的新产品的beta版可用性时,我感到非常兴奋的原因:
什么是工作流程? (What is Workflows?)
In a nutshell, Workflows allows you to connect ‘things’ together. What kind of things? Pretty much anything that has a public API. You can connect multiple Cloud Functions together, or mix-match Cloud Functions with Cloud Run services with Google Cloud APIs or even external APIs.
简而言之, 工作流使您可以将“事物”连接在一起。 什么样的东西 具有公共API的几乎所有东西。 您可以将多个Cloud Functions连接在一起,也可以将Cloud Functions与带有Google Cloud API甚至外部API的Cloud Run服务混合匹配。
In addition to connecting disparate services, Workflows handles parsing and passing values among services. It has built-in error handling and retry policies. And it’s serverless, scales seamlessly with demand with scaling down to zero.
除了连接不同的服务之外,工作流还处理服务之间的解析和传递值。 它具有内置的错误处理和重试策略。 而且它是无服务器的,可以按需无缝扩展到零。
This sounds great, let’s see what we can do with it!
这听起来很棒,让我们看看我们可以做什么!
连接两个云功能 (Connect two Cloud Functions)
As a first sample, let’s connect two Cloud Functions using Workflows.
作为第一个示例,让我们使用工作流连接两个Cloud Functions。
First service is randomgen. It generates a random number between 1–100:
第一服务是randomgen 。 它生成一个介于1到100之间的随机数:
import random, json
from flask import jsonifydef randomgen(request):
randomNum = random.randint(1,100)
output = {"random":randomNum}
return jsonify(output)
Deploy to Cloud Functions:
部署到云功能:
gcloud functions deploy randomgen \
--runtime python37 \
--trigger-http \
--allow-unauthenticated
Second service is multiply. It multiplies the received input by 2:
第二项服务是乘法 。 它将接收到的输入乘以2:
import random, json
from flask import jsonifydef multiply(request):
request_json = request.get_json()
output = {"multiplied":2*request_json['input']}
return jsonify(output)
Deploy to Cloud Functions:
部署到云功能:
gcloud functions deploy multiply \
--runtime python37 \
--trigger-http \
--allow-unauthenticated
Connect the two services using a first workflow. Create a workflow.yaml
file:
使用第一个工作流程连接两个服务。 创建一个workflow.yaml
文件:
- randomgenFunction:
call: http.get
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/randomgen
result: randomgenResult
- multiplyFunction:
call: http.post
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/multiply
body:
input: ${randomgenResult.body.random}
result: multiplyResult
- returnResult:
return: ${multiplyResult}
Notice how easy it is to call different services with just their url, parse the results of one and feed into the other service.
请注意,仅使用URL调用不同的服务,解析一个服务的结果并馈入另一个服务是多么容易。
Deploy the workflow:
部署工作流程:
gcloud beta workflows deploy workflow \
--source=workflow.yaml
Execute the workflow:
执行工作流程:
gcloud beta workflows execute workflow
This gives you a command to see the progress of your workflow. In my case the command is like this:
这为您提供了查看工作流程进度的命令。 就我而言,命令是这样的:
gcloud beta workflows executions describe bc0e0c53-2b77-438a-a2dc-824be577f5f9 \
--workflow workflow{
"body": {
"multiplied": 34
},
"code": 200,
"headers": {
...
Notice that we got a multiplied
value and status code 200
. Nice, our first workflow is up and running!
注意,我们得到了一个multiplied
数值和状态码200
。 好的,我们的第一个工作流程已启动并正在运行!
连接外部服务 (Connect an external service)
In the next sample, let’s connect to math.js as an external service in the workflow.
在下一个示例中,让我们作为工作流中的外部服务连接到math.js。
In math.js
, you can evaluate mathematical expressions like this:
在math.js
,您可以像这样评估数学表达式:
curl https://api.mathjs.org/v4/?expr=log(56)+4.02535169073515
This time, we’ll use Cloud Console to update our workflow. Find Workflows
in Google Cloud Console:
这次,我们将使用Cloud Console更新我们的工作流程。 在Google Cloud Console中查找Workflows
:

Go to our workflow and click on Definition
tab:
转到我们的工作流程,然后单击“ Definition
选项卡:
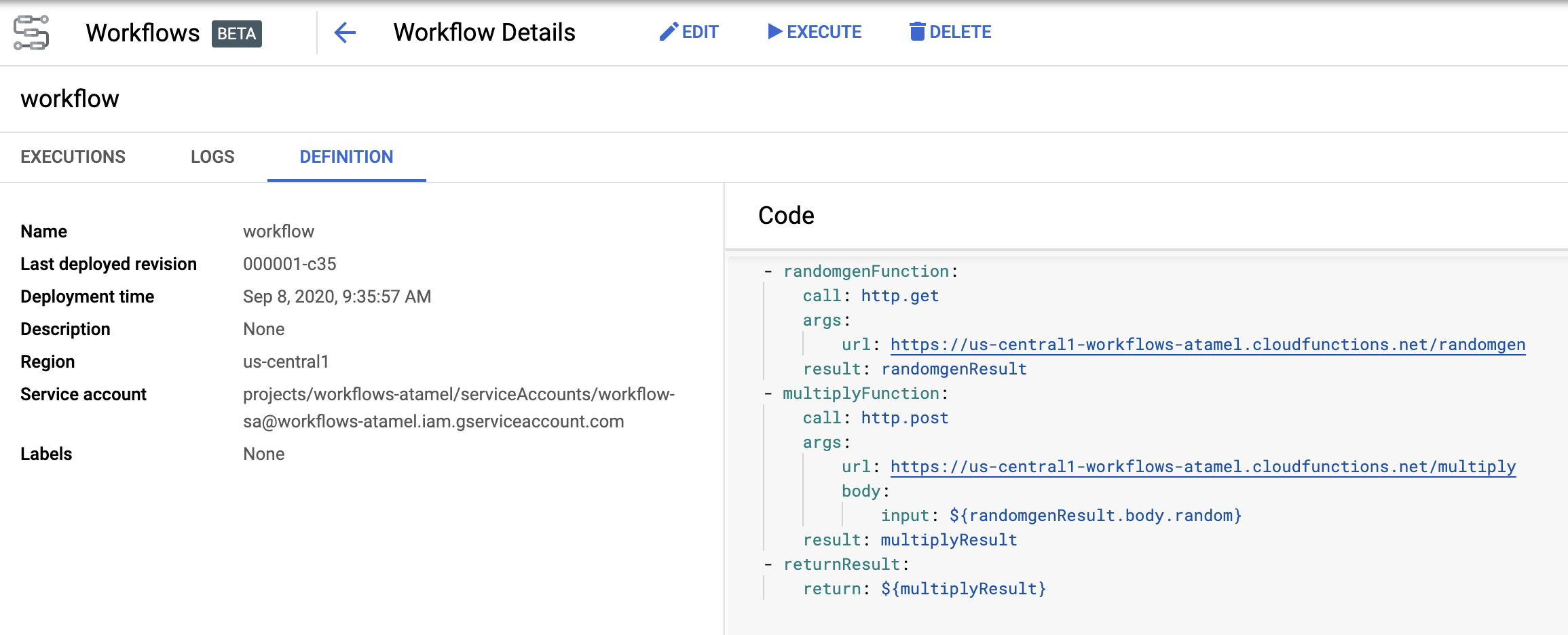
Edit the definition to include math.js
:
编辑定义以包括math.js
:
- randomgenFunction:
call: http.get
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/randomgen
result: randomgenResult
- multiplyFunction:
call: http.post
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/multiply
body:
input: ${randomgenResult.body.random}
result: multiplyResult
- logFunction:
call: http.get
args:
url: https://api.mathjs.org/v4/
query:
expr: ${"log(" + string(multiplyResult.body.multiplied) + ")"}
result: logResult
- returnResult:
return: ${logResult}
This will guide you to edit and deploy the workflow. Once done, click on Execute
to execute the workflow. You'll be presented with the details of the execution:
这将指导您编辑和部署工作流。 完成后,单击Execute
以执行工作流程。 您将看到执行细节:

Notice the status code 200
.
注意状态码200
。
We just integrated an external service into our workflow, super cool!
我们只是将外部服务集成到我们的工作流程中,非常酷!
连接经过身份验证的Cloud Run服务 (Connect an authenticated Cloud Run service)
In the last sample, let’s finalize our workflow with a call to a Cloud Run service. To make it more interesting, deploy an internal Cloud Run service. This means that the workflow needs to be authenticated to call the Cloud Run service.
在上一个示例中,让我们通过调用Cloud Run服务来完成我们的工作流程。 为了使其更加有趣,请部署内部Cloud Run服务。 这意味着需要对工作流进行身份验证才能调用Cloud Run服务。
The service floor returns math.floor
of the passed in number:
服务层返回传入号码的math.floor
:
import json
import logging
import os
import mathfrom flask import Flask, requestapp = Flask(__name__)@app.route('/', methods=['POST'])
def handle_post():
content = json.loads(request.data)
input = float(content['input'])
return f"{math.floor(input)}", 200if __name__ != '__main__':
# Redirect Flask logs to Gunicorn logs
gunicorn_logger = logging.getLogger('gunicorn.error')
app.logger.handlers = gunicorn_logger.handlers
app.logger.setLevel(gunicorn_logger.level)
app.logger.info('Service started...')
else:
app.run(debug=True, host='0.0.0.0', port=int(os.environ.get('PORT', 8080)))
As you might know, Cloud Run deploys containers, so you need a Dockerfile
and your container needs to bind to 0.0.0.0
and PORT
env variable, hence the code above.
如您所知,Cloud Run部署了容器,因此您需要一个Dockerfile
并且您的容器需要绑定到0.0.0.0
和PORT
env变量,因此需要上面的代码。
You can use the following Dockerfile.
您可以使用以下Dockerfile 。
Build the container:
构建容器:
export SERVICE_NAME=floor
gcloud builds submit --tag gcr.io/${PROJECT_ID}/${SERVICE_NAME}
Deploy to Cloud Run. Notice the no-allow-unauthenticated
flag. This makes sure the service only accepts authenticated calls:
部署到Cloud Run。 注意no-allow-unauthenticated
标志。 这样可以确保服务仅接受经过身份验证的呼叫:
gcloud run deploy ${SERVICE_NAME} \
--image gcr.io/${PROJECT_ID}/${SERVICE_NAME} \
--platform managed \
--no-allow-unauthenticated
工作流服务帐户 (Service Account for Workflows)
Before we can configure Workflows to call the Cloud Run service, we need to create a service account for Workflows to use:
在配置工作流以调用Cloud Run服务之前,我们需要创建一个服务帐户以供工作流使用:
export SERVICE_ACCOUNT =workflows-sa
gcloud iam service-accounts create ${ SERVICE_ACCOUNT }
Grant run.invoker
role to the service account. This will allow the service account to call authenticated Cloud Run services:
将run.invoker
角色授予服务帐户。 这将允许服务帐户调用经过身份验证的Cloud Run服务:
export PROJECT_ID=$(gcloud config get-value project)
gcloud projects add-iam-policy-binding ${PROJECT_ID} \
--member "serviceAccount:${SERVICE_ACCOUNT}@${PROJECT_ID}.iam.gserviceaccount.com" \
--role "roles/run.invoker"
更新工作流程 (Update the workflow)
Update the workflow.yaml
to include the Cloud Run service. Notice how we're also including auth
field to make sure Workflows passes in the authentication token in its calls to the Cloud Run service:
更新workflow.yaml
以包括Cloud Run服务。 请注意,我们还包括auth
字段以确保Workflows在其对Cloud Run服务的调用中传递身份验证令牌:
- randomgenFunction:
call: http.get
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/randomgen
result: randomgenResult
- multiplyFunction:
call: http.post
args:
url: https://us-central1-workflows-atamel.cloudfunctions.net/multiply
body:
input: ${randomgenResult.body.random}
result: multiplyResult
- logFunction:
call: http.get
args:
url: https://api.mathjs.org/v4/
query:
expr: ${"log(" + string(multiplyResult.body.multiplied) + ")"}
result: logResult
- floorFunction:
call: http.post
args:
url: https://floor-wvdg6hhtla-ew.a.run.app
auth:
type: OIDC
body:
input: ${logResult.body}
result: floorResult
- returnResult:
return: ${floorResult}
Deploy the workflow, this time passing in the service-account
:
部署工作流,这次传入service-account
:
gcloud beta workflows deploy workflow \
--source=workflow.yaml \
--service-account=${SERVICE_ACCOUNT}@${PROJECT_ID}.iam.gserviceaccount.com
Execute the workflow:
执行工作流程:
gcloud beta workflows execute workflow
In a few seconds, you can take a look at the workflow execution to see the result. It should be an integer number returned from the floor function.
几秒钟后,您可以查看工作流程的执行情况以查看结果。 它应该是floor函数返回的整数。
结语 (Wrap up)
Hopefully, this post helped you to have a good idea on what Workflows is. I’m definitely impressed with the potential of this new product. I’ll try out some more complicated use cases in a future blog post.
希望这篇文章可以帮助您对什么是工作流有个好主意。 这个新产品的潜力绝对给我留下了深刻的印象。 我将在以后的博客文章中尝试一些更复杂的用例。
In the meantime, if you want to check it out, here are some links:
同时,如果您想检查一下,这里有一些链接:
workflow-samples repo with the code in this post
带有本文中代码的工作流样本回购
Feel free to reach out to me on Twitter @meteatamel or read my previous posts on medium/@meteatamel.
欢迎通过Twitter @meteatamel与我联系,或阅读我以前在medium / @ meteatamel上的帖子。
Originally published at https://atamel.dev.
最初发布在 https://atamel.dev 。
翻译自: https://medium.com/google-cloud/a-first-look-at-serverless-orchestration-with-workflows-d80e41e9e04f
com 初探