魅族mx5游戏模式小熊猫
Pandas library has two main data structures which are DataFrame and Series. There are many built-in functions to create, manipulate, and analyze these structures.
熊猫库有两个主要的数据结构,分别是DataFrame和Series。 有许多内置函数可以创建,操纵和分析这些结构。
In this post, we will master a group of Pandas functions used for manipulating DataFrames and Series. These functions are map, apply, and applymap.
在本文中,我们将掌握一组用于操纵DataFrame和Series的Pandas函数。 这些函数是map , apply和applymap 。
In some cases, Pandas offer better options to use instead of map, apply, and applymap. We will also try to cover these options.
在某些情况下,Pandas提供了更好的选择来代替map,apply和applymap。 我们还将尝试介绍这些选项。
Let’s start with the definitions:
让我们从定义开始:
- Map: Maps (i.e. update, change, modify) the values of a Series. 映射:映射(即更新,更改,修改)系列的值。
- Apply: Applies a function along an axis of a DataFrame. 应用:沿DataFrame的轴应用功能。
- Applymap: Applies a function to a DataFrame element-wise. Applymap:将函数应用于DataFrame元素。
It is important to note that there are cases in which these functions perform the same operation and return the same output. We will see these cases as well as the ones that have differences.
重要的是要注意,在某些情况下,这些功能执行相同的操作并返回相同的输出。 我们将看到这些情况以及有差异的情况。
One of the major differences is that these functions work on different objects. Applymap works on dataframe whereas map works on series. Apply works on both.
主要区别之一是这些功能可在不同的对象上工作。 Applymap适用于数据框,而map适用于序列。 将作品应用于两者。
I will first create a simple dataframe to do examples.
我将首先创建一个简单的数据框来做示例。
import pandas as pd
import numpy as npdf = pd.DataFrame(np.random.randint(10, size=(5,5)), columns=list('ABCDE'))df
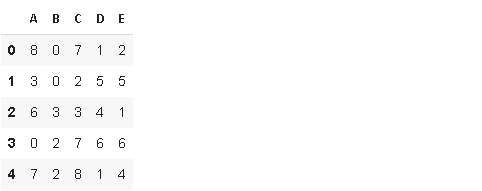
Consider we have a function that calculates the average of given values. If we apply this function to the dataframe with “apply”, it will return the averages of rows or columns.
考虑一下我们有一个计算给定值平均值的函数。 如果我们通过“ apply”将此函数应用于数据框,它将返回行或列的平均值。
def calculate_average(row_col):
return row_col.mean()
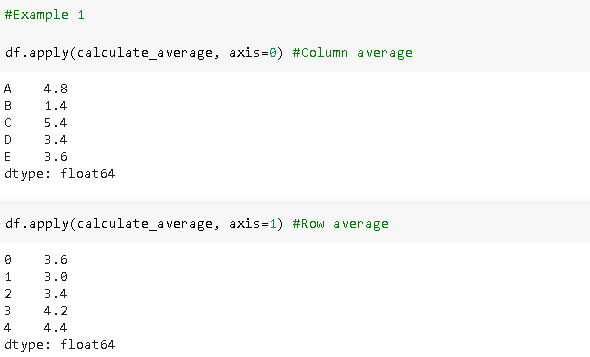
The function is applied along an axis (row or column). Pandas provide functions to perform simple statistical operations on dataframes. In such cases, these functions are preferred over the apply function.
该功能沿轴(行或列)应用。 熊猫提供了对数据框执行简单统计操作的功能。 在这种情况下,这些功能优于apply功能。
For instance, we can calculate the mean of each column with df.mean(axis=0)
and of each row with df.mean(axis=1)
. However, it is no harm to also know about the apply function.
例如,我们可以使用df.mean(axis=0)
计算每一列的平均值,并使用df.mean(axis=1)
计算每一行的df.mean(axis=1)
。 但是,知道应用功能也无害。
Applymap and map work on individual elements, not along an axis. To see the difference between map and applymap, let’s do a very simple math operation.
Applymap和map在单个元素上工作,而不是沿着轴。 要查看map和applymap的区别,让我们做一个非常简单的数学运算。
Applymap can be applied to the entire dataframe:
Applymap可以应用于整个数据框:
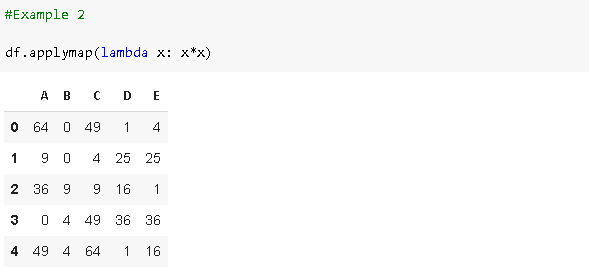
This function can be applied to a row or column using the map function. Thus, the map function cannot be applied to an entire dataframe.
使用map函数可以将此功能应用于行或列。 因此,映射功能不能应用于整个数据帧。
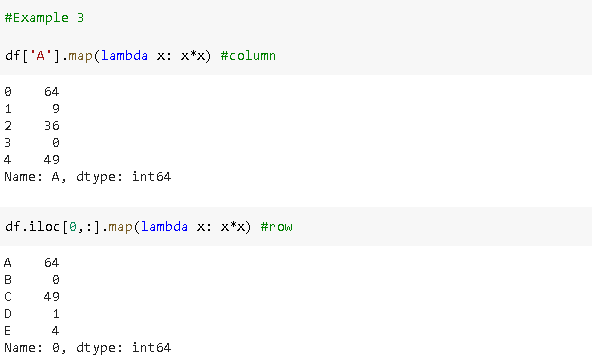
The simple math operations can be done as a vectorized operation which has a simplex syntax and is faster than map or applymap.
简单的数学运算可以作为向量运算完成,该向量运算具有单一语法,并且比map或applymap更快。
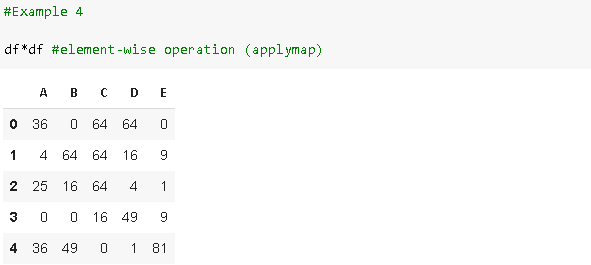
The same goes for the map function:
地图功能也是如此:
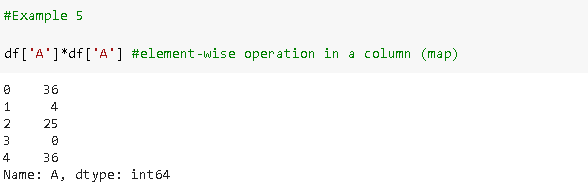
In addition to a function, the map also takes a dictionary or series to map values.
除了功能外,地图还采用字典或序列来映射值。
Let’s add two new columns to our dataframe:
让我们在数据框中添加两个新列:
df['F'] = ['Abc','Dbc','Fcd','Afc','Kcd']
df['G'] = ['A',np.nan,'B1','B1','C']df
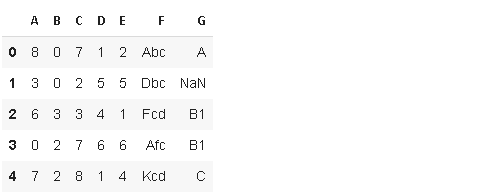
We want to change the values “B1” in column G as “B”.
我们希望将G列中的值“ B1”更改为“ B”。
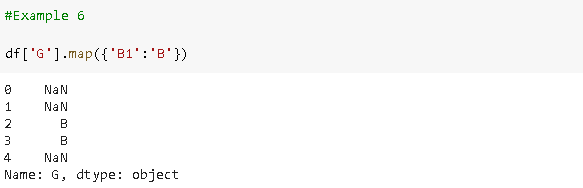
As you can see, the other values are mapped NaN which is the standard missing value representation. We also need to specify what other values should be mapped to.
如您所见,其他值被映射为NaN,这是标准的缺失值表示形式。 我们还需要指定其他值应该映射到什么。
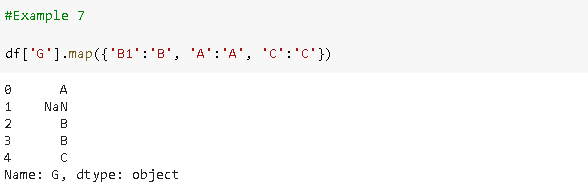
Having to also specify the other values makes the map function not an optimal choice in this case. The replace function of Pandas is a better choice here.
在这种情况下,还必须指定其他值会使映射功能不是最佳选择。 在这里,Pandas的替换功能是一个更好的选择。
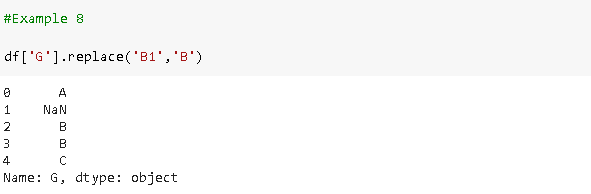
We can also use map and apply to return a list based on the existing values:
我们还可以使用map并应用以基于现有值返回列表:

Each item is a list that contains the original string converted to lowercase letters and the length of the string.
每个项目都是一个列表,其中包含转换为小写字母的原始字符串以及该字符串的长度。
These functions work in an iterative fashion which makes them relatively slower. In many cases, vectorized operations or list comprehensions are preferred over using map or applymap.
这些函数以迭代方式工作,这使它们相对较慢。 在许多情况下,矢量化操作或列表推导要比使用map或applymap更好。
Here is a comparison of a list comprehension, for loop, map function on squaring 50000 elements.
这是对平方运算50000个元素的列表理解,循环和映射功能的比较。

There are also some cases in which map function is preferred over a list comprehension. List comprehension loads the entire output list into memory. This is acceptable or even desirable for small or medium-sized lists because it makes the operation faster. However, when we are working with large lists (e.g. 1 billion elements), list comprehension should be avoided. It may cause your computer to crash due to the extreme amount of memory requirement. The map function does not also cause a memory problem.
在某些情况下,地图功能比列表理解更可取。 列表理解将整个输出列表加载到内存中。 对于中小型列表,这是可以接受的,甚至是理想的,因为它使操作更快。 但是,当我们处理大型列表(例如10亿个元素)时,应避免理解列表。 由于极高的内存需求,可能会导致计算机崩溃。 映射功能也不会引起内存问题。
There is no free lunch! The map function does not cause a memory issue but they’re relatively slower than list comprehensions. Similarly, the speed of list comprehension comes from excessive memory usage. You can decide which one to use depending on your application.
天下没有免费的午餐! map函数不会引起内存问题,但是它们比列表理解要慢。 同样,列表理解的速度也来自过多的内存使用。 您可以根据自己的应用程序决定使用哪个。
Thank you for reading. Please let me know if you have any feedback.
感谢您的阅读。 如果您有任何反馈意见,请告诉我。
翻译自: https://towardsdatascience.com/master-pandas-map-apply-and-applymap-in-5-minutes-d2bd1bba12a5
魅族mx5游戏模式小熊猫