递归二分查找代码实现?
NOTE:- Use laptop as a viewing screen or naturally landscape aligned screens for better pictures.
注意:-使用笔记本电脑作为查看屏幕或自然横向对齐的屏幕以获得更好的图片。
If there is one most beautiful piece of code in computer science then it is artistically applied recursion. DFS, transversal, memoization, regenerative approaches are all under its belt. Its something very much similar to the concept of infinity in mathematics, cut short by a base case. And if you are able to optimize tail calls then you have highly memory optimized approach. Avoid the tail calls don’t build stack, don’t build stack!! Recursive depth exceeded!!. There you have killed the beauty.
如果计算机科学中有一段最漂亮的代码,那么它在艺术上适用于递归。 DFS,横向,记忆,再生方法都可以使用。 它与数学中的无穷大概念非常相似,但通过基本情况加以简化。 而且,如果您能够优化尾调用,那么您将拥有高度内存优化的方法。 避免尾部调用不要建立堆栈,不要建立堆栈! 超出了递归深度!! 在那里,你杀死了美丽。
Adding to the cherry are fractals, recursive structures found in nature, snow flakes, clouds, flower petals, arteries, etc. Mathematicians have always been fascinated by them. The Mandelbrot's set needs a mention here. This amazing pattern is generated by complex numbers escaping the boundary or recursively falling back on hitting the wall.(Random thought reminds me of control system in electrical engineering, plots to verify the stability of a system)(wikipedia) (Numperhile).
分形,自然界中的递归结构,漫天飞舞的雪花,云朵,花瓣,动脉等,也增添了樱桃的魅力。数学家们一直对它们着迷。 Mandelbrot的场景需要在这里提及。 这种惊人的模式是由逃避边界或递归击中墙壁的复数产生的。( 随机想法让我想起了电气工程中的控制系统,绘制了验证系统稳定性的图 )( Wikipedia )( Numperhile )。
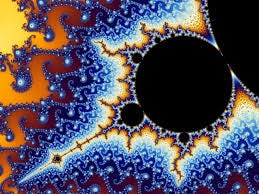
Fractals have been used to generate random numbers, used extensively in computer graphics to generate images/graphics. To study growth patterns of bacteria. Stock market trading. I do not know all the applications they have been put to but, they have a seemingly numerous applications, like other things in technology this is also inspired from nature.
分形已被用于生成随机数,在计算机图形学中广泛使用分形来生成图像/图形。 研究细菌的生长方式。 股市交易。 我不知道它们已经应用于所有应用程序,但是它们似乎有许多应用程序,就像技术上的其他事物一样,这也是从自然界中得到启发的。
Here i have listed some fractals which i have written, thanks to the amazing course(mooc) by Gregor Kiczales (cs professor at UBC) which imparted to me some understanding of these amazing pattern. The code are in python ad Racket which makes it quite readable and self explanatory enjoy!
在这里,我列出了我写的一些分形,这要归功于Gregor Kiczales (UBC的CS教授)的惊人课程(mooc),使我对这些惊人的模式有了一定的了解。 该代码位于python ad Racket中,使其可读性强,易于解释!
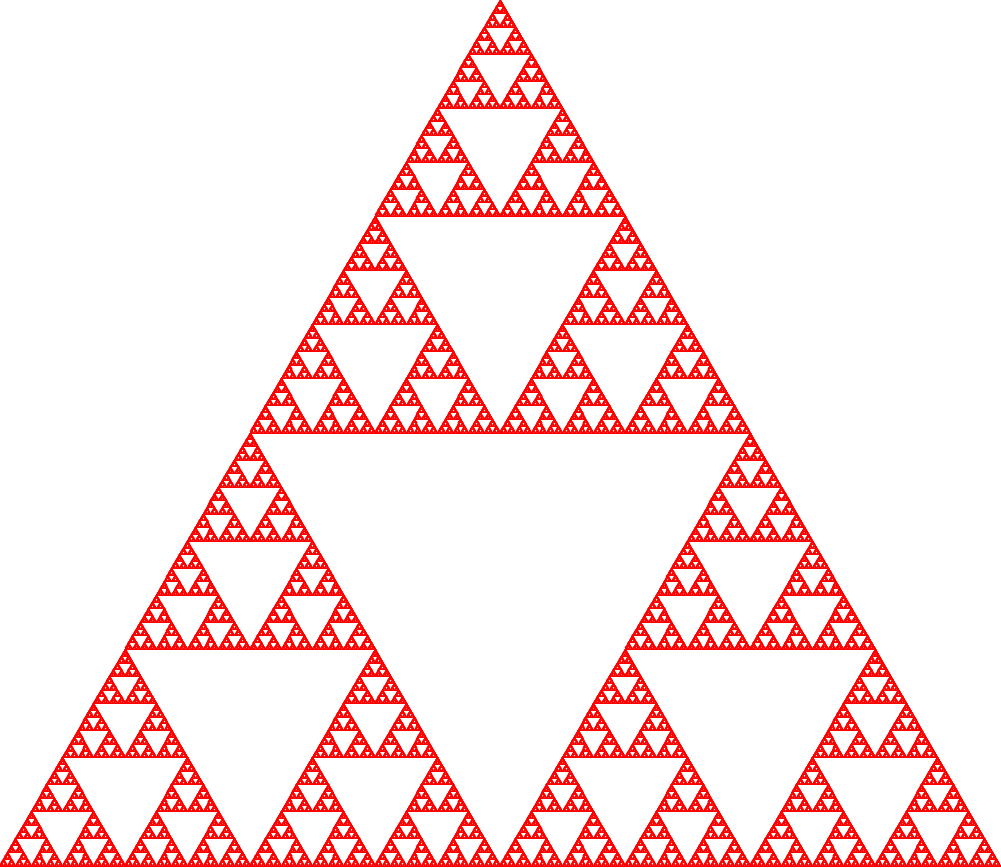
(define (stri s)
(if (<= s 4)
(triangle s “outline” “red”)
(overlay (triangle s “outline” “red”)
(local [(define sub (stri (/ s 2)))]
(above sub
(beside sub sub ))))))
The overlay keyword is to draw over past image, local to assign a local variable (local scope), assigns the result of past recursion to “sub” variable which are further placed in ⸫ fashion. These triangles are of half the size(height, width) than the earlier ones and are placed over the first one. The big triangle gets drawn as you enter the recursion. Base or cutoff is the if condition which keeps size(height,width) greater than 4.
overlay关键字用于绘制过去的图像,使用local分配一个局部变量(局部作用域),将过去递归的结果分配给“ sub”变量,这些变量进一步以⸫的方式放置。 这些三角形的大小(高度,宽度)是较早三角形的一半,并放置在第一个三角形上。 输入递归时将绘制大三角形。 基本条件或截断条件是if条件,其大小(高度,宽度)大于4。
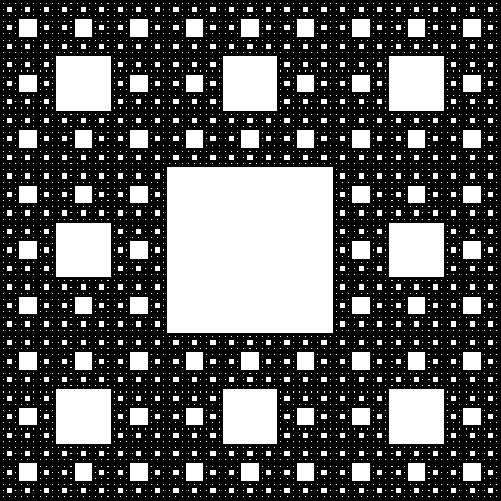
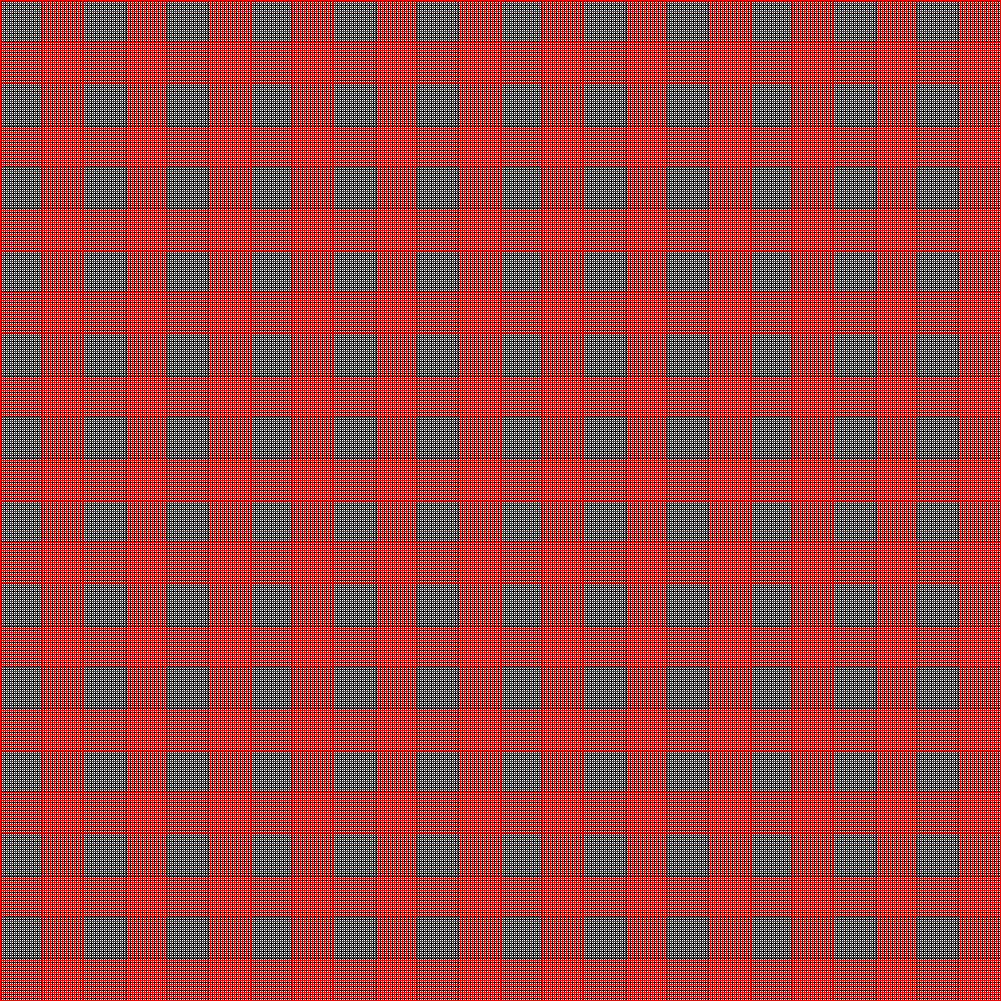
(define (carpet s)
(if (<= s 2)
(square s "outline" "black")
(overlay (square s "outline" "black")
(local [(define sub (carpet (/ s 3)))
(define ctr (square (/ s 3) "solid" "white"))]
(above (beside sub sub sub)
(beside sub ctr sub)
(beside sub sub sub))))))
The left one is achieved through above code, where inside a outer square nine length/3 squares are placed. The center square is solid white, rest being black. The right one uses the same code with all squares being in outline mode and colored interchangeably as red and black.
左边的代码是通过上面的代码实现的,在该代码的外部正方形内部放置了9个长度/ 3个正方形。 中心方块为纯白色,其余为黑色。 右边的代码使用相同的代码,所有正方形均处于轮廓模式,并且交替显示为红色和黑色。
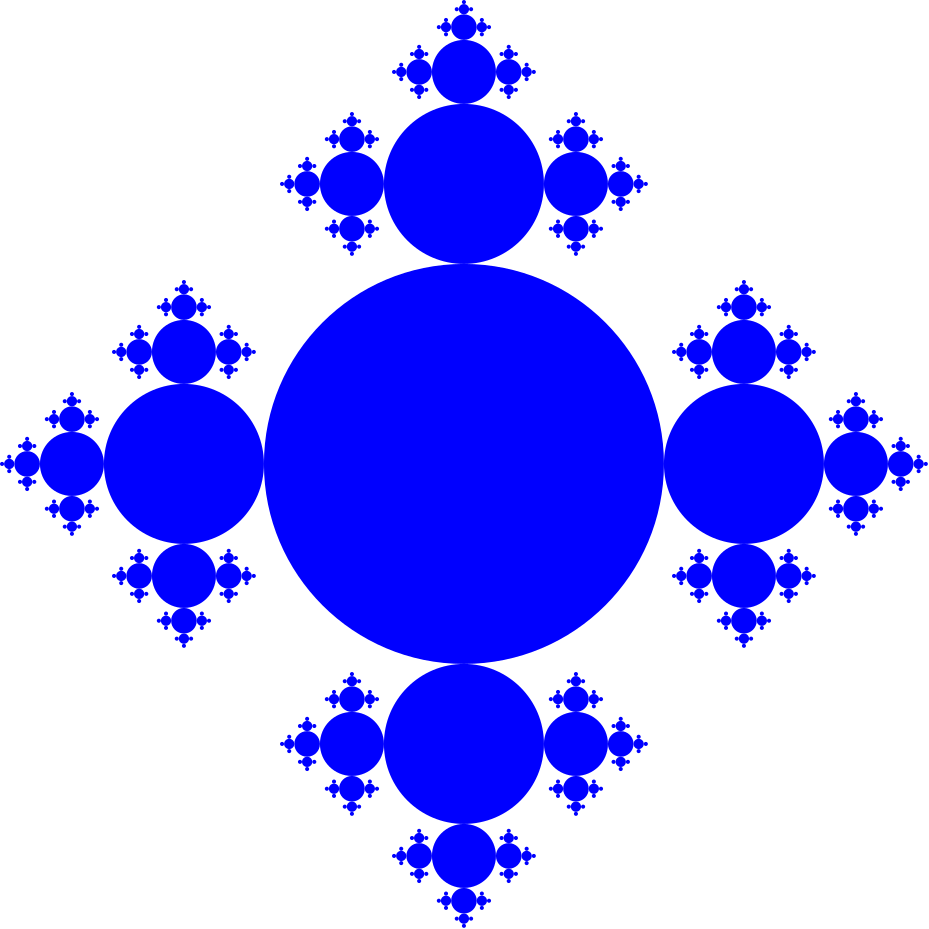
(define (circle-fractal n)
(local [(define top-leaf (draw-leaf (* n STEP)))
(define center (circle n "solid" "blue"))]
(above top-leaf
(beside (rotate 90 top-leaf) center (rotate -90 top-leaf))
(rotate 180 top-leaf)))); <template as gen-rec subroutine>
(define (draw-leaf n)
(if (<= n TRIVIAL-SIZE)
(circle n "solid" "blue")
(local [(define center (circle n "solid" "blue"))
(define leaf (draw-leaf (* n STEP)))]
(above leaf
(beside (rotate 90 leaf) center (rotate -90 leaf))))))
There are two functions circle-fractal, the main function and draw-leaf a subroutine which gets called to draw leafs around the center circle. “TRIVIAL-SIZE” is set to 5. The result of the subroutine is placed on four corners, that is rotated by 0, 90, -90 and 180. The subroutine is the one which does the main work or heavy lifting by generating the recursive pattern of petals. Recursively generating and placing them on top(“above”) and on both side (“beside”. Which is used by the main function.
圆形分形有两个功能,一个是主函数,另一个是绘制叶的子例程,该子例程被调用来绘制围绕中心圆的叶子。 “ TRIVIAL-SIZE”设置为5。该子例程的结果放置在四个角上,分别旋转了0、90,-90和180。该子例程是通过生成该子例程来执行主要工作或繁重工作的子例程。花瓣的递归模式。 递归地生成并将它们放置在顶部(“上方”)和两侧(“侧面”),由主函数使用。
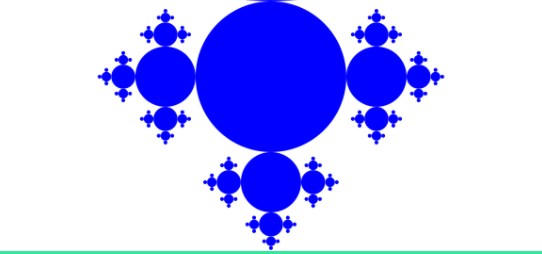
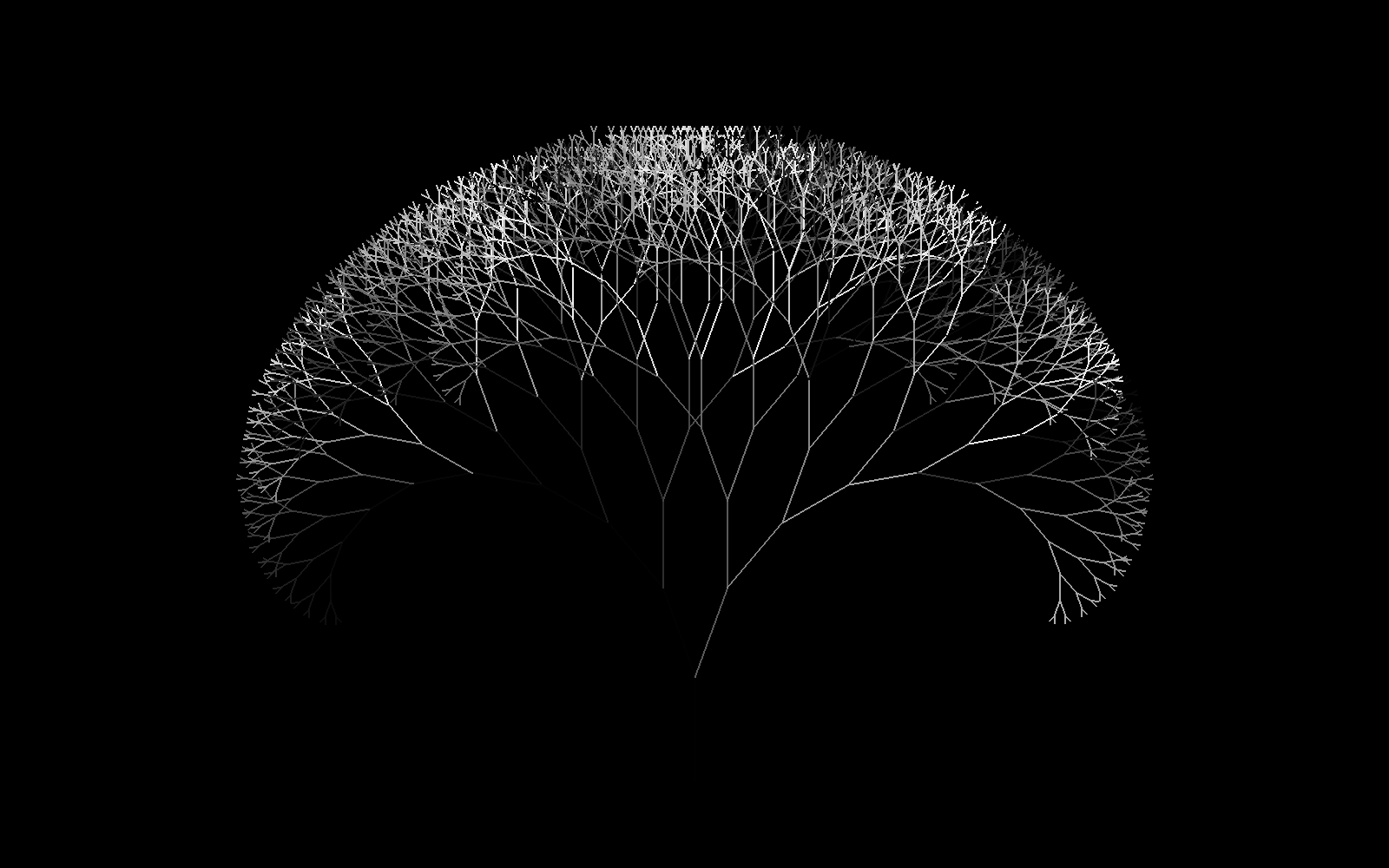
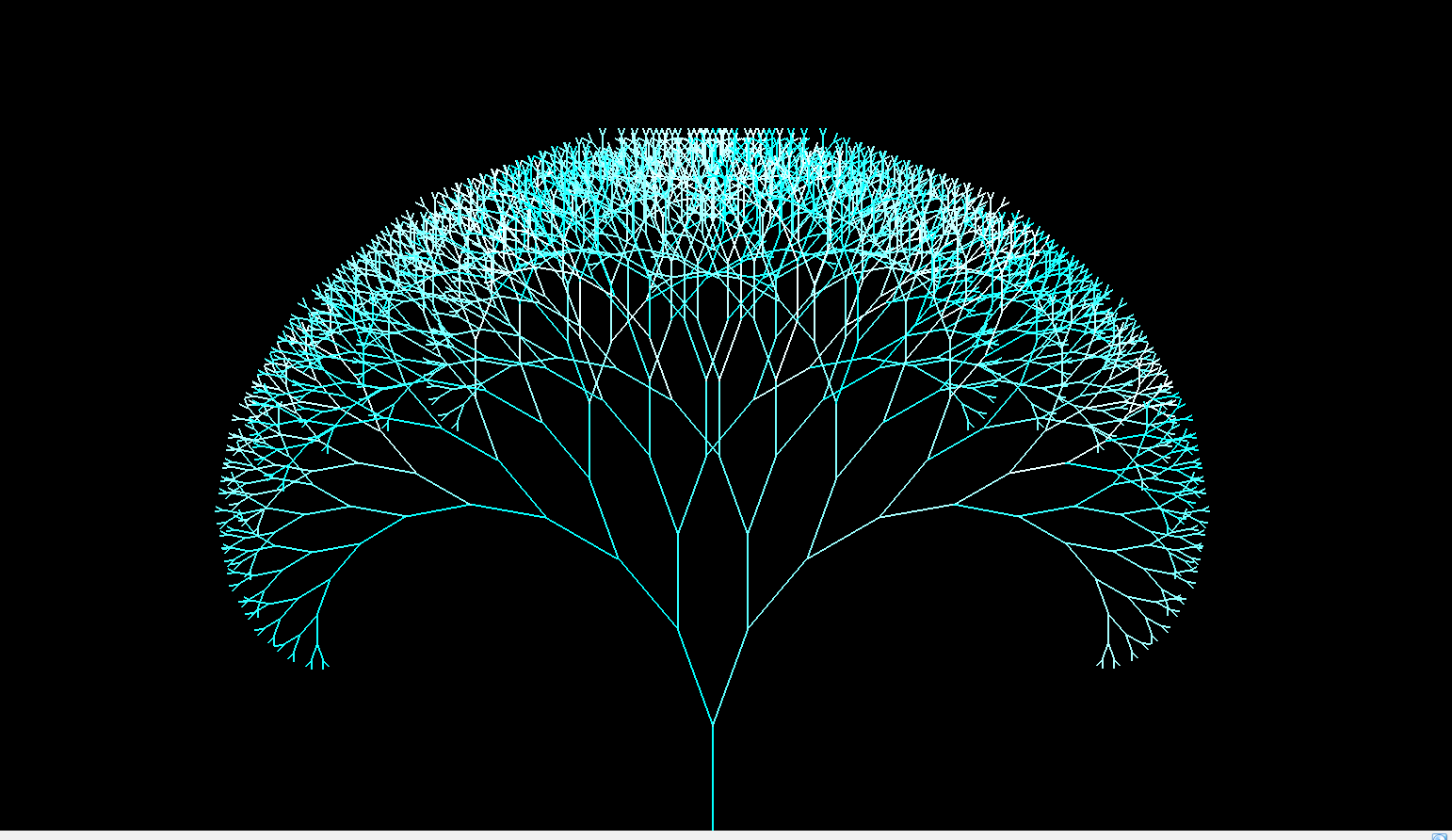
color_r_value = 0
color_b_value = 244
def changeColorR():
global color_r_value
if color_r_value<244:
color_r_value = color_r_value+1
color_r_value = 0
return color_r_valuedef changeColorB():
global color_b_value
if color_b_value>1:
color_b_value=color_b_value-1
color_b_value = 244
return color_b_value
def drawTree(x1, y1, angle, depth):
fork_angle = 20
base_len = 10.0
if depth > 0:
x2 = x1 + int(math.cos(math.radians(angle))*depth*base_len)
y2 = y1 + int(math.sin(math.radians(angle))*depth*base_len)
r = changeColorR()
b = changeColorB()
pygame.draw.line(screen, (r,255,255), (x1, y1), (x2, y2), 2)
drawTree(x2, y2, angle - fork_angle, depth -1 )
drawTree(x2, y2, angle + fork_angle, depth -1 )
This generates the green fractal, adding a switching if clause to select from “r” or “b” as color would generate color combination similar to shown below. Notice the two different lines drawn recursively from the same point at different angles which help us achieve the above effect. The left one uses black color as seed. Code credits.
这将生成绿色的分形,并添加一个if子句以从“ r”或“ b”中进行选择,因为颜色会生成类似于以下所示的颜色组合。 请注意,从同一点以不同的角度递归绘制的两条不同的线有助于我们达到上述效果。 左边的使用黑色作为种子。 代码积分。
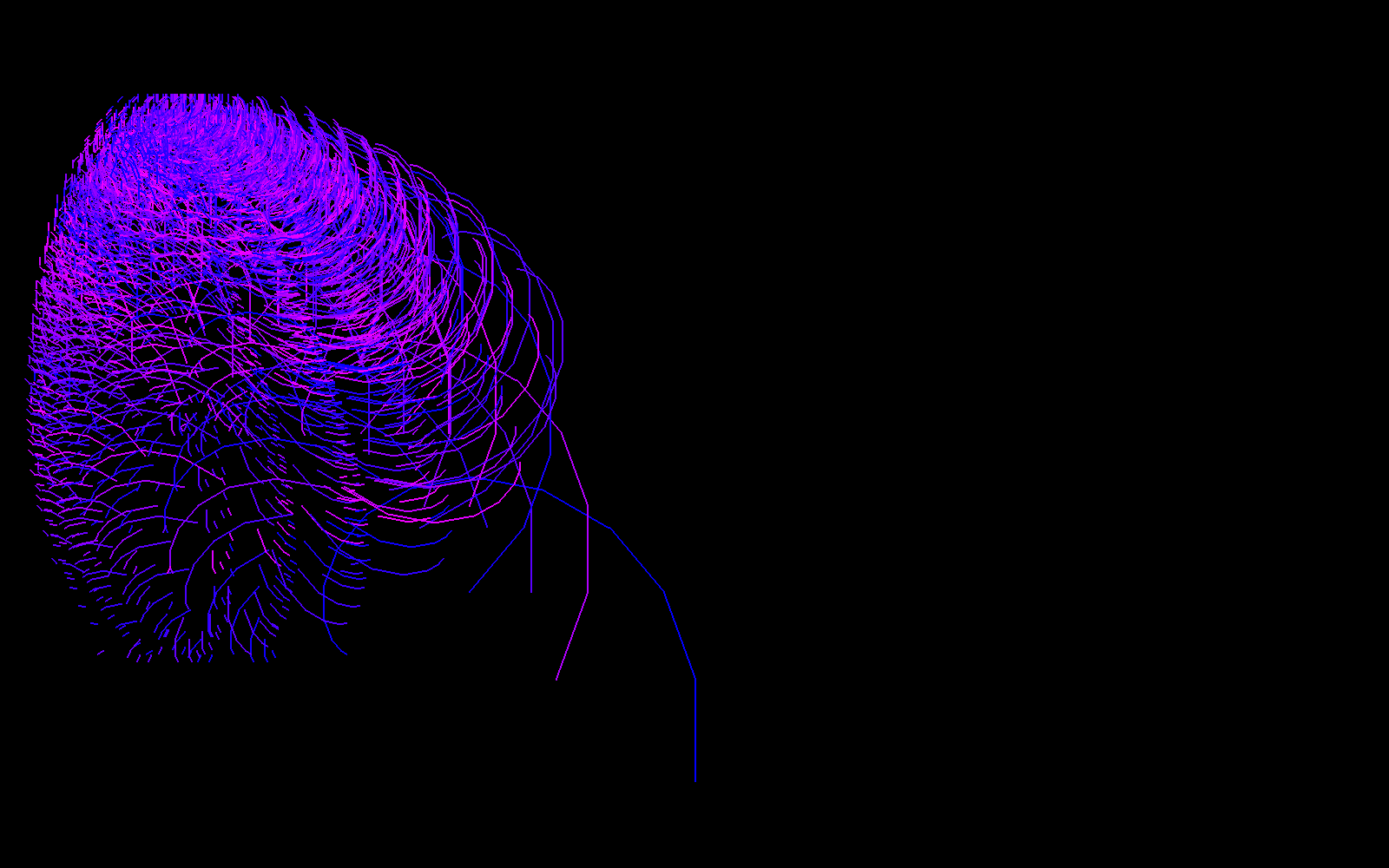
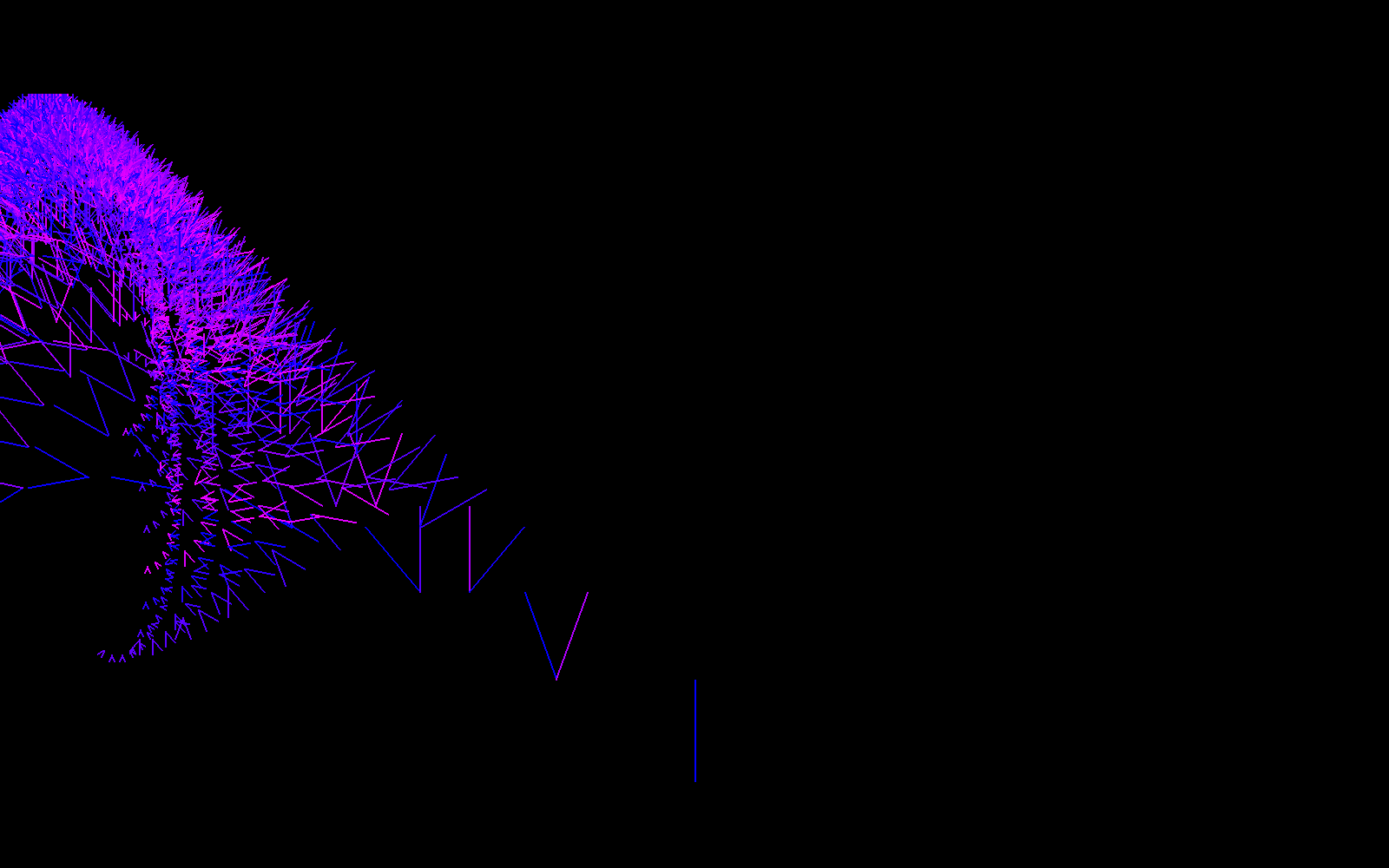
These have are obtained by playing around with the angles and recursive calls in the tree fractal above. We decrease the class from two to one, recursive call change the depth of recursion and length of line.
这些是通过在上面的树形分形中处理角度和递归调用而获得的。 我们将类从二减少到一,递归调用改变了递归的深度和行的长度。
Mentions for inspiration, do check out these resources.
提及灵感,请查看这些资源。
翻译自: https://medium.com/@ghostpc75/achieving-some-fractals-using-code-the-beauty-of-recursion-fa94dbc7783
递归二分查找代码实现?