数据结构二叉树的实现
We are about to implement tree data structure in Swift. First, let’s understand basic about tree structure.
我们将要在Swift中实现树数据结构。 首先,让我们了解有关树结构的基本知识。
A tree is a data structure that simulates the hierarchical relationship between objects. The structure is just like natural tree with a root value and subtrees of children with a parent node.
树是一种模拟对象之间层次关系的数据结构 。 该结构就像具有根值的自然树和具有父节点的孩子的子树一样。
Important Terms:
重要条款:
Root: The root of is the entry point to the tree data structure.
根:的根是树数据结构的入口点。
Node: Node is a block of data in the tree structure. Root itself is also a node.
节点:节点是树结构中的数据块。 根本身也是一个节点。
Leaf: Leaf is a node with no children.
叶子:叶子是没有子节点。
Level: Level of a node represents the generation of a node. Root is at level 0, while its next child is at level 1.
级别:节点的级别代表节点的生成。 根级别为0,下一个子级别为1。
As shown in diagram, we have implemented a tree with real life example. Tree is made up of nodes, Indoor and Outdoor are children of root — Sports. Likewise, each of them has two children. Table tennis, chess, football and rugby, each of them is a leaf, as they don’t have children.
如图所示,我们实现了带有实际示例的树。 树由节点组成,“室内”和“室外”是根的子级-运动。 同样,他们每个人都有两个孩子。 乒乓球,国际象棋,橄榄球和橄榄球,因为它们没有孩子,所以每个都是一片叶子。
Implementing in Swift
在Swift中实施
We will be implementing the basic Sports tree structure in Swift. As trees are made up of nodes, we are going to create a basic node class in Swift Playground.
我们将在Swift中实现基本的Sports树结构。 由于树由节点组成,因此我们将在Swift Playground中创建一个基本的节点类。
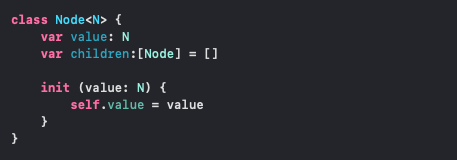
We have written a basic Node with a generic value “N” that it holds. It facilitates code reuse by allowing to build trees that hold different data types, just like arrays.
我们编写了一个具有通用值“ N”的基本节点。 它允许通过构建包含不同数据类型的树来促进代码重用,就像数组一样。
We’ve also declared an initializer which is required for initializing all non-optional stored properties for our class.
我们还声明了一个初始化器,该初始化器是初始化类的所有非可选存储属性所必需的。
Additionally, we declared “children” as an array of nodes. Each child represents a node that is 1 level deeper that the current node.
此外,我们将“子级”声明为节点数组。 每个子节点代表一个比当前节点深1级的节点。
Insertion
插入
We want to add values to our tree, we need a function for it.
我们想为树添加值,我们需要一个函数。
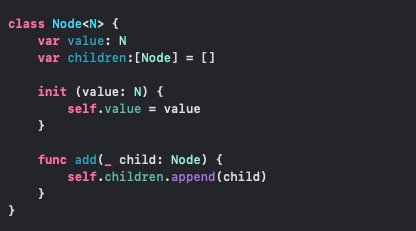
We have declared an add(child:) method in our Node class to handle insertion in our tree.
我们已经在Node类中声明了add(child :)方法来处理在树中的插入。
Let’s add values to create our Sports Tree.
让我们添加值来创建“运动树”。
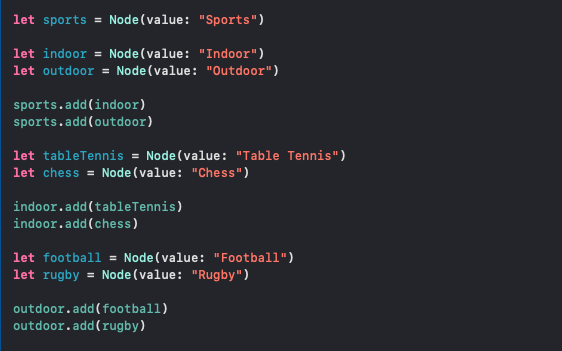
We have created our tree which corresponds to the “Sports” tree structure.
我们创建了与“运动”树结构相对应的树。
We will implement Depth First Traversal and Level Order Traversal of Tree Structure in our next blog.
我们将在下一个博客中实现树结构的深度优先遍历和层顺序遍历。
Complete code:
完整的代码:
class Node<N> {
var value: N
var children:[Node] = []
init (value: N) {
self.value = value
}
func add(_ child: Node) {
self.children.append(child)
}
}
let sports = Node(value: "Sports")
let indoor = Node(value: "Indoor")
let outdoor = Node(value: "Outdoor")
sports.add(indoor)
sports.add(outdoor)
let tableTennis = Node(value: "Table Tennis")
let chess = Node(value: "Chess")
indoor.add(tableTennis)
indoor.add(chess)
let football = Node(value: "Football")
let rugby = Node(value: "Rugby")
outdoor.add(football)
outdoor.add(rugby)
翻译自: https://medium.com/swlh/implementing-tree-data-structure-in-swift-39dc5a28da72
数据结构二叉树的实现