mpvue中使用ui组件库
This article will talk about server-driven UI, its implementation using re-usable components called UIComponents, and creating a generic vertical list view for rendering UI components. It will conclude with a brief discussion of how UI components can serve different purposes.
本文将讨论服务器驱动的UI,使用称为UIComponents的可重用组件的实现,以及创建用于呈现UI组件的通用垂直列表视图。 最后将简要讨论UI组件如何实现不同的目的。
什么是服务器驱动的UI? (What Is Server-Driven UI?)
- It is an architecture where the server decides the UI views that need to be rendered on the application screen. 它是服务器决定需要在应用程序屏幕上呈现的UI视图的体系结构。
- There exists a contract between the application and the server. The basis of this contract gives the server control over the UI of the application. 应用程序和服务器之间存在合同。 该合同的基础使服务器可以控制应用程序的UI。
What is that contract?- The server defines the list of components. For each of the components defined at the server, we have a corresponding UI implementation in the app (UIComponent). Consider an entertainment app like Hotstar, whose contract is defined as shown below. On the left are the components from the server, and on the right are the corresponding UI components.
那是什么合同?-服务器定义组件列表。 对于服务器上定义的每个组件,我们在应用程序(UIComponent)中都有一个相应的UI实现。 考虑像Hotstar这样的娱乐应用,其合同定义如下。 左边是服务器中的组件,右边是相应的UI组件。
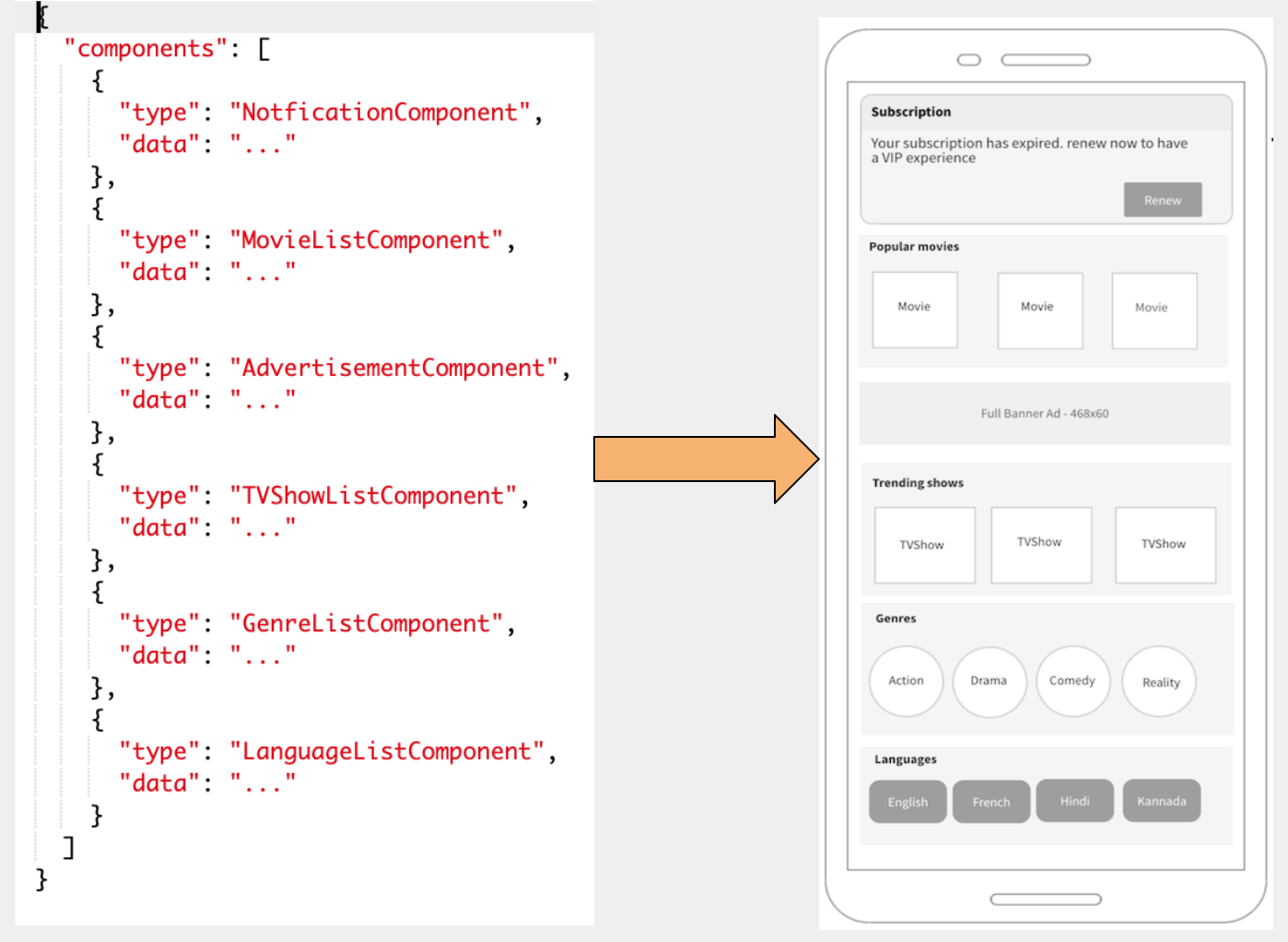
Working — The screen does not have a predefined layout like a storyboard. Rather, it consists of a generic list view rendering multiple different views vertically, as per the server response. To make it possible, we have to create views that are standalone and can be reused throughout the application. We call these re-usable views the UIComponent.
工作中-屏幕没有预定义的布局,例如情节提要。 而是由一个通用列表视图组成,根据服务器响应,该列表视图垂直呈现多个不同的视图。 为了使之成为可能,我们必须创建独立的视图,并且可以在整个应用程序中重复使用这些视图。 我们称这些可重用视图为UIComponent 。
Contract — For every server component, we have a UIComponent.
合同-对于每个服务器组件,我们都有一个UIComponent。
SwiftUI (SwiftUI)
Swift is a UI toolkit that lets you design application screens in a programmatic, declarative way.
Swift是一个UI工具包,可让您以编程的声明性方式设计应用程序屏幕。
SwiftUI中服务器驱动的UI实施 (Server-Driven UI Implementation in SwiftUI)
This is a three-step process.
这是一个三步过程。
- Define the standalone UIComponents. 定义独立的UIComponent。
- Construct the UIComponents based on the API response. 根据API响应构造UIComponent。
- Render the UIComponents on the screen. 在屏幕上呈现UIComponents。
1. 定义独立的UIComponents (1. Define the standalone UIComponents)
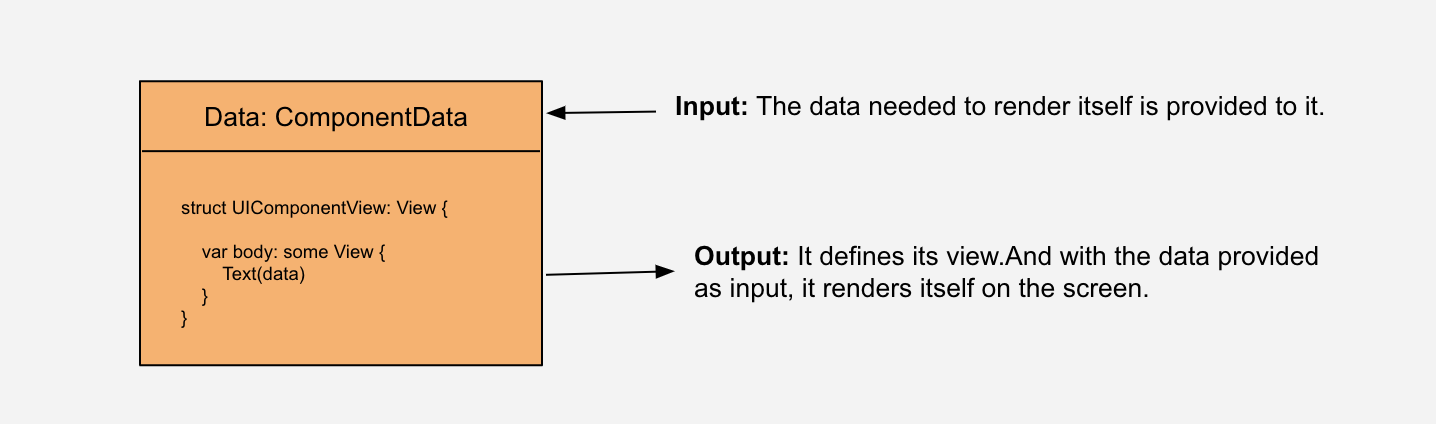
Input: Firstly, for the UIComponent to render itself, it should be provided with data.
输入:首先,要使UIComponent呈现自身,应为其提供数据。
Output: UIComponent defines its UI. When used for rendering inside a screen, it renders itself based on the data (input) provided to it.
输出: UIComponent定义其UI。 当用于在屏幕内部进行渲染时,它根据提供给它的数据(输入)进行渲染。
UIComponent implementation
UIComponent实现
- All the UI views have to conform to this UI-component protocol. 所有UI视图都必须符合此UI组件协议。
As the components are rendered inside a generic vertical list, each UIComponent has to be independently identified. The
uniqueId
property is used to serve that purpose.由于组件是在通用垂直列表中呈现的,因此每个UIComponent必须独立标识。
uniqueId
属性用于实现此目的。The
render()
is where the UI of the component is defined. Calling this function on a screen will render the component. Let's look atNotificationComponent
.render()
是定义组件的UI的位置。 在屏幕上调用此函数将渲染组件。 让我们看一下NotificationComponent
。
NotificationUIModel
is the data required by the component to render. This is the input to the UIComponent.NotificationUIModel
是组件呈现所需的数据。 这是UIComponent的输入。NotificationView
is a SwiftUI view that defines the UI of the component. It takes inNotificationUIModel
as a dependency. This view is the output of the UIComponent when used for rendering on the screen.NotificationView
是一个SwiftUI视图,用于定义组件的UI。 它以NotificationUIModel
作为依赖项。 当用于在屏幕上呈现时,此视图是UIComponent的输出。
2. 根据API响应构造UIComponents (2. Construct the UIComponents based on the API response)
HomePageController
loads the server components from the repository and converts them into the UIComponents.HomePageController
从存储库加载服务器组件,并将它们转换为UIComponents。The
uiComponent
's property is responsible for holding the list of UIComponents. Wrapping it with the@Published
property makes it an observable. Any change in its value will be published to theObserver(View)
. This makes it possible to keep theView
in sync with the state of the application.uiComponent
的属性负责保存UIComponents的列表。 用@Published
属性对其进行包装使其可观察。 其值的任何更改都将发布到Observer(View)
。 这样可以使View
与应用程序状态保持同步。
3. 在屏幕上渲染UIComponents (3. Render UIComponents on the screen)
This the last part. The screen’s only responsibility is to render the UIComponents
. It subscribes to the uiComponents
observable. Whenever the value of the uiComponents
changes, the HomePage
is notified, which then updates its UI. A generic ListView
is used for rendering the UIComponents.
这是最后一部分。 屏幕的唯一责任是呈现UIComponents
。 它订阅了可观察到的uiComponents
。 每当uiComponents
的值更改时,就会通知HomePage
,然后更新其UI。 通用ListView
用于呈现UIComponent。
Generic Vstack:
All the UIComponents are rendered vertically using a VStack
inside. As the UIComponents are uniquely identifiable, we can use the ForEach
construct for rendering.
通用Vstack:
使用内部的VStack
垂直呈现所有UIComponent。 由于UIComponent是唯一可识别的,因此我们可以使用ForEach
构造进行渲染。
Since all the components conforming to UIComponent protocol must return a common type, the render()
function returns AnyView
. Below is an extension on the View
for converting it toAnyView
.
由于所有符合UIComponent协议的组件都必须返回公共类型,因此render()
函数将返回AnyView
。 下面是View
的扩展,用于将其转换为AnyView
。
结论 (Conclusion)
We saw how UIComponent
can be used to give the server control over the UI of the application. But with UIComponents
you can achieve something more.
我们看到了如何使用UIComponent
来使服务器控制应用程序的UI。 但是,使用UIComponents
可以实现更多目标。
Let’s consider a case without server-driven UI. It's often the case that the pieces of UI are used many times across the application. This leads to duplication of the view and view logic. So, it’s better to divide the UI into meaningful, reusable UI-components.
让我们考虑没有服务器驱动的UI的情况。 UI片段在整个应用程序中经常使用多次。 这导致视图和视图逻辑的重复。 因此,最好将UI分成有意义的,可重用的UI组件。
Having them this way will let the domain-layer/business layer define and construct the UI components. Additionally, the business-layer can take the responsibility of controlling the UI.
以这种方式拥有它们将使域层/业务层定义和构造UI组件。 此外,业务层可以负责控制UI。
You can find the project on GitHub.
您可以在GitHub上找到该项目 。
Have a look at the article “Android Jetpack Compose — Create a Component-Based Architecture,” which explains UI-Components in detail. As it uses Jetpack compose-Android’s declarative UI kit, it wouldn’t be hard to understand.
看一下文章“ Android Jetpack撰写-创建基于组件的架构 ” 详细解释了UI组件。 由于它使用Jetpack compose-Android的声明性UI套件,因此不难理解。
mpvue中使用ui组件库