mfc窗口支持伸缩
Hello everyone!, thanks @Yves Sinkgraven for the opportunity of writing articles to ITNEXT.IO.
大家好!感谢@ Yves Sinkgraven 给 ITNEXT.IO 撰写文章的机会 。
Inject is a small library I made a few days ago which enables an easy way of injecting your dependencies using a dependency resolver.
Inject是我几天前制作的一个小型库,它提供了一种使用依赖项解析器注入依赖项的简便方法。
这个主意 (The idea)
The idea came as I was reading a medium article which explained how dependency injection could work for Swift using property wrappers.
这个想法是在我读一篇中篇文章时提出的 ,该篇文章解释了依赖注入如何使用属性包装器对Swift进行工作。
I’m also learning ASP.NET core with C# and I really liked how easy is to inject dependencies to your services:
我也在学习C#的ASP.NET核心 ,我真的很喜欢将依赖注入到您的服务中有多么容易:
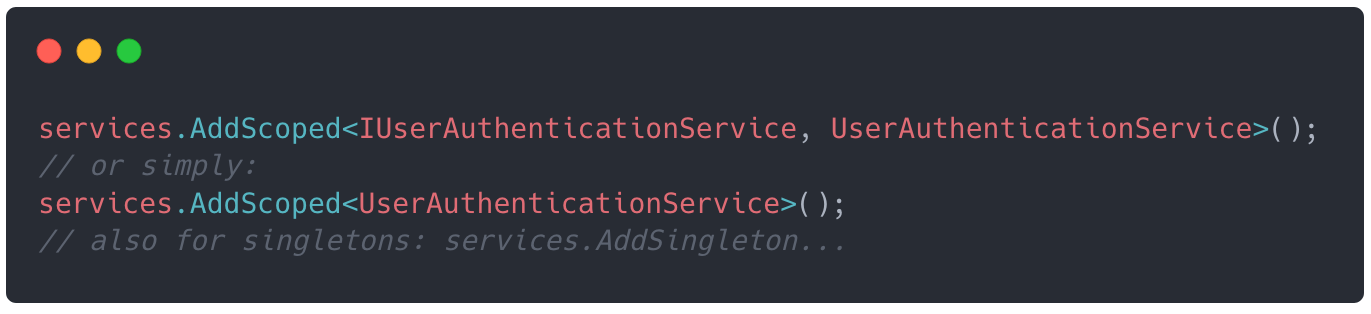
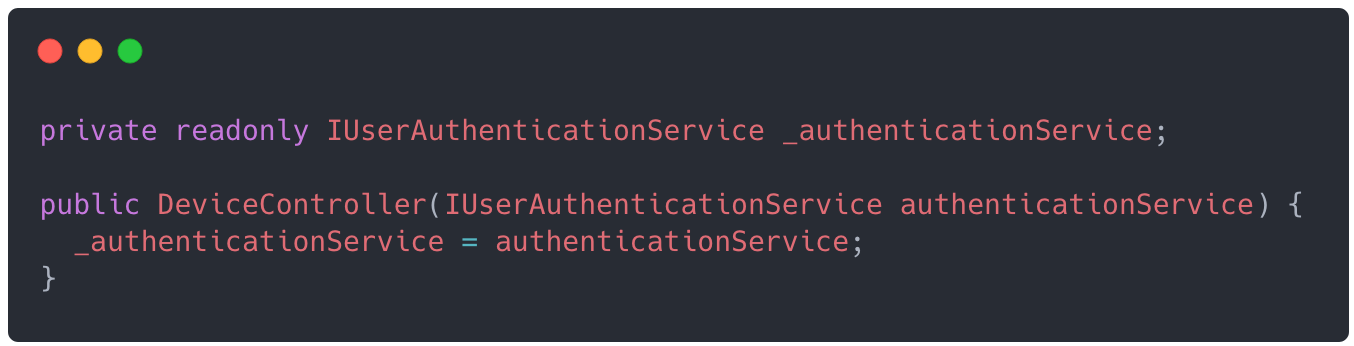
With these ideas in mind I decided to make a library for Swift.
考虑到这些想法,我决定为Swift创建一个库。
But first thing first, what is dependency injection? Do I need to use any external library for that? what does this library offers?
但是首先, 什么是依赖注入? 我需要使用任何外部库吗? 这个图书馆提供什么?
依赖注入 (Dependency injection)
(this is a just brief explanation, if you already know what is all about you can skip this section)
(这只是一个简短的说明,如果您已经知道全部内容,则可以跳过本节)
Basically, lets say you have a view/viewController in your app in which you want to retrieve user data which is fetched from a remote service or a database, so you can later use it on a label, or button, etc. You may want to use the repository pattern, in which you create different classes to encapsulate the logic to access or modify a data source.
基本上,假设您的应用程序中有一个view / viewController,您想在其中检索从远程服务或数据库中获取的用户数据,以便以后可以在标签或按钮等上使用它。使用存储库模式,您可以在其中创建不同的类来封装访问或修改数据源的逻辑。
One thing you could do is create an instance of that repository in you view, so whenever you want to use some data (retrieve user, modify, etc) you use that repository.
您可以做的一件事是在视图中创建该存储库的实例,因此,每当您要使用某些数据(检索用户,修改等)时,都可以使用该存储库。
One improvement over this is not to instantiate that repository inside that view class because that view shouldn’t know about how the repository is implemented or created, but rather it just wants to use it. Basicall we should put in practice the Dependency inversion principle, which in this case is as simple as moving the instantiation of the dependency (our repository) to the view constructor, something like MyView(userRepository: SomeUserRepository()).
对此的一种改进不是在实例化视图类中实例化该存储库,因为该视图不应该知道该存储库是如何实现或创建的,而只是想使用它。 基本上,我们应该实践Dependency Inversion原理 ,在这种情况下,就像将依赖项(我们的存储库)的实例移动到视图构造函数一样简单,就像MyView(userRepository: SomeUserRepository()).
Another improvement is to create an interface/protocol in Swift for your repository (repository or anything that is a dependency). So you could have a real implementation or a mock implementation to fake some data. Or you could have an implementation that right now uses a local database but later on uses a remote database or just some web services. Having a common interface for your dependencies is a very nice choice.
另一个改进是在Swift中为您的存储库 (存储库或任何依赖项) 创建接口/协议 。 因此,您可以使用真实的实现或模拟的实现来伪造某些数据。 或者,您可能有一个现在使用本地数据库但稍后使用远程数据库或仅某些Web服务的实现。 为依赖项提供通用接口是一个很好的选择。

As another improvement, we could even use the factory pattern to create a factory class for our repository, so instead of instantiating the repo directly we do it in a centralized place. Advantages: if you want to change all your repository instantiations used accrosed the app you just change it in one place.
作为另一个改进,我们甚至可以使用工厂模式为我们的存储库创建工厂类,因此,我们直接在集中的位置进行操作,而不是直接实例化仓库。 优点 :如果您想更改使用的所有存储库实例化应用程序,则只需在一个地方进行更改即可。
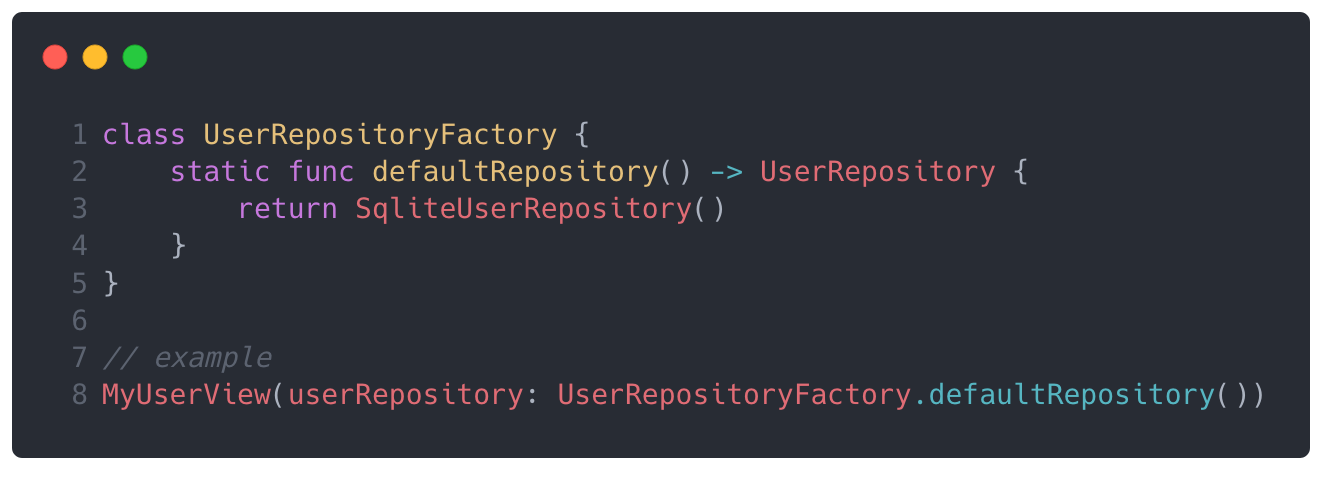
Almost the same case is for singletons, but would need a shared instance of it.
单例几乎相同,但需要共享实例。
注入 (Inject)
Before explaining why you might want to use my library, first let me introduce you to a small example on how to add your dependencies and use it. This is the equivalent of what we’ve talked about before but using inject.
在解释为什么要使用我的库之前,首先让我向您介绍一个有关如何添加依赖项和使用它的小示例。 这等效于我们之前讨论的但使用注入的内容。

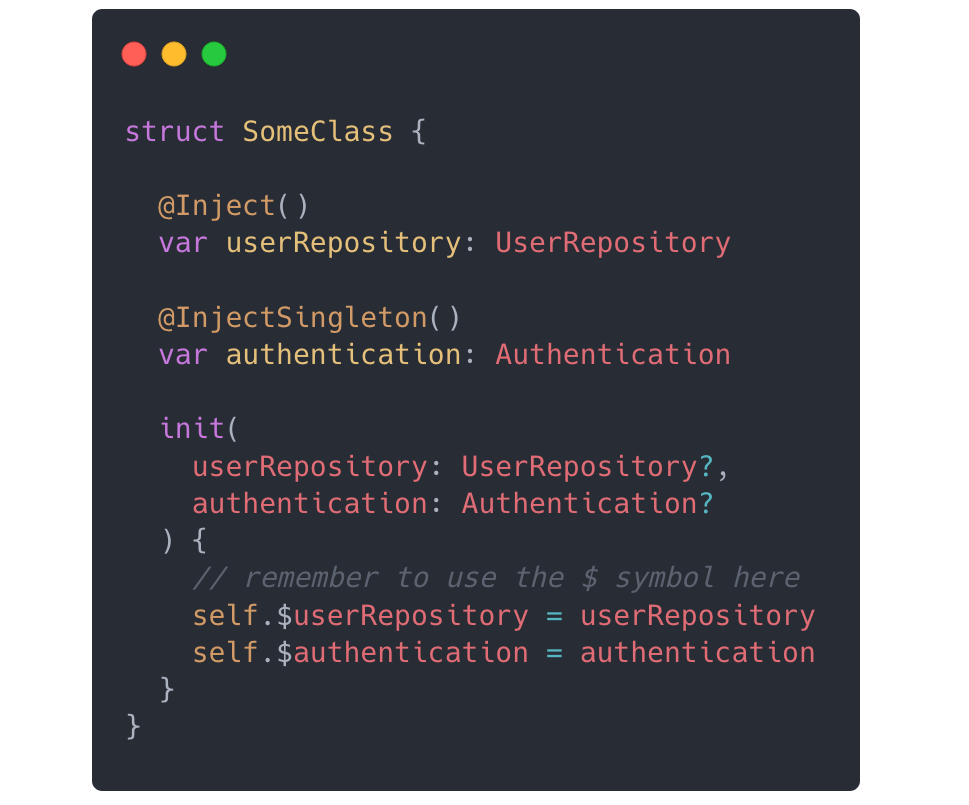
We add the dependencies by using a protocol and an implementation of it. Later we declare them on the classes we want to use them and use the Inject property wrapper, from this point you can normally use your dependencies inside your classes (you only need to use $yourDependency in your init method, and it’s actually optional to do so).
我们通过使用协议及其实现来添加依赖项 。 稍后, 我们在要使用它们的类上声明它们并使用Inject属性包装器 ,从这一点出发,您通常可以在类内部使用依赖项(您只需要在init方法中使用$ yourDependency,并且实际上是可选的)所以)。
How it works?
这个怎么运作?
Inject uses a dependency resolver, you must statically instantiate it in order to use it. It basically registers your dependencies and how to build them so it can later resolve it.
Inject使用依赖项解析器,您必须静态实例化它才能使用它。 它基本上注册了您的依赖关系以及如何建立依赖关系,以便以后可以解决它。
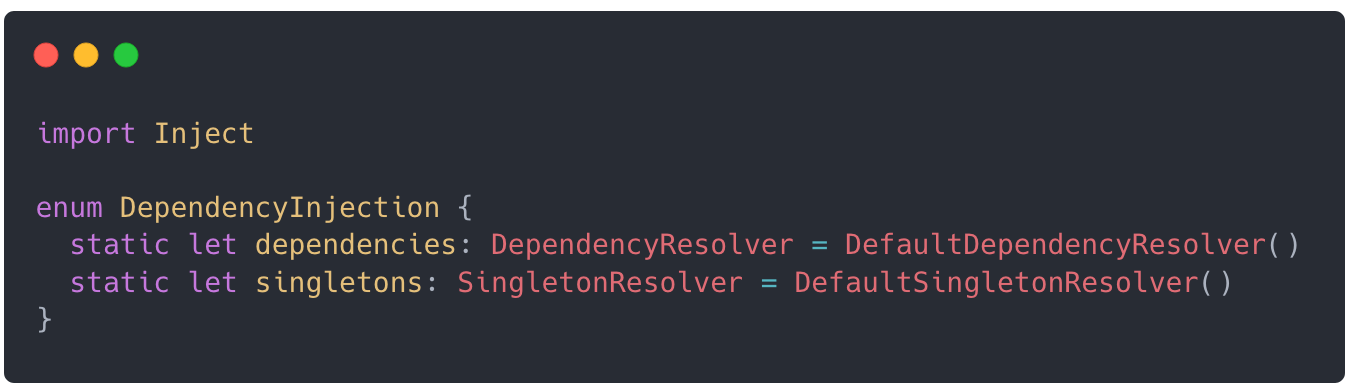
This is how the Inject property wrapper really works, it uses a dependency resolver passed in the constructor, and later when you use the variable it resolves the dependency implementation.
这就是Inject属性包装程序真正起作用的方式,它使用构造函数中传递的依赖项解析器,稍后,当您使用变量时,它解析依赖项实现。
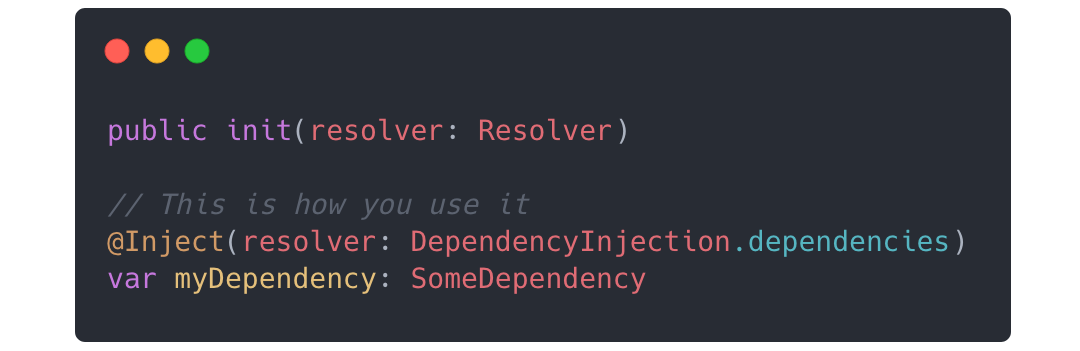
If you don’t want to keep passing your dependency resolver around you can just create a local extension that uses that in an empty constructor.
如果您不想一直传递依赖项解析器,则可以创建一个在空构造函数中使用它的本地扩展。
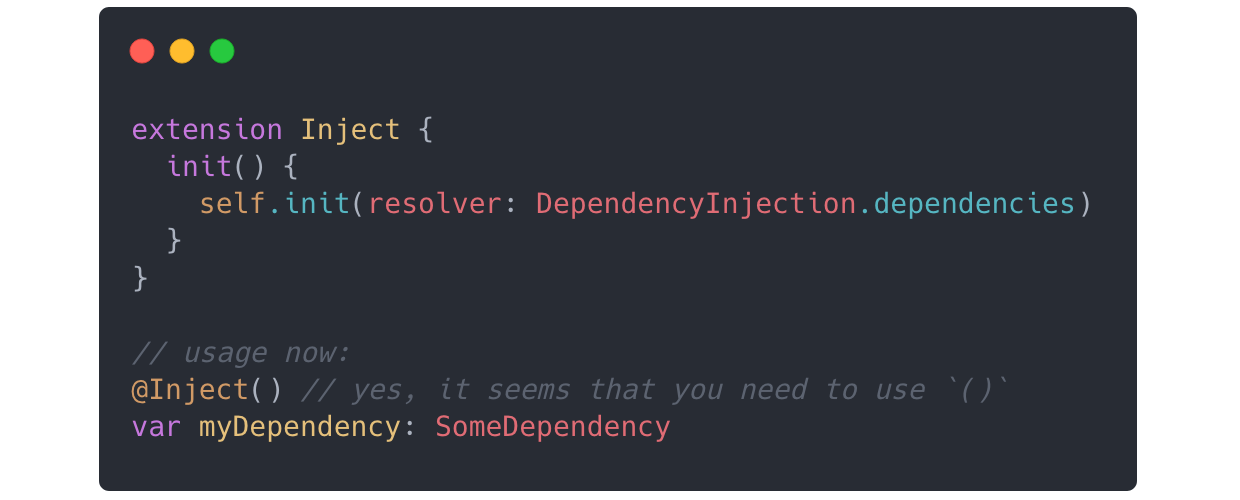
Also, Singletons
另外,单身人士
What about singletons? Inject
supports them. You just need to create a class (or even a struct and wrap it inside a Box<T>
type), create some methods, and add it using a singleton resolver. You can add a type implementation directly or use a protocol, as with previous examples.
那单身呢? Inject
支持他们。 您只需要创建一个类(甚至是一个结构,并将其包装在Box<T>
类型中),创建一些方法,然后使用单例解析器添加即可。 与前面的示例一样,您可以直接添加类型实现或使用协议。

You don’t need a static variable and you can use a protocol for your singleton too.
您不需要静态变量,也可以为单例使用协议。
In this case the singleton resolver will store a reference value to the singleton instance acroos the app. Unlike the normal resolver, which only saves a function to build a new dependency.
在这种情况下,单例解析器将在acroos应用程序中为单例实例存储参考值。 与普通解析器不同,常规解析器仅保存函数以构建新的依赖项。
But wait! There’s more
可是等等! 还有更多
Nested dependencies!
嵌套的依赖项!

In this case we have a repository which itself depends upong a database manager class or something. Thanks to inject, we just add all the dependencies and magically use the injected CarRepository :)
在这种情况下,我们有一个存储库,它本身依赖于数据库管理器类之类。 多亏了注入,我们只需添加所有依赖项并神奇地使用注入的CarRepository :)
More stuff
更多东西
You can also use @AutoWired
, which is another property wrapper which doesn’t have a setter. I don’t recommend it too much because you can’t easily change its value as when using inject, but some people might prefer it this way.
您还可以使用@AutoWired
,这是另一个没有设置器的属性包装器。 我不建议您过多使用它,因为您不能像使用inject那样轻易更改它的值,但是有些人可能会喜欢这种方式。
结论—优点,缺点,为什么要使用Inject (Conclusion — Pros, Cons, why you might want to use Inject)
Cons:
缺点 :
- Maybe too much stuff for simple/small projects with a couple dependencies. 对于具有几个依赖项的简单/小型项目而言,可能太多了。
- It’s a project dependency, it needs to be updated as swift does (which it will I hope). 这是一个项目依赖性,需要像swift一样进行更新(我希望如此)。
Pros:
优点 :
- Easy to use once you set it up. 设置后易于使用。
- Swift package manager support. Swift包管理器支持。
- It uses some kind of factory pattern but under the hood, you just need to add your dependencies and use them. 它使用某种工厂模式,但是在后台,您只需要添加依赖项并使用它们。
Special support for singletons, no need to use to create more static variables for them; just use them as normal dependencies!
对单身人士的特殊支持,无需使用为其创建更多静态变量; 只需将它们用作常规依赖项即可!
- Lazily resolves your dependencies. 懒惰地解决您的依赖关系。
- Great nested dependencies support. 极大的嵌套依赖关系支持。
Well, that’a all for today! If you are still interested and think Inject is for you, check its github repo here:
好吧,今天就这些! 如果您仍然有兴趣并且认为Inject适合您,请在此处查看其github存储库:
And here is a project example using it:
这是一个使用它的项目示例:
Thanks for reading! :D
谢谢阅读! :D
mfc窗口支持伸缩