android 交互动画
Welcome back! This week’s posts cover an assortment of SwiftUI micro-interactions that I’ve made for my apps. The benefits these interactions bring can really help make your app feel polished and simple to use. Today’s micro-interactions are all based on my custom Wave
shape.
欢迎回来! 本周的帖子涵盖了我为我的应用所做的各种SwiftUI微交互。 这些交互带来的好处可以真正帮助您使应用程序更美观,更易于使用。 今天的微交互都基于我自定义的Wave
形状。
If you found this tip helpful, please consider subscribing using this link, and if you aren’t reading this on TrailingClosure.com, please come check us out sometime!
如果您发现此提示有用,请考虑使用此链接进行订阅,如果您未在TrailingClosure.com上阅读此提示,请随时联系我们!
SwiftUI Wave
(SwiftUI Wave
Shape)
These animations all start with one thing in common and that’s my custom SwiftUI Shape struct, Wave
. The way this shape works is by drawing a continuous wave from the leading to the trailing side of the frame. Wave
has two properties which can change how the shape looks:
这些动画都是从一个共同点开始的,那就是我自定义的SwiftUI Shape结构Wave
。 这种形状的工作方式是从框架的前侧到后侧绘制连续波。 Wave
具有两个可以更改形状外观的属性:
phase
- The phase at which the wave startsphase
-波浪开始的相位waveHeight
- The height (or really the amplitude) of the wave.waveHeight
的高度(或实际上是振幅)。
Here’s the code for the Wave
shape:
这是Wave
的代码:
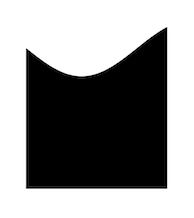
Wave
Shape
Wave
struct Wave: Shape {
var waveHeight: CGFloat
var phase: Angle
func path(in rect: CGRect) -> Path {
var path = Path()
path.move(to: CGPoint(x: 0, y: rect.maxY)) // Bottom Left
for x in stride(from: 0, through: rect.width, by: 1) {
let relativeX: CGFloat = x / 50 //wavelength
let sine = CGFloat(sin(relativeX + CGFloat(phase.radians)))
let y = waveHeight * sine //+ rect.midY
path.addLine(to: CGPoint(x: x, y: y))
}
path.addLine(to: CGPoint(x: rect.maxX, y: rect.midY)) // Top Right
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY)) // Bottom Right
return path
}
}
我们的第一个例子! (Our First Example!)
Yes, our first example! The Wave
shape is all we need to start creating some amazing interactions for our apps. Check out this one I made using two Wave
shapes placed inside a ScrollView
. Notice how when the user scrolls, the phase
of the waves are changed, and thus they appear to move across the screen.
是的,我们的第一个例子! Wave
形状是我们开始为我们的应用程序创建一些惊人的交互所需要的。 看看我用两个放置在ScrollView
内的Wave
形状制作的形状。 请注意,当用户滚动时,波形的phase
会发生变化,因此它们似乎在屏幕上移动。
var body: some View {
ScrollView(.vertical, showsIndicators: false) {
VStack(spacing: 0) {
// Other Views...
GeometryReader { geo in
ZStack {
Wave(waveHeight: 30, phase: Angle(degrees: (Double(geo.frame(in: .global).minY) + 45) * -1 * 0.7))
.foregroundColor(.orange)
.opacity(0.5)
Wave(waveHeight: 30, phase: Angle(degrees: Double(geo.frame(in: .global).minY) * 0.7))
.foregroundColor(.red)
}
}.frame(height: 70, alignment: .center)
// Other Views...
}
}
}
使用波浪作为遮罩 (Using the Wave as a Mask)
Another way to use the Wave
shape is to create a custom AnimatableModifier
that applies the shape as a mask to your view. The one noticeable change you'll see below is that the mask grows or shrinks in size according to the pct
property. Take a look at the code below before we get to our second example!
使用Wave
形状的另一种方法是创建一个自定义的AnimatableModifier
,将形状作为蒙版应用到视图。 您将在下面看到的一个明显变化是,遮罩的大小根据pct
属性而增大或缩小。 在进入第二个示例之前,请看下面的代码!
struct WaveMaskModifier: AnimatableModifier {
var pct: CGFloat
var animatableData: CGFloat {
get { pct }
set { pct = newValue }
}
func body(content: Content) -> some View {
content
.mask(
GeometryReader { geo in
VStack {
Spacer()
ZStack {
Wave(waveHeight: 30, phase: Angle(degrees: (Double(pct) * 720 * -1) + 45))
.opacity(0.5)
.scaleEffect(x: 1.0, y: 1.2, anchor: .center)
.offset(x: 0, y: 30)
Wave(waveHeight: 30, phase: Angle(degrees: Double(pct) * 720))
.scaleEffect(x: 1.0, y: 1.2, anchor: .center)
.offset(x: 0, y: 30)
}
.frame(height: geo.size.height * pct, alignment: .bottom)
}
}
)
}
}
我们的第二个例子! (Our Second Example!)
Yes, the second example! We’re moving along quickly today. Below you’ll see the animating mask as applied to an array of Image
views. When the user taps the screen, the WaveMaskModifer
is applied as a custom AnyTransition
to animate the change in the image displayed. This kind of transition can be useful for various photo/video-sharing apps that showcase those types of content.
是的,第二个例子! 我们今天进展很快。 在下面,您将看到应用于Image
视图阵列的动画蒙版。 当用户点击屏幕时, WaveMaskModifer
将作为自定义AnyTransition
应用,以动画方式显示显示的图像。 这种过渡对于展示这些类型的内容的各种照片/视频共享应用程序很有用。
I’ll provide the code for the transition below, in addition to the code for the example.
除了示例代码之外,我还将提供以下过渡代码。
WaveMaskModifier
作为过渡 (WaveMaskModifier
as a Transition)
Here is the code for the custom transition.
这是自定义转换的代码。
extension AnyTransition {
static let waveMask = AnyTransition.asymmetric(insertion:
AnyTransition.modifier(active: WaveMaskModifier(pct: 0), identity: WaveMaskModifier(pct: 1))
, removal:
.scale(scale: 1.1)
)
}
示例2的代码 (Code for Example 2)
Below is the code for the example above.
下面是上面示例的代码。
struct ContentView: View {
@State var index: Int = 0
var images: [Image] = [
Image("stock_1"),
Image("stock_2"),
Image("stock_3"),
Image("stock_4"),
]
var body: some View {
ZStack {
ForEach(images.indices) { i in
if i == index {
images[index]
.resizable()
.aspectRatio(contentMode: .fill)
.transition(.waveMask)
}
}
}.onTapGesture {
withAnimation(.easeOut(duration: 3)) {
index = (index + 1) % images.count
}
}.edgesIgnoringSafeArea(.all)
}
}
流动波背景 (Flowing Wave Background)
Finally, we’re going to use a set of Wave
shapes as a background for one of our views. The twist is that the wave will be animating in the background seamlessly to give the view some life while we wait for the user to interact with the screen. See below for the video example!
最后,我们将使用一组Wave
形状作为其中一个视图的背景。 扭曲之处在于,当我们等待用户与屏幕进行交互时,波浪将在背景中进行无缝动画处理,以赋予视图一些生命。 请参见下面的视频示例!
我们的第三个例子! (Our Third Example!)
By animating the phase
property of the Wave
shapes, we get this flowing background that presents itself nicely while waiting for user interaction.
通过对Wave
形状的phase
属性进行动画处理,我们得到了一个流畅的背景,在等待用户交互时很好地呈现了自己。
喜欢本教程吗? (Like this tutorial?)
告诉我们你做了什么! (Show us what you’ve made!)
Send us pics! Drop us a link! Anything! Find us on Twitter @TrailingClosure, on Instagram, or email us at
给我们发图片! 给我们一个链接! 随便啦! 在Twitter @TrailingClosure , Instagram或通过以下地址给我们发送电子邮件
翻译自: https://levelup.gitconnected.com/micro-interactions-animated-wave-14e0b74a69a4
android 交互动画