u8api openapi
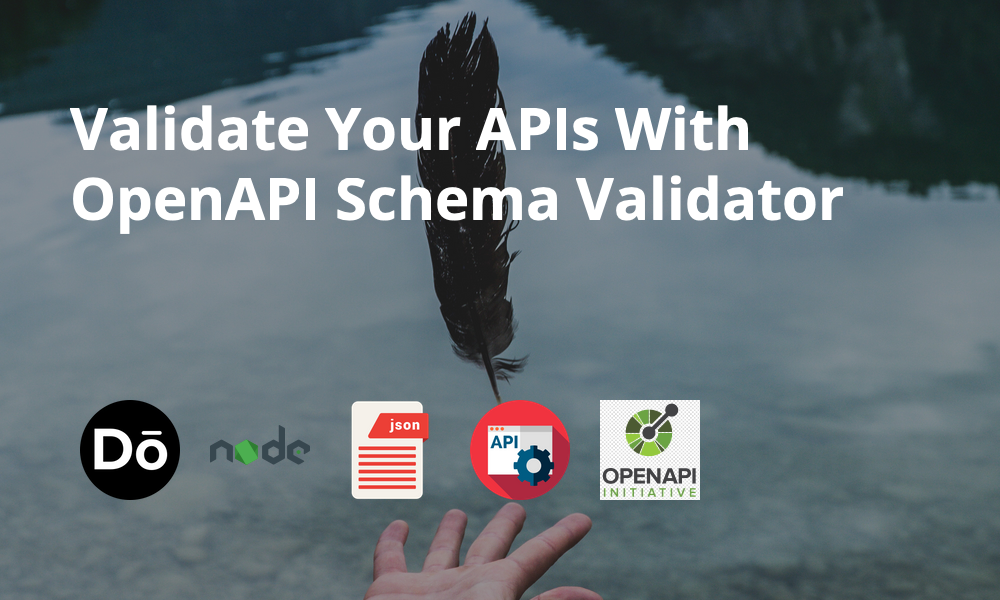
In the previous post, I went on a small spike to explore generating TypeScript types from valid JSON schemas. Now, I want to look a little deeper at OpenAPI Schema validation.
在上一篇文章中,我花了一点时间研究从有效的JSON模式生成TypeScript类型。 现在,我想更深入地了解OpenAPI Schema验证。
This example will build a little more on top of the previous post, however, it is not required reading.
这个例子将在前一篇文章的基础上再做一点,但是,不需要阅读。
入门 (Getting Started)
In a Yarn or NPM project directory, install the following:
在Yarn或NPM项目目录中,安装以下内容:
yarn add openapi-schema-validator
We are also going to add in a few of the pieces I wrote for the Book schema in the previous post, so create book.json
and add the following:
我们还将在上一篇文章中添加我为Book模式编写的一些内容,因此创建book.json
并添加以下内容:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"id": "#/components/schemas/Book",
"definitions": {
"user": {
"type": "object",
"properties": {
"name": { "type": "string" },
"preferredName": { "type": "string" },
"age": { "type": "number" },
"gender": { "enum": ["male", "female", "other"] }
},
"required": ["name", "preferredName", "age", "gender"]
}
},
"type": "object",
"properties": {
"author": { "$ref": "#/components/schemas/User" },
"title": { "type": "string" },
"publisher": { "type": "string" }
},
"required": ["author", "title", "publisher"]
}
The above is actually a little different to what I had in the previous blog posts, so make sure you do copy it across.
上面的内容实际上与我之前的博客文章有所不同,因此请确保将其复制。
We are going to import this in and using it for our values for a component.
我们将其导入并将其用于组件的值。
创建开放规范 (Creating the Open Specification)
I am awaiting the release of the OpenAPI 3.1 spec as it is the culmination of a huge effort to align the latest JSON Schema draft and the OpenAPI specification, but for now, we will run with 3.0.3.
我正在等待OpenAPI 3.1规范的发布,因为这是为使最新的JSON Schema草案和OpenAPI规范保持一致而付出的巨大努力的结晶,但是目前,我们将以3.0.3运行。
OpenAPI can be written in YAML or JSON, so, for now, we will keep things as JSON.
OpenAPI可以用YAML或JSON编写,因此,到目前为止,我们将其保留为JSON。
Basically I just copied across the example they gave for using components and transformed it from YAML to JSON. Then I made some adjustments to check for a path /books
where the GET request expects back an array of type Books.
基本上,我只是复制了他们给出的使用组件的示例,并将其从YAML转换为JSON。 然后,我进行了一些调整,以检查GET请求期望返回类型为Books的数组的路径/books
。
{
"openapi": "3.0.3",
"info": {
"title": "Sample API",
"description": "Optional multiline or single-line description in [CommonMark](http://commonmark.org/help/) or HTML.",
"version": "0.1.0"
},
"paths": {
"/books": {
"get": {
"summary": "Get all books",
"responses": {
"200": {
"description": "A list of books",
"content": {
"application/json": {
"schema": {
"type": "array",
"items": {
"$ref": "#/components/schemas/Book"
}
}
}
}
}
}
}
}
}
}
设置验证器(Setting Up The Validator)
Add the following to index.js
:
将以下内容添加到index.js
:
const OpenAPISchemaValidator = require("openapi-schema-validator").default
const openAPIValidator = new OpenAPISchemaValidator({
version: 3,
})
const fs = require("fs")
const path = require("path")
const main = async () => {
// read the schema details
const schemaFilepath = path.join(__dirname, "book.json")
const bookSchema = JSON.parse(fs.readFileSync(schemaFilepath, "utf-8"))
// Validating the OpenAPI
const openApiJsonFilepath = path.join(__dirname, "openapi.json")
const openApiSchema = JSON.parse(
fs.readFileSync(openApiJsonFilepath, "utf-8")
)
// Adjust the openApiSchema to use the definitions from `book.json`.
openApiSchema.components = {
schemas: {
User: bookSchema.definitions.user,
Book: {
type: bookSchema.type,
properties: bookSchema.properties,
required: bookSchema.required,
},
},
}
const res = openAPIValidator.validate(openApiSchema)
if (res.errors.length) {
console.error(res.errors)
process.exit(1)
}
}
main()
The most confusing part here may be where I am adjusting the Open API Schema to use the definitions from book.json
. I am doing this is keep in line with what I was doing with combining the other JSON files. I am thinking in my own work that I may follow the precedent of splitting up component definitions and combining when required.
这里最令人困惑的部分可能是我正在调整Open API Schema以使用book.json
的定义。 我这样做与我结合其他JSON文件所做的保持一致。 我在自己的工作中认为,我可以遵循先例进行拆分,然后在需要时进行合并。
运行验证器 (Running The Validator)
Run node index.js
. If nothing happens, then perfect!
运行node index.js
。 如果什么都没有发生,那就完美了!
If you want to test the validity is doing what it needs, adjust a value in openapi.json
and see what happens.
如果要测试有效性是否在做所需的工作,请在openapi.json
调整一个值,然后看看会发生什么。
I changed "description": "A list of books",
into "descriptions": "A list of books",
. Running the file again will give us the following logged out:
我将"description": "A list of books",
更改为"descriptions": "A list of books",
。 再次运行该文件将使我们注销:
> node index.js
[
{
keyword: 'additionalProperties',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/additionalProperties',
params: { additionalProperty: 'descriptions' },
message: 'should NOT have additional properties'
},
{
keyword: 'required',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/required',
params: { missingProperty: 'description' },
message: "should have required property 'description'"
},
{
keyword: 'additionalProperties',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/additionalProperties',
params: { additionalProperty: 'descriptions' },
message: 'should NOT have additional properties'
},
{
keyword: 'additionalProperties',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/additionalProperties',
params: { additionalProperty: 'content' },
message: 'should NOT have additional properties'
},
{
keyword: 'required',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/required',
params: { missingProperty: '$ref' },
message: "should have required property '$ref'"
},
{
keyword: 'oneOf',
dataPath: ".paths['/books'].get.responses['200']",
schemaPath: '#/oneOf',
params: { passingSchemas: null },
message: 'should match exactly one schema in oneOf'
}
]
Success! Now we have a way to validate our OpenAPI schema.
成功! 现在,我们有一种方法来验证我们的OpenAPI模式。
资源和进一步阅读 (Resources and Further Reading)
Image credit: Markos Mant
图片来源: Markos Mant
Originally posted on my blog.
最初发布在我的博客上。
翻译自: https://medium.com/@dennisokeeffe/validate-your-apis-with-openapi-schema-validator-4a34c8bc5f6e
u8api openapi