测试驱动开发tdd
When it comes to programming, testing your code is just as important to a developer as the actual code itself. Some may argue that it’s even more important. Why is that, you ask? Writing your own test before you code forces you to think about how it should work. This can help you cut down on time during development, isolate specific problems or bugs, and can even avoid regressions whenever new features are added. This blog will define what TDD is and introduce the “Red, Green, Refactor” principle.
在编程方面,测试代码对开发人员而言与实际代码本身同样重要。 有人可能会说它甚至更重要。 你问为什么呢? 在编写代码之前编写自己的测试会迫使您考虑一下它应该如何工作。 这可以帮助您减少开发过程中的时间,隔离特定的问题或错误,甚至可以避免在添加新功能时出现退化。 该博客将定义TDD是什么,并介绍“红色,绿色,重构”原则。
什么是TDD? (What is TDD?)
TDD, or Test-Driven Development, is a coding pattern that helps programmers structure their code in an incremental approach. It is writing the test code, also known as a test-suite before you start writing code. The goal here is to force you to think about how something should work so you can build the code piece by piece.
TDD或测试驱动开发是一种编码模式,可以帮助程序员以增量方式构造其代码。 它是在开始编写代码之前编写测试代码,也称为测试套件。 这里的目标是迫使您考虑应该如何工作,以便您可以逐步构建代码。
Below is an example of a test block written in RSpec, the behavior-driven development, or BDD, language for Ruby. (Note TDD and BDD have slight differences but the over-arching concept is the same — using the test suite to drive development). We’ll use this example because the syntax is very readable and can help you grasp the idea quickly, in my opinion.
下面是用RSpec编写的测试块的示例,RSpec是针对Ruby的行为驱动开发或BDD语言。 (注意,TDD和BDD略有不同,但是总体概念是相同的-使用测试套件来驱动开发)。 我们将使用此示例,因为该语法可读性强,并可以帮助您快速理解该想法。
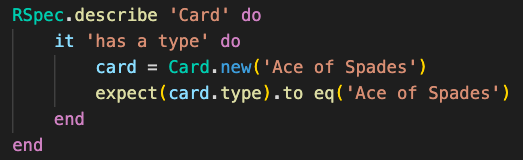
I won’t go into detail about the syntax of the language, but this example tests for a Card class to have a type called “Ace of Spades” when a new instance is created. To make this test pass, we can approach it incrementally and:
我不会详细介绍该语言的语法,但是此示例将在创建新实例时测试Card类是否具有名为“黑桃A”的类型。 要使此测试通过,我们可以逐步进行测试并:
- Create a Card class 创建卡类
- Initialize it with an instance variable called ‘type’ 使用名为“ type”的实例变量对其进行初始化
- Add a getter method to get the value 添加getter方法以获取值
Let’s execute the above test block:
让我们执行上面的测试块:

When executing this test block before the code is written, it failed. This is obvious because there is no code to test. The test threw a “NameError: uninitialized constant Card” because we haven’t created the Card class yet.
在编写代码之前执行此测试块时,它失败。 这很明显,因为没有要测试的代码。 该测试引发了“ NameError:未初始化的常量Card”,因为我们尚未创建Card类。
Let’s solve this NameError by creating the Card class. Once we created a Card class, following the TDD pattern, we would then execute the same test block. Here is the result:
让我们通过创建Card类来解决此NameError问题。 按照TDD模式创建Card类后,我们将执行相同的测试块。 结果如下:

A new error! This means that we successfully created the Card class and can move on to the next piece of the puzzle, which is solving the ArgumentError.
一个新的错误! 这意味着我们成功创建了Card类,并且可以继续进行下一个难题,即解决ArgumentError。
This pattern of executing the test block, reading the error, solving the problem, and moving on to a new error is known as TDD. The developer is using the test suite they created to help guide them during development by building the code incrementally — piece by piece. If we continued on with the above example by following the steps we laid out earlier, we would get the test to pass. This means we have successfully met the test block expectations and created the correct code we sought out to make.
执行测试块,读取错误,解决问题并转移到新错误的这种模式称为TDD。 开发人员正在使用他们创建的测试套件,通过逐步构建代码来逐步指导开发过程。 如果我们按照前面列出的步骤继续执行上面的示例,我们将通过测试。 这意味着我们已经成功满足了测试块的期望,并创建了我们想要制作的正确代码。

红色,绿色,重构 (Red, Green, Refactor)
The core pattern of TDD is also known as the ‘Red, Green, Refactor’ principle. Red corresponds to the errors you predictably will receive because the corresponding code hasn’t been written correctly. Green corresponds to the bare minimum code you have to write in order to make the test pass. After getting the green pass, you can refactor your code for optimization and preferred style. When refactoring your code, a red error message may occur and then you would have to re-write your code to get the green message again. This is repeated until you are happy with the refactor and the code still passes the test-suite.
TDD的核心模式也称为“红色,绿色,重构”原理。 红色对应您可以预见的错误,因为相应的代码未正确编写。 绿色对应于您必须编写以通过测试的最低限度的代码。 获得绿色通行证后,您可以重构代码以进行优化和首选样式。 重构代码时,可能会出现红色错误消息,然后您必须重新编写代码才能再次获得绿色消息。 重复此过程,直到您对重构感到满意并且代码仍然通过测试套件为止。
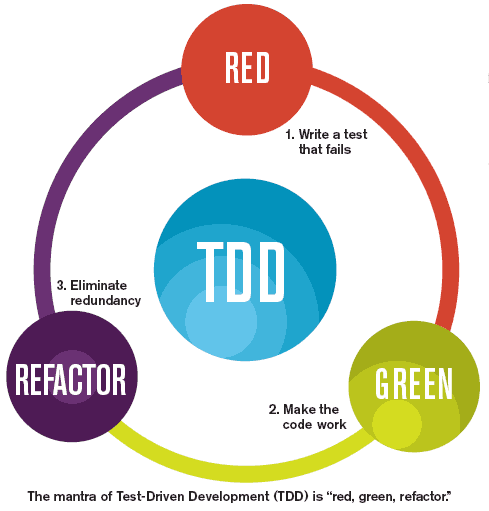
TDD is a powerful tool that should be used by every programmer. It will help guide you during development so you can stay focused and efficient when writing code.
TDD是每个程序员都应使用的功能强大的工具。 它会在开发过程中为您提供指导,以便您在编写代码时保持专注和高效。
Look out for a follow-up blog that explains different types of tests you can use when writing a test-suite and which one is better. The short answer — ‘it depends.’
寻找一个后续博客,该博客介绍编写测试套件时可以使用的不同测试类型,哪种更好。 简短的答案-“取决于情况。”
Happy coding 😄
快乐编码😄
测试驱动开发tdd