java懒惰加载
Third-party libraries, images and huge amount of static data can all influence your application bundle size. This can cause unexpected high loading times, which may lead to a bad first site impression. React.Lazy and React.Suspense are common techniques (as of mid 2020), to perform code splitting for bundle size reduction and speeding up page load. In this article I want to show quick you may add code splitting to your React application, highlighting the differences in performance (Lighthouse benchmark/check).
第三方库,图像和大量静态数据都可能影响应用程序捆绑包的大小。 这可能会导致意外的高加载时间,从而可能导致不良的第一站点印象。 React.Lazy和React.Suspense是常用技术(截至2020年中期),用于执行代码拆分以减小包大小并加快页面加载。 在本文中,我想快速展示一下您可以将代码拆分添加到React应用程序中,突出显示性能差异(灯塔基准测试/检查)。
基础应用 (The base application)
The idea is that we have a React component, that just displays some static data from a JSON file. I have chosen the programming-quotes-api in order to have some data that makes sense. This data is not being fetched at runtime, but put into a local JSON file, which means it will be bundled into the application. To make the data a bit bigger, I have duplicated its content.
这个想法是,我们有一个React组件,它只显示JSON文件中的一些静态数据。 我选择了programming-quotes-api ,以便获得一些有意义的数据。 不会在运行时获取此数据,而是将其放入本地JSON文件中,这意味着它将被捆绑到应用程序中。 为了使数据更大一些,我复制了其内容。
The app boilerplate was created by the common create-react-app
tool as described here. From there on, I have created a React component, call it VeryBigJokesList
, that displays the static content.
该应用程序的样板是由共同创建create-react-app
所描述的工具在这里 。 从那里开始,我创建了一个React组件,将其VeryBigJokesList
,它显示了静态内容。
import React from 'react'
import preDefinedJokes from './preDefinedJokes.json'
const VeryBigJokesList = ({ jokes = preDefinedJokes }) => {
if (!Array.isArray(jokes)) {
return <p>No jokes found.</p>
}
return (
<ul>
{
jokes.map((joke, i) => <li key={i}>{joke && joke.en}</li>)
}
</ul>
);
}
export default VeryBigJokesList;
非懒惰(急切)情况 (The non-lazy (eager) case)
Usually, I would just import the VeryBigJokesList
component and render it in the App
component, created by the boilerplate.
通常,我只是导入VeryBigJokesList
组件并将其呈现在样板创建的App
组件中。
import * as React from 'react';
import VeryBigJokesList from './VeryBigJokesList';
function App() {
return (
<div className="App">
<header className="App-header">
<div style={{ maxWidth: 600 }}>
<VeryBigJokesList />
</div>
</header>
</div>
);
}
export default App;
This causes to the user load all content from VeryBigJokesList
when loading App
, since it will be "placed" in the same final bundle. When building the application via yarn build
or npm run build
, you will see a list of all bundled files of your application.
这将导致用户在加载App
时从VeryBigJokesList
加载所有内容,因为它将“放置”在同一最终捆绑包中。 通过yarn build
或npm run build
应用程序时,您将看到应用程序所有捆绑文件的列表。
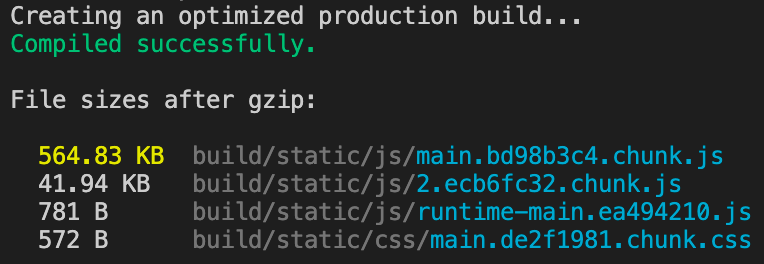
As you can see, the main bundle is yellow highlighted, indicating that its size may be too big. This makes sense, since the JSON data that VeryBigJokesList
includes is roughly this size. When running a Lighthouse performance check, you should also see some loading specific issues.
如您所见,主捆绑包以黄色突出显示,表明它的大小可能太大。 这是有道理的,因为VeryBigJokesList
包含的JSON数据大约是这个大小。 运行Lighthouse性能检查时,您还应该看到一些特定于加载的问题。
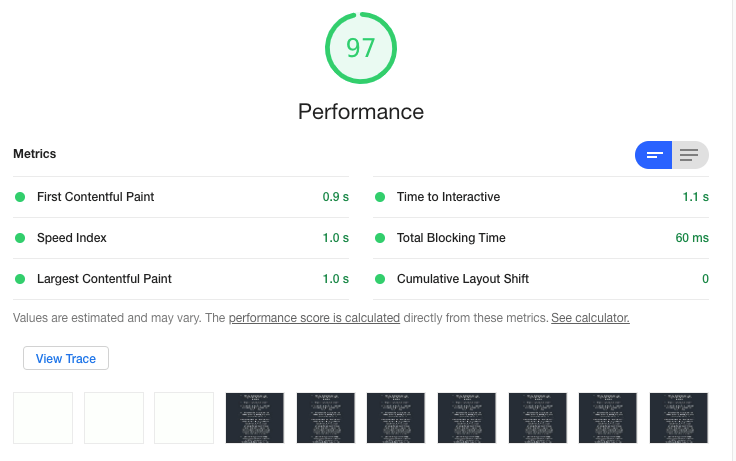
懒惰的情况 (The lazy case)
When planning to use React.Lazy, you mostly need to consider the fact that VeryBigJokesList
is being exported via export default
and is put as a child (of any depth) of a React.Suspense component. Suspense allows you to render a fallback component (like a loading indicator), while its content is loading.
计划使用React.Lazy时 ,您最需要考虑的事实是, VeryBigJokesList
是通过export default
导出的,并且被放置为React.Suspense组件的子级(任何深度)。 挂起允许您在加载内容时渲染后备组件(如加载指示器)。
import * as React from 'react';
const VeryBigJokesList = React.lazy(() => import('./VeryBigJokesList'));
function App() {
return (
<div className="App">
<header className="App-header">
<div style={{ maxWidth: 600 }}>
<React.Suspense fallback={<p>Loading list...</p>}>
<VeryBigJokesList />
</React.Suspense>
</div>
</header>
</div>
);
}
export default App;
Adjusting VeryBigJokesList
to load lazily was rather simple. If everything worked well, you should see a loading text, before the list is displayed. When building the application, you should also see a difference.
调整VeryBigJokesList
以延迟加载非常简单。 如果一切正常,则在显示列表之前,您应该会看到一个加载文本。 在构建应用程序时,您还应该看到区别。
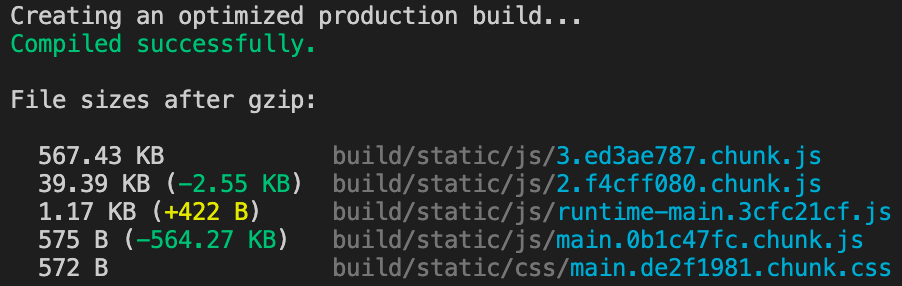
The main bundle size has decreased dramatically, since the static JSON content has moved to a different chunk of the bundle. When running a Lighthouse performance check, you should see a difference in loading times.
由于静态JSON内容已移动到捆绑包的其他块中,因此主要捆绑包的大小已大大减少。 运行Lighthouse性能检查时,您应该看到加载时间有所不同。
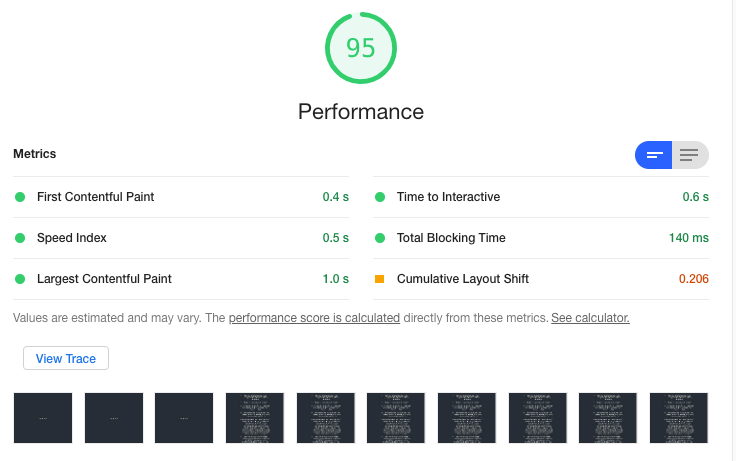
Originally published at https://mariusreimer.com on July 26, 2020.
最初于 2020年7月26日 在 https://mariusreimer.com 上 发布 。
翻译自: https://medium.com/@reime005/faster-react-page-loads-with-lazy-and-suspense-62f04d793cb7
java懒惰加载