虚拟dom和直接操作dom
DOM Manipulation can make web applications customizable and the data of the webpage can be updated without refreshing the page. By DOM manipulation we can add, change and remove any HTML elements or attributes and CSS styles associated with it. Any event can also be attached to the element.
DOM操作可以使Web应用程序可自定义,并且可以在不刷新页面的情况下更新网页的数据。 通过DOM操作,我们可以添加,更改和删除任何HTML元素或与之关联的属性和CSS样式。 任何事件也可以附加到元素上。
Let’s learn what is the DOM and how it is created.
让我们学习什么是DOM以及如何创建它。
什么是DOM? (What is the DOM?)
The DOM (Document Object Model) is an object oriented representation of an HTML or XML document in a logical tree structure where each node contains an object which represents a part of the document.
DOM(文档对象模型)是逻辑树结构中HTML或XML文档的面向对象的表示,其中每个节点都包含一个代表文档一部分的对象。
Node can also have events attached to them which when triggered the event handler attached to them is executed. The DOM also acts as an interface between JavaScript and the HTML or XML document which allows creation of customizable web pages.
节点还可以具有附加到其上的事件,这些事件在触发时将执行附加到其上的事件处理程序。 DOM还充当JavaScript与HTML或XML文档之间的接口,该接口允许创建可定制的网页。
DOM是如何创建的? (How is DOM created?)
The browser read the HTML of webpage in raw bytes format and translate them to individual characters. The string of characters is then converted into distinct tokens as specified by W3C. The tokens for string between angle brackets <> has a special meaning and has its own set of rules. After tokens are produced they are converted into objects which has their properties and rules. These objects are structured in a tree structure with parent-child relationship according to the tags in HTML markup which is the DOM.
浏览器以原始字节格式读取网页HTML,并将其转换为单个字符 。 然后,将字符串转换为W3C指定的不同标记 。 尖括号<>之间的字符串标记具有特殊含义,并且具有自己的规则集。 生成令牌后,它们将被转换为具有其属性和规则的对象 。 这些对象根据HTML标记(即DOM)中的标签以具有父子关系的树结构进行构造。
The process is as follows:
流程如下:

DOM操作方法 (DOM Manipulation Methods)
DOM methods allows access to the tree, with which we can make changes in the DOM structure and manipulate the DOM as required. Following are the most common methods and the ways to manipulate DOM.
DOM方法允许访问树,通过它我们可以更改DOM结构并根据需要操作DOM。 以下是最常见的方法和操作DOM的方法。
document.createElement(tagName) - Dynamically creates new node of HTML elements within your JavaScript code.
document.createElement(tagName)-在JavaScript代码中动态创建HTML元素的新节点。
var paraEle = document.createElement(‘p’);
document.createTextNode(text)- Create a text node.
document.createTextNode(text)- 创建一个文本节点。
var node = document.createTextNode(“This is a para”);
document.createDocumentFragment()- Document Fragment is basically the virtual invisible HTML element. .createDocumentFragment() is used to create the document fragment, append elements to the document fragment and then append the document fragment to the DOM tree. In the DOM tree, the document fragment is replaced by all its children.
document.createDocumentFragment()-文档片段基本上是虚拟的不可见HTML元素。 .createDocumentFragment() 用于创建文档片段,将元素附加到文档片段,然后将文档片段附加到DOM树。 在DOM树中,文档片段被其所有子元素替换。
var fragment = document.createDocumentFragment();
If we are writing a page that does a large amount of DOM manipulation, using this method can dramatically increase performance of your page. Appending children to a fragment prevents the reflow of UI which increases the performance and because of this createElement() is differentiated from createDocumentFragment().
如果我们要编写执行大量DOM操作的页面,则使用此方法可以显着提高页面的性能。 在片段上添加子元素可防止UI回流,从而提高性能,并且由于此createElement()与createDocumentFragment()有所区别。
parentElement.appendChild(newElement) : This method inserts the child node(passed in argument) as the last child node of the parent element. i.e. it appends the child node element to the parent node element
parentElement.appendChild(newElement) :此方法将子节点(传入参数)作为父元素的最后一个子节点插入。 即它将子节点元素附加到父节点元素
paraEle.appendChild(node);
fragment.appendChild(paraEle);document.body.appendChild(fragment);
parentElement.insertBefore(newElement, element) : Allows to insert the new child node element (passed as first argument) in the existing parent node element before one of its child node(passed as second argument) in the parent element.
parentElement.insertBefore(newElement,element) :允许在现有父节点元素中将新的子节点元素(作为第一个参数传递)插入到父元素中其子节点之一(作为第二个参数传递)之前。
const list = document.createElement('ul');
document.body.insertBefore(list, paraEle);
Node.setAttribute(name, value) : Sets the attribute of element in the HTML dynamically.
Node.setAttribute(名称,值) : 动态设置HTML中element的属性。
paraEle.setAttribute('class','paragraph');
Then we can set CSS for class paragraph and the styles will be applied to the element.
然后,我们可以为类段落设置CSS,并将样式应用于元素。
Node.textContent() : Allows to set and get the text content of the node (commonly known as element).
Node.textContent() : 允许设置和获取节点(通常称为元素)的文本内容。
const para = document.getElementByClassName('paragraph');
console.log(para.textContent); // console the para text
para.textContent = "This is the changed paragraph" ; /*set the paragraph text*/
Node.innerHTML() : Set the inner HTML content.
Node.innerHTML() :设置内部HTML内容。
para.innerHTML = '<em>This is the changed paragraph</em>'; // text will be italics
The difference between innerHTML() and textContent() is that innerHTML() can set both HTML tags and text inside the element but in text Content() only text can be set.
innerHTML()和textContent()之间的区别在于innerHTML()可以在元素内设置HTML标签和文本,但在文本Content()中只能设置文本。
Node.classList : Allows you to modified the classes attached to the element.
Node.classList :允许您修改附加到元素的类。
console.log(paraEle.classList);
//console all the classes attachedparaEle.classList.add('myClass')
//styles of myClass class will be appliedparaEle.classList.remove('myClass');
// remove the classparaEle.classList.replace('myClass1','myClass2');
//replaces one class with other;paraEle.classList.toggle('myClass')
//if class present removes it, else if not present attaches the class to the element;paraEle.classList.contains('myClass')
//returns true or false whether the class is in the classList or not
Node.dataset : Allows you to set and get the values of custom data attributes that we have on HTML elements.
Node.dataset :允许您设置和获取我们在HTML元素上拥有的自定义数据属性的值。
//HTML file
<div id="myDiv" data-name="Item" data-item-price="10"></div>//JavaScript
const myDiv = document.getElementById('myDiv');console.log(myDiv.dataset)
//{name:"Iteml", item-price:"10"}myDiv.dataset.name = "new Item1"
//changes the name property of myDiv.removeAttribute('data-name')
//removes the name attribute of data
Node.cloneNode : Allows to make a copy or clone on a node.
Node.cloneNode :允许在节点上进行复制或克隆。
var paraCopy = paraEle.cloneNode();
//It doesn't include the childNode by default it only copies the current node which is <p></p>var paraCopy = paraEle.cloneNode(true);
//deep copy happends i.e. childNode will also be copieddocument.body.appendChild(paraCopy);
Node.nextElementSibling : gives next element of the element you are calling on.
Node.nextElementSibling :给出您正在调用的元素的下一个元素。
Node.nextSibling : gives the next Node. It also considers any type of nodes including text nodes but nextElementSibling doesn’t.
Node.nextSibling : 给出下一个节点。 它还考虑任何类型的节点,包括文本节点,但nextElementSibling则不考虑。
Element.getBoundingClientRect() : This method will return an object containing the properties of an element such as width, height, top, left, right, bottom value as well as x, y -coordinates.
Element.getBoundingClientRect() :此方法将返回一个对象,该对象包含元素的属性,例如宽度,高度,顶部,左侧,右侧,底部值以及x,y坐标。
console.log(paraEle.getBoundingClientRect());
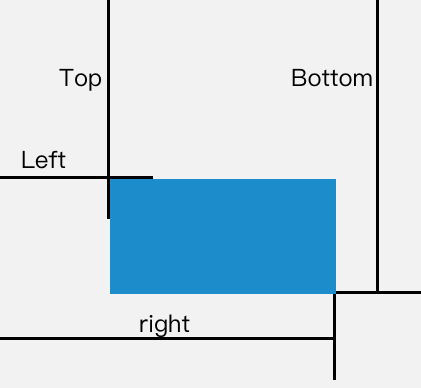
Element.outerHTML : Gives the outer HTML that is element tag including the id, classes, etc.
Element.outerHTML :提供作为元素标签的外部HTML,包括id,class等。
document.getElementById(idname) : lets you find the element with the provided Id
document.getElementById( idname ) :让您查找具有提供的ID的元素
document.getElementByClassName(classname) : lets you find the element with the provided ClassName
document.getElementByClassName( classname ) :让您查找具有提供的ClassName的元素
Element.querySelector(selector) : lets you find the first Element that matches the CSS selector.
Element.querySelector( selector ) :让您找到与CSS选择器匹配的第一个Element。
document.querySelector('#myDiv');
//gives element with the above selector i.e. id of myDivdocument.querySelector('.myClass');
//gives element with the above selector i.e. class of myClass
Element.querySelectorAll(selector)- lets you find a list of the elements that matches the CSS selector.
Element.querySelectorAll( 选择器 )-可让您找到与CSS选择器匹配的元素列表。
document.querySelectorAll('.myClass');
//gives the array of element with the above selector
window.parent : document is equal to window.parent.document referring to our HTML document
window.parent :文档等于引用我们HTML文档的window.parent.document
Before the browser render the page, DOM is constructed. As a result, we need to ensure that HTML is delivered quickly to the browser as possible. Excessive DOM manipulation results in rendering the page again and again causing the reflow of UI which is an expensive and costs performance.
在浏览器呈现页面之前,将构建DOM。 因此,我们需要确保将HTML尽快传递到浏览器。 过多的DOM操作导致一次又一次呈现页面,从而导致UI回流,这既昂贵又降低了性能。
Therefore we must ensure there is no excessive DOM manipulation. Also, there are some libraries such as React that use a Virtual DOM, so that performance is not affected and the webpages are dynamic.
因此,我们必须确保没有过多的DOM操作。 此外,还有一些库(例如React)使用虚拟DOM,因此不会影响性能,并且网页是动态的。
翻译自: https://medium.com/javascript-in-plain-english/dom-manipulation-985995eb858a
虚拟dom和直接操作dom