自己写富文本编辑器jss
JSS is a JavaScript library that allows you to create objects that follow a CSS-like syntax for styling components. More technically, from the JSS docs, JSS lets you “use JavaScript to describe styles in a declarative, conflict-free and reusable way”.
JSS是一个JavaScript库,允许您创建对象,这些对象遵循类似于CSS的语法来设计组件样式。 从技术上讲,从JSS文档开始 ,JSS允许您“使用JavaScript以声明性,无冲突且可重用的方式描述样式”。
Many JavaScript libraries make use of JSS (also known as CSS-In-JS). As a React developer, I use it daily in place of regular CSS. Attempting to properly style and customize Material-UI components has also encouraged my use of JSS.
许多JavaScript库都使用JSS(也称为CSS-In-JS)。 作为一个React开发人员,我每天都用它代替常规CSS。 尝试正确设置样式和自定义Material-UI组件也鼓励了我使用JSS。
In fact, bumping into disabled, hovered, or otherwise special-state Material-UI components is what pushed me to write this article: even armed with knowledge of CSS syntax, it can be hard to get the selectors correct with JSS.
实际上,碰到禁用,悬停或其他特殊状态的Material-UI组件是促使我写这篇文章的原因:即使拥有CSS语法知识,也很难通过JSS来使选择器正确。
In this article, I will give a few examples of selecting pseudo-classes and child elements with JSS. If you want an example using Material-UI, see this article. Here is the CodeSandbox for the below examples.
在本文中,我将提供一些使用JSS选择伪类和子元素的示例。 如果要使用Material-UI的示例, 请参阅本文 。 这是下面示例的CodeSandbox 。
JSS一般规则和语法 (JSS General Rules and Syntax)
·JSS uses camel-case instead of dashes (i.e. fontWeight instead of font-weight)
·JSS使用驼峰大小写而不是破折号(即,用fontWeight代替font-weight)
·There is no ‘.’ in front of class names (i.e. buyButton instead of .buyButton)
·没有“。” 在类名的前面(即buyButton而不是.buyButton)
·Imports and other variables are fine. Remember, this is JavaScript
·导入和其他变量都可以。 记住,这是JavaScript
·Other JavaScript syntax is fine (i.e. object destructuring)
·其他JavaScript语法也可以(例如,对象分解)
Remember that when writing JSS, you are creating a JavaScript object. Therefore, all normal JavaScript Syntax applies.
请记住,在编写JSS时,您正在创建一个JavaScript对象。 因此,所有普通JavaScript语法都适用。
示例1 —:hover伪类的JSS选择器 (Example 1 — JSS selector for :hover pseudo-class)
Let’s add a :hover selector to a button. The elements are below (using JSX).
让我们在按钮上添加一个:hover选择器。 元素如下(使用JSX)。
<form>
<button className={classes.buyButton}>
Click Me!
</button>
</form>
And here is the JSS:
这是JSS:
buyButton: {
backgroundColor: 'blue',
width: 200,
height: 80,
borderRadius: 4,
fontSize: 24,
'&:hover': {
border: '4px solid red',
cursor: 'pointer'
}
}
Notice the & has no space after it. This makes sense because we are not selecting a hovered child of buyButton.
注意&后面没有空格。 这是有道理的,因为我们没有选择buyButton的悬浮子项。
示例2 —适用于一个元素的两个伪类的JSS选择器 (Example 2 — JSS selector for two pseudo-classes applied to one element)
This time let’s resize a checked and disabled checkbox. The JSX:
这次让我们重新调整选中和禁用复选框的大小。 JSX:
<div>
<input
className={classes.personChecker}
type='checkbox'
id='person1'
name='person1'
value='Person'
checked={true}
disabled={true}
/>
<label for='person1'> I am a person</label>
</div>
And the JSS:
和JSS:
personChecker: {
'&:checked:disabled': {
width: 20,
height: 20
}
}
Notice once again that there are no spaces. There is also only one ‘&’.
再次注意,没有空格。 也只有一个“&”。
示例3 —嵌套伪类 (Example 3 — Nested pseudo-classes)
Here is an example of what the code would look like for a nested pseudo-class selector. This one is not in the CodeSandbox.
这是一个嵌套伪类选择器的代码示例。 此代码不在CodeSandbox中。
buyButton: {
'&:hover': {
backgroundColor: 'blue',
'&:before: {
textDecoration: 'bold',
}
}
}
This nested pseudo-selector allows some styles to be applied on hover (backgroundColor in this case) with separate styling applied on both :hover and :before.
此嵌套的伪选择器允许将某些样式应用于悬停(在本例中为backgroundColor),同时将单独的样式应用于:hover 和 :before。
示例4 —子选择器 (Example 4 — Child selector)
Let’s select all spans in the form. JSX:
让我们选择表单中的所有跨度。 JSX:
<form className={classes.form}>
<span>Child span</span>
<span>Another child span</span>
</form>
JSS:
JSS:
form: {
"& span": {
backgroundColor: "red",
margin: 4
}
}
Notice how I had to use form as a class.
请注意,我必须如何将表单用作类。
React Material-UI (React Material-UI)
If you are a React developer and looking for a good component library, I recommend using Material-UI. Material-UI uses the JSS syntax above for styling components. If you want to gain an in-depth understanding of Material-UI syntax and APIs for styling components, I recommend you read my book, Styling Material-UI Components (affiliate link). I cover the class object API, Styled Components, and overriding components using the theme. Furthermore, there are many examples of custom component styling.
如果您是React开发人员,并且正在寻找一个好的组件库,我建议您使用Material-UI。 Material-UI使用上面的JSS语法对组件进行样式设置。 如果您想深入了解Material-UI语法和用于样式化组件的API,建议您阅读我的书, 样式化Material-UI组件 (关联链接)。 我将介绍使用主题的类对象API,样式化组件和重写组件。 此外,还有许多自定义组件样式的示例。
Read it for FREE with a free Kindle Unlimited trial membership (affiliate link).
用读它免费 免费的Kindle无限试用会员 (会员链接)。
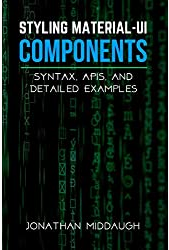
Are you a developer looking to start your first side hustle? Learn how here.
您是否正在寻找开始第一手的开发人员? 在这里了解如何 。
翻译自: https://medium.com/the-clever-dev/jss-selectors-and-syntax-rules-e4008e07966b
自己写富文本编辑器jss