javascript闭包
JavaScript Basics is a series that explore some core concepts that every frontend software engineer should understand. Those concepts are not only important for success in job interviews but also for a career as a developer.
“ JavaScript基础知识”系列探讨了每个前端软件工程师都应理解的一些核心概念。 这些概念不仅对于求职面试的成功很重要,而且对于开发人员的职业也很重要。
In this section of our JavaScript Basics Series, we will explain a very important concept known as closure. What makes it particularly important that it is not only a core concept of JavaScript itself but also very likely to come up in JavaScript-related interviews. Without further ado let’s get into it! 😃
在我们JavaScript基础系列的这一部分中,我们将解释一个非常重要的概念,称为闭包。 尤其重要的是,它不仅是JavaScript本身的核心概念,而且很可能出现在与JavaScript相关的访谈中。 事不宜迟,让我们开始吧! 😃
What is Closure?
什么是封包?
One of the better definitions of this is the one provided by Mozilla’s web docs:
更好的定义之一是Mozilla的网络文档提供的定义:
A closure is a combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). In other words, a closure gives you access to an outer function’s scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
的 封闭件 是捆绑在一起的函数的组合(封闭)与到其周围的状态的引用( 词法环境 )。 换句话说,闭包使您可以从内部函数访问外部函数的范围。 在JavaScript中,每次创建函数时都会在函数创建时创建闭包。
In other words, it’s the fact of putting a function inside of another, which gives the enclosed function access to the outer one’s variables. The term ‘lexical environment’ is referring to the scope of the outer function in this case. Let’s illustrates this with an example:
换句话说,这是将一个函数放在另一个内部的事实,这使封闭的函数可以访问外部一个变量。 在这种情况下,术语“词汇环境”是指外部功能的范围。 让我们用一个例子来说明这一点:
function outer(){
let outerVar = "outside"; function inner(){ let innerVar = "inside";
console.log("The outer variable is " + outerVar); } return inner;
}
Output: [Function: inner]
输出: [Function: inner]
In JavaScript, any function that is executed creates a closure by default
在JavaScript中,默认情况下,执行的任何函数都会创建一个闭包
The inner function has access to the outer function’s variable because it is within its local or lexical scope. More specifically, I’d like to point out the following points:
内部函数可以访问外部函数的变量,因为它在其局部或词法范围内。 更具体地说,我想指出以下几点:
The inner has access to the outer function’s variable, whereas the latter doesn’t have access to innerVar. Lexical scope is a one-way street only the inner function can access anything in the outer function.
内部变量可以访问外部函数的变量,而后者不能访问innerVar 。 词法作用域是一条单向路,只有内部功能可以访问外部功能中的任何内容。
- The outer function can only get results (return value, console output, etc) from the inner function by returning it. 外部函数只能通过返回内部函数来获取结果(返回值,控制台输出等)。
Only the function definition is returned, not the invocation! The inner function is not called.
仅返回函数定义 ,不返回调用! 内部函数未调用。
The inner function is returned from the outer function before being executed.
内部函数在执行之前从外部函数返回。
This means that technically it shouldn’t be able to access the outer function’s variables. In many programming languages, all the variables of an outer function would have been declared and continued to exist up until the point where the function returns a value (the inner function in this case). When a function is called, it creates a call (or execution) stack, which is simply specialized allocated memory space. Each call creates its own execution stack with a set of local variables. In traditional languages like C/C++, for example, calling the inner function would create its own memory stack (see diagram below). Hence, the inner function would not have access to any variables outside of its own scope. However, in JavaScript, every function that is executed creates a closure. In other words, every function is created with the ability to enclose functions inside of it, this enclosure gives their inner functions access to all variables within them. Therefore, closure is simply the combination of a function and its lexical scope.
这意味着从技术上讲,它不应访问外部函数的变量。 在许多编程语言中,外部函数的所有变量都会被声明并继续存在,直到该函数返回值为止(在这种情况下为内部函数)。 调用函数时,它将创建一个调用(或执行)堆栈,该堆栈只是专门分配的内存空间。 每个调用都使用一组局部变量创建自己的执行堆栈。 例如,在像C / C ++这样的传统语言中,调用内部函数将创建自己的内存堆栈(请参见下图)。 因此, 内部函数将无法访问其自身范围之外的任何变量 。 但是,在JavaScript中,每个执行的函数都会创建一个Closure 。 换句话说,每个函数都具有将函数封装在内部的功能,此封装使内部函数可以访问其中的所有变量 。 因此,闭包只是函数及其词法范围的组合。
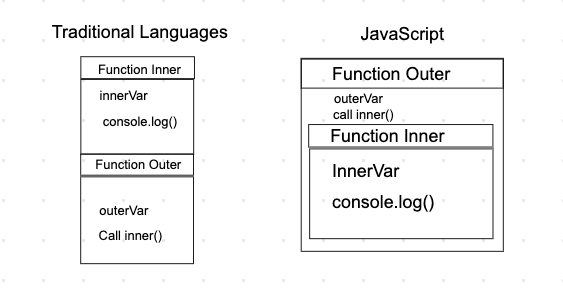
Now, let’s say we’d like to return the actual value inside the second function, as opposed to its definition. For that, we could add the following lines of code:
现在,假设我们想在第二个函数中返回实际值,而不是其定义。 为此,我们可以添加以下代码行:
let innerOutput = outer(); //Output:
/*
Here's what happens behind the scenes after the code above runs:
innerOutput = inner
*/console.log(innerOutput())
//Same as innerOutput = inner();
Output: The outer variable is outside
输出: The outer variable is outside
What makes closure so important is the fact that it keeps inner variables private. Nothing on the outside of the enclosed function can access its variables. This is important when using event handlers, partial applications, and call back functions. The goal is to keep things simple, therefore we can cover those concepts in another blog.
使闭包如此重要的原因是它使内部变量保持私有。 封闭函数外部的任何内容都无法访问其变量。 使用事件处理程序,部分应用程序和回调函数时,这一点很重要。 目的是使事情简单,因此我们可以在另一个博客中介绍这些概念。
For now, we can summarize the above as follows:
现在,我们可以总结如下:
- Closure is the fact of enclosing a function within another such that the enclosed function can access the variables outside of its own scope. 封闭是将一个函数封闭在另一个函数中的事实,这样封闭的函数可以访问其自身范围之外的变量。
- For every function that is created, JavaScript creates a closure by default. 对于创建的每个函数,JavaScript默认情况下都会创建一个闭包。
- Closures allow for privacy of the variables of the enclosed function 封闭允许封闭函数的变量的私密性
Thank you for reading! Happy coding! 😃
感谢您的阅读! 祝您编码愉快! 😃
翻译自: https://levelup.gitconnected.com/javascript-basics-series-closure-9caaf2a85487
javascript闭包