Kotlin was introduced to make Android development a whole lot easier and faster. After being named an official language for Android, it has slowly taken over Java and is the first choice for Android developers today.
引入Kotlin是为了使Android开发变得更加轻松和快捷。 在被命名为Android的官方语言之后,它已逐渐取代Java,并且是当今Android开发人员的首选。
For Java developers switching to Kotlin, the transition couldn’t have been easier, owing to their similarities. But at the same time, it’s really easy to ignore the uniqueness or rather idiomatic aspects of Kotlin. This can lead to writing code similar to Java.
对于转用Kotlin的Java开发人员来说,由于它们的相似性,过渡从未如此简单。 但是同时,很容易忽略Kotlin的独特性或惯用语言。 这可能导致编写类似于Java的代码。
Gladly, we can leverage some of the Kotlinic (a term inspired by Pythonic) ways of writing less verbose code in Android. Let’s get started.
很高兴地,我们可以利用Kotlinic的一些方式(受Pythonic启发)来在Android中编写较少冗长的代码。 让我们开始吧。
使用“ let”功能检查可空属性 (Use ‘let’ Function for Checking Nullable Properties)
It’s fairly common to use the “if not null” control structure for doing a null check on types before proceeding. Using Kotlin’s let
extension function helps avoid the complex branching logic.
在继续操作之前,通常使用“ if not null”控制结构对类型进行null检查。 使用Kotlin的let
扩展功能有助于避免复杂的分支逻辑。
Kotlin’s let
is a scoping function such that properties declared within it cannot be used outside. One can use this for nested let
s or for chaining a bunch of nullable. Here’s an example:
Kotlin的let
是作用域函数,因此在其中声明的属性不能在外部使用。 可以将其用于嵌套let
或链接一堆可为空的对象。 这是一个例子:
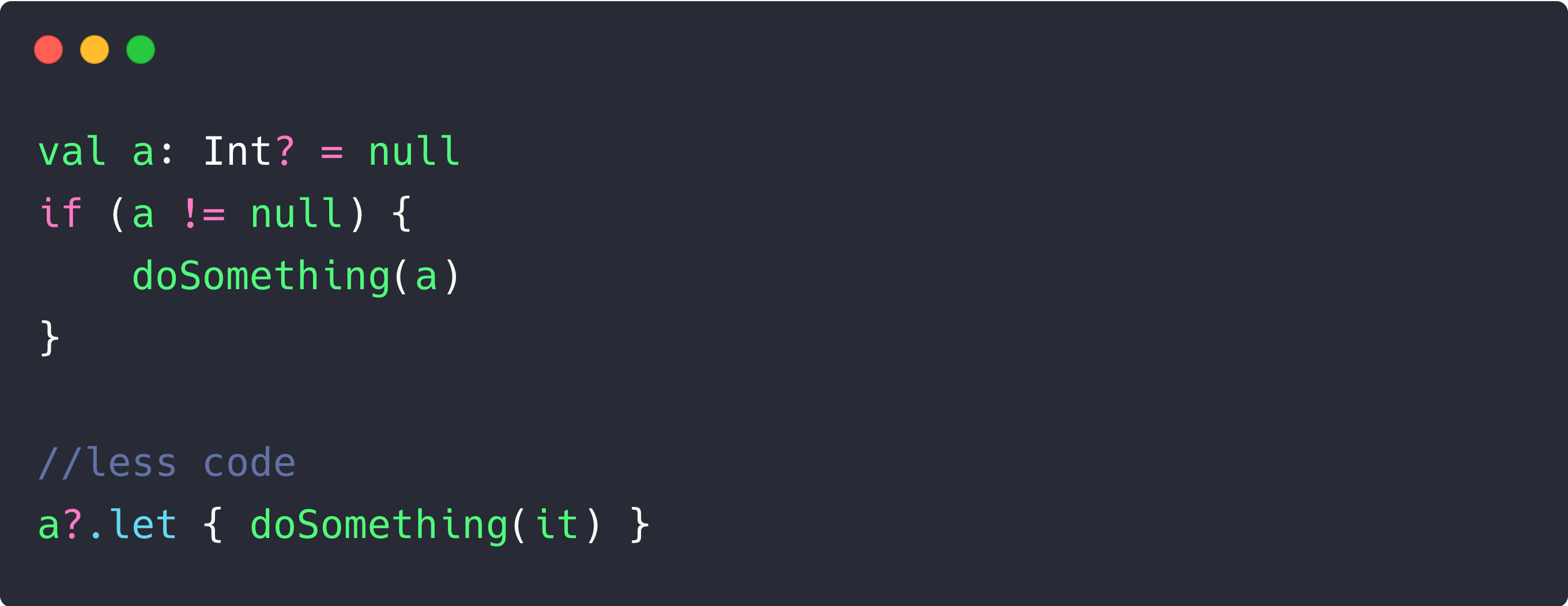
Using Kotlin’s Elvis operator(?:
) helps provide default values for nullable properties. For instance, in the above code, you can call the function as doSomething(a ?: 0)
— thereby ensuring a
has a default value.
使用Kotlin的Elvis运算符( ?:
:)有助于为可空属性提供默认值。 例如,在上面的代码中,您可以将函数调用为doSomething(a ?: 0)
-从而确保a
具有默认值。
用单行代码生成Kotlin列表 (Generate Kotlin Lists With a Single Line of Code)
If you’re looking to create a list with default values, it’s fairly straightforward. The below line of code creates an integer array of length 10 with each element initialized as 1.
如果您要创建具有默认值的列表,则非常简单。 下面的代码行创建一个长度为10的整数数组,每个元素都初始化为1。
IntArray(10) { 1 }.asList()
While this was quick and easy, one might wonder how to create a list with different elements. Of course, the classical way of doing this is with for
loops. But by leveraging the power of Kotlin, you can do it in a single line.
尽管这既快速又简单,但您可能会想知道如何创建具有不同元素的列表。 当然,经典的实现方式是使用for
循环。 但是通过利用Kotlin的功能,您可以在一行中完成它。

使用“需要”或“检查”功能处理提前退出条件 (Use ‘require’ or ‘check’ Functions for Early Exit Conditions)
The require
function validates the argument passed and throws an IllegalArgumentException
if it’s false
.
require
函数验证传递的参数,如果为false
,则抛出IllegalArgumentException
。
The check
function, on the other hand, throws IllegalStateException
when the object state is false.
另一方面,当对象状态为false时, check
函数将引发IllegalStateException
。
Both of these are handy when setting early exit conditions in your Kotlin codebases in Android.
在Android的Kotlin代码库中设置提前退出条件时,这两种方法都很方便。

使用“应用”和“使用”功能减少样板代码 (Use the ‘apply’ and ‘with’ Functions to Reduce Boilerplate Code)
Apply
and with
are two important scoped functions that help eliminate explicit references on an object when setting object properties. In a way both of the functions let you transform an object before returning it.
Apply
和with
是两个重要的作用域函数,可帮助您在设置对象属性时消除对对象的显式引用。 在某种程度上,这两个函数都可以让您在返回对象之前对其进行转换。
The apply
function is called on the object initialization, while the with
function requires passing the object as an argument.
在对象初始化时调用apply
函数,而with
函数则需要将对象作为参数传递。
By leveraging them, we can reduce some boilerplate code and make our codebases clear and concise.
通过利用它们,我们可以减少一些样板代码,并使我们的代码库清晰明了。
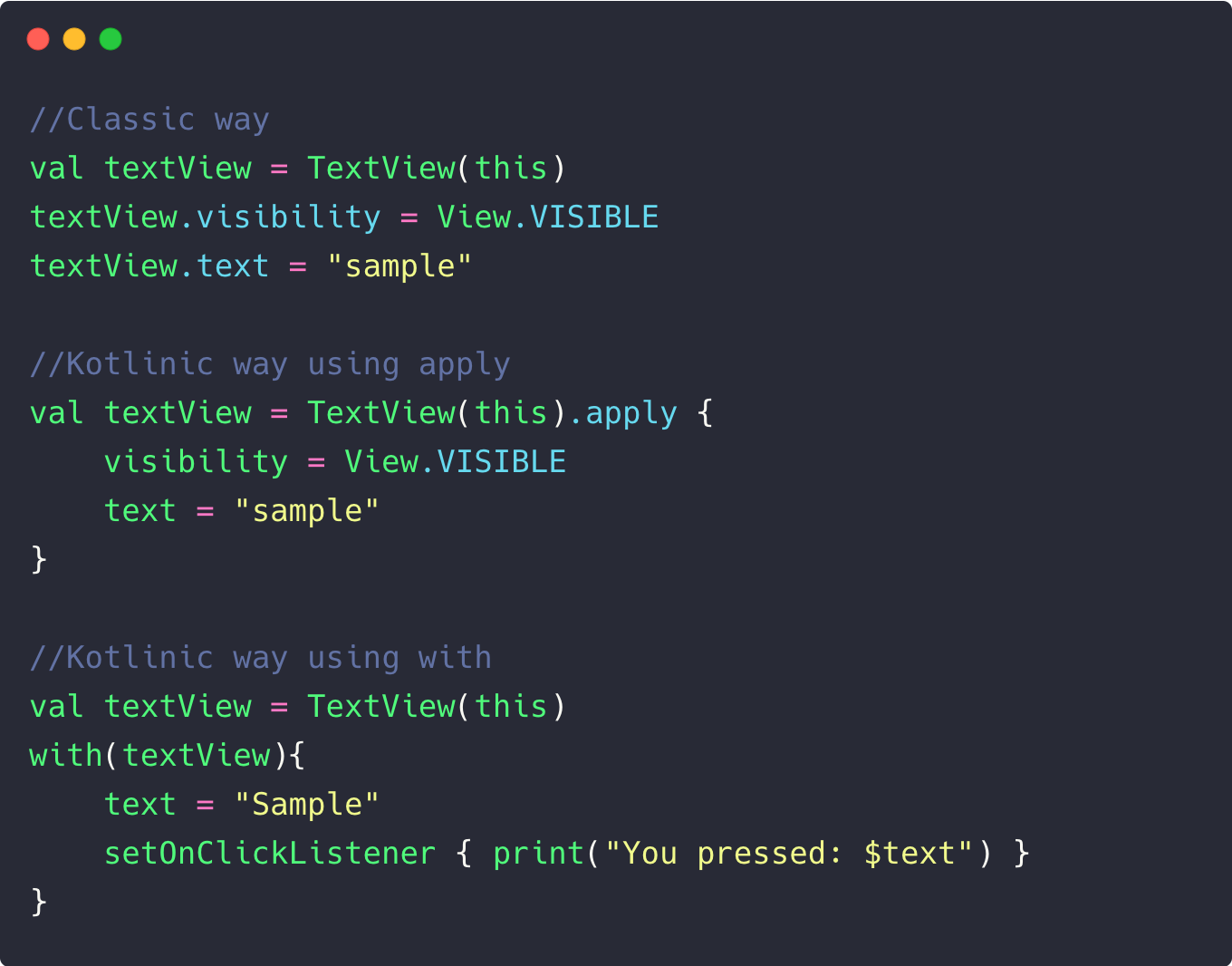
'partition'和'groupBy'运算符使您可以轻松拆分列表 (‘partition’ and ‘groupBy’ Operators Allow You to Split Lists Easily)
partition
lets you split a list into a pair of sublists where the first one contains elements that meet the condition specified and the remaining are put inside the second sublist.
partition
使您可以将列表拆分为一对子列表,其中第一个包含满足指定条件的元素,其余元素放在第二个子列表中。
groupBy
, on the other hand, returns a map of sublists in the form of key-value pairs. By invoking the values property on groupBy
, you get a list of lists. The following example demonstrates a use case of each.
另一方面, groupBy
以键值对的形式返回子列表的映射。 通过在groupBy
上调用values属性,您将获得一个列表列表。 以下示例演示了每种方法的用例。

用单行代码交换两个属性 (Swap Two Properties With a Single Line of Code)
Swapping two variables is among the first programming questions developers face. Traditionally, you’d define a temporary variable to interchange the properties. While there is a way to eliminate that and reduce the code to two lines, using a = a-b
and b = b-a
, we can make it even better.
交换两个变量是开发人员面临的第一个编程问题。 传统上,您将定义一个临时变量来交换属性。 虽然可以使用a = ab
和b = ba
消除这种情况并将代码减少到两行,但我们可以做得更好。
By using Kotlin’s idiomatic expression, swapping two properties can be done in a single line, as shown below:
通过使用Kotlin的惯用表达式,可以在一行中完成两个属性的交换, 如下所示:
a = b.also { b = a }
对于简单方案,首选方法引用优于Lambda表达式 (Prefer Method References Over Lambda Expressions for Simple Scenarios)
While lambda expressions are good in most use cases, sometimes when you just need to access a property, using method references over it
is a much better proposition.
虽然lambda表达式在大多数使用情况良好,有时当你只需要访问属性,使用方法引用了it
是一个更好的命题。
Member references are denoted with ::
. The class or object is written on the left and it’s invoked property on the right. Here’s an example scenario where using member references instead of a lambda expression makes the code much shorter and easier to read.
成员引用用::
表示。 类或对象写在左侧,它的调用属性在右侧。 这是一个示例场景,其中使用成员引用而不是lambda表达式使代码更短并且更易于阅读。

结论 (Conclusion)
I hope you found the above-mentioned Kotlin tricks useful. Thanks for reading.
希望您发现上述Kotlin技巧有用。 谢谢阅读。
翻译自: https://medium.com/better-programming/7-quick-kotlin-tips-for-android-developers-884d1021ab1d