junit android
时给定模式 (The Given-When-Then Pattern)
Do you know about the Given-When-Then (GWT) pattern given by uncle bob? if yes, then good, you already know something ahead then others, if no, let me give a simple explanation of what is GWT pattern by uncle bob.
您是否知道bob叔叔给出的“当下即送(GWT)”模式 ? 如果是,那么很好,您已经知道其他事情,如果不是,让我简单介绍一下bob叔叔的GWT模式。
The given part describes the state of the world before you begin the behavior you're specifying in this scenario. You can think of it as the pre-conditions to the test.
给定的部分描述了在这种情况下开始指定的行为之前的世界状态。 您可以将其视为测试的前提条件。
The when section is that behavior that you're specifying.
when部分是您指定的行为。
Finally, the then section describes the changes you expect due to the specified behavior.
最后, then部分描述由于指定的行为而导致的预期更改。
as an example, you could see something like this
例如,您可能会看到类似的内容
Feature: User trades stocks
Scenario: User requests a sell before close of trading
Given I have 100 shares of MSFT stock
And I have 150 shares of APPL stock
And the time is before close of trading
When I ask to sell 20 shares of MSFT stock
Then I should have 80 shares of MSFT stock
And I should have 150 shares of APPL stock
And a sell order for 20 shares of MSFT stock should have been executed
Okay, right now, you already know about the GWT pattern. I would say that it’s easy for us to follow this using Spek2 with their Gherkin Language that following cucumber gherkin style. but, Spek itself contains some bugs and needs a little bit of time to understand what happens inside it. That I could say, better to not using it for some time until these bugs are fixed. But is there any unit testing framework that we could use it on Android development which following the GWT pattern? if yes, it that buggy like Spek?
好的,现在,您已经了解了GWT模式。 我要说的是,我们很容易使用Spek2及其Cucumber语言来遵循Cucumber小Cucumber风格 。 但是,Spek本身包含一些错误 ,需要一点时间才能了解其中发生的情况。 我可以说,最好是在修复这些错误之前一段时间不使用它。 但是,是否有任何可以遵循GWT模式在Android开发中使用的单元测试框架? 如果是,那像Spek这样的越野车?
Here it comes, JUnit5 to the rescue. But wait, before jumping to JUnit5, do you know what is JUnit5?
到了这里, JUnit5得以营救。 但是,等等,在跳转到JUnit5之前,您知道什么是JUnit5吗?
JUnit5 (JUnit5)
JUnit5 is a combination of JUnit Platform, JUnit Jupiter, and JUnit Vintage, as said on their web JUnit5 = JUnit Platform + JUnit Jupiter + JUnit Vintage
. But wait, what is that all?
JUnit5是JUnit Platform,JUnit Jupiter和JUnit Vintage的组合,如在其Web上JUnit5 = JUnit Platform + JUnit Jupiter + JUnit Vintage
那样: JUnit5 = JUnit Platform + JUnit Jupiter + JUnit Vintage
。 但是等等,那是什么?
- JUnit Platform is libs that help you to run your JUnit test (aka JUnitPlatformRunner. JUnit Platform是可帮助您运行JUnit测试(即JUnitPlatformRunner。
- JUnit Jupiter is a lib that helps you to work on JUnit5 itself. JUnit Jupiter是一个库,可以帮助您处理JUnit5本身。
- JUnit Vintage is a lib that helps you to work on your legacy JUnit3 or JUnit4 test. JUnit Vintage是一个库,可以帮助您处理旧版JUnit3或JUnit4测试。
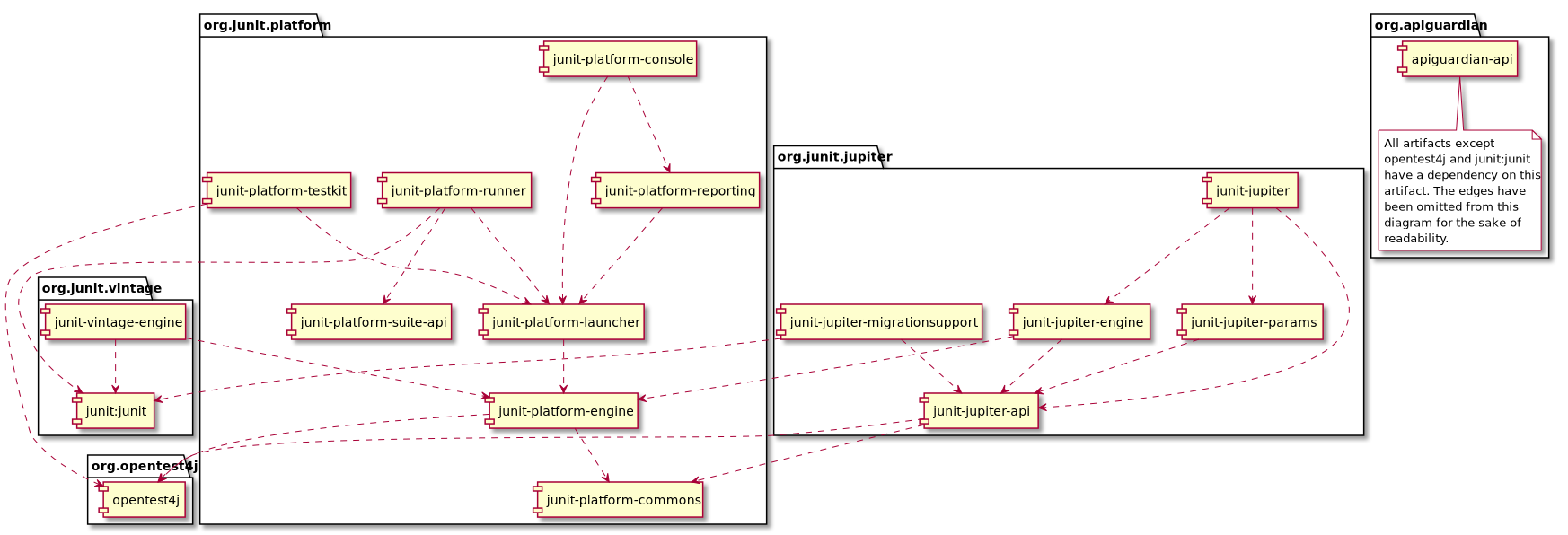
配置 (Setting up)
You can use JUnit5 with their own style, or following the GWT pattern given by uncle bob, to help you make your unit test readable and easier to understand. Not only by you but by most developers and non-developers on your team. But how? before showing the code, lemme show you how to configure JUnit5 on your project. You just need to add these 3 lines into your app/build.gradle
您可以按照自己的样式使用JUnit5,也可以按照bob叔叔给出的GWT模式使用JUnit5,以帮助您使单元测试易读易懂。 不仅由您自己,而且由团队中的大多数开发人员和非开发人员使用。 但是如何 ? 在显示代码之前,lemme向您展示了如何在项目上配置JUnit5 。 您只需要将这3行添加到您的app / build.gradle中
testImplementation "org.junit.platform:junit-platform-runner:1.6.2"
testImplementation "org.junit.jupiter:junit-jupiter-api:5.6.2"
testRuntimeOnly "org.junit.jupiter:junit-jupiter-engine:5.6.2"
创建测试 (Creating Test)
Then, how do I made the GWT pattern by uncle bob using JUnit5/Jupiter is shown by this code.
然后,此代码显示了我如何通过bob叔叔使用JUnit5 / Jupiter制作GWT模式。
package com.kamilmasyhur.gwtpattern.domain
import org.junit.jupiter.api.*
@DisplayName("Given Calculator")
internal class CalculatorTest {
val calculator = Calculator()
val initialValue = 0
val addedValue = 10
val expectedResult = 10
var result = 0
@DisplayName("When Adding 2 number")
@Nested
inner class Add {
@BeforeEach
fun whenCondition() {
result = calculator.add(initialValue, addedValue)
}
@Test
@DisplayName("Then should add two number")
fun thenCondition() {
Assertions.assertEquals(expectedResult, result)
}
}
}
But I said it will made your life, your college at work easier, how is it possible?
但是我说过,这将使您的生活,您的大学工作更加轻松,这怎么可能?

see, how it helps you to make your test became easier to read and to be understood by others. Now you can make your test became readable and easier to separate the concern, instead of using one-liner like this.
看,它如何帮助您使测试变得更易于阅读和理解。 现在,您可以使测试变得易读并且更容易区分关注点, 而不是像这样使用单一代码。
package com.kamilmasyhur.gwtpattern.domain
import org.junit.jupiter.api.Assertions
import org.junit.jupiter.api.DisplayName
import org.junit.jupiter.api.Test
internal class AnotherCalculatorTest {
val calculator = Calculator()
val initialValue = 0
val addedValue = 10
val expectedResult = 10
var result = 0
@Test
@DisplayName("Given Calculator, When Adding 2 number, Then should add two number")
fun thenCondition() {
result = calculator.add(initialValue, addedValue)
Assertions.assertEquals(expectedResult, result)
}
}

结论 (Conclusion)
From my side, I would suggest you using the GWT pattern, because it giving you a really loved time to understand what happened, easier to understand from its concern separation, and it will help other engineers to read it faster.
就我而言,我建议您使用GWT模式,因为它为您提供了一个非常钟爱的时间来了解发生了什么,从关注点分离中更容易理解它,并且它将帮助其他工程师更快地阅读它。
翻译自: https://proandroiddev.com/given-when-then-style-on-android-development-using-junit5-f47388053573
junit android