Data structures and algorithms are the foundations to programming. It has become a norm for every interviewer to test on these basics as they provide techniques for handling data efficiently. A programmer without an understanding of these techniques may build an inefficient solution or take a longer time to resolve the problem. This article introduces you to one of the commonly used linear data structures called Linked Lists.
数据结构和算法是编程的基础。 由于这些面试官提供了有效处理数据的技术,因此已成为每个面试官测试这些规范的规范。 不了解这些技术的程序员可能会构建效率低下的解决方案,或者花费更长的时间来解决问题。 本文向您介绍一种称为链接列表的常用线性数据结构。
线性数据结构 (Linear Data Structure)
A data structure is a container that stores data in a specific layout which allows for efficient access and modification. A data structure can either be linear or non-linear. While a linear data structure has elements arranged sequentially, a non-linear structure contains elements in a hierarchical order.
数据结构是一种以特定布局存储数据的容器,从而可以进行有效的访问和修改。 数据结构可以是线性的也可以是非线性的。 线性数据结构具有按顺序排列的元素,而非线性结构包含按分层顺序排列的元素。
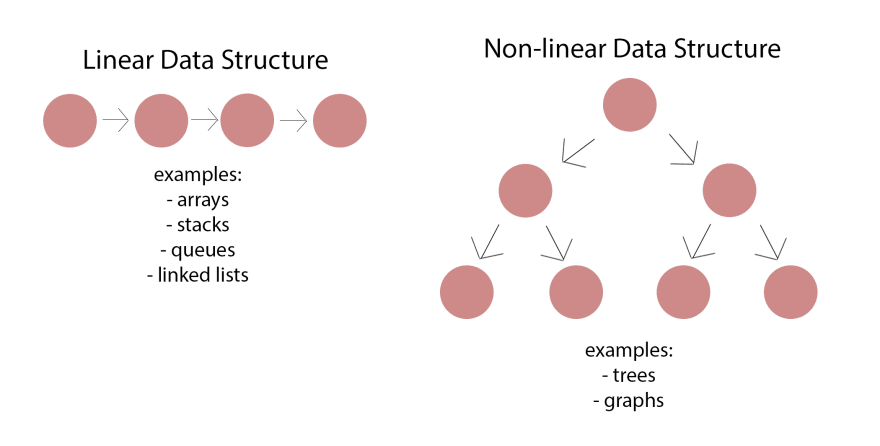
线性数据结构特征 (Linear Data Structure Characteristics)
Data Element Arrangement: Sequential
数据元素排列:顺序
Levels: All data elements are present at a single level
级别:所有数据元素都存在于单个级别
Traversal: Can be traversed completely in a single run
遍历:一次即可完全遍历
Implementation Complexity: Easier to implement
实施复杂性:易于实施
Memory Utilization: Inefficient
内存利用率:低效
Time Complexity: Increases with increase in size
时间复杂度:随着大小的增加而增加
链表(Linked List)
A linked list is a collection of elements called nodes arranged in a linear fashion. Each node contains a data field and a pointer to the succeeding node in the list called next. The first and last node of a linked list usually are called the head and tail of the list. The head pointer points to the first node and the last node will point to a null value. Note that the head is not a separate node but a reference to the first node. When the list is empty, the head pointer points to null.
链表是线性排列的称为节点的元素的集合。 每个节点在列表中都包含一个数据字段和一个指向下一个节点的指针,称为next 。 链接列表的第一个和最后一个节点通常称为列表的头和尾。 头指针指向第一个节点,最后一个节点指向空值。 请注意,头部不是单独的节点,而是对第一个节点的引用。 当列表为空时,头指针指向空。
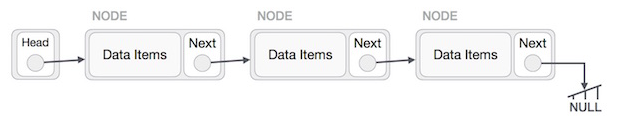
Linked lists are dynamic and flexible and can expand and contract in size. Memory for linked lists is allocated during execution or runtime.
链接列表是动态且灵活的,并且可以扩展和缩小大小。 链表的内存是在执行或运行时分配的。
链表的类型 (Types Of Linked List)
Singly Linked List: Being unidirectional in nature, the node traversal can be done in the forward direction only (see pic above).
单链表:本质上是单向的,节点遍历只能在正向进行(请参见上图)。
Doubly Linked List: Being bi-directional, the node traversal can be done in both forward and backward directions. Each node has an additional pointer called prev, pointing to the previous node.
双链表:双向,节点遍历既可以向前也可以向后。 每个节点都有一个称为prev的附加指针,指向上一个节点。
Circular Linked List: A variation of linked list in which the last element is linked to the first element forming a circular loop. It can take either of the above two forms. In a circular doubly linked list, the prev pointer of the head points to the tail and the next pointer of the tail points to the head.
循环链表:链表的一种变体,其中最后一个元素链接到第一个元素,形成一个循环循环。 它可以采用上述两种形式之一。 在圆形双向链表,头部指向尾部的分组指针和尾指向所述头部的下一个指针。
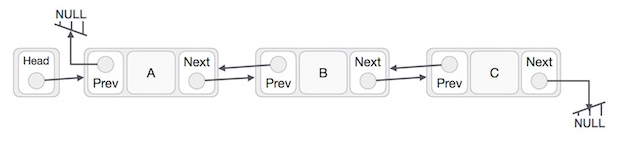


链表操作(Linked List Operations)
Searching: To find the first element with the given value in the linked list by a simple linear search and return a pointer to this element.
搜索:通过简单的线性搜索在链表中查找具有给定值的第一个元素,并返回指向该元素的指针。
Insertion: To insert an element ‘x’ into the linked list is a multi-step process. An insertion can be done in 3 different ways: insert at the beginning of the list, insert at the end of the list and insert in the middle of the list.
插入:将元素“ x”插入到链表中是一个多步骤过程。 可以通过3种不同的方式进行插入:在列表的开头插入,在列表的末尾插入以及在列表的中间插入。
Deletion: To remove an element ‘x’ from the linked list is a multi-step process. A deletion can be done in 3 different ways: delete from the beginning of the list, delete from the end of the list and delete from the middle of the list.
删除:从链接列表中删除元素“ x”是一个多步骤过程。 可以通过3种不同的方式进行删除:从列表的开头删除,从列表的末尾删除和从列表的中间删除。
Traversal: To traverse all the nodes one after another.
遍历:遍历所有节点。
Updating: To update a given node.
更新:更新给定的节点。
Sorting: To arrange nodes in a linked list in a specific order.
排序:以特定顺序排列链接列表中的节点。
Merging: To merge two linked lists into one.
合并:将两个链接列表合并为一个。
Node Insertion
节点插入
Shown below is an insert operation to the middle of a singly linked list:
下面显示的是对单链列表中间的插入操作:
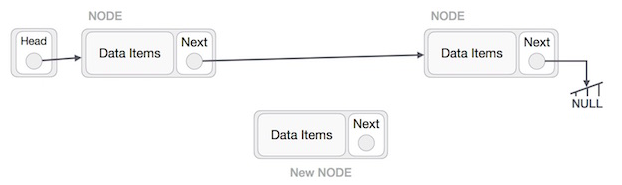
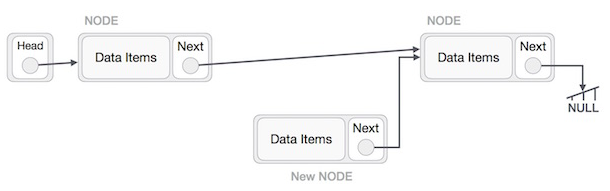
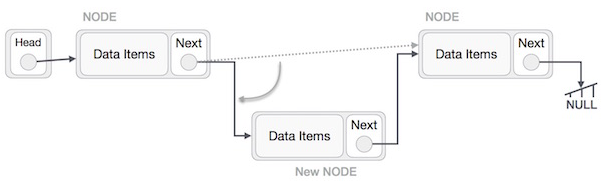

Node Deletion
节点删除
Shown below is a delete operation from the middle of a singly linked list:
下面显示的是从单链列表中间进行的删除操作:



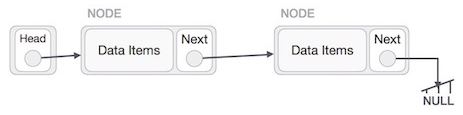
时间复杂度 (Time Complexity)
A linked list is accessed via its head node. One has to only traverse through each node to reach the target node. Thus access is O(n).
链表是通过其头节点访问的。 一个人仅需遍历每个节点即可到达目标节点。 因此访问为O(n) 。
Searching for a given value in a linked list similarly requires traversing all the elements until you find that value. Thus search is O(n).
在链接列表中搜索给定值类似地需要遍历所有元素,直到找到该值为止。 因此搜索为O(n) 。
Inserting into a linked list requires re-pointing the previous node to the inserted node, and pointing the newly-inserted node to the next node. Thus insertion is O(1).
插入到链表中需要将前一个节点重新指向插入的节点,并将新插入的节点指向下一个节点。 因此插入为O(1) 。
Deleting from a linked list requires re-pointing the previous node (the node before the deleted node) to the next node (the node after the deleted node). Thus deletion is O(1).
从链接列表中删除需要将上一个节点(已删除节点之前的节点)重新指向下一个节点(已删除节点之后的节点)。 因此删除是O(1) 。
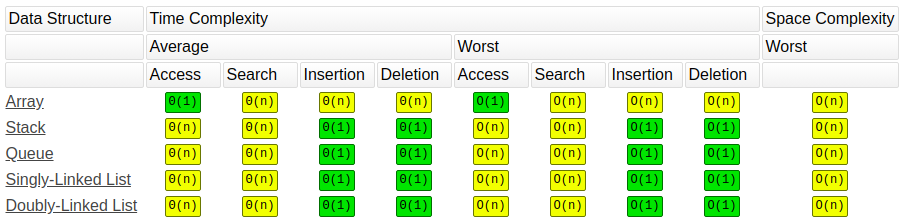
好处 (Advantages)
- Ease of insertion and deletion which takes O(1) time易于插入和删除,耗时O(1)
- Less memory wastage due to dynamic memory allocation 由于动态内存分配,减少了内存浪费
缺点(Disadvantages)
- Random access is not allowed不允许随机访问
- Requires extra memory space to store a reference to the next node需要额外的内存空间来存储对下一个节点的引用
应用领域(Applications)
- Implementation of stacks, queues and graphs and in areas that require dynamic memory allocation在需要动态内存分配的区域中实现堆栈,队列和图形
- Used for symbol table management in compiler design用于编译器设计中的符号表管理
- Used in switching between programs using Alt + Tab (implemented using Circular Linked List)用于在使用Alt + Tab的程序之间进行切换(使用循环链表实现)
- Manipulation of polynomials by storing constants in the node of linked list 通过将常数存储在链接列表的节点中来处理多项式
- Used in music player where the songs are linked to previous & next songs用于音乐播放器,其中的歌曲链接到上一首和下一首歌曲
- Used by browsers to implement backward and forward navigation of visited web pages i.e. back and forward button浏览器用于实现所访问网页的后退和前进导航,即后退和前进按钮
- Many modern operating systems use doubly linked lists to maintain reference to active processes, threads and other dynamic objects许多现代操作系统使用双向链接列表来维护对活动进程,线程和其他动态对象的引用
结语(Wrap Up)
While linked lists have their own share of pros and cons, they are still widely used today in most data structures and applications. Being a foundational topic, it is important for every developer to have an understanding of this linear data structure. I hope this article is helpful in meeting its objective. Feel free to share your views in the comments section below.
尽管链表各有优缺点,但今天它们仍在大多数数据结构和应用程序中得到广泛使用。 作为基础主题,对每个开发人员来说,了解这种线性数据结构都非常重要。 我希望本文有助于实现其目标。 欢迎在下面的评论部分中分享您的观点。
Common Data Structures every programmer should know
翻译自: https://medium.com/swlh/an-introduction-to-the-linked-lists-5e565e3c1b9c