ruby 两数最大值
Today, we’re gonna go to space. While also learning how to use a suite of array enumerations! Let’s learn to love the <=>.
今天,我们要去太空。 同时还学习如何使用一组数组枚举! 让我们来学习爱<=>。
An essential part of programming is knowing your comparison operators. These are things like == (checking equality), > (greater than), < (less than), etc. They’re staples of mathematics & boolean logic, and comprise a whole lot of our code.
编程的重要部分是了解您的比较运算符。 这些是==(检查相等性),>(大于),<(小于)等之类的东西。它们是数学和布尔逻辑的基础,并且构成了我们很多代码。
However, you might not know all of the comparison operators out there. I wouldn’t blame you — there are a LOT of these bad boys out there across many languages. One that you may have seen & scratched your head at is the “three way comparison”.
但是,您可能不知道所有比较运算符。 我不会怪你-许多不同语言的坏男孩在那里。 您可能已经看到并摸不着头脑的一个就是“三向比较”。
<=>
Whoa. What the heck is that thing?
哇 那东西到底是什么?
We affectionately refer to this bad boy as the “spaceship” operator, & its usage is way less intimidating than it may look at first glance! Since it is a comparison operator, it is used to compare two pieces of data. However, instead of a simple true/false return, it has three states of return, based on the three characters it contains.
我们亲切地把这个坏男孩称为“太空飞船”操作员,它的用法比乍看之下少吓人! 由于它是一个比较运算符,因此可用于比较两个数据。 但是,它不是简单的true / false返回,而是基于它包含的三个字符而具有三种返回状态。
Let’s take an example: a <=> b.
让我们举个例子:a <=> b。
- If a is less than b (a < b), the comparison will return -1. 如果a小于b(a <b),则比较将返回-1。
- If a is equal to b (a == b), the comparison will return 0. 如果a等于b(a == b),则比较将返回0。
- If a is greater than b (a > b), the comparison will return 1. 如果a大于b(a> b),则比较将返回1。
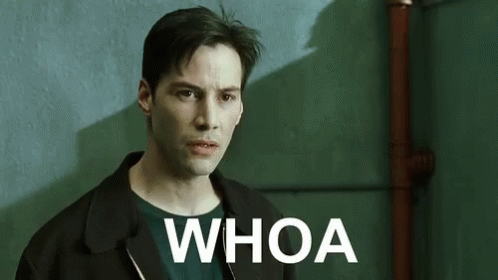
Ok Conrad. That’s neato. But how do we use that? I can’t use that in my if statements, since those require true/false. Well, you’d be partially right! Technically, you can use them in your if statements, it would just require an extra step (ex. if (a <=> b) == 0
is the same as if a == b
, but with extra steps.) But the real meat & potatoes of the spaceship is with some enumerators that Ruby provides for us! We’re gonna cover 3 things here: .max, . max_by vs .max, & other enumerables that use the spaceship!
好的,康拉德。 那是整洁的。 但是,我们该如何使用呢? 我不能在我的if语句中使用它,因为它们需要true / false。 好吧,你会部分正确! 从技术上讲,您可以在if语句中使用它们,这只需要一个额外的步骤(例如if (a <=> b) == 0
与if a == b
相同,但有额外的步骤。)但是Ruby提供给我们的一些枚举器是飞船的真正肉和土豆! 我们将在这里介绍3件事:.max, max_by与.max,以及其他使用飞船的枚举!
.max (.max)
Let’s say you need to find the highest integer in an array of data. There are a number of ways we can go about this, but let’s use .max! A statement using .max may look something like this:
假设您需要在数据数组中找到最高的整数。 我们可以通过多种方法来解决这个问题,但是让我们使用.max! 使用.max的语句可能看起来像这样:
array = [0, 4, 18, 6]array.max do |a, b|
a <=> b
end=> 18
Okay, cool. We’ve successfully used our first spaceship! Give yourself a pat on the back! But… huh? What’s a & b in this example? And how is this actually working? I’m glad you asked, friend! An effective way to visualize .max is as a fighting ring. Put up your dukes.
好,好酷 我们已经成功使用了第一艘太空飞船! 拍拍自己的背部! 但是... 在此示例中,a&b是什么? 实际情况如何? 我很高兴你问,朋友! 可视化.max的有效方法是作为战斗环。 举起你的公爵。
Let’s think of our array of numbers as a series of challengers (I have money on 6 being our champion. He’s such a good underdog story.) & rewrite our code to fit that analogy.
让我们将一系列数字视为一系列挑战者(我有6个钱是我们的冠军。他是一个很好的失败者。)并重写我们的代码以适合这个类比。
array.max do |challenger, champion|
challenger <=> champion
end
Challenger vs. champion, only one person leaves the ring. By default, the 0th element of our array will start as champion. Then, max will iterate through the array, pitting each challenger (the next index) against the reigning champion. At the end of each fight, whoever wins (is the bigger number) will become our new champion, and move onto the next round! This will continue until we’ve iterated through the entire array, and eventually found our grand champion (ie, the highest value in the array.)
挑战者与冠军的争夺战,只有一个人离开。 默认情况下,数组的第0个元素将从冠军开始。 然后,max将遍历整个数组,使每个挑战者(下一个索引)与卫冕冠军一较高下。 在每场比赛的最后,无论谁获胜(人数更多),都将成为我们的新冠军,并进入下一轮! 这将一直持续到我们遍历整个数组,最终找到我们的大冠军(即数组中的最高值)。
If we were to visualize it:
如果我们将其可视化:
- Champion (array[0]) fights challenger (array[1]) 冠军(array [0])与挑战者(array [1])战斗
- Champion of last match fights challenger (array[2]) 最后一场比赛的冠军与挑战者作战(array [2])
- Champion of last match fights challenger (array[3]) 最后一场比赛的冠军与挑战者搏斗(array [3])
- etc. until we've reached the end of the array. 等等,直到到达数组末尾。
That makes a whole lot of sense, yeah? Now that we’ve learned the nitty-gritty of how our spaceship works inside of max, let’s simplify things
是很有意义的,是吗? 既然我们已经了解了宇宙飞船如何在max内工作的本质,那么让我们简化一下事情
- A tie between fighters will always go to the champion. (if we’re getting technical, it’ll go to whoever is on the right side of the spaceship.) 战士之间的平局永远归冠军。 (如果我们要掌握技术,它将交给位于飞船右侧的任何人。)
- You don’t need to write the whole block if you’re comparing an array of integers. For example, [0..2].max (that’s the same as [0,1,2].max) will return 2, without any of the spaceship-y fanfare that we wrote before. 如果要比较整数数组,则无需编写整个块。 例如,[0..2] .max(与[0,1,2] .max相同)将返回2,而没有我们之前编写的任何关于y的幻想。
- If you use .max to compare an array of strings, make sure to specify that you’re comparing length. Otherwise, you’ll be comparing the first character of each string, which goes into some weird Unicode nonsense we don’t have time for today. 如果使用.max比较字符串数组,请确保指定要比较的长度。 否则,您将比较每个字符串的第一个字符,这变成了我们今天没有时间的一些奇怪的Unicode废话。
- If you try to compare an array of different data types, you’ll throw an error. Don’t try to compare different types, silly. 如果尝试比较不同数据类型的数组,则会引发错误。 不要愚蠢地比较不同的类型。
- If you add an argument to .max, it’ll return the top x values. For example, [0, 14, 8, 7].max(2) will return [14, 8]. 如果将参数添加到.max,它将返回前x个值。 例如,[0,14,8,7] .max(2)将返回[14,8]。
.max_by与.max (.max_by vs .max)
The .max_by enumerable is a “simplified” version of .max. We’re still going to be iterating through an array and returning the highest element, but no spaceship is gonna be used in our block. Very sad.
.max_by枚举是.max的“简化”版本。 我们仍然要遍历数组并返回最高的元素,但是在我们的块中将不使用任何太空飞船。 很伤心
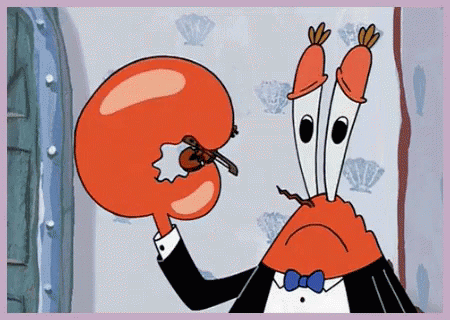
Let’s say that we want to find the string in an array of strings with the highest character count. We’d write it like this.
假设我们要在字符数最多的字符串数组中找到该字符串。 我们会这样写。
name_array = [“bob”, “conrad”, “rick”]name_array.max_by do |name|
name.length
end
Basically, max_by will find the highest value based on the criteria inside the block (in this case, the length of each name). Writing this as a .max looks very similar:
基本上,max_by将根据块内的条件(在这种情况下,每个名称的长度)找到最大值。 将其写为.max看起来非常相似:
name_array.max do |name_challenger, name_champion|
name_challenger.length <=> name_champion.length
end
The first thing I thought when I learned about .max_by was, “okay, so why would I ever use .max instead? .max_by is a lot less writing for the same result.” And this was a fantastic question. From my understanding, you should use .max when the variables you’re comparing are different based on whether you’re the champion or challenger. For example, sticking with our fighting ring example:
当我了解.max_by时,我想到的第一件事是:“好吧,那为什么我要改用.max? .max_by要写出相同的结果要少得多。” 这是一个奇妙的问题。 据我了解, 当您比较的变量基于冠军还是挑战者而不同时 , 应使用.max。 例如,坚持我们的战斗环示例:
people_array.max do |challenger, champion|
challenger.max_hp <=> champion.current_hp
end
In this example, we’re pitting our challenger’s maximum HP against our champion’s current HP — two separate values. This is pretty appropriate to our metaphor: our champion isn’t gonna be in top shape if he doesn’t get a break and keeps fighting! (let’s ignore the fact that his HP isn’t actually going down after each loop in our code… keep our metaphor simple!)
在此示例中,我们将挑战者的最大HP与我们冠军的当前HP (两个单独的值)进行比较。 这很适合我们的隐喻:如果我们的冠军没有休息并继续战斗,他就不会处于最佳状态! (让我们忽略一个事实,即在我们的代码中的每个循环之后,他的HP实际上并没有下降……简化我们的隐喻!)
If I just wanted to compare maximum HP, then yes, a .max_by loop would be the right call.
如果我只是想比较最大HP,那么,.max_by循环将是正确的选择。
类似的枚举和示例 (Similar enumerables & examples)
Here’s a list of enumerables that work almost identically to .max & .max_by.
这是与.max和.max_by几乎相同的可枚举列表。
- .min/.min_by: Returns the minimum value. .min / .min_by:返回最小值。
- .minmax/.minmax_by: Returns both the minimum & maximum values as an array. Min is element 0 of the returned array, max is element 1. You cannot specify how many mins/maxes to output like you could with .max/.min. .minmax / .minmax_by:将最大值和最小值作为数组返回。 Min是返回数组的元素0,max是元素1。您无法像.max / .min一样指定要输出多少个mins / maxes。
- .sort/.sort_by: Returns the array in order from min to max. Keep in mind that, according to the Ruby Documentation, “When the comparison of two elements [is equal], the order of the elements is unpredictable.” .sort / .sort_by:按从最小到最大的顺序返回数组。 请记住,根据Ruby文档,“当两个元素的比较[相等]时,元素的顺序是不可预测的。”
Let’s create some examples, just to solidify everything in your head!
让我们创建一些示例,只是为了巩固您的头脑!
numbers = [9, 832, 5, 11, 11, 2]
names = ["bob", "conrad", "melinda", "me"]numbers.max(2) => [832, 11]
numbers.min => 2
numbers.minmax => [2, 832]
numbers.sort => [2, 5, 9, 11, 11, 832]names.max_by {|name| name.length} => "melinda"
names.min(3) {|challenger, champion| challenger.length <=> champion.length} => ["me", "bob", "conrad"]
Congrats! We’ve learned about the spaceship & its enumerators! I’ll see you guys on Jupiter!
恭喜! 我们已经了解了飞船及其枚举器! 我会在木星上见到你们!
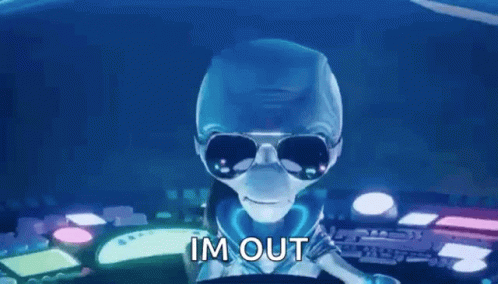
翻译自: https://medium.com/@conicsonic5/ruby-max-max-by-the-spaceship-795265db3ece
ruby 两数最大值