messagequeue
Message Queues play an important role in large-scale solutions, as the project becomes more and more sophisticated — micro-services are added and we become dependant of the message-queue functionality.
随着项目变得越来越复杂,消息队列在大规模解决方案中扮演着重要的角色-添加了微服务,我们变得依赖于消息队列功能。
One day we wake up and discover that current message-queue implementation doesn't meet our changing needs, we change it — and we fall into the endless refactor and regression tests loop.
有一天,我们醒来,发现当前的消息队列实现无法满足我们不断变化的需求,我们对其进行了更改—陷入了无休止的重构和回归测试循环。
So can we devise a framework that allows us to change the implementation without affecting the whole project?
那么我们是否可以设计一个框架,使我们能够在不影响整个项目的情况下更改实现?
In this post, I would share with you a framework design that enables just that, as I’ve developed in the final project of Dan Gittik’s great course “Advanced System Design” at Tel-Aviv University.
在这篇文章中,我将与您分享一个框架设计,该框架设计将使这一切成为可能,正如我在特拉维夫大学Dan Gittik的出色课程“高级系统设计”的最后一个项目中开发的那样。
This post would be the first in a series in which I share with you some flexible yet SOLID and durable solutions to design challenges.
这篇文章将是本系列的第一篇文章,在该系列文章中,我将与您分享一些灵活但 可靠的SOLID 和持久的解决方案来应对设计挑战。
从实现中分离消息队列 (Detaching Message-Queue from its Implementation)
The most important goal of the framework, is to enable us to change message-queue implementation (i.e. RabbitMQ to Kafka), with ease.
该框架最重要的目标是使我们能够轻松更改消息队列实现(即RabbitMQ到Kafka)。
We want that such change would be friendly and require a minimal code change and that validating the architectural specifications would be understandable for non-coders too.
我们希望这样的更改是友好的,并且需要最少的代码更改,并且对于非编码人员而言,验证体系结构规范也可以理解。
So, we end up creating a configuration file — just plug & play!
因此,我们最终创建了一个配置文件-即插即用!
Such a configuration file would describe how to create for each micro-service it’s implementation message-queue:
这样的配置文件将描述如何为每个微服务创建其实现消息队列:
- Publishers (which enable us to send messages to the message-queue) 发布者(使我们能够将消息发送到消息队列)
- Consumers (which enable us to receive messages from the message queue) 使用者(使我们能够从消息队列接收消息)
The configuration file structure would be as the following:
配置文件的结构如下:
# Message Queue configuration version tag.
version: "1"
# Default Header
message_queue:
# Type of micro-service (server, parser, saver, etc...)
micro_service_type:
# Category : Publishers
publishers:
# Item
- publisher_1:
# Fields required to initialize implementation (Context fields values)
[field_1] : [value_1]
[field_2] : [value_2]
[field_3] : [value_3]
# Category : Consumers
consumers:
# Item
- consumer_1:
# Fields required to initialize implementation (Context fields values)
[field_1] : [value_1]
[field_2] : [value_2]
[field_3] : [value_3]
Publishers and Consumers describe the fields required to create their specific implementation (i.e. Specific RabbitMQ publisher/consumer exchange), those fields recipes are called “Context”.
发布者和消费者描述创建其特定实现所需的字段(即,特定RabbitMQ发布者/消费者交换),这些字段配方称为“上下文”。
NOTE: This is an example of a YAML configuration file, yet it could be any dictionary-structured file — as I’ve implemented in the project.
注意:这是一个YAML配置文件的示例,但是它可以是任何字典结构的文件-正如我 在项目中实现的那样 。
NOTE: An example of a RabbitMQ MessageQueue configuration file available here.
注意: RabbitMQ MessageQueue配置文件 的示例 可在此处获得 。
利用MessageQueue上下文与框架 (Harnessing MessageQueue Context to the Framework)
So we’ve created a configuration file, we now can — based on micro-service type- load it’s publishers and consumers, using the fields which we call “Context”.
因此,我们已经创建了一个配置文件,现在我们可以基于微服务类型,使用我们称为“上下文”的字段将其分发给发布者和消费者。
We would implement a factory that loads the configuration file; receives a micro-service type, and creates its relevant context objects.
我们将实现一个工厂来加载配置文件。 接收微服务类型,并创建其相关的上下文对象。
Such a context object would contain all the relevant fields for the implementation, and mandatorily holds information regarding its role (either publisher or consumer).
这样的上下文对象将包含实现的所有相关字段,并强制保存有关其角色(发布者或消费者)的信息。
From a framework user perspective, we would like to be able to run micro-service publishers and consumers based on the loaded context objects.
从框架用户的角度来看,我们希望能够基于加载的上下文对象来运行微服务发布者和使用者。
So let’s present how it all comes together:
因此,让我们介绍一下它们是如何结合在一起的:
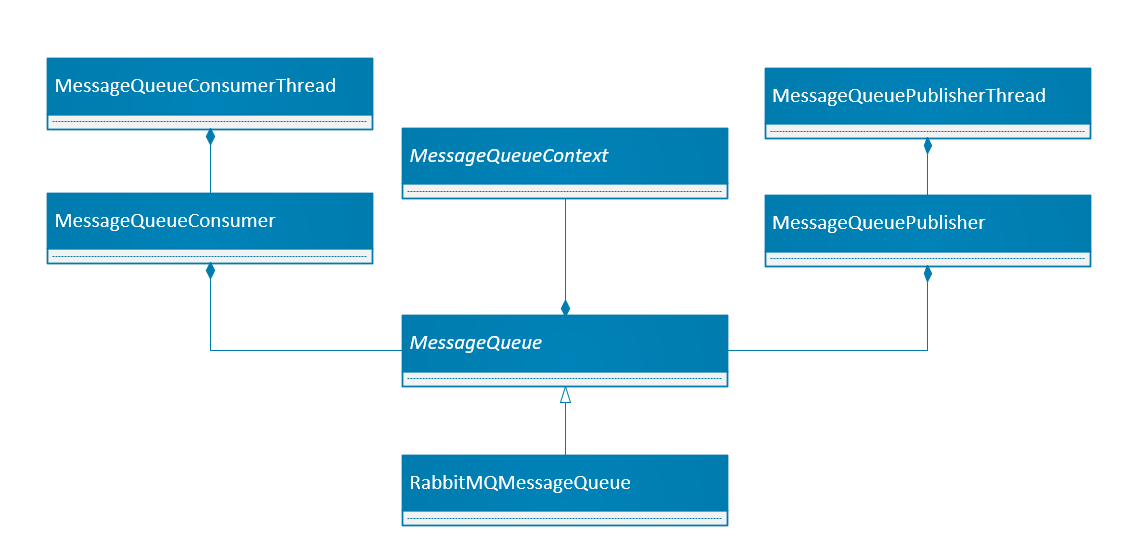
This how the flow works:
该流程的工作方式:
- Using the MessageQueue factory, we create a Context object. 使用MessageQueue工厂,我们创建一个Context对象。
- Context used to initialize either a MessageQueuePublisher or MessageQueueConsumer. 用于初始化MessageQueuePublisher或MessageQueueConsumer的上下文。
- MessageQueuePublisher and MessageQueueConsumer, call a module that loads the concrete implementation (i.e. RabbitMQMessageQueue) based on the selected MessageQueue type (can be configured as environment variable). This implementation takes the Context object and initializes as Publisher or Consumer. MessageQueuePublisher和MessageQueueConsumer,调用一个模块,该模块根据所选的MessageQueue类型(可以配置为环境变量)来加载具体的实现(即RabbitMQMessageQueue)。 此实现采用Context对象,并初始化为Publisher或Consumer。
So when we want to change a MessageQueue implementation type, we just:
因此,当我们想要更改MessageQueue实现类型时,我们只需:
- Create a configuration file 创建一个配置文件
- Create a class that inherits from MessageQueue abstract class, and implement the methods for initialization from Context, publishing, and consuming (i.e. RabbitMQ). 创建一个从MessageQueue抽象类继承的类,并实现用于从Context初始化,发布和使用的方法(即RabbitMQ)。
NOTE! You can view an example for initialization at Parser micro-service.
注意! 您可以在 Parser micro-service上 查看初始化示例 。
…Till next post!
…直到下一篇文章!
翻译自: https://medium.com/swlh/generic-messagequeue-framework-29a4780f202c
messagequeue