java 线程学习
One of the main reasons to use threads in Java is to make a task run parallel to another task.
在Java中使用线程的主要原因之一是使一个任务与另一个任务并行运行。
为什么我们在Java中使用线程? (Why we use Threads in Java?)
We use Threads to make Java applications faster by doing multiple things at the same time. In technical terms, Thread helps us to achieve parallelism in Java programs. Since CPU is very fast and nowadays it even contains multiple cores, just one thread is not able to take advantage of all the cores, which means our costly hardware will remain idle for most of the time. By using multiple threads, we can take full advantage of multiple cores by serving more clients and serving them faster. Since, in today’s fast-paced world, response time matters a lot and that’s why we have multi-core CPUs, multi-threading is one way to exploiting the huge computing power of CPU in Java application.
我们使用线程通过同时执行多项操作来使Java应用程序更快。 用技术术语来说,线程可以帮助我们在Java程序中实现并行性。 由于CPU速度非常快,如今它甚至包含多个内核,因此只有一个线程无法利用所有内核,这意味着我们昂贵的硬件将在大多数时间内保持空闲状态。 通过使用多个线程,我们可以通过为更多客户端提供服务并更快地为其服务,从而充分利用多个内核。 由于在当今快节奏的世界中,响应时间非常重要,这就是我们拥有多核CPU的原因,因此多线程是在Java应用程序中利用CPU巨大计算能力的一种方法。
在Java中使用多线程的原因 (Reasons for using Multithreading in Java)
Even Java application contains at least one thread, which is called the main thread which executes your main method. There are more threads used by JVM e.g. daemon threads which do garbage collections and some other housekeeping works. As an application developer, we can also add new user threads to make our application faster and more efficient. Here are a couple of common reasons and scenarios to use multiple threads in Java.
甚至Java应用程序也包含至少一个线程,该线程称为执行您的main方法的主线程。 JVM使用了更多线程,例如执行垃圾收集和其他一些内部管理工作的守护程序线程 。 作为应用程序开发人员,我们还可以添加新的用户线程,以使我们的应用程序更快,更高效。 这是在Java中使用多个线程的两个常见原因和方案。
1)并行编程 (1) Parallel Programming)
One of the main reasons to use threads in Java is to make a task run parallel to another task e.g. drawing and event handling. GUI applications e.g. Swing and Java FX GUIs are the best examples of multi-threading in Java. In a typical GUI application, the user initiates an action e.g. downloading a file from the network or loading games modules from hard disk.
在Java中使用线程的主要原因之一是使一个任务与另一个任务并行运行,例如绘图和事件处理。 GUI应用程序(例如Swing和Java FX GUI)是Java中多线程的最佳示例。 在典型的GUI应用程序中,用户启动一个操作,例如从网络下载文件或从硬盘加载游戏模块。
These actions require some time to complete but we cannot freeze the GUI because then the user will think our application is hung. Instead, we need a separate thread to carry out the time-consuming task and keep showing relevant messages to the user or allow him to do other tasks at the same time to keep your GUI alive. This is achieved by using multiple threads in Java.
这些操作需要一些时间才能完成,但是我们无法冻结GUI,因为这样用户会认为我们的应用程序已挂起。 相反,我们需要一个单独的线程来执行耗时的任务,并继续向用户显示相关消息,或者允许他同时执行其他任务以使GUI保持活动状态。 这是通过在Java中使用多个线程来实现的。
2)充分利用CPU能力。 (2) To take full advantage of CPU power.)
Another common reason for using multiple threads in Java is to improve the throughput of the application by utilizing full CPU power. For example, if we have got 32 core CPU and we are only using 1 of them for serving 1000 clients and assuming that our application is CPU bound, we can improve throughput to 32 times by using 32 threads, which will utilize all 32 cores of your CPU.
在Java中使用多个线程的另一个常见原因是通过利用完整的CPU能力来提高应用程序的吞吐量 。 例如,如果我们有32个核心CPU,而我们仅使用其中1个来为1000个客户端提供服务,并且假设我们的应用程序受CPU限制,那么我们可以通过使用32个线程来利用32个线程,从而将吞吐量提高到32倍。您的CPU。
3)减少响应时间 (3) For reducing response time)
We can also use multiple threads to reduce response time by doing fast computation by dividing a big problem into smaller chunks and processing them by using multiple threads. For example, the map-reduce pattern is based upon dividing a big problem into smaller ones and processing them.
我们还可以使用多个线程来减少响应时间,方法是将一个大问题分成多个较小的块并使用多个线程进行处理 ,从而进行快速计算。 例如,map-reduce模式是基于将大问题分为较小的问题并进行处理。
4)同时切断多个客户端。 (4) To sever multiple clients at the same time.)
One of the most common scenarios where using multiple threads significantly improve an application’s performance is a client-server application. A single-threaded application means only one client can connect to the server at a time, but a multi-threaded server means multiple clients can connect to the server at the same time. This means the next client doesn’t have to wait until our application finish processing the request of the previous client.
客户端-服务器应用程序是使用多个线程显着提高应用程序性能的最常见方案之一。 单线程应用程序意味着一次只能有一个客户端可以连接到服务器,而多线程服务器意味着多个客户端可以同时连接到服务器 。 这意味着下一个客户端不必等到我们的应用程序完成对上一个客户端的请求的处理。
线程的生命周期 (Life Cycle of a Thread)
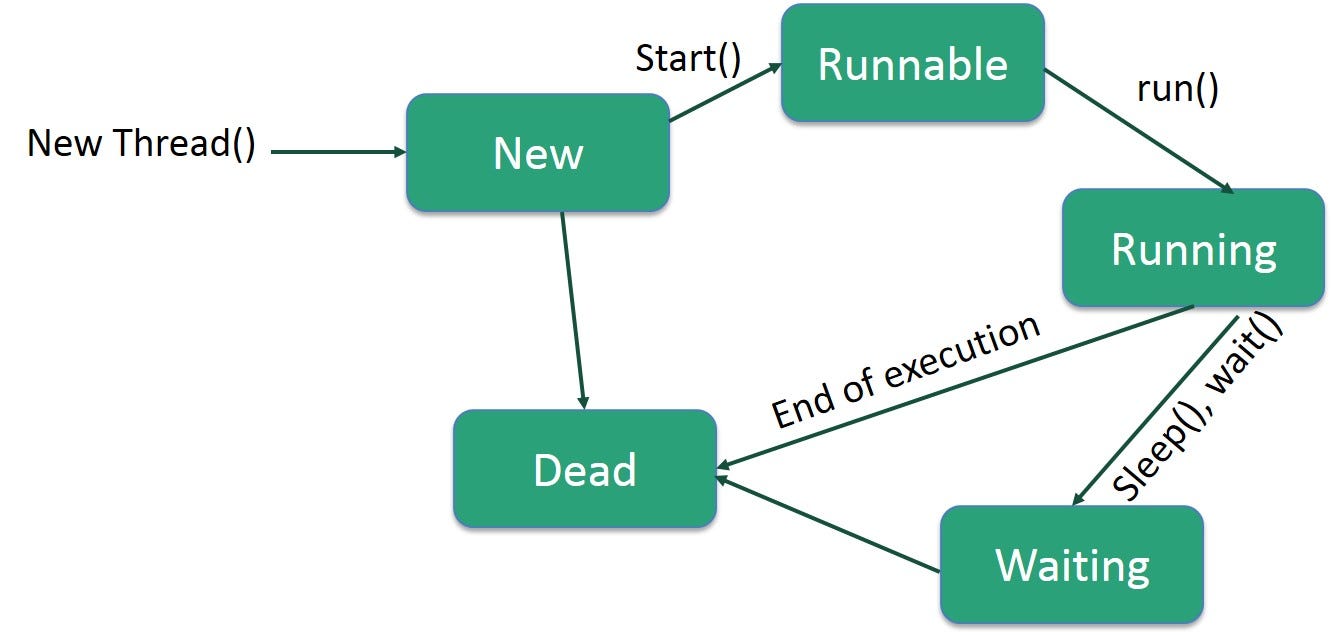
如何在Java中创建线程 (How to create Threads in Java)
There are two ways to create a thread:
有两种创建线程的方法:
By extending Thread class.
通过扩展Thread类。
By implementing the Runnable interface.
通过实现Runnable接口 。
线程类 (Thread class)
Thread class provides constructors and methods to create and perform operations on a thread. Thread class extends Object class and implements the Runnable interface.
Thread类提供了构造函数和方法来在线程上创建和执行操作。 线程类扩展了Object类并实现了Runnable接口。
线程类的常用构造函数 (Commonly used Constructors of Thread class)
- Thread() 线()
- Thread(String name) 线程(字符串名称)
- Thread(Runnable r) 线程(可运行r)
- Thread(Runnable r, String name) 线程(Runnable r,字符串名称)
Thread类的常用方法 (Commonly used methods of Thread class)
public void run(): is used to perform action for a thread.public void start(): starts the execution of the thread. JVM calls the run() method on the thread.public void sleep(long milliseconds): causes the currently executing thread to sleep (temporarily cease execution) for the specified number of milliseconds.public void join(): waits for a thread to die.public void join(long milliseconds): waits for a thread to die for the specified milliseconds.public int getPriority(): returns the priority of the thread.public int setPriority(int priority): changes the priority of the thread.public String getName(): returns the name of the thread.public void setName(String name): changes the name of the thread.public Thread currentThread(): returns the reference of currently executing thread.public int getId(): returns the id of the thread.public Thread.State getState(): returns the state of the thread.public boolean isAlive(): tests if the thread is alive.public void yield(): causes the currently executing thread object to temporarily pause and allow other threads to execute.public boolean isDaemon(): tests if the thread is a daemon thread.public void setDaemon(boolean b): marks the thread as daemon or user thread.public void interrupt(): interrupts the thread.public boolean isInterrupted(): tests if the thread has been interrupted.public static boolean interrupted(): tests if the current thread has been interrupted.
可运行的界面 (Runnable interface)
The Runnable interface should be implemented by any class whose instances are intended to be executed by a thread. The runnable interface has only one method named run().
Runnable接口应该由实例旨在由线程执行的任何类实现。 可运行接口只有一个名为run()的方法。
public void run(): is used to perform action for a thread.
启动线程 (Starting a thread)
start() method of Thread class is used to start a newly created thread. It performs the following tasks.
Thread类的start()方法用于启动新创建的线程。 它执行以下任务。
- A new thread starts(with a new call stack). 一个新的线程开始(带有一个新的调用栈)。
- The thread moves from New state to the Runnable state. 线程从“新建”状态移动到“可运行”状态。
- When the thread gets a chance to execute, its target run() method will run. 当线程有机会执行时,其目标run()方法将运行。
Let's go through a simple example to see how the threads are working.
让我们看一个简单的例子,看看线程是如何工作的。
public class ThreadDemo {
public static void main(String[] args) {
//tight coupling in Thread class
/*code is executing directly
*/
ThreadOne t1 = new ThreadOne();
t1.start();
//loose coupling in Runnable interface
/*code is not executing directly, we have to create a new thread object to access the start() method
*/
ThreadTwo t2 = new ThreadTwo();
Thread t = new Thread(t2);
t.start();
}
}
class ThreadOne extends Thread {
@Override
public void run() {
try{
for (int i = 1; i <= 5; i++) {
System.out.println("ThreadOne: " + i);
//sleep ThreadOne for one second
Thread.sleep(1000);
}
}catch (Exception e) {
System.out.println(e);
}
}
}
class ThreadTwo implements Runnable {
@Override
public void run() {
try{
for (int i = 1; i <= 5; i++) {
System.out.println("ThreadTwo: " + i);
//sleep ThreadTwo for one second
Thread.sleep(1000);
}
}catch (Exception e) {
System.out.println(e);
}
}
}
/*output(can be differ from time to time)
ThreadOne: 1
ThreadTwo: 1
ThreadOne: 2
ThreadTwo: 2
ThreadTwo: 3
ThreadOne: 3
ThreadOne: 4
ThreadTwo: 4
ThreadOne: 5
ThreadTwo: 5
*/
线程同步 (Thread Synchronization)
Synchronization in java is the capability to control the access of multiple threads to any shared resource.
java中的同步是控制多个线程对任何共享资源的访问的能力。
Java Synchronization is a better option where we want to allow only one thread to access the shared resource.
Java同步是一个更好的选择,我们希望只允许一个线程访问共享资源。
The synchronization is mainly used to
同步主要用于
To prevent thread interference.
为了防止线程干扰。
To prevent consistency problems.
为了防止一致性问题。
Java锁的概念 (Concept of Lock in Java)
Synchronization is built around an internal entity known as the lock or monitor. Every object has a lock associated with it. By convention, a thread that needs consistent access to an object’s fields has to acquire the object’s lock before accessing them, and then release the lock when it’s done with them.
同步是围绕称为锁或监视器的内部实体构建的。 每个对象都有一个与之关联的锁。 按照约定,需要对对象的字段进行一致访问的线程必须在访问对象之前获取对象的锁,然后在完成对它们的锁定后释放该锁。
In this example, there is no synchronization, so the output is inconsistent. Let’s see the example:
在此示例中,没有同步,因此输出不一致。 让我们来看一个例子:
class Pattern {
void printPattern(int n) { //method not synchronized
for (int i = 1; i <= 5; i++) {
System.out.println(n * i);
try {
Thread.sleep(400);
} catch (Exception e) {
System.out.println(e);
}
}
}
}
class MyThread1 extends Thread {
Pattern p;
MyThread1(Pattern p) {
this.p = p;
}
public void run() {
p.printPattern(5);
}
}
class MyThread2 extends Thread {
Pattern p;
MyThread2(Pattern p) {
this.p = p;
}
public void run() {
p.printPattern(100);
}
}
public class TestSynchronization1 {
public static void main(String args[]) {
Pattern obj = new Pattern();
MyThread1 p1 = new MyThread1(obj);
MyThread2 p2 = new MyThread2(obj);
p1.start();
p2.start();
}
}
/*output(can be differ from time to time)
5
100
200
10
15
300
20
400
25
500
*/
Java同步方法 (Java synchronized method)
If we declare any method as synchronized, it is known as a synchronized method.
如果我们将任何方法声明为同步方法,则称为同步方法。
A synchronized method is used to lock an object for any shared resource.
同步方法用于锁定任何共享资源的对象。
When a thread invokes a synchronized method, it automatically acquires the lock for that object and releases it when the thread completes its task. See the example below.
当线程调用同步方法时,它将自动获取该对象的锁,并在线程完成其任务时释放该锁。 请参见下面的示例。
class Pattern{
synchronized void printPattern(int n){//synchronized method
for(int i=1;i<=5;i++){
System.out.println(n*i);
try{
Thread.sleep(400);
}catch(Exception e){System.out.println(e);}
}
}
}
class MyThread1 extends Thread{
Pattern p;
MyThread1(Pattern p){
this.p=p;
}
public void run(){
p.printPattern(5);
}
}
class MyThread2 extends Thread{
Pattern p;
MyThread2(Pattern p){
this.p=p;
}
public void run(){
p.printPattern(100);
}
}
public class TestSynchronization2{
public static void main(String args[]){
Pattern obj = new Pattern();//only one object
MyThread1 t1=new MyThread1(obj);
MyThread2 t2=new MyThread2(obj);
t1.start();
t2.start();
}
}
/*output(can be differ from time to time)
5
10
15
20
25
100
200
300
400
500
*/
摘要 (Summary)
Here we arrived at the end of this article, So far we learned the Threads in Java. and how they are implementing. Hope this article helped you to develop your knowledge about these concepts. Let’s meet with another interesting article in the near future.
我们到了本文的结尾,到目前为止,我们已经学习了Java中的线程。 以及他们如何实施。 希望本文能帮助您发展有关这些概念的知识。 让我们在不久的将来见到另一篇有趣的文章。
Thank you for reading my article and Happy Learning 🙌😊
感谢您阅读我的文章和快乐学习🙌😊
翻译自: https://levelup.gitconnected.com/lets-learn-java-threads-eaf89b0fb929
java 线程学习