reactjs
Properties in React are immutable, read-only objects used to pass down data from a parent to its child component. Props facilitate the transfer of a piece of state or functionality you wish to make accessible to a sub component down the hierarchy. Syntactically, props resemble the way attributes are written in HTML. The difference being that the value of a prop is assigned to a JSX tag.
P中roperties阵营是不可改变的,只读的对象使用从父到其子组件传递下去的数据。 道具有助于转移您希望使层次结构中的子组件可以访问的状态或功能。 从语法上讲,道具类似于用HTML编写属性的方式。 区别在于将prop的值分配给JSX标签。
React is a component-based UI library that uses props as a means of directing data to an apportioned section of the application. It may be helpful to think of a prop like a mailbox. In the real-world, a mailbox is a container that stores physical structures in the form envelopes and packages. Envelopes hold information like letters, bills or if you’re lucky, money. In React, a prop can similarly store a variety of JavaScript data-structures including — but not limited to — primitive data-types, arrays, objects and functions.
R eact是基于组件的UI库,它使用prop作为将数据定向到应用程序分配部分的一种方式。 想到一个像邮箱这样的道具可能会有所帮助。 在现实世界中,邮箱是一个以信封和包装形式存储物理结构的容器。 信封中包含诸如信件,账单之类的信息,或者如果您幸运的话,还有金钱。 在React中,道具可以类似地存储各种JavaScript数据结构,包括但不限于原始数据类型,数组,对象和函数。
Operationally, the child component takes on the form of a house which the mailbox belongs to. When the parent component — the mailman — wants to pass data — a letter — down to a child, it adds the specified content into the rendering components corresponding prop — mailbox.
操作上 ,子组件采用邮箱所属房屋的形式。 当父组件-邮递员 -想要将数据-字母-传递给孩子,它将指定的内容添加到呈现组件对应的prop-邮箱中。
Passing props to a rendering component is no different from that of arguments to a function. It’s important to reiterate the notion that props are immutable, meaning they cannot — themselves — be modified. The value stored inside however, can.
P assing道具到呈现部件是从该的参数的函数没有什么不同。 重要的是要重申道具是不可变的,这意味着它们本身无法修改。 但是,可以存储在内部的值。
Since React is built on top of JavaScript, there are a variety of ways to write the syntax in the examples below. It ultimately depends on which version of JS or React you are using. Though I am running the current version of both, I chose to be more explicit so that you can better visualize what is happening.
因为 React是在JavaScript之上构建的,所以在下面的示例中有多种编写语法的方法。 最终取决于您使用的JS或React版本。 尽管我正在运行两者的当前版本,但我还是选择了更加明确的名称,以便您可以更好地可视化正在发生的事情。
将字符串作为道具传递 (Passing a String as a Prop)
App.jsx
App.jsx

http://localhost:3000
http://localhost:3000

App.js
is the parent component. It currently has no state declared and even so, no child component to pass it to.Let’s see what passing down a prop looks like from a parent component to it’s child.
App.js
是父组件。 它目前没有声明状态,甚至没有声明要传递给它的子组件,让我们来看看从父组件传递到子组件的prop传递的样子。
传递道具 (Passing A Prop)
App.js
App.js
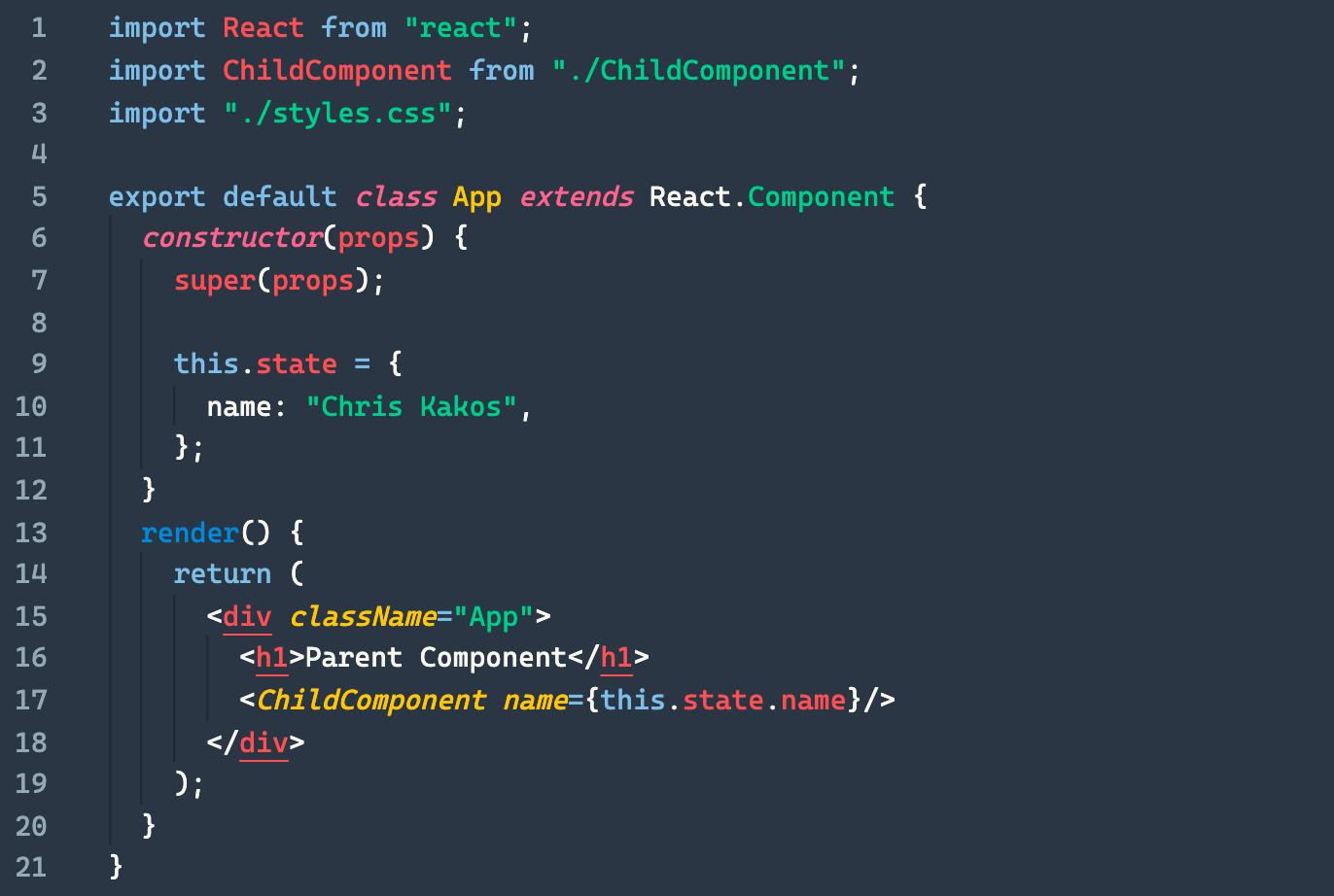
Now App.js
has some state defined with a key of name
set to the value of a string — "Chris Kakos"
. On line 17, the parent is passing a prop — which is appropriately called name
— down to its child component and setting the value of it to the current state — this.state.name
— inside of JSX tags.
现在, App.js
定义了一些状态 ,该状态的键name
设置为字符串的值"Chris Kakos"
。 在第17行上,父级将一个prop(适当地称为name
)传递到其子级组件,并将其值设置为JSX标签内的当前状态this.state.name
。
接收道具 (Receiving A Prop)
ChildComponent.js
ChildComponent.js

ChildComponent.js
is a functional component written in ES6 syntax. It is important to understand that props are sent down from the parent as an object. When a child component receives props, it can access them by using the identifer appropriately name props
. Therefore, any prop passed down can be accessed with dot notation, starting with props
followed by the name of the prop you wish to access — in this case name
— as demonstrated on line 11.
ChildComponent.js
是使用ES6语法编写的功能组件。 重要的是要理解道具是作为对象从父级发送下来的。 当子组件接收道具时,它可以通过使用适当的标识符props
来访问它们。 因此,任何传下来的道具都可以用点符号来访问,以props
开头,后跟要访问的props
名称(在本例中为name
,如第11行所示 。
http://localhost:3000
http://localhost:3000

传递功能作为道具 (Passing a Function as a Prop)
App.js
App.js

A more intermediate example would be to pass down some state as well as functionality that modifies state. First thing is to declare a piece of state to modify. In this case, I decided to create a counter that increments by 1. On Line 11, I set a key named count
to a value of 0
. Next I have to create the functionality that is going to modify the current value of count
.
一个更中间的示例是传递某些状态以及修改状态的功能。 第一件事是声明一个要修改的状态。 在这种情况下,我决定创建一个递增1的计数器。在第11行,将名为count
的键设置为0
。 接下来,我必须创建要修改count
的当前值的功能。
On Line 15, I wrote an ES6 arrow function. I start by making a copy of the current state of count
— it is not recommended to directly modify state. I then set the state to increment by 1 every time increment()
is invoked.
在第15行上 ,我编写了一个ES6箭头函数。 我开始通过使当前状态的副本count
- 不 建议 直接修改状态 。 然后,我将状态设置为在每次调用increment()
时increment()
1。
Now that the functionality has been built, I use some ES6 to demonstrate the destructuring of two variables — Line 23 — and pass the current state of them, as well as my newly built functionality, into the ChildComponent
as props.
现在的功能已经建成,我用一些ES6证明解构两个变量的L - INE 23 -并通过他们的当前状态,以及我的新建功能,进入ChildComponent
作为道具。
ChildComponent.js
ChildComponent.js

The props are received the same as the previous example only this time, I need a way to invoke increment()
in order to modify the current state of count
. To achieve this — on Line 19 — I added a button with an onClick
Event Handler that will invoke increment()
each time the button is clicked, ultimately modifying the current state of count
.
这次仅以与前面的示例相同的方式接收道具,我需要一种方法来调用increment()
以修改count
的当前状态。 为此,在第19行上,我添加了一个带有onClick
事件处理程序的按钮 每次单击按钮时,都会调用increment()
,最终修改count
的当前状态。
http://localhost:3000
http://localhost:3000

*点击* (*CLICK*)

*点击* (*CLICK*)

*点击* (*CLICK*)

结论 (Conclusion)
Props play a vital role in making code more modular. This was a quick demonstration to illustrate how to get started. There is a lot more complex was to use props that really optimize the functionality. I hope this has been a good starting point. For further information and examples, look no further than the official React documentation.
道具在使代码更具模块化方面起着至关重要的作用。 这是一个快速演示,以说明如何入门。 使用真正能够优化功能的道具要复杂得多。 我希望这是一个好的起点。 有关更多信息和示例,请参阅官方的React 文档 。
Onward.
向前 。
翻译自: https://medium.com/@ckakos/react-js-passing-props-a65bb5200891
reactjs