6.5.0-next.0
This is a curation of Next.js documentation, explanations, and advice. With a few code snippets and images to help along the way.
这是Next.js文档,说明和建议的策划。 提供一些代码片段和图像,以帮助您。
It focusses mainly on how the ‘server-side’ works in Next.js. Spoiler — it can do more than just render HTML
它主要关注Next.js中“服务器端”的工作方式。 破坏者-它不仅可以呈现HTML,还可以做更多的事情
Currently, Next.js latest version is 9.5.
当前,Next.js的最新版本是9.5。
什么是Next.js? (What is Next.js?)
It’s a framework for building server-side rendered React applications.
这是一个用于构建服务器端渲染的React应用程序的框架。
But Next.js is not just for building an application’s frontend, because of its ‘server-side’, it can be used to build a full backend for the application as well.
但是Next.js不仅用于构建应用程序的前端,因为它具有“服务器端”的功能,它也可以用于构建应用程序的完整后端。
服务器端如何工作? (How does the server-side work?)
The ‘server-side’ in Next.js applications can be separated into two parts:
Next.js应用程序中的“服务器端”可以分为两部分:
- The server-side rendering — how Next.js generates HTML on the server-side and sends it to the client 服务器端渲染-Next.js如何在服务器端生成HTML并将其发送给客户端
- The backend server — how Next.js supports a backend server and APIs 后端服务器-Next.js如何支持后端服务器和API
服务器端渲染(预渲染) (The server-side rendering (pre-rendering))
Pre-rendering = general term for rendering HTML before it is sent to the client
预渲染=在将HTML发送给客户端之前渲染HTML的通用术语
By default, Next.js will pre-render every page, but can do so in two different ways:
默认情况下,Next.js将预渲染每个页面,但是可以采用两种不同的方式进行渲染:
- Static generation 静态生成
- Server-side rendering (SSR) 服务器端渲染(SSR)
An application can use a combination of these methods, where the method used for a given page is determined by the page’s data requirements — see the ‘data fetching on the server-side’ section below for more details.
应用程序可以使用这些方法的组合,其中用于给定页面的方法由页面的数据要求决定-有关更多详细信息,请参见下面的“服务器端数据获取”部分。
Static generation means that the HTML is generated once on the server at build-time, and this HTML is reused for each client request.
静态生成意味着HTML在构建时在服务器上生成一次,并且该HTML可用于每个客户端请求。
This is the recommended approach for performance reasons (better caching), however, it comes with some obvious limitations when it comes to fetching data for that page — the data can only be fetched during the application’s build process using the getStaticProps
method.
出于性能原因(更好的缓存),这是推荐的方法,但是在获取该页面的数据时,它存在一些明显的限制-只能在应用程序的构建过程中使用getStaticProps
方法来获取数据。
This might be acceptable when the data being fetched does not change often, and/or in scenarios where the user does not always need the most updated data. But often it is crucial that the user is shown a current view of the data, meaning that static generation of pages cannot be used.
当获取的数据不经常更改时,和/或在用户并不总是需要最新数据的情况下,这可能是可以接受的。 但是向用户显示数据的当前视图通常很关键,这意味着不能使用页面的静态生成。
Server-side rendering (SSR) means that the HTML is generated on the server on each request for the page — the HTML is ‘dynamic’ rather than ‘static’ as it will depend on the data required.
服务器端呈现(SSR)意味着HTML是在每次请求页面时在服务器上生成的-HTML是“动态的”而不是“静态的”,因为它将取决于所需的数据。
Each time a client requests the page, the server will fetch the data for that page, and generate the page’s HTML using the data. Only once all of this is complete will the HTML be sent back to the client.
每次客户端请求页面时,服务器将获取该页面的数据,并使用该数据生成页面HTML。 只有完成所有这些操作后,HTML才会发送回客户端。
Next.js offers two data fetching methods for SSR — getServerSideProps
and getInitialProp
— which are discussed in the ‘data fetching on the server-side’ section below.
Next.js报价两个数据获取用于SSR方法- getServerSideProps
和getInitialProp
-这是在“数据获取在服务器侧”下面部分讨论。
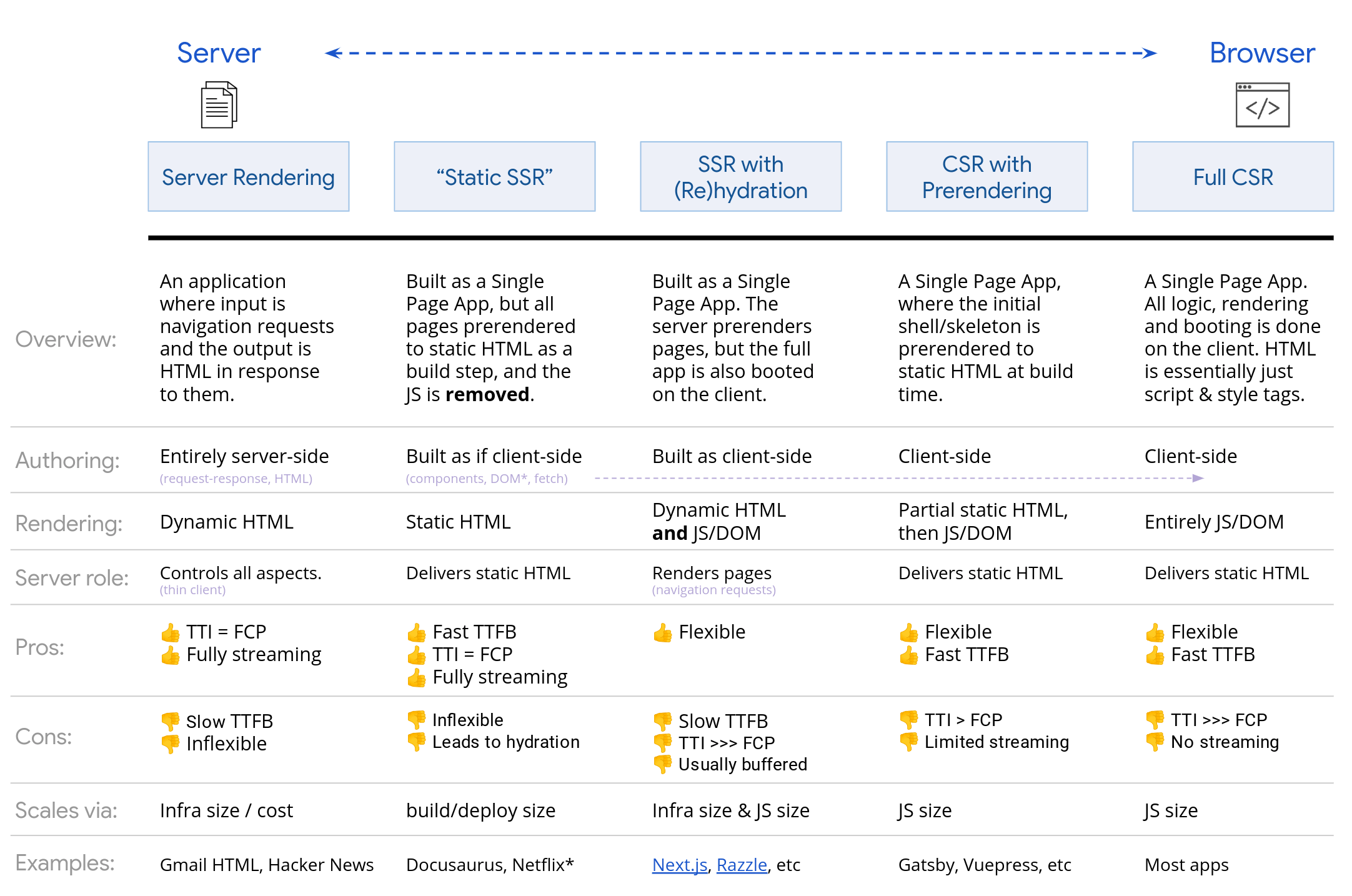
后端服务器 (The backend server)
The backend part of a Next.js application (assuming you need one) can be built in two ways:
可以通过两种方式构建Next.js应用程序的后端部分(假设您需要一个):
- Using a custom server 使用自定义服务器
- Using serverless API Routes 使用无服务器API路由
A custom server is a normal NodeJS server which can be built using your choice of framework and libraries — typically Express.
定制服务器是普通的NodeJS服务器,可以使用您选择的框架和库(通常是Express)来构建。
The server must return the static resources for each frontend route in the application (using the Next.js getRequestHandler
function), but apart from this, the server can behave like any other with middlewares, API routes, database connection etc.
服务器必须为应用程序中的每个前端路由返回静态资源(使用Next.js getRequestHandler
函数),但除此之外,服务器可以像其他任何中间件,API路由,数据库连接等一样运行。
API Routes are Next.js built-in functions for writing backend APIs. Each function is a request handler written using “Express.js-like methods”, and will be deployed as a serverless function.
API路由是用于编写后端API的Next.js内置函数。 每个函数都是使用“类似于Express.js的方法”编写的请求处理程序,并将被部署为无服务器函数。
(req, res) => { // send back some json }
(req, res) => { // send back some json }
API routes use file-system routing — any file under the /page/api/
directory will be deployed as an API endpoint. For example, a function handler in /pages/api/users.js
will be available at for requests athttp://your-domain.com/api/users
API路由使用文件系统路由- /page/api/
目录下的任何文件都将部署为API端点。 例如,可以通过http://your-domain.com/api/users
上的请求获得/pages/api/users.js
的函数处理程序。
Dynamic routing is also supported, allowing for URL query parameters to be used. For example, a handler in /pages/api/users/[userId].js
will have access to req.query.userId
还支持动态路由,允许使用URL查询参数。 例如,/ /pages/api/users/[userId].js
的处理程序将有权访问req.query.userId
Finally, catch-all routes are also possible as /pages/api/[...rest].js
(where 'rest' can be named anything). These can be useful if the application has some of its own APIs but also acts as a proxy to an external backend service.
最后, /pages/api/[...rest].js
路线也可以作为/ /pages/api/[...rest].js
/ [... rest] /pages/api/[...rest].js
(其中“ rest”可以命名为任何东西)。 如果应用程序具有一些自己的API,但还可以充当外部后端服务的代理,则这些功能将非常有用。
Some of the main differences between API Routes and a typical Express/NodeJS server can be seen in the code above:
可以在上面的代码中看到API路由和典型的Express / NodeJS服务器之间的一些主要区别:
Connecting to the database must be done at the start of every handler. The database
connect()
function here will actually first check if there is already an active connection, and only if there is not will it attempt to connect.连接到数据库必须在每个处理程序的开始处完成。 实际上,这里的数据库
connect()
函数将首先检查是否已经有活动的连接,只有在没有活动的连接时,它才会尝试连接。Middleware comes in the form of higher-order functions that wrap and enhance the handler — the above function is using
withAuth
middleware that checks for authentication before allowing the request to be handled.中间件以包装和增强处理程序的高级函数的形式提供—上面的函数使用
withAuth
中间件,该中间件在允许处理请求之前检查身份验证。
我应该采用哪种后端方法? (Which backend approach should I go for?)
Next.js officially recommends using API Routes for many good reasons that are explained in the feature’s initial RFC.
Next.js正式建议使用API路由,这有很多很好的理由, 该功能的初始RFC中对此做了解释 。
In summary, using a custom server has a number of drawbacks when compared to API Routes: it will remove certain optimizations, it usually requires a separate step for builds and local development, and it cannot scale as well.
总而言之,与API路由相比,使用自定义服务器有许多缺点:它将删除某些优化,通常需要单独的步骤进行构建和本地开发,并且无法扩展。
Whilst a custom server might be tempting at first — you can probably copy and paste the code from the last Express server you wrote — my advice is to prefer using API routes whenever possible, especially for new projects.
尽管自定义服务器起初可能很诱人-您可能可以从上次编写的Express服务器中复制和粘贴代码-但我的建议是尽可能使用API路由,特别是对于新项目。
Using them makes building full-stack applications incredibly fast — building an API doesn't have to take any longer than building a React component. It also guarantees that your backend is scalable, decoupled, and performant.
使用它们使构建全栈应用程序的速度变得异常快-构建API不需要花比构建React组件更多的时间。 它还可以确保您的后端可扩展,分离和高性能。
Migrating an existing custom server to API routes isn’t always necessary, but if you do choose to migrate, then be sure to check out this great blog post which helps to explain how.
将现有的自定义服务器迁移到API路由并非总是必要的,但是,如果您确实选择迁移,那么一定要查看这篇出色的博客文章 , 该文章有助于解释如何进行迁移。
在服务器端获取数据 (Data fetching on the server-side)
Next.js supports two types of rendering on the server as explained above (static generation and SSR) — which of these it uses for a given page is determined by how that page fetches data.
如上所述,Next.js在服务器上支持两种类型的呈现(静态生成和SSR)-它用于给定页面的哪种呈现方式取决于该页面获取数据的方式。
General rule: for SSR prefer to use getServerSideProps
over getInitialProps
to avoid the situation where data fetching can happen on both server and client-side.
一般规则 :对于SSR,宁愿使用getServerSideProps
不是getInitialProps
来避免在服务器端和客户端都可能发生数据获取的情况。
For the initial page load,
getInitialProps
will run on the server only.getInitialProps
will then run on the client when navigating to a different route via thenext/link
component or by usingnext/router
.对于初始页面加载,
getInitialProps
仅在服务器上运行。 通过next/link
组件或使用next/router
导航到其他路由时,getInitialProps
将在客户端上运行 。
This means that your getInitialProps
code has to be written in a way that works on both client and server-sides — redirecting a user might need ctx.res.writeHead
(server) or it might need Router.push
(client).
这意味着您的getInitialProps
代码必须以在客户端和服务器端均可使用的方式编写-重定向用户可能需要ctx.res.writeHead
(服务器),或者可能需要Router.push
(客户端)。
getServerSideProps
has the same effect as getInitialProps
, but is more predictable because it will only ever run on the server.
getServerSideProps
与getInitialProps
具有相同的效果,但更可预测,因为它只会在服务器上运行。
要完成的两个技巧: (Two tips to finish off:)
Next.js moves fast — be sure to check out their blog for the latest updates as it’ll nearly always include something awesome.
Next.js运作Swift-请务必查看其博客以获取最新更新,因为它几乎总是包含一些很棒的内容。
If you’re starting a new Next.js application, take a look at the official examples repo to see if there’s an example setup for your chosen tech stack (there almost always will be).
如果您要启动一个新的Next.js应用程序,请查看官方示例存储库,以查看是否有所选技术堆栈的示例设置(几乎总是如此)。
翻译自: https://medium.com/weekly-webtips/next-js-on-the-server-side-notes-to-self-e2170dc331ff
6.5.0-next.0