angular 结构型指令
If you’ve ever done any development with Angular (and I assume you have if you’ve made it here), it is inevitable that you have come across Structural directives. They’re super easy to identify because they are all prefaced with a *; some of their greatest hits are NgIf, NgFor and NgSwitch, all of which are crucial to helping us as developers build lean, clean apps. But why the star? To understand Angular’s use of asterixes, we first need to understand what Structural directives actually do and then we can figure out what’s actually going on behind the scenes.
如果您曾经使用Angular进行过任何开发(并且我假设您已经在这里完成了开发),那么不可避免地会遇到Structured指令。 它们非常容易识别,因为它们都以*开头。 NgIf,NgFor和NgSwitch是其中的佼佼者,当开发人员构建精简,干净的应用程序时,它们对帮助我们至关重要。 但是为什么是明星? 要了解Angular对星号的使用,我们首先需要了解Structured指令的实际作用,然后我们才能弄清楚幕后的实际情况。
Within Angular, there are three main types of directives — structural, attribute and component. We’re going to largely ignore components as while they’re technically directives that manage a section of HTML based on their template, they do not quite fit into the scope of this blog.
在Angular中,有三种主要类型的指令-结构,属性和组件。 我们将在很大程度上忽略组件,因为从技术上来说,组件是根据其模板管理HTML的一部分的指令,但它们并不完全适合本博客的范围。
Attribute directives are used to change the element they are added to. Attribute directives, for the most part, appear to be normal HTML attributes. In the below example, we have used the Angular CLI to run ng g d exampleDirective (long form: ng generate directive exampleDirective) which has automatically created our exampleDirective.directive.ts and exampleDirective.directive.spec.ts files (in whatever directory you ran your command in) and has also automatically declared our new directive in our AppModule. This means that we are now free to use our directive like so:
属性指令用于更改添加到它们的元素。 在大多数情况下,属性指令似乎是普通HTML属性。 在下面的示例中,我们使用Angular CLI运行ng gd exampleDirective (长格式:ng generate指令exampleDirective),它自动创建了exampleDirective.directive.ts和exampleDirective.directive.spec.ts文件(在您运行的任何目录中)您的命令),并且还自动在AppModule中声明了我们的新指令。 这意味着我们现在可以像下面这样自由使用我们的指令:
<div exampleDirective>Lorem Ipsum</div>
Attribute directives, when attached to a host element, look like standard HTML attributes we might set in the course of building a web page. This will not give us any default behavior though — within our directive.ts file, usually in the constructor, we will grab an elementRef to our target element and apply whatever style changes we want to be applied to our targeted element. There is an awful lot that can be done with Attribute directives including stacking multiple on a host element or even having an Attribute directive affect the behavior of another directive but again, outside the scope of this article — if you’re interested, the Angular documentation is a great place to get a better grasp on these.
属性指令附加到宿主元素后,看起来就像我们在构建网页时可能设置的标准HTML属性。 不过,这不会给我们任何默认行为-在我们的directive.ts文件中,通常是在构造函数中,我们将获取一个elementRef到目标元素,并将想要应用于样式的任何样式更改应用于目标元素。 使用Attribute指令可以完成很多工作,包括在主机元素上堆叠多个指令,甚至让Attribute指令影响另一个指令的行为,但是同样,超出了本文的范围,如果您感兴趣的话,请访问Angular文档是更好地掌握这些内容的好地方。
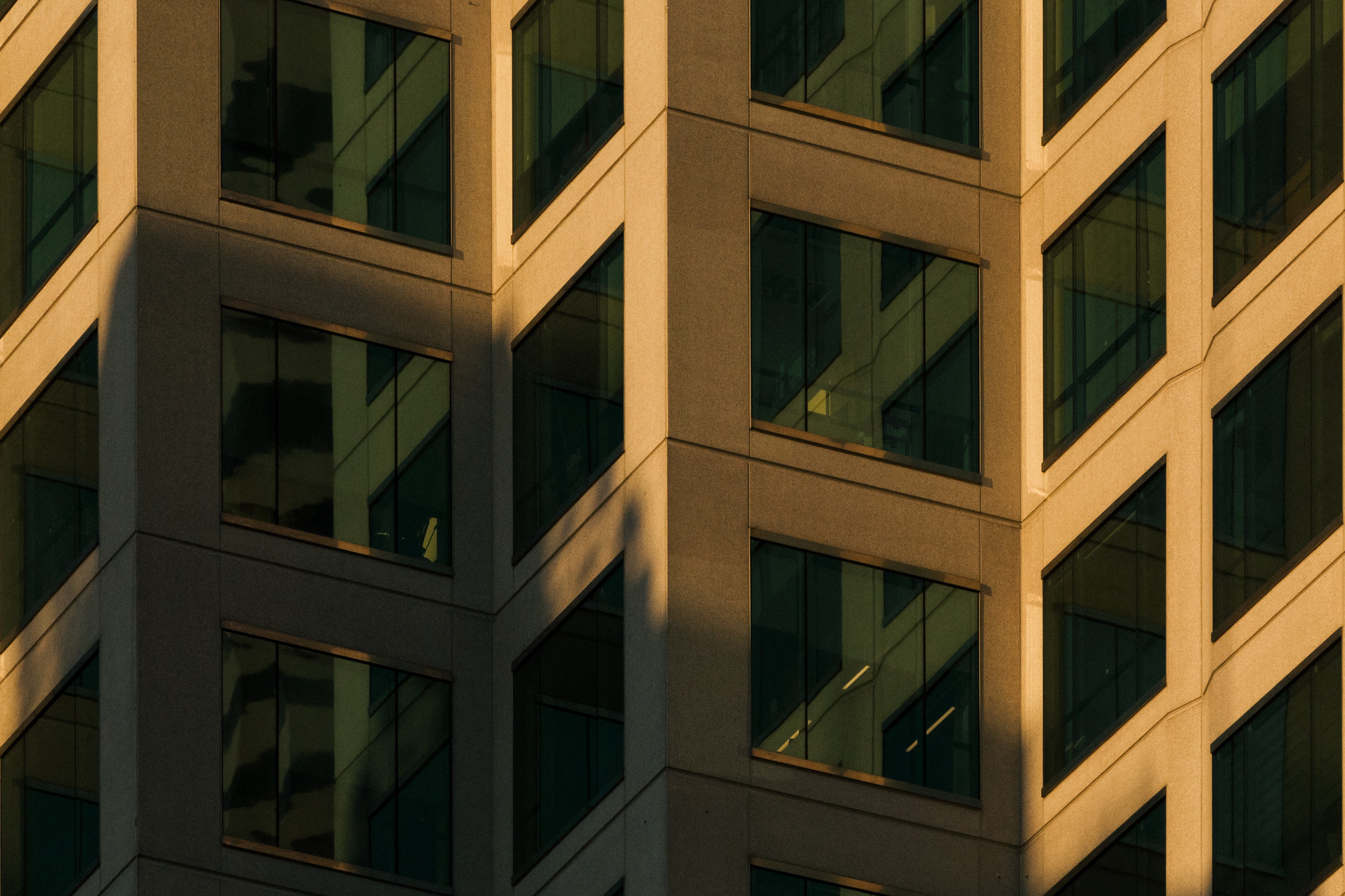
Structural directives differ from Attribute directives in that rather than modifying their host element, Structural directives modify the DOM and surrounding elements. Rather than directly accessing the DOM, structural directives allow us to write logic that takes the from of if X, then Y
or for each thing, do this
and so on. There can only be ONE structural directive per element. Below is an example using NgIf which evaluates an expression and returns a boolean.
结构化指令与属性指令的不同之处在于, 结构化指令不是修改其宿主元素,而是修改DOM和周围的元素。 结构指令允许我们编写逻辑,而不是直接访问DOM,而该逻辑采用if X, then Y
或其他for each thing, do this
取值,等等。 每个元素只能有一个结构指令。 以下是使用NgIf的示例,该示例对表达式求值并返回布尔值。
<div *ngIf="candy = tastesGood">
Candy always tastes good so this will show.
</div><div *ngIf="red = blue">
Red will never equal blue so ngIf evaluates to false.
This div WILL NOT BE PART OF THE DOM.
</div>
Even though these two examples are simplistic pseudo code, they do a good job of showing how we use a structural directive to change the structure of the DOM. It’s worth noting that the div which has the ngIf evaluating to false is not hidden or obscured, it is rather FULLY REMOVED from the DOM. Angular’s default behavior is meant to help us prevent memory leaks and make sure that our app is running as quickly and smoothly as possible. In some situations, it may make more sense to not fully destroy and recreate components and you may want to circumvent this default behavior; this is a consideration that you will be able to make with experience and context within your app.
即使这两个示例都是简单的伪代码,它们也很好地说明了我们如何使用结构化指令来更改DOM的结构。 值得注意的是,具有ngIf评估为false的div不会被隐藏或掩盖,而是从DOM中完全删除的。 Angular的默认行为旨在帮助我们防止内存泄漏,并确保我们的应用程序尽可能快且平稳地运行。 在某些情况下,不完全销毁和重新创建组件可能更有意义,并且您可能想规避这种默认行为; 这是您可以在应用程序中的经验和上下文中做出的考虑。
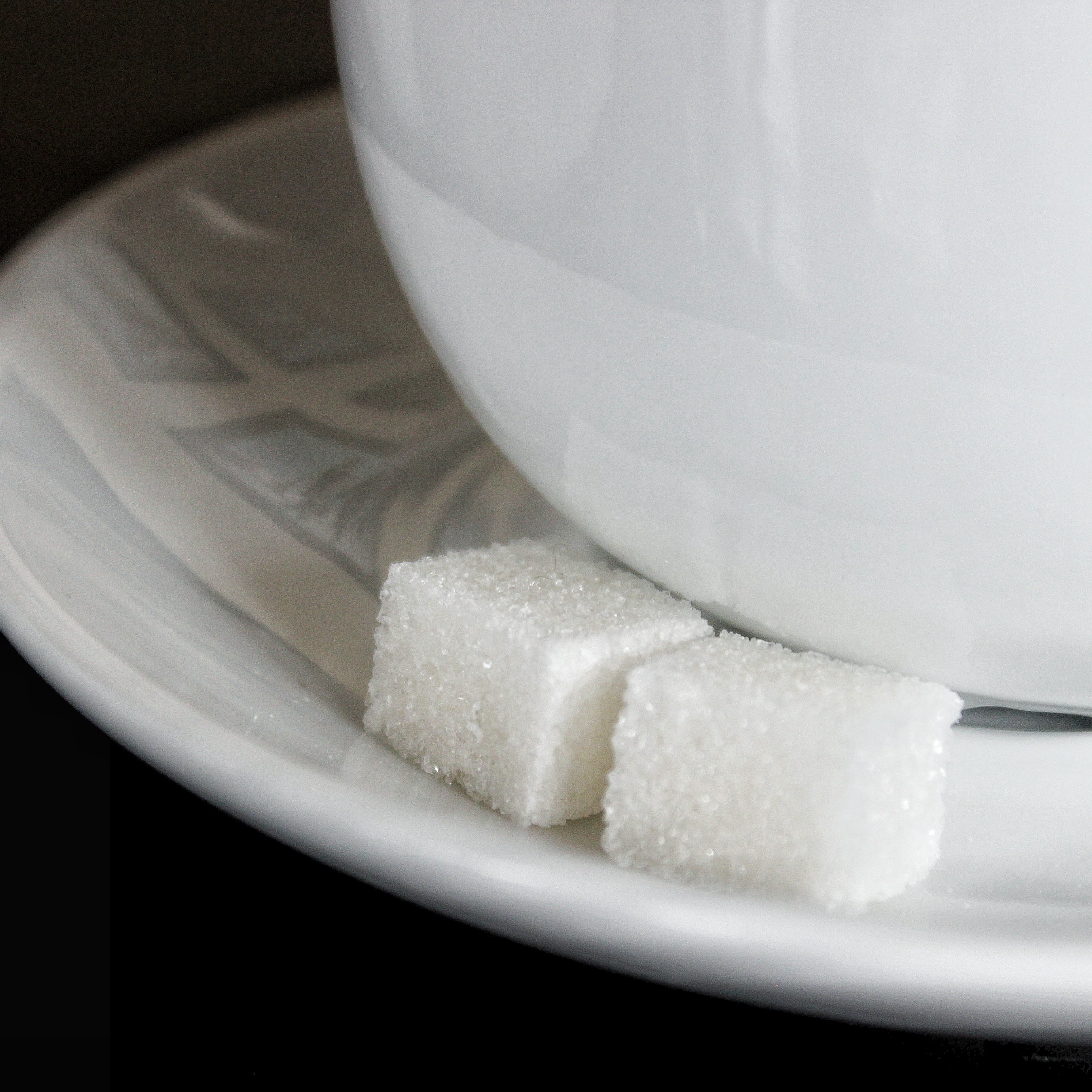
Finally, we’re ready to talk about the *s all over our apps and unfortunately, this may be the most boring part of the whole article because what the stars really come down to is simple syntactic sugar. When Angular reads our code and goes to compile, our *ngIf (or any other directives) are actually read as:
最后,我们准备讨论所有应用程序中的*,但是不幸的是,这可能是整篇文章中最无聊的部分,因为真正的含义是简单的语法糖。 当Angular读取我们的代码并进行编译时,我们的* ngIf(或任何其他指令)实际上被读取为:
<ng-template [ngIf]="tastesGood">
<div>Candy always tastes good so this will show.</div>
</ng-template>
Those *s serve as a signal to Angular that what we are actually asking for is to take our targeted element, wrap it in an <ng-template> element, and then on the wrapper use ngIf property binding. Even though this example is specific to *ngIf, the behind the scenes behavior of structural directives is largely the same. Let’s pretend we have an array called TMNT with four entries.
这些*信号向Angular发出信号,我们实际上要的是获取目标元素,将其包装在<ng-template>元素中,然后在包装器上使用ngIf属性绑定。 即使此示例特定于* ngIf,结构指令的幕后行为在很大程度上也相同。 假设我们有一个名为TMNT的数组,其中包含四个条目。
<table *ngFor="let turtle of TMNT">
<tr> {{turtle}} </tr>
</table>//becomes<ng-template ngFor let-turtle [ngForOf]="TMNT">
<table>
<tr> {{turtle}} </tr>
</table>
</ng-template>
This is of course nothing more than a pseudo simplification — *ngFor has many more optional args than I have incorporated here as this is meant purely to show you how much syntactic sugar can be provided by a single *.
当然,这不过是伪简化— * ngFor的可选参数比我在这里结合的要多,因为这纯粹是为了向您展示单个*可以提供多少语法糖。
Feel free to reach out with any questions, comments or struggles you’ve had learning Angular. I am far from a master but I love to keep paying my learning forward in any way that I can.
随时与您学习过Angular的任何问题,评论或挣扎联系。 我离大师还很远,但是我喜欢继续以我能做的任何方式继续学习。
翻译自: https://medium.com/@rossperkel/look-at-the-st-rs-structural-directives-in-angular-ca4b6f59d7f9
angular 结构型指令