npm 更新 npm
You might have faced issues with npm install
automatically picking up a new version of a dependency that broke your build. And you might have also heard of the incident where a programmer deleted several npm packages that millions of people depended on. Fortunately, there are some good practices we can adopt to minimize our exposure to external factors.
您可能会遇到npm install
自动选择破坏您的构建的新版本依赖项的问题。 您可能还听说过程序员删除了数以百万计的人依赖的几个npm软件包的事件。 幸运的是,我们可以采用一些良好的做法来最大程度地减少与外部因素的接触。
npm版本标准 (npm versioning standard)
npm packages follow the major.minor.patch
versioning format (e.g., 7.3.4). While the numbers themselves are arbitrary, how they change is not:
npm软件包遵循major.minor.patch
版本格式(例如7.3.4)。 尽管数字本身是任意的,但它们的变化方式不是:
MAJOR versions are upgraded when a major overhaul of existing interfaces is committed. This often entails backwards incompatibility. If you depend on a dependency that you’re upgrading major versions, you might have to modify your code.
承诺对现有接口进行大修时,将升级MAJOR版本。 这通常需要向后不兼容。 如果您依赖于要升级主要版本的依赖项,则可能必须修改代码。
MINOR versions are upgraded when new features or APIs have been added the product. Since existing interfaces are not modified in a backwards-incompatible manner, you may safely upgrade to a minor version without modifying your code.
在产品中添加了新功能或API后,将升级MINOR版本。 由于没有以向后不兼容的方式修改现有接口,因此您可以安全地升级到次要版本,而无需修改代码。
PATCH versions are upgraded when a hotfix or implementation changes are made that are inconsequential to the users. You can almost always upgrade patch versions without worry.
当进行修补程序或实现更改对用户无关紧要时,将升级PATCH版本。 您几乎可以随时升级补丁程序版本而不必担心。
If you would like to learn more about this versioning protocol, you can read more about it over at semver.org.
如果您想了解有关此版本协议的更多信息,可以在semver.org上阅读有关此版本协议的更多信息。
With the understanding of npm package versions, let’s get started with the obvious good practice of controlling dependency versions.
了解npm软件包版本后,让我们开始控制依赖版本的显而易见的好习惯。
限制依赖版本 (Restrict dependency versions)
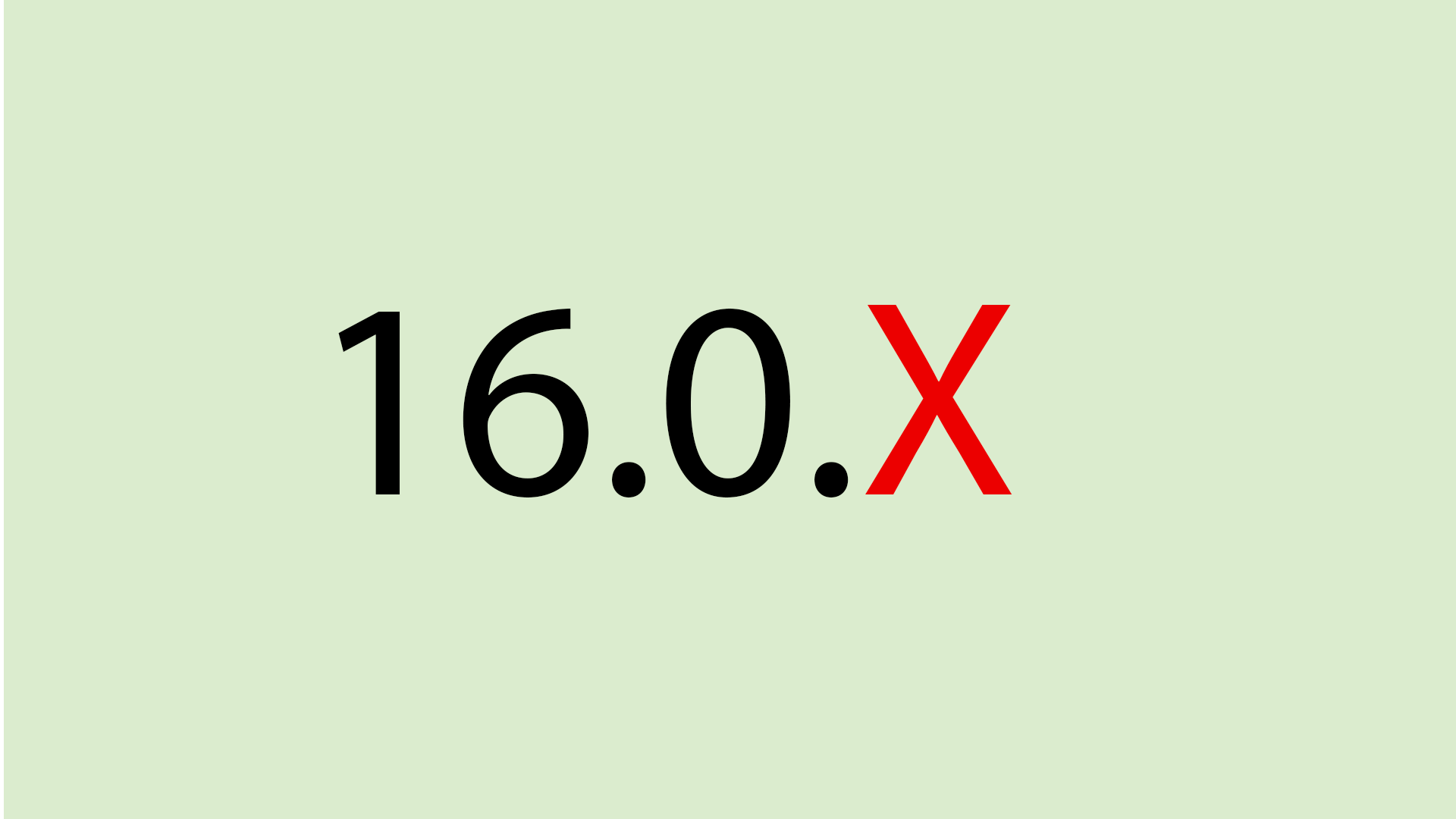
npm supports tokens that you can use along the version of a dependency to inform npm what versions are okay to ‘automatically’ move to. Here are the most important tokens:
npm支持可用于依赖项版本的令牌,以告知npm可以“自动”转移到哪个版本。 这是最重要的标记:
The Tilde (~) token: Add this token before a version to restrict the dependency version to upgrading patch versions only. For example, if you specify your
react
dependency inpackage.json
as~16.0.4
, you can be assured that you will stay on the16.0.x
versions.Tilde(〜)令牌 :在版本之前添加此令牌,以将依赖版本限制为仅升级补丁程序版本 。 例如,如果您在
package.json
中将react
依赖项指定为~16.0.4
,则可以确保您将使用16.0.x
版本。The Caret (^) token: Add this tokens before a version to automatically upgrade patch and minor versions, but not major. Referencing the
react
example, if you were to specify^16.0.4
as the dependency in yourpackage.json
, it is allowed to be upgraded automatically in the16.x.x
range, but you will never be moved to versions ≥17.x.x
.脱字号(^)令牌:在版本之前添加此令牌可自动升级补丁程序和次要版本,但不是主要版本。 引用
react
示例,如果要在package.json
^16.0.4
指定为依赖项,则可以在16.xx
范围内自动升级它,但绝不会移至版本17.xx
。≥, >, ≤, < operators: You can also use the inequality operators to declare your upper or lower bound of a dependency. Beware, however, that the ≥, > only declare lower bounds, and you will be upgraded to new major versions automatically.
≥,>,≤,<运算符:您还可以使用不等式运算符声明依赖项的上限或下限。 但是请注意,≥,>仅声明下限,您将自动升级到新的主要版本。
As a quick tip, you can also use these tokens in the command-line when you are installing the dependencies! For example, if you were installing react
and you wanted to restrict yourself to the 16.x.x
versions, you can perform the following command
快速提示,您还可以在安装依赖项时在命令行中使用这些令牌! 例如,如果您要安装react
并希望将自己限制为16.xx
版本,则可以执行以下命令
npm install --save react@^16
At the moment of writing, this will install react@16.13.1
for you, and insert the this in your package.json
file:
在撰写本文时,这将为您安装react@16.13.1
并将其插入您的package.json
文件中:
"react": "^16.13.1"
提交您的package-lock.json (Committing your package-lock.json)
The package-lock.json
is the ultimate source of truth; npm generates this file after you do an npm install
, telling you precisely what dependency versions were installed — and the dependencies of these dependencies!
package-lock.json
是真理的最终来源。 npm npm install
完成后,npm会生成此文件,并准确告诉您所安装的依赖版本以及这些依赖的依赖!
While you can control the direct versions of the dependencies you choose to use, you are not in control of what dependencies these dependencies use (if that makes sense).
尽管您可以控制选择使用的依赖项的直接版本,但是您无法控制这些依赖项使用的依赖项(如果有道理)。
However, the package-lock.json
can serve as a snapshot of the exact installation that you performed when your build was working. If you version control this file, then you can always reinstall the exact same versions of all dependencies! Here is a quote from the npm documentation on package locks.
但是, package-lock.json
可以用作您在构建工作时执行的确切安装的快照。 如果您对该文件进行版本控制,则始终可以重新安装所有依赖项的完全相同的版本! 这是npm文档中有关程序包锁的报价。
To prevent this potential issue, npm uses package-lock.json or, if present, npm-shrinkwrap.json. These files are called package locks, or lockfiles.
为了防止这种潜在的问题,NPM用途包lock.json或者,如果存在的话, NPM-shrinkwrap.json 。 这些文件称为程序包锁或锁文件。
This file describes an exact, and more importantly reproducible
node_modules
tree. Once it’s present, any future installation will base its work off this file, instead of recalculating dependency versions off package.json.该文件描述了一个精确的 ,更重要的是可复制的
node_modules
树。 一旦存在,任何以后的安装都将基于此文件进行工作,而不是根据package.json重新计算依赖项版本。
奖励:如果npm下降怎么办? (Bonus: what if npm is down?)
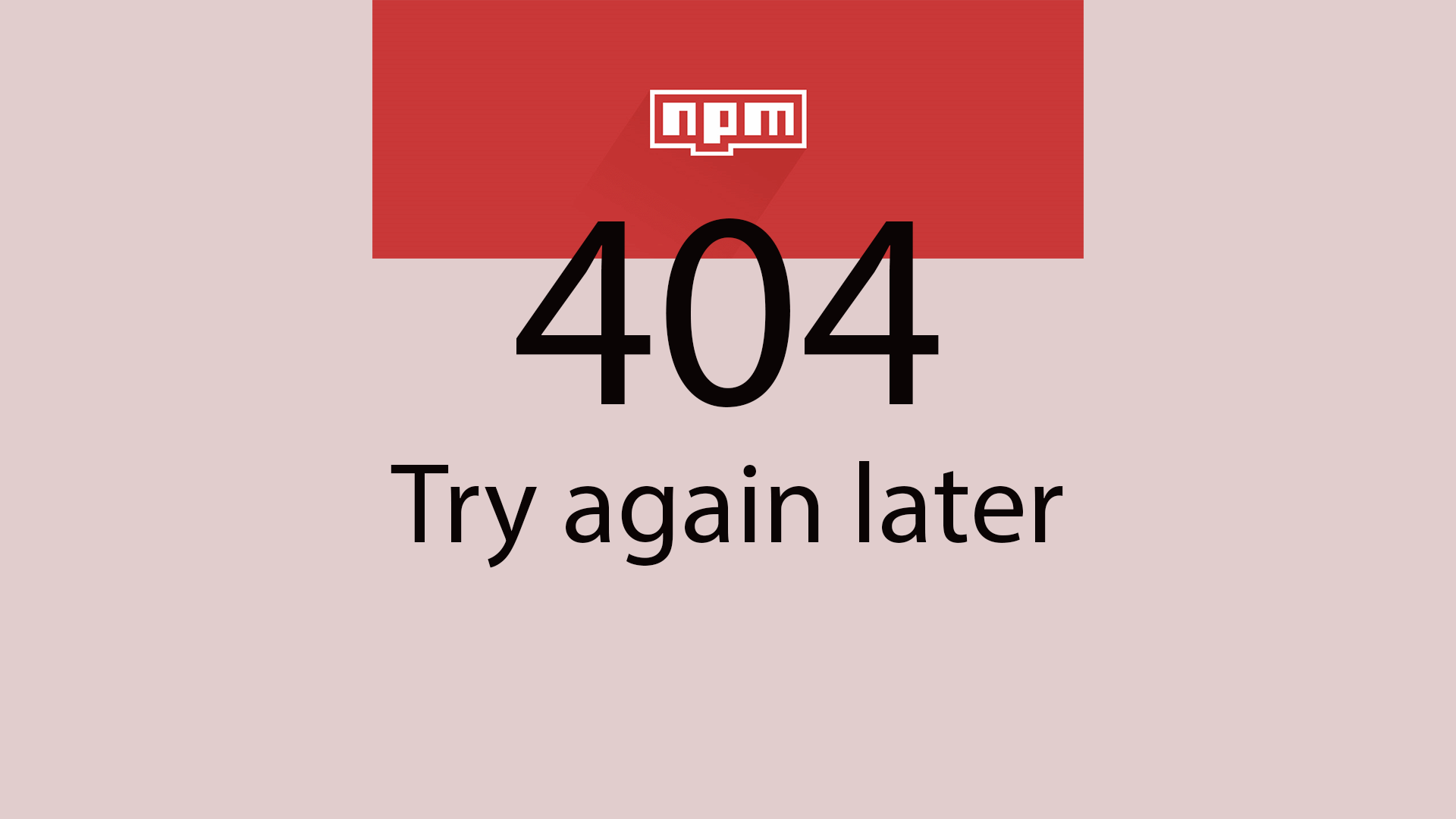
This is a real concern. What if the npm repository is down? Any and all npm commands that depend on connecting to the npm repository will fail. This should not be a threat to your production since files and dependencies should be static on production builds. But for development builds, you might be using npm install
regularly.
这是一个真正的问题。 如果npm存储库已关闭怎么办? 依赖于连接到npm存储库的所有npm命令都会失败。 这不会对您的生产构成威胁,因为文件和依赖项在生产构建中应该是静态的。 但是对于开发版本,您可能会定期使用npm install
。
If your use case would be severely impacted by even a few hours of downtime, you might want to consider archiving and storing the whole node_modules
tree so that you are never dependent on the npm repository.
如果您的用node_modules
因甚至几个小时的停机而受到严重影响,则您可能需要考虑归档和存储整个node_modules
树,以使您永远node_modules
依赖npm存储库。
翻译自: https://medium.com/swlh/avoid-npm-headaches-with-good-practices-d2d52ff257f9
npm 更新 npm