next.js
There are so many ways we can develop and deploy React application. Mostly we are deploying React applications in serverless mode nowadays. Next.js is a React Framework for production. Next.js gives you the best developer experience with all the features you need for a production environment such as hybrid static & server rendering, TypeScript support, smart bundling, route pre-fetching, and more. No config needed.
我们有很多方法可以开发和部署React应用程序。 如今,大多数情况下,我们正在以无服务器模式部署React应用程序。 Next.js是一个用于生产的React框架。 Next.js为您提供最佳的开发人员体验,以及生产环境所需的所有功能,例如混合静态和服务器渲染,TypeScript支持,智能捆绑,路由预取等。 无需配置。
When you have an API with NodeJS and deploying with it in the production you can build and deploy without any issues. But, when it comes to the development environment you have to configure a proxy to redirect all the API calls from the Next.js App.
当您拥有带有NodeJS的API并在生产环境中进行部署时,就可以毫无问题地进行构建和部署。 但是,在开发环境方面,您必须配置代理以重定向Next.js应用程序中的所有API调用。
What is proxying
什么是代理
Example Project
示例项目
What is Custom Server
什么是自定义服务器
Proxy Setup
代理设置
How To Proxy to Multiple APIs
如何代理到多个API
Rewrite the Path URL
重写路径URL
Multiple app entries to one API endpoint
一个API端点有多个应用程序条目
Summary
摘要
Conclusion
结论
什么是代理 (What is proxying)
In general, A proxy or proxy server serves as a gateway between your app and the internet. It’s an intermediate server between client and servers by forwarding client requests to resources.
通常,代理服务器或代理服务器充当您的应用程序与Internet之间的网关。 通过将客户端请求转发到资源,它是客户端和服务器之间的中间服务器。
In Next.js, we often use this proxying in the development environment. Next.js is a React framework. If we look at the following diagram, app UI is running on port 3000, and the backend server is running on port 3080. All the calls start with /api will be redirected to the backend server and rest all fall back to the same port.
在Next.js中,我们经常在开发环境中使用此代理。 Next.js是一个React框架。 如果我们看下图,则应用程序UI在端口3000上运行,后端服务器在端口3080上运行。 以/ api开头的所有调用都将重定向到后端服务器,其余所有都回落到同一端口。
In subsequent sections, we will see how we can accomplish this and other options as well.
在随后的部分中,我们将看到我们如何也可以完成此操作和其他选择。
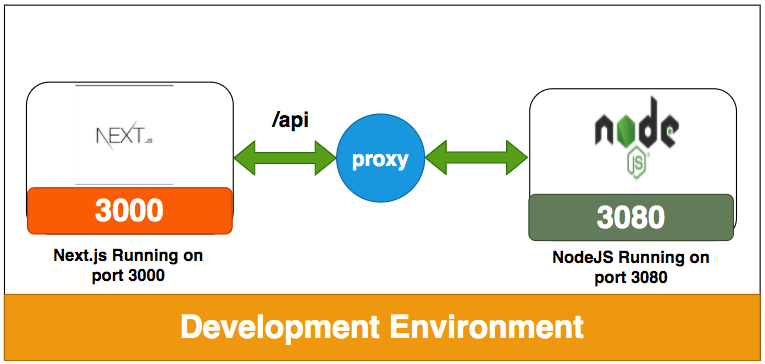
示例项目 (Example Project)
Here is an example project with the Github link you can clone it and try it on your machine.
这是带有Github链接的示例项目,您可以克隆它并在您的计算机上尝试。
// clone the project
git clone https://github.com/bbachi/nextjs-proxy-example.git// Run the API
cd api
npm install
npm run dev// Run the Next.JS App
cd todo-app
npm install
npm run dev
Once you install all the dependencies, you can start both the Next.js app and node js server on 3000 and 3080 respectively.
一旦安装了所有依赖项,就可以分别在3000和3080上启动Next.js应用程序和node js服务器。
You can start the Next.js app with these commands npm run dev
or next dev
and here is the Next.js app running on 3000.
您可以使用以下命令启动Next.js应用程序: npm run dev
或next dev
,这是在3000上运行的Next.js应用程序。
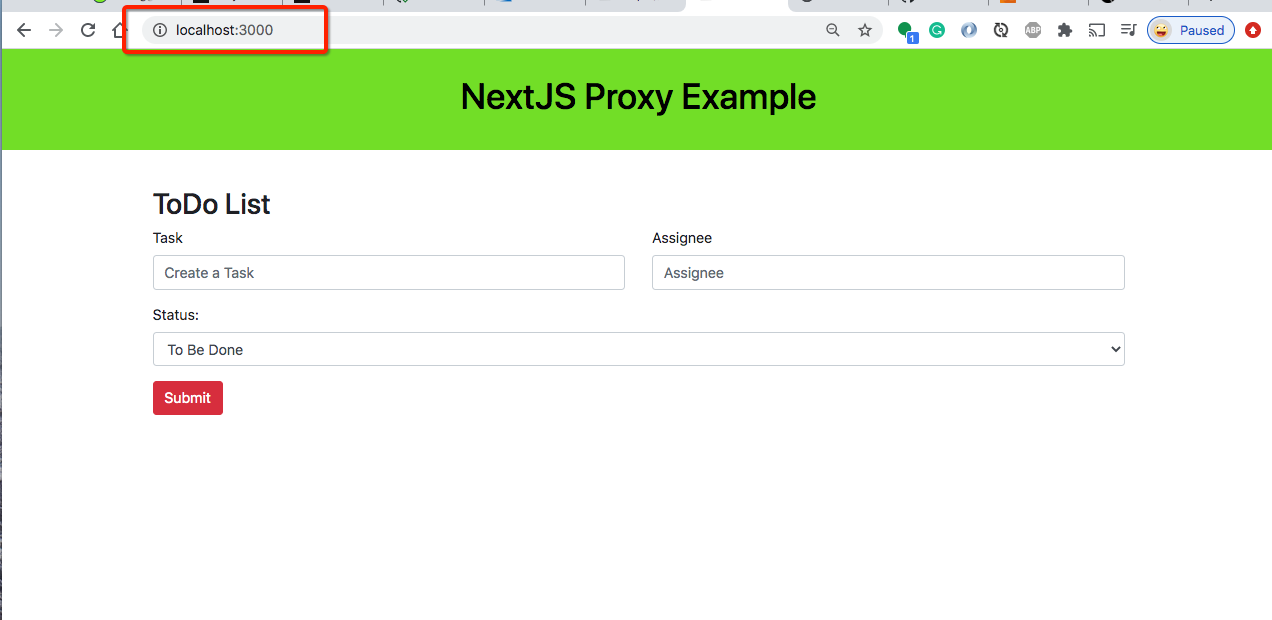
Let’s start the server with this command npm run dev
and test this API on port 3080.
让我们使用命令npm run dev
启动服务器,并在端口3080上测试此API。
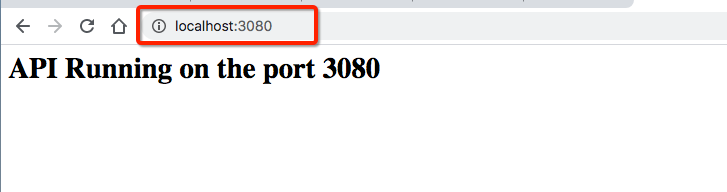
什么是自定义服务器 (What is Custom Server)
If you are familiar with the react library we can set up a proxy with the proxy object in the package.json like below.
如果您熟悉react库,我们可以使用package.json中的代理对象设置代理,如下所示。
"proxy": "http://localhost:3080"
Even though Next.js is React framework it doesn't work that way at the time of writing. Typically you start your next server with next start
. It's possible, however, to start a server 100% programmatically in order to use custom route patterns.
即使Next.js是React框架,在撰写本文时它也无法正常工作。 通常,您使用next start
下一个服务器。 但是,可以使用编程方式100%启动服务器以使用自定义路由模式。
Then, to run the custom server you’ll need to update the scripts
in package.json.
You can find more about this on their site.
然后,要运行自定义服务器,您需要更新package.json.
的scripts
package.json.
您可以在他们的网站上找到更多有关此的信息。
代理设置 (Proxy Setup)
We can set up a proxy with the help of a custom server. We need to install http-proxy-middleware
in the Next.js UI.
我们可以在自定义服务器的帮助下设置代理。 我们需要在Next.js UI中安装http-proxy-middleware
。
npm install http-proxy-middleware --save
Here is the custom server.js file where we are using http-proxy-middleware
to proxy all the calls with context path /api.
We are proxying only if it is a development environment and all other requests will be redirected to Next.js router.
这是我们使用http-proxy-middleware
的自定义server.js文件,用于使用上下文路径/api.
代理所有调用/api.
仅当它是开发环境并且所有其他请求将被重定向到Next.js路由器时,我们才进行代理。
Here is the package.json file of Next.js App and we are using server.js with the command npm run dev.
Here are the instructions to run the app in the local environment.
这是Next.js App的package.json文件,我们正在将server.js与命令npm run dev.
以下是在本地环境中运行该应用程序的说明。
// on Terminal 1cd todo-app
npm run dev// on Terminal 2cd api
npm start
Here is the App running on the port 3000 and all the API calls are proxying to the port 3080.
这是在端口3000上运行的应用程序,所有API调用都代理到端口3080。
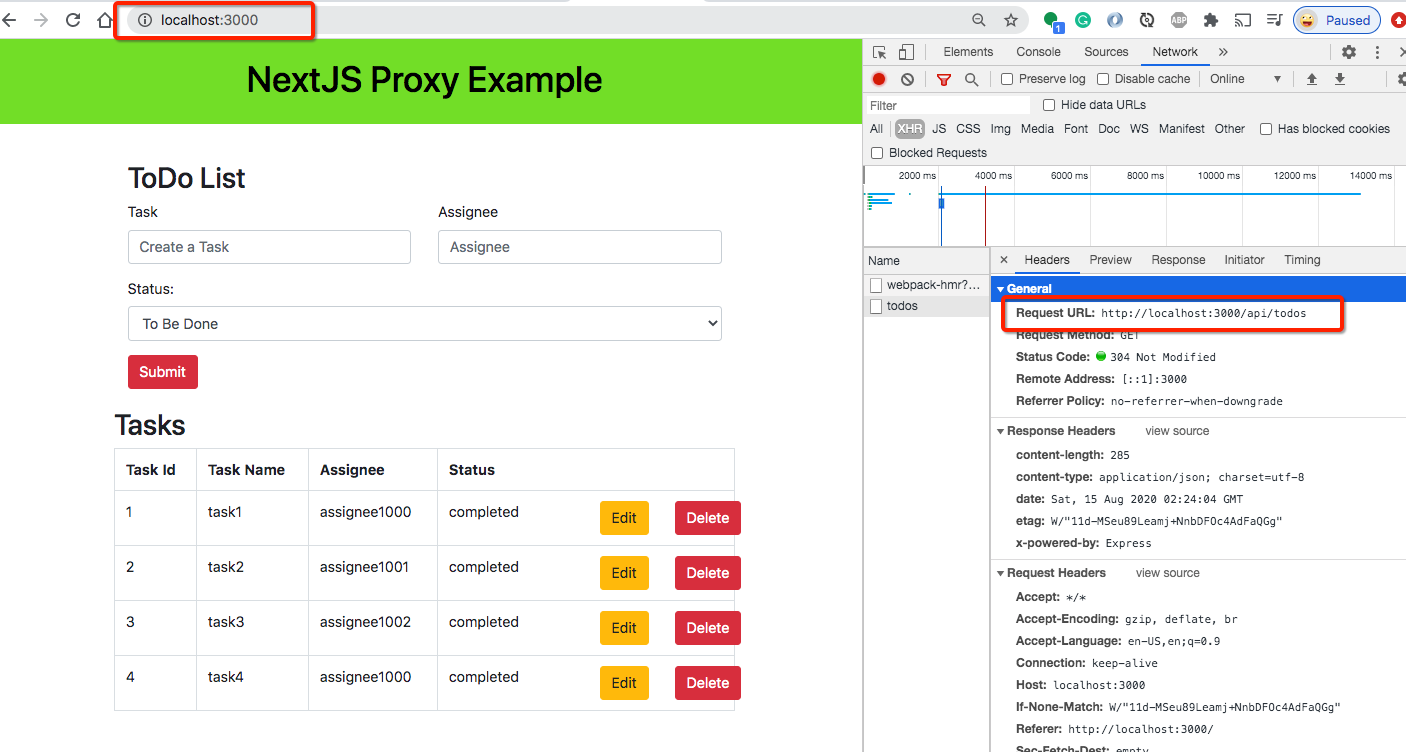
如何代理到多个API (How To Proxy to Multiple APIs)
We have seen how we can proxy into one API from your Next.js UI. This is not the case most of the time. Your Next.js app might need to call multiple APIs to get the data. Let’s see how we can do that.
我们已经了解了如何从您的Next.js UI代理到一个API。 大多数情况下并非如此。 您的Next.js应用程序可能需要调用多个API才能获取数据。 让我们看看我们如何做到这一点。
For example, if you look at the below diagram the Next.js UI is calling two APIs: api and api2. All the calls that start with /api should be redirected to User API and all the calls that start with the /api2 should be redirected to User API2.
例如,如果您查看下图,则Next.js UI会调用两个API: api和api2 。 以/ api开头的所有调用都应重定向到用户API ,以/ api2开头的所有调用都应重定向到User API2。
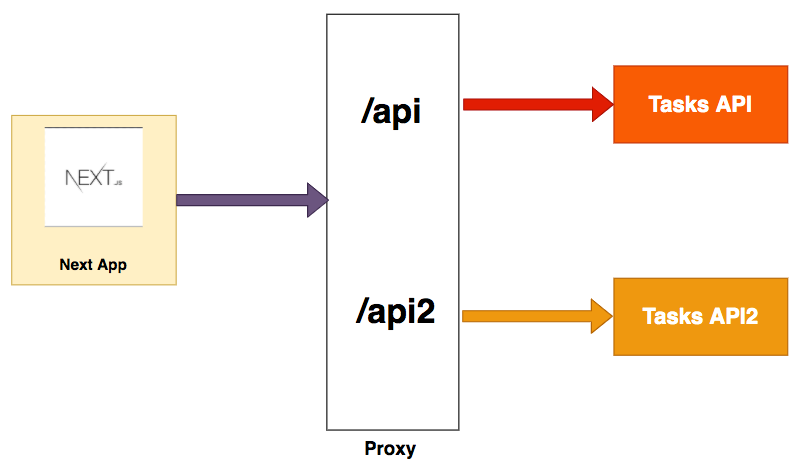
We can implement this scenario with the custom server. I have added one more API with the context path api2 and we need to add one more middleware entry as shown in the server.js file.
我们可以使用定制服务器来实现此方案。 我用上下文路径api2和添加了一个API 我们需要再添加一个中间件条目,如server.js文件中所示。
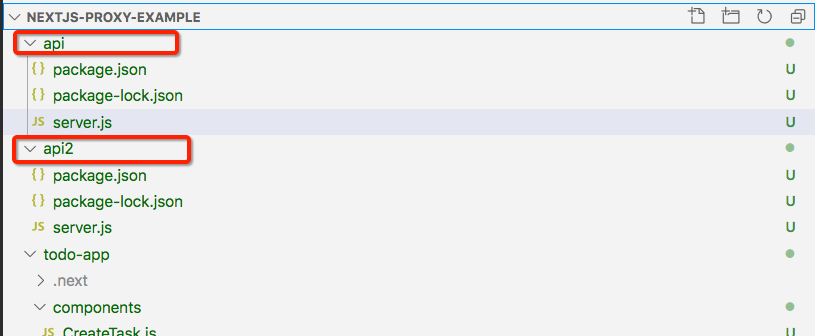
In the api2 API we have the same functionality but running on a different port 3081. Here is the server.js file from api2.
在api2 API中,我们具有相同的功能,但是在不同的端口3081上运行。这是api2的server.js文件。
Let’s test these two APIs from the Next.js port 3000.
让我们从Next.js端口3000测试这两个API。
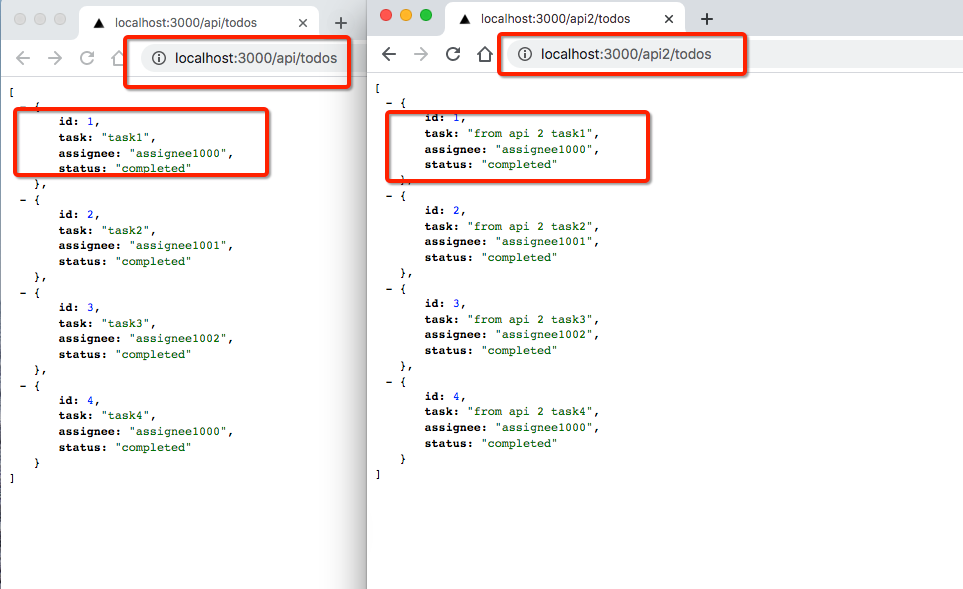
重写路径URL (Rewrite the Path URL)
Whenever there is a change in the URLs, we often rewrite the path of the backend server endpoints. We can do that with the pathRewrite.
每当URL发生更改时,我们通常都会重写后端服务器端点的路径。 我们可以使用pathRewrite.
做到这pathRewrite.
Let’s understand the pathRewrite option. For instance, our backend URL /api/todos
is changed to /api/tasks/todos
and we want to test in development before it goes to production. We can achieve this with the option pathRewrite like below.
让我们了解pathRewrite选项。 例如,我们的后端URL /api/todos
更改为/api/tasks/todos
,我们希望在投入生产之前进行开发测试。 我们可以使用如下所示的pathRewrite选项来实现。
一个API端点有多个应用程序条目 (Multiple app entries to one API endpoint)
Sometimes we have multiple modules with services in our app. We might have a scenario where multiple entries or services will call the same API endpoint.
有时我们的应用程序中有多个带有服务的模块。 我们可能会遇到多个条目或服务将调用同一API端点的情况。
For example, we have two paths that are needed to be redirected to the same endpoint (line 19). This is possible with the following setup.
例如,我们有两个路径需要重定向到同一端点(第19行)。 使用以下设置可以做到这一点。
Now if you hit both URLs in the browser http://localhost:3000/api/todos and http://localhost:3000/api2/todos both will be redirected to the same API.
现在,如果您在浏览器中同时点击了两个URL,则http:// localhost:3000 / api / todos和http:// localhost:3000 / api2 / todos都将被重定向到相同的API。
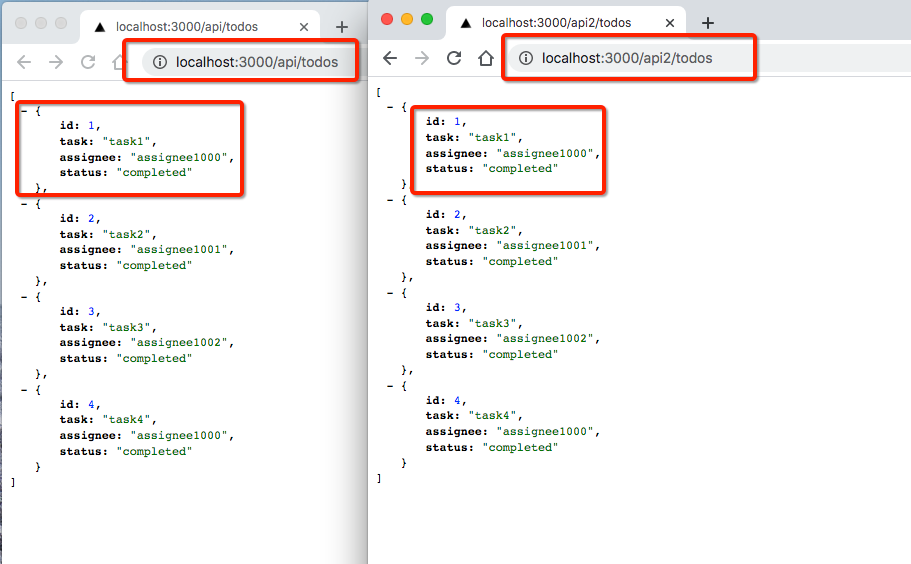
摘要 (Summary)
- In React, the create-react-app proxy is used mostly in the development environment to facilitate the communication between server and UI. 在React中,create-react-app代理主要用于开发环境中,以促进服务器和UI之间的通信。
- Even though Next.js is a react js framework, it doesn’t work that way. 即使Next.js是一个react js框架,它也无法正常工作。
- We need to set up a custom server manually in Next.js applications. Next.js doesn't recommend custom servers for production environments. 我们需要在Next.js应用程序中手动设置自定义服务器。 Next.js不建议在生产环境中使用自定义服务器。
- We need to have a backend server and UI running on different ports. 我们需要在不同端口上运行后端服务器和UI。
The proxy entry should be added to the server.js file that is redirected to API calls based on the URL.
代理条目应添加到基于URL重定向到API调用的server.js文件中。
- We need to make sure the Next.js App and Backends are running on different ports for successful communication. 我们需要确保Next.js应用程序和后端在不同的端口上运行,以便成功进行通信。
We need to install http-proxy-middleware for the customized setup.
我们需要为自定义设置安装http-proxy-middleware 。
We can rewrite the path with the option pathRewrite.
我们可以使用选项pathRewrite重写路径。
- We can proxy multiple entries to one backend API with it as well. 我们也可以将多个条目代理到一个后端API。
- We can proxy multiple entries to multiple backends as well with multiple entries in the middleware. 我们可以使用中间件中的多个条目将多个条目代理到多个后端。
结论 (Conclusion)
This is an excellent feature for the development phase. If you are working on the Next.js application, this feature really speeds up your development. You need to limit this feature only for development. Next.js doesn’t recommend this for production environments.
这是开发阶段的出色功能。 如果您正在使用Next.js应用程序,那么此功能确实可以加快开发速度。 您只需要为开发限制此功能。 Next.js不建议在生产环境中使用此功能。
翻译自: https://medium.com/bb-tutorials-and-thoughts/next-js-how-to-proxy-to-backend-server-987174737331
next.js