金山打字通英文课程带注释
In this article, we will discuss:
在本文中,我们将讨论:
- What are types 什么是类型
- Two systems to set types in typescript: annotations vs inference 在打字稿中设置类型的两种系统:注释与推理
- Usage of types for primitive values 基本值类型的用法
Types with functions: arrow functions,
function
keyword, anonymous functions具有函数的类型:箭头函数,
function
关键字,匿名函数- Why we should always use annotations to set the return type 为什么我们应该始终使用批注设置返回类型
- Types with objects: object destructuring, nested objects 带对象的类型:解构对象,嵌套对象
In typescript, any value —anything at all that can be saved to a variable, has a type.
在打字稿中,任何值(可以保存到变量的所有值)都具有类型。
In plain words, we can think of a type as a way to easily refer to the different properties and functions that a value has. When we think of a value having methods, first thing that comes to mind is a class or an object with custom methods in them. But remember, that even primitive types have many built-in methods associated with the type. For example, type of number
has toString()
method and type of string
has charAt()
method. When we use proper types for the values, typescript will also check for this built-in methods availability on a certain type.
简而言之,我们可以将类型视为轻松引用值所具有的不同属性和功能的一种方式。 当我们想到具有方法的值时,首先想到的是类或带有自定义方法的对象。 但是请记住,即使基本类型也具有与该类型关联的许多内置方法。 例如, number
类型具有toString()
方法,而string
类型具有charAt()
方法。 当我们使用适当的类型作为值时,typescript还将检查特定类型的此内置方法的可用性。
There are two different systems that are used by typescript to figure out the type of a value, type annotations and type inference, which work side by side.
打字稿使用两种不同的系统来计算值的类型, 类型 注释和类型 推断,它们可以并行工作。
In essence, when we use type annotations it means that the developer explicitly told the typescript what the type is. On the other hand, type inference means that the typescript “guessed” the type (derived it from the code around the value).
本质上,当我们使用类型注释时,这意味着开发人员明确地告诉打字稿类型是什么。 另一方面,类型推断意味着打字稿“猜测”了该类型(从值周围的代码中得出)。
使用具有原始值的类型 (Using types with primitive values)
In most cases, it is totally fine to rely on inference system for primitive types. For example, this code will result in the same exact error in both cases:
在大多数情况下,对于原始类型依赖推理系统是完全可以的。 例如,在两种情况下,此代码将导致相同的确切错误:
let apples: number = 5;let count = 7;// Type '"plenty"' is not assignable to type 'number'
// Type '"a lot"' is not assignable to type 'number'apples = 'a lot';count = 'plenty';
However, there are three cases when we are better off using type annotations and setting the types explicitly. Let’s go over them one by one:
但是,在三种情况下,最好使用类型注释并显式设置类型。 让我们一一介绍它们:
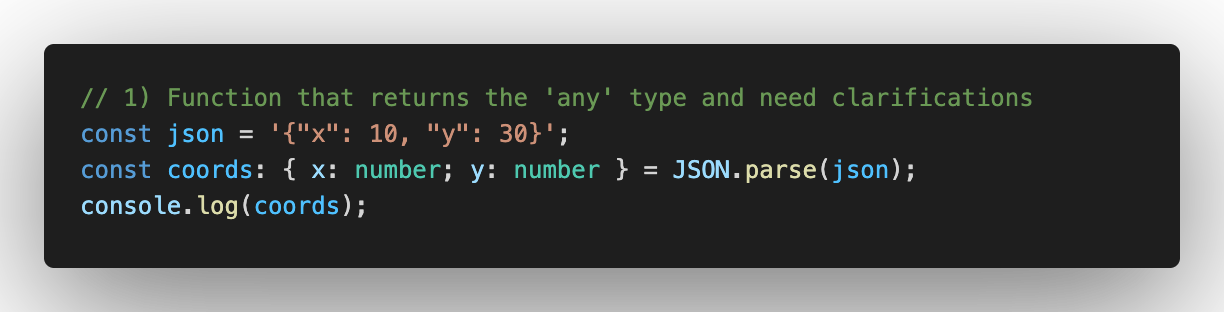
The first case is when the function returns type any
and we want to clarify it. In this situation JSON.parse
returns valid JS from a string. It can be any number of things, depending on the passed string. Here we use type annotation to set explicitly that we expect coords
value to be an object with x
and y
properties; both are a number
.
第一种情况是当函数返回类型any
,我们想对其进行澄清。 在这种情况下, JSON.parse
从字符串返回有效的JS。 它可以是任意数量的东西,具体取决于传递的字符串。 在这里,我们使用类型注释来明确设置期望coords
值是具有x
和y
属性的对象。 两者都是number
。
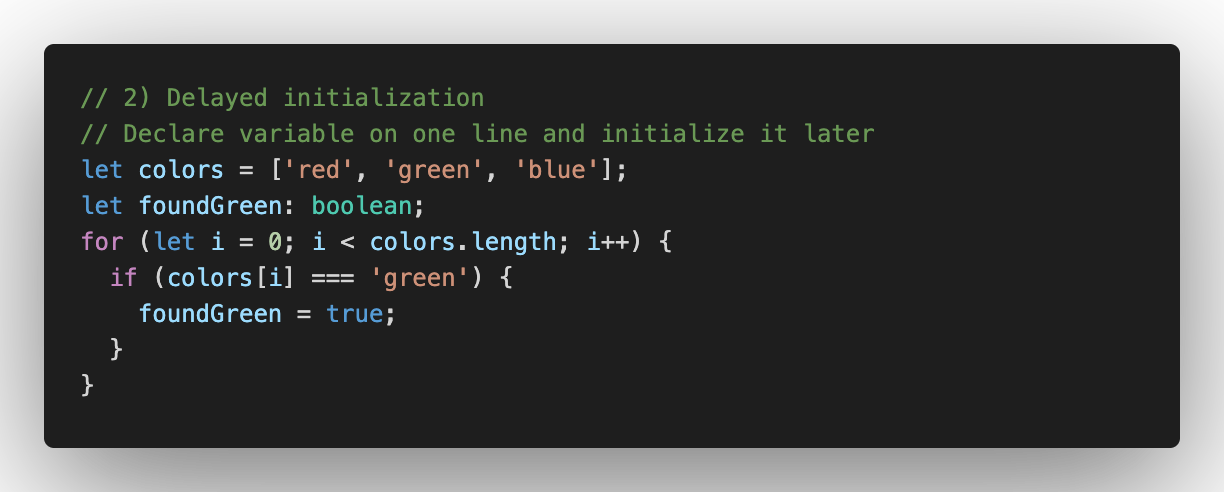
Granted, this is a bit of a strange example; and in reality, we would possibly initialize foundGreen
to false
or, even better, use includes()
array method. However, if for any reason we need to declare a variable before initializing it, we have to use type annotations to specify the type.
当然,这是一个奇怪的例子。 实际上,我们可能foundGreen
初始化为false
或者甚至更好地使用includes()
数组方法。 但是,如果由于某种原因需要在初始化变量之前声明一个变量,则必须使用类型注释来指定类型。
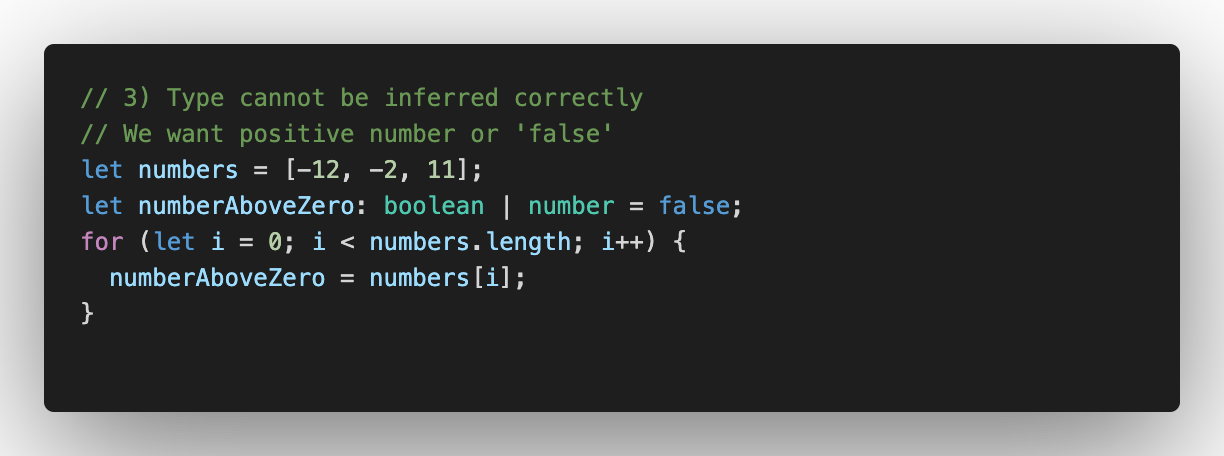
In this example, we want to have a variable with a positive number or false
. If we intend for a variable to change type — we have to specify all of the possible types ahead of time.
在此示例中,我们想要一个具有正数或false
的变量。 如果我们打算使用变量来更改类型-我们必须提前指定所有可能的类型。
To sum it up, for primitive values we will rely on type inference as much as we can, and use type annotations for the three cases described above.
综上所述,对于原始值,我们将尽可能地依赖类型推断,并在上述三种情况下使用类型标注。
使用带功能的类型 (Using types with functions)
It is pretty straightforward with primitive types, but it gets a bit more complex with functions. With functions, we want to specify types not only for arguments, but also for a return value. Besides regular numbers and strings, a function can implicitly return undefined
or have no return value at all, but throw an error instead.
对于原始类型来说,这非常简单,但是对于函数而言,它却变得更加复杂。 对于函数,我们不仅要为参数指定类型,还要为返回值指定类型。 除了常规数字和字符串,函数还可以隐式返回undefined
或根本没有返回值,但是会引发错误。
Type annotations will be exactly the same for arrow functions, regular functions declared with function
keyword and for anonymous functions.
对于箭头函数,使用function
关键字声明的常规函数以及对于匿名函数,类型注释将完全相同。
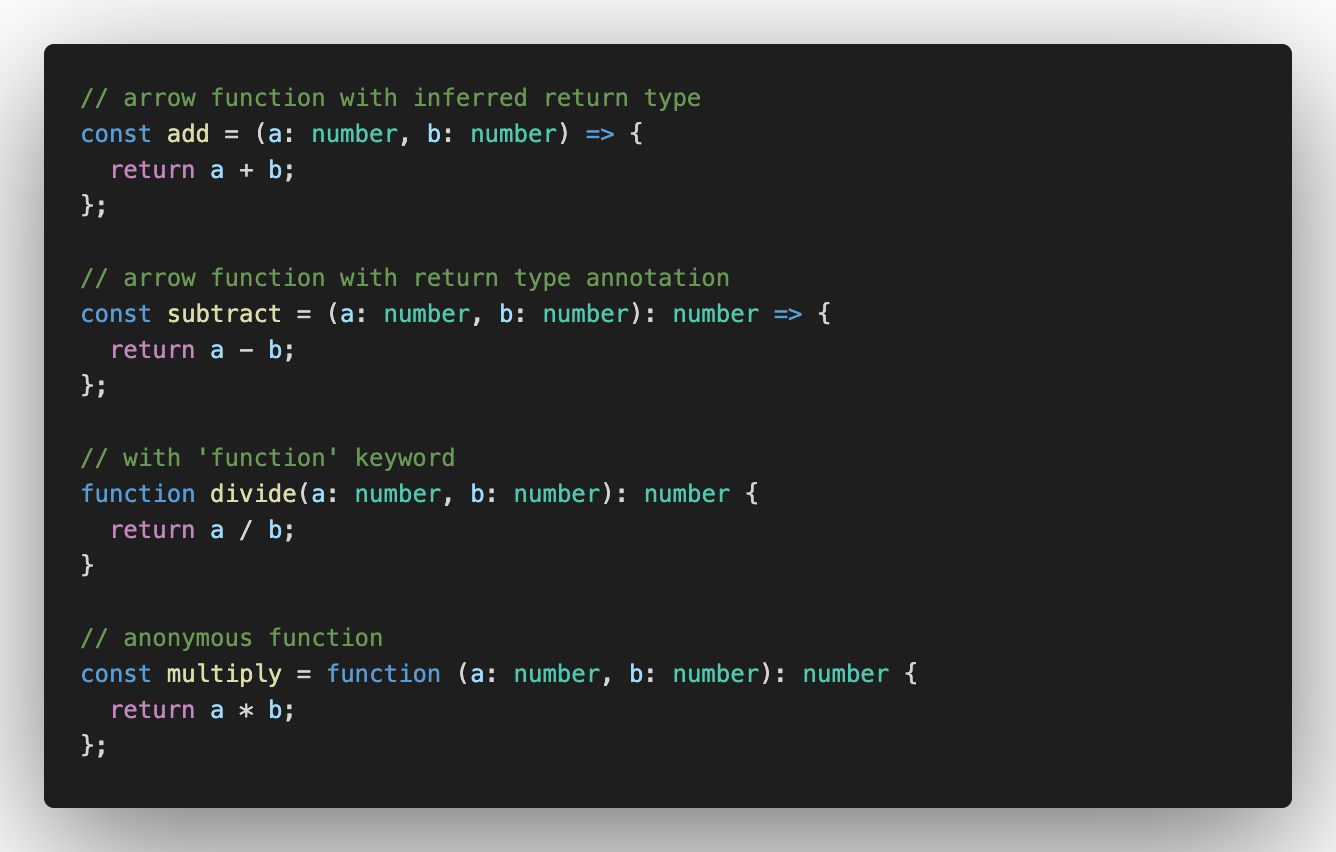
One thing I want to point out specifically is that we should always explicitly set the return type, even if it works perfectly with inferred type. The reason for it is that otherwise, if the developer forgets by mistake to return value from a function, typescript will just infer the return type to be void
and won’t mark it as an error.
我想特别指出的一件事是,即使返回类型可以完美地与推断类型配合使用,我们也应该始终明确地设置返回类型。 这样做的原因是,否则,如果开发人员错误地忘记了从函数返回值,则打字稿只会推断出返回类型为void
并且不会将其标记为错误。
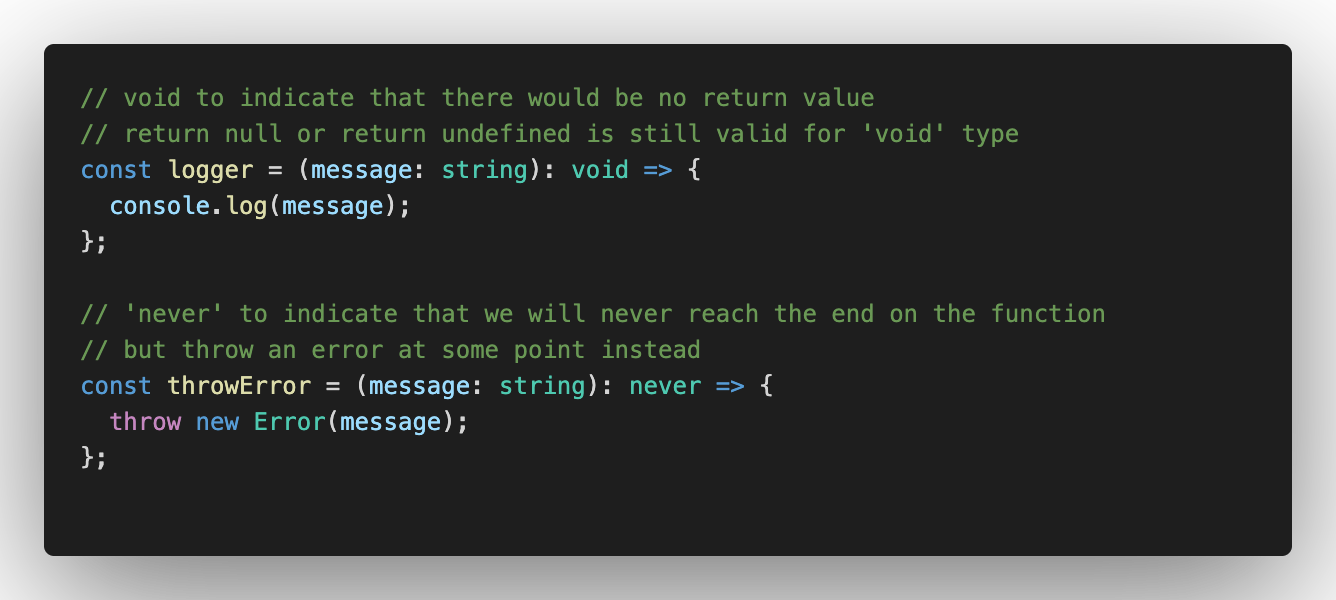
For functions that do not have a return value, there is a special return type called void
. Void indicates that a function is not intended to have a return value. As we know, functions without return
keyword implicitly return undefined
in JavaScript. So having return undefined
or return null
will still count as void
return type.
对于没有返回值的函数,有一个特殊的返回类型称为void
。 无效表示函数不打算具有返回值。 众所周知,没有return
关键字的函数在JavaScript中隐式返回undefined
。 因此, return undefined
或return null
仍将算作void
返回类型。
Here is an example of how to use arguments destructuring with functions in typescript.
这是一个如何在TypeScript中对函数进行参数分解的示例。
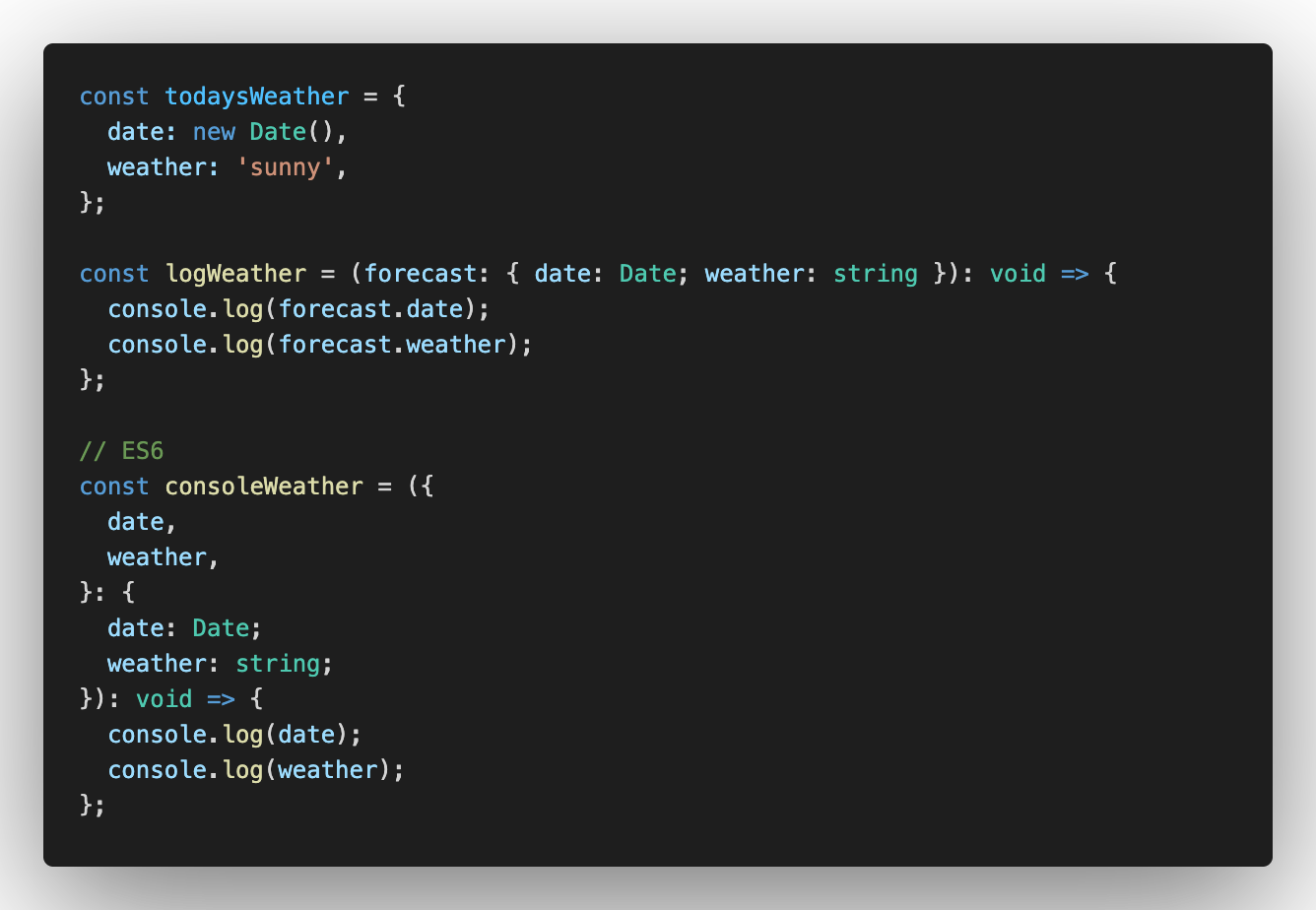
The sintax will take a bit of getting used to in this case, so let’s go over what is happening step by step.
在这种情况下,sintax将需要一点时间来适应,所以让我们逐步研究一下正在发生的事情。
logWeather
function receives an object as an argument and we use type annotation that looks practically identical to object literal in JavaScript. Note that we use semicolon to separate properties instead of a comma here. In this example, we say that logWeather
receives an object that has property date
of type Date
and property weather
of type string
, and returns void
logWeather
函数将对象作为参数接收,我们使用的类型注释实际上与JavaScript中的对象文字相同。 请注意,我们在这里使用分号来分隔属性,而不是逗号。 在此示例中,我们说logWeather
接收到一个对象,该对象具有Date
类型的属性date
和string
类型的属性weather
,并返回void
In the case with consoleWeather
function, we go one step further and use ES6 argument destructuring. Note that we do not attempt to specify types in the destructured object itself. The syntax instead is to have a colon separated type annotation right after the destructuring. We use semicolon to separate properties inside of the type annotation.
在使用consoleWeather
函数的情况下,我们更进一步,并使用ES6参数解构。 注意,我们不尝试在解构对象本身中指定类型。 相反,语法是在解构之后立即使用冒号分隔的类型注释。 我们使用分号在类型注释中分隔属性。
To sum it up, for functions we will use annotations in all cases.
综上所述,对于函数,我们将在所有情况下都使用注释。
带对象文字的类型 (Types with object literals)
Finally, let’s take a look at using type annotations with objects and nested objects.
最后,让我们看一下对对象和嵌套对象使用类型注释。
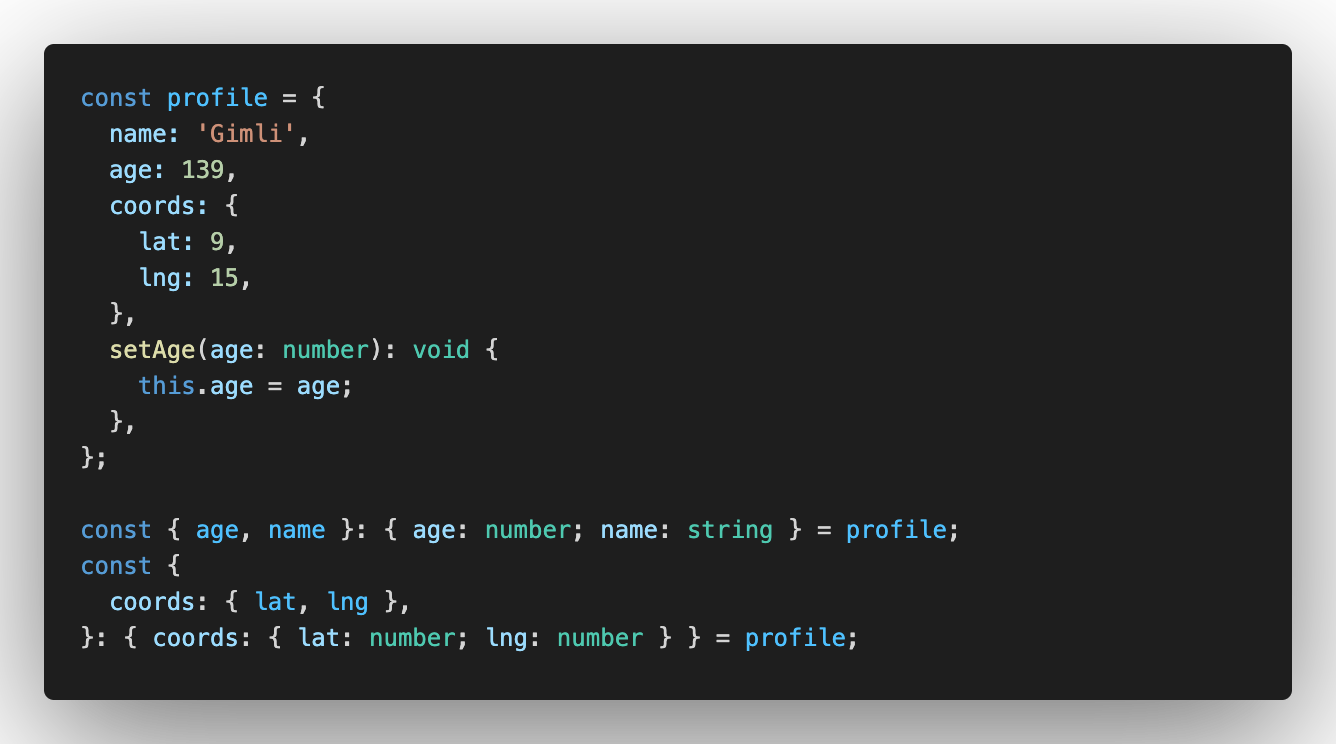
In this example, when destructuring { age, name }
we see the same familiar syntax as was used while destructuring an argument to a function. The idea behind this syntax becomes more clear when we have to deal with destructuring nested objects. The syntax looks hard, but in reality we do the same thing as we would do in plain JavaScript, then put a colon and define a “type object” to describe the structure of the destructured argument. Once again, remember to separate properties in the object’s type annotation with semicolons instead of regular commas.
在此示例中,当解构{ age, name }
我们看到的语法与解构函数自变量时使用的语法相同。 当我们必须处理解构嵌套对象时,此语法背后的想法变得更加清晰。 语法看起来很困难,但实际上,我们做的事情与普通JavaScript相同,然后放了一个冒号并定义了一个“类型对象”来描述结构化参数的结构。 再一次,请记住用分号(而不是常规逗号)分隔对象类型注释中的属性。
This concludes this quick guide to setting simple types in typescript. The typescript is a powerful addition to JavaScript world and is growing in popularity all the time. Of course, there is a lot more to it than setting the types that we discussed here. There are interfaces, unions and many other cases, but one thing at a time.
到此结束本快速指南,以设置打字稿中的简单类型。 打字稿是JavaScript世界的有力补充,并且一直在不断普及。 当然,除了设置我们在此讨论的类型之外,还有很多其他功能。 有接口,联合和许多其他情况,但一次只能做一件事。
In this article we examined :
在本文中,我们研究了:
- The difference between type annotations and inferred types in typescript 类型注释和打字稿中的推断类型之间的区别
- When to rely on inferred type system and when to use annotations 何时依赖推断类型系统以及何时使用批注
- Types for arrow, regular and anonymous functions 箭头,常规和匿名函数的类型
- Types with object literals and in object destructuring 具有对象文字和对象分解的类型
Thank you for reading and I hope it clarifies the concept of types a bit further for you!
感谢您的阅读,我希望它为您进一步阐明类型的概念!
Build readable and scalable apps with typescript, have fun and, as always, Happy Coding!
使用打字稿构建可读性强且可扩展的应用程序,尽其所能,尽情享受快乐编码!
金山打字通英文课程带注释