rad xe 5 使用教程
镭能解决什么问题? (What problem does Radium solve?)
React offers several options when it comes to styling your components. We can always put our styles in a .css file and import it but I’m a big fan of taking advantage of the mental model React offers with JSX by coupling JavaScript, HTML, and styling together in one place. It does come with certain limitations though. There are some common CSS features and techniques that inline styles don’t easily accommodate: media queries, browser states (:hover, :focus, :active) and modifiers.
在设计组件样式时, R eact提供了多种选择。 我们总是可以将样式放在.css文件中,然后将其导入,但是我非常喜欢通过在一个地方将JavaScript,HTML和样式耦合在一起来利用React通过JSX提供的思维模型。 但是它确实有某些限制。 内联样式无法轻松适应一些常见CSS功能和技术:媒体查询,浏览器状态(:hover,:focus,:active)和修饰符。
Let’s consider the following problem — by default we can’t assign a hover style for our button short of using a .css file (button:hover). Hover is not a css property and we cannot change its value on our style object. As we mentioned before, we could simply work around this by styling the button in the .css file the drawback being that the style would not be scoped to this component only, and all the buttons in our application would get the styling unless we added an id or a class. Personally, I don’t like having to jump to the .css file to set styles I will only be used in one component, and inline styling just makes more sense.
让我们考虑以下问题-默认情况下,除非使用.css文件(button:hover),否则我们无法为按钮分配悬停样式。 悬停不是css属性,我们无法在样式对象上更改其值。 如前所述,我们可以通过对.css文件中的按钮进行样式化来解决此问题,缺点是样式将不仅仅局限于该组件,并且除非我们添加了一个按钮,否则应用程序中的所有按钮都将获得样式化id或类。 就我个人而言,我不喜欢跳到.css文件来设置仅在一个组件中使用的样式,并且内联样式更有意义。
镭到底是什么? (What exactly is Radium?)
I encourage you to read the official docs here to find out more. In short, Radium is a third-party package that offers a standard interface and abstractions for dealing with the previously mentioned limitations.
我鼓励您在这里阅读官方文档以了解更多信息。 简而言之,Radium是第三方程序包,它提供了用于处理前面提到的限制的标准接口和抽象。
它具有以下功能: (It offers the following features:)
- A conceptually simple extension of normal inline styles 常规内联样式的概念上简单的扩展
Browser state styles to support
:hover
,:focus
, and:active
支持
:hover
,:focus
和:active
浏览器状态样式- Media queries 媒体查询
- Automatic vendor prefixing 供应商自动前缀
- Keyframes animation helper 关键帧动画助手
ES6 class and
createClass
supportES6类和
createClass
支持
It first needs to be installed and added to the project first before we can use it.
首先,需要先安装它并将其添加到项目中,然后才能使用它。
npm install --save radium
I like to always add –save to make sure the package is saved in my package.json. Once it is installed, it needs to be imported in the component where we intend to use it.
我总是喜欢添加–save以确保将包保存在我的package.json中。 安装后,需要将其导入我们打算使用它的组件中。
import React, from 'react'
import Radium from 'radium'
import Puppy from "./Puppy"
Now with that imported, you can scroll all the way down where you export your app and there you can call radium as a function and wrap your app with it.
现在,在导入了该文件之后,您可以一直向下滚动到导出应用程序的位置,然后可以在其中调用镭作为函数并将其包装到应用程序中。
export default Radium(App)
What’s happening here is we are wrapping our App component in a higher-order component (sometimes referred to as HOC), injecting extra functionality, in this case, the extra syntax which will parse our styles and extra features and can be used both with class components and functional components.
这里发生的是我们将App组件包装在一个高阶组件(有时称为HOC)中,注入了额外的功能,在这种情况下,额外的语法将解析我们的样式和额外的功能,并且可以与class一起使用组件和功能组件。
Please note it is important to wrap the component correctly if you are using any additional libraries. For example, this is the correct way to wrap our App component when using ‘react-redux’ connect method:
请注意,如果使用任何其他库,正确包装组件很重要。 例如,这是使用'react-redux' 连接 方法时包装App组件的正确方法:
export default connect(mapSTP, mapDTP)(Radium(App))
On the other hand, the following will produce an error:
另一方面,以下内容将产生错误:
export default Radium(connect(mapSTP, mapDTP)Radium(App))
Now that we have Radium correctly added to our application, we can start adding or using new features. Let’s create a style object which will eventually be assigned to a button. Let’s add a hover style which unlike the regular CSS selectors needs to be wrapped in quotation mark — “:hover” — because it starts with a colon and is not a valid JavaScript property name.
现在我们已将Radium正确添加到我们的应用程序中,我们可以开始添加或使用新功能。 让我们创建一个样式对象,该对象最终将分配给按钮。 让我们添加一个悬浮样式,该样式不同于常规CSS选择器需要用引号引起来:“:hover”,因为它以冒号开头,并且不是有效JavaScript属性名称。
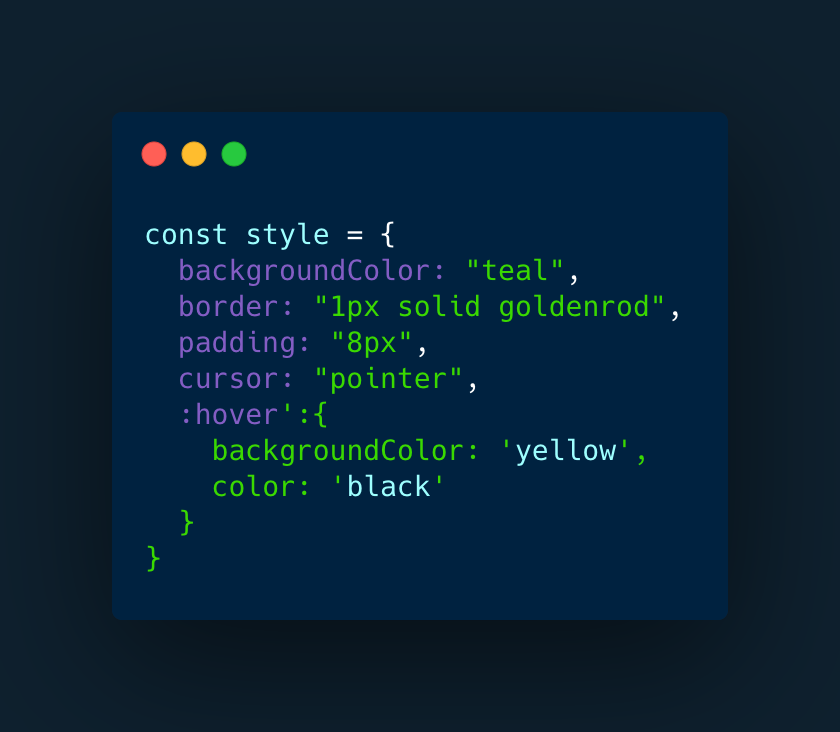
We assign another object with a set of styles to the hover state where the background will change its color to yellow when we hover over the button.
我们将另一个具有一组样式的对象分配给鼠标悬停状态,当我们将鼠标悬停在按钮上时,背景颜色会变为黄色。
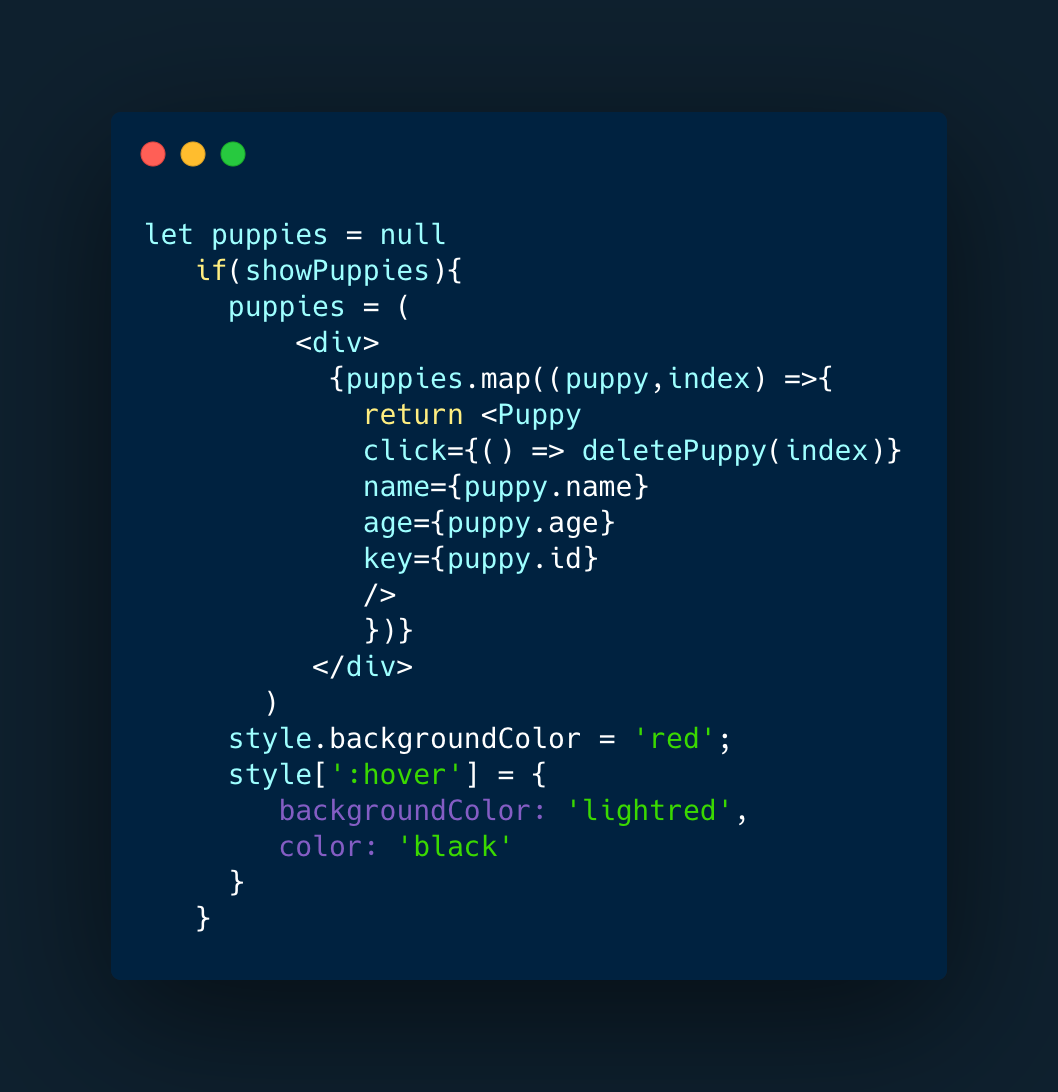
Here we are assigning a new value, a new javascript object where we use light red and black text taking advantage of another radium feature.
在这里,我们分配了一个新值,一个新的javascript对象,在其中我们利用浅红色和黑色文本来利用另一个镭功能。
用Radium进行媒体查询 (Media queries with Radium)
We’ve added radium and used it to dynamically change our button and add the hover pseudo-selector. We can also use radium to apply media queries. Let’s do this in a Puppy component instead of the default way of defining it in the .css file
我们添加了镭,并使用它来动态更改按钮并添加悬停伪选择器。 我们还可以使用镭来进行媒体查询 。 让我们在Puppy组件中执行此操作,而不是在.css文件中定义它的默认方法
// in Puppy.css
@media (min-width: 500px) {
.Puppy{
width: '450px'
}
}
We could definitely do that but taking advantage of Radium to include our media queries in the component file allows us to scope it to a component as well as change it dynamically. For that, let’s add a new style variable where we will define our media queries using ‘@media’ selector and define the styles as a javascript object:
我们绝对可以做到这一点,但是利用Radium将媒体查询包括在组件文件中,可以使我们将其范围限定为组件并动态更改。 为此,我们添加一个新的样式变量,在该变量中,我们将使用“ @media”选择器定义媒体查询,并将样式定义为javascript对象:
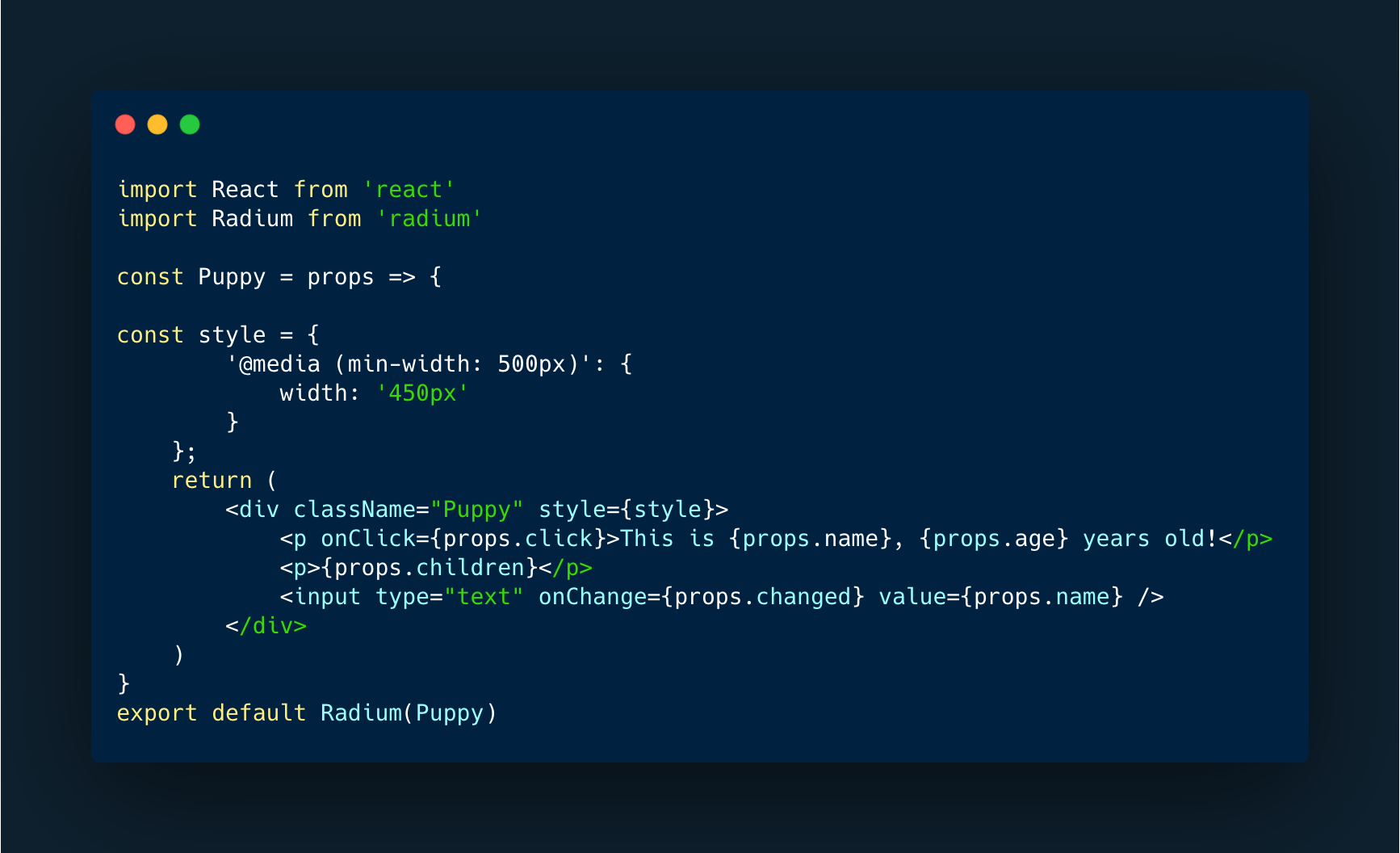
Since it’s a string, it’s a valid name, and radium will parse it and understand it.
因为它是一个字符串,所以它是一个有效的名称,镭会解析它并理解它。
We have defined but not yet assigned our style in our component. Please note the style defined locally will overwrite class settings if there are any, not because of Radium but because of CSS specificity rules.
我们已经定义了但尚未在我们的组件中分配样式。 请注意,本地定义的样式(如果有的话)将覆盖类设置,这不是因为Radium,而是因为CSS特殊性规则 。
It might look like we’re all set but if we reload the application now, we will get an error. While wrapping the application in Radium is enough to use the package for pseudo-selectors, it is not enough for transforming selectors such as keyframes and media queries. We need to also wrap everything in the return statement in the component where the style is applied with another HOC (higher-order component) provided by Radium called <StyleRoot>. We also need to import it as a named export from Radium.
看起来我们已经准备就绪,但是如果现在重新加载应用程序,将会收到错误消息。 虽然将应用程序包装在Radium中足以将程序包用于伪选择器,但不足以转换 诸如关键帧和媒体查询之类的 选择器 。 我们还需要将所有内容包装在组件中的return语句中,在组件中,样式由Radium提供的另一个名为<StyleRoot>的 HOC(高阶组件)应用。 我们还需要将其作为从Radium的命名导出导入。
import Radium, { StyleRoot } from 'radium'
Now we can simply wrap our whole application with it in our return statement. Here is how our entire App.js file might look after applying Radium to
现在,我们可以在return语句中简单地包装整个应用程序。 这是将Radium应用于以下示例后整个App.js文件的外观
Which will then pass on the props to our Puppy component
然后将道具传递给我们的Puppy组件
It will now work properly and we can check the desired effect by resizing the window.
现在它将正常工作,我们可以通过调整窗口大小来检查所需的效果。
把它们加起来 (To sum it up)
Using radium is one way of getting the best of both worlds — scoped styles and advanced CSS features like pseudos selectors and media queries.
使用镭是获得两全其美的一种方法-作用域样式和高级CSS功能(如伪选择器和媒体查询)。
翻译自: https://medium.com/swlh/make-your-react-app-responsive-with-radium-d66898cd0ee2
rad xe 5 使用教程