matlab 交叉口仿真
This post aims at explaining intersection observer API with examples so that you gain an understanding on how to use them in production sites. I recently started looking into this amazing API and came across some really good resources. One of them being Kevin Powell’s, and this article is heavily inspired by his videos.
这篇文章旨在通过示例解释路口观察器API,以便您了解如何在生产现场使用它们。 我最近开始研究这个惊人的API,并遇到了一些非常好的资源。 其中之一就是凯文·鲍威尔 ( Kevin Powell )的作品,这篇文章的灵感很大程度上来自他的视频。
So we will basically get started by an introduction to the concept and finish it off with some examples.
因此,我们基本上将从概念的入门入手,并通过一些示例结束它。
那么什么是路口观察员 (So what is Intersection Observer)
Intersection Observer API provides an easy way to watch and register callbacks to trigger when elements on a page come into view.
Intersection Observer API提供了一种简便的方法来观察和注册回调,以在页面上的元素进入视图时触发。
We simply create an intersection observer object like:
我们只需创建一个相交观察器对象,如:
let observer = new IntersectionObserver(callback, options);
let observer = new IntersectionObserver(callback, options);
The IntersectionObserver
object’s constructor takes two parameters. The first one is a callback function that’s executed once the observer notices an intersection and has asynchronously delivered some data about that intersection.
IntersectionObserver
对象的构造函数采用两个参数 。 第一个是回调函数,一旦观察者注意到路口并已异步传递有关该路口的一些数据,该回调函数便会执行。
The second (optional) parameter is options, an object with information to define what’s going to be the “intersection” . We may not want to know when an element is about to come into view, but only when it’s fully visible. Something like that is defined through the options parameter.
第二个(可选)参数是options ,一个具有信息的对象,用于定义将要成为“交集”的信息。 我们可能不希望知道元素何时会出现,而仅在完全可见时才知道。 像这样的东西是通过options参数定义的。
options has three properties:
选项具有三个属性:
root — The ancestor element/viewport that the observed element will intersect.
root —观察元素将相交的祖先元素/视口。
rootMargin — A string with values in the same format as for a CSS margin or padding value. For example: ‘3rem 2rem’. This creates a margin of the specified size around the root element, to effectively create an inset or an outset for the intersection point. It defaults to ‘0px’.
rootMargin —一个字符串,其值的格式与CSS边距或填充值的格式相同。 例如:“ 3rem 2rem”。 这将在根元素周围创建指定大小的边距,以有效地为相交点创建插入点或起点。 默认为“ 0px”。
threshold — An array of values (between 0 and 1.0), each representing the distance an element has intersected into or crossed over in the root at which the callback is to be triggered. If the callback is 0.5, the callback is triggered when the element is in or passes its half-visible threshold.
阈值 — 值的数组(0到1.0之间),每个值表示元素在触发回调的根中相交或相交的距离。 如果回调为0.5,则在元素处于或超过其半可见阈值时触发回调。
导航栏背景更改 (Navbar background change)
Quite often when working on sites we have navigation bars which are transparent to begin with but as we scroll past a certain height we want them to have a suitable background and contrast according to the page. You can notice the problem in the below demo. As soon as we reach the Random columns section, the background seems to blend with the section’s making it difficult to read its content.
通常,在网站上工作时,导航栏一开始都是透明的,但是当滚动到一定高度时,我们希望它们具有适合页面的背景和对比度。 您可以在下面的演示中注意到该问题。 当我们到达“ 随机列”部分时,背景似乎与该部分的内容融为一体,使得难以阅读其内容。
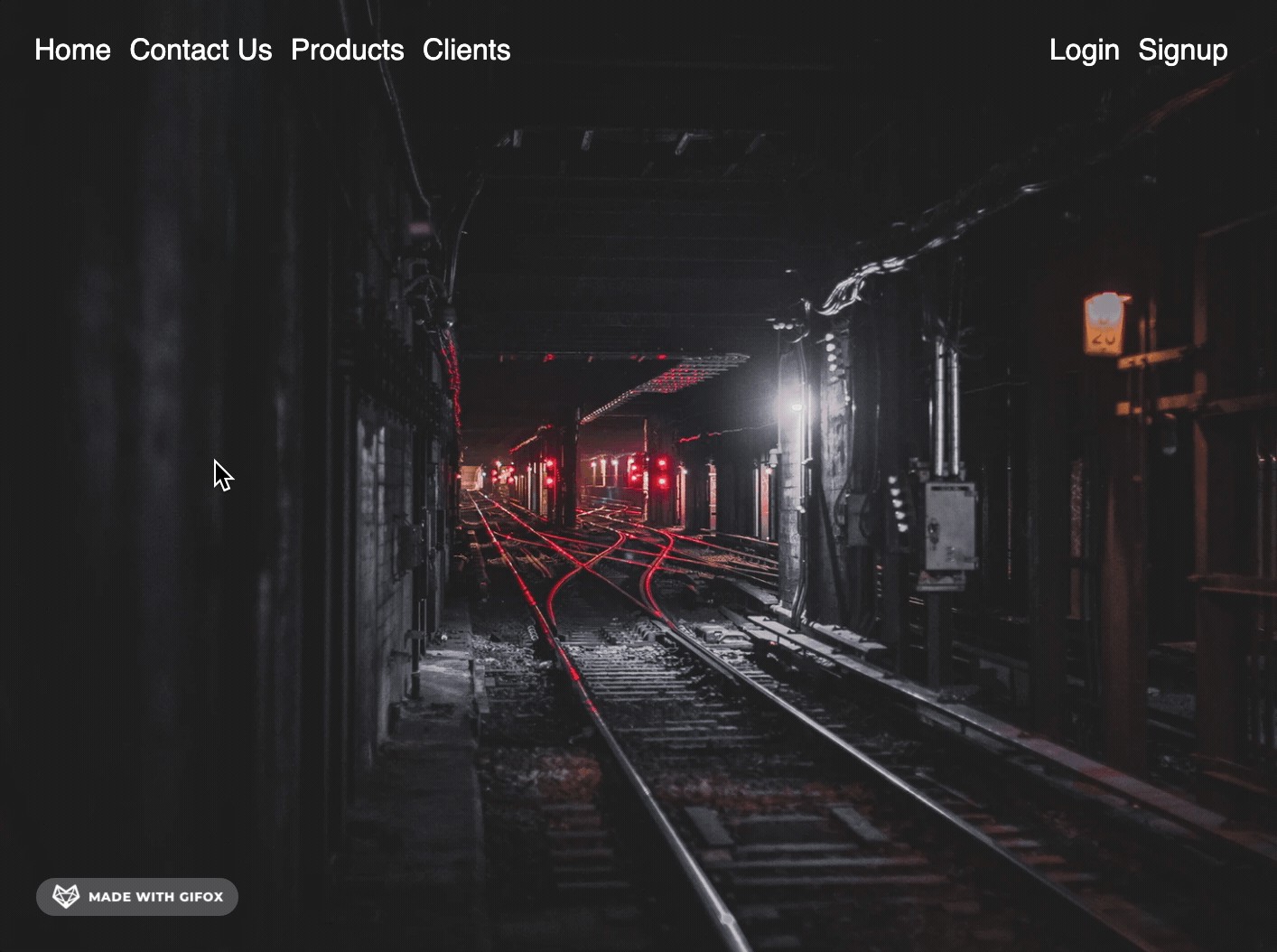
We can make use of intersection observer here to make the navbar’s background more intuitive.
我们可以在此处使用相交观察器,以使导航栏的背景更加直观。
Let’s grab the header and the top-container elements first:
让我们首先获取header和top-container元素:
const header = document.querySelector(“header”);
const topContainer = document.querySelector(“.top-container”);
Here top container is the parent or the ancestor element to which the intersection will trigger. We will also define a threshold in the options to allow the header to get the background style just before the header leaves the parent element.
在这里,顶层容器是相交将触发到的父元素或祖先元素 。 我们还将在选项中定义一个阈值,以允许标题在标题离开父元素之前获得背景样式。
const topContainerOptions = { threshold: 0.1 };
const topContainerOptions = { threshold: 0.1 };
Creating the intersection observer:
创建相交观察器:
const topContainerObserver = new IntersectionObserver(function(entries) {
entries.forEach(entry => {
// code to add/remove background from header
});
},
topContainerOptions);
The observer accepts entries in the fallback and we simply loop over them. The list of entries received by the callback includes one entry for each target which reporting a change in its intersection status.
观察者在回退中接受条目,我们仅在它们上循环。 回调收到的条目列表包括每个目标的一个条目,这些条目报告其交集状态发生变化。
Inside of the loop by checking the isIntersecting property on the entry object, we can accomplish the required behavior.
通过检查入口对象的isIntersecting属性,可以在循环内部完成所需的行为。
const topContainerObserver = new IntersectionObserver(function(entries) {
entries.forEach(entry => {
if (!entry.isIntersecting) {
header.classList.add("nav-scrolled");
} else {
header.classList.remove("nav-scrolled");
}
});
},
topContainerOptions);
If the entry found is not intersecting, simply add the nav-scrolled
class otherwise remove it. This class just adds background styling to the header.
如果找到的条目不相交,则只需添加nav-scrolled
类,否则将其删除。 此类仅向标题添加背景样式。
.nav-scrolled {
background: #999;
z-index: 2;
}
Finally observing the target element:
最后观察目标元素:
topContainerObserver.observe(topContainer);
topContainerObserver.observe(topContainer);
Here’s the final output:
这是最终的输出:
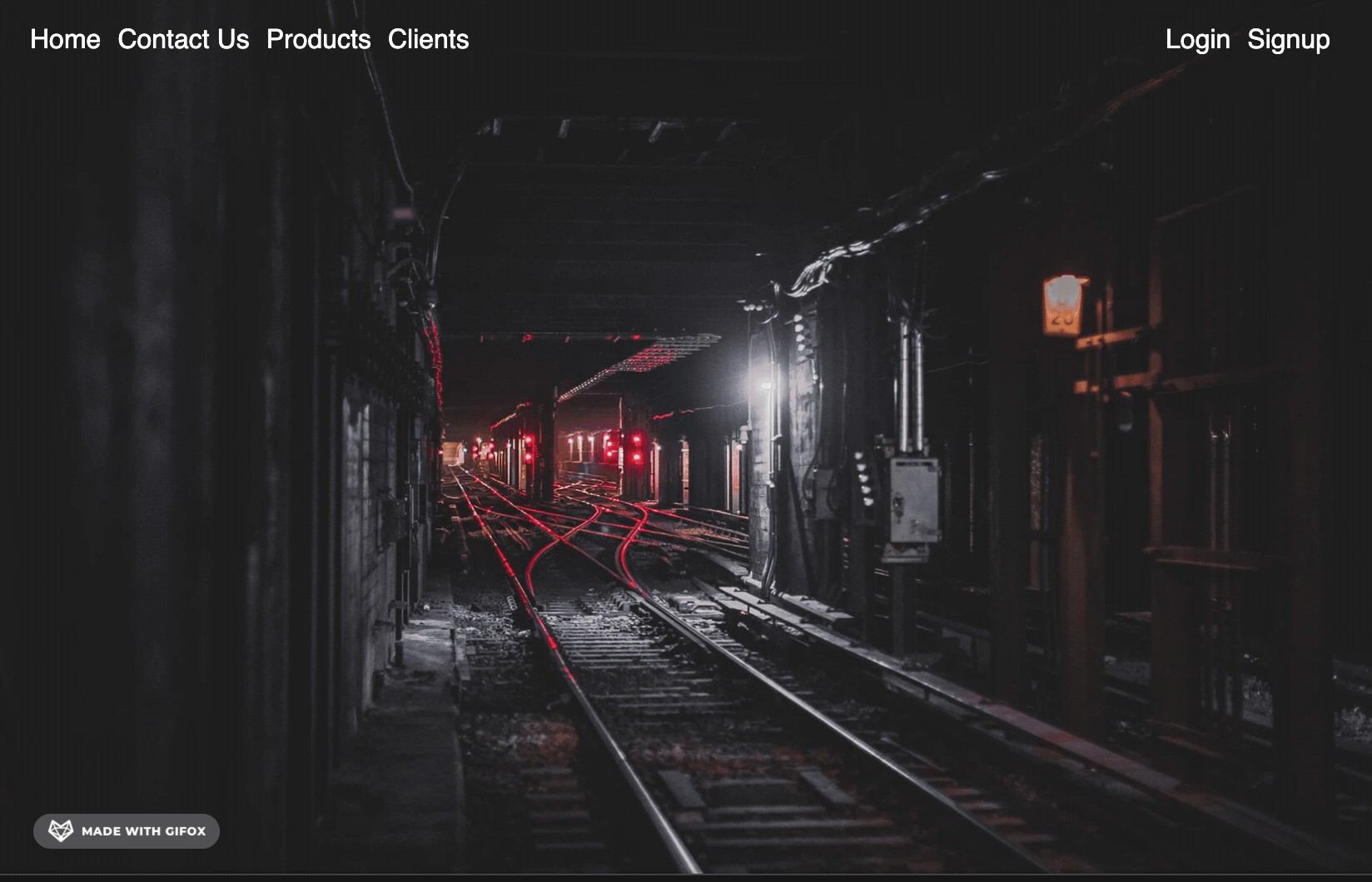
You can adjust the threshold value depending on when you want the styles to apply or the intersection to trigger.
您可以根据希望何时应用样式或触发相交来调整阈值。
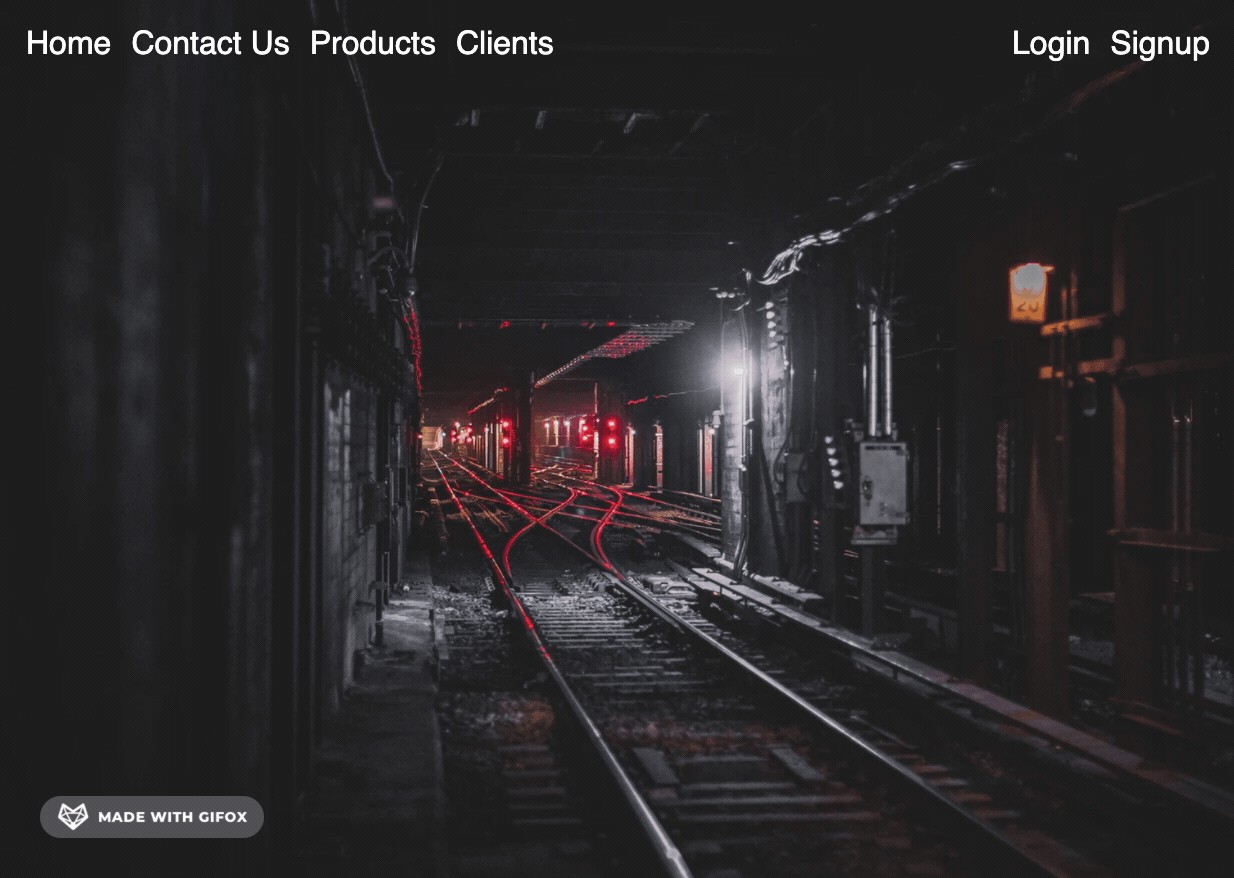
Code sandbox:
代码沙箱:
延迟加载图像 (Lazy loading images)
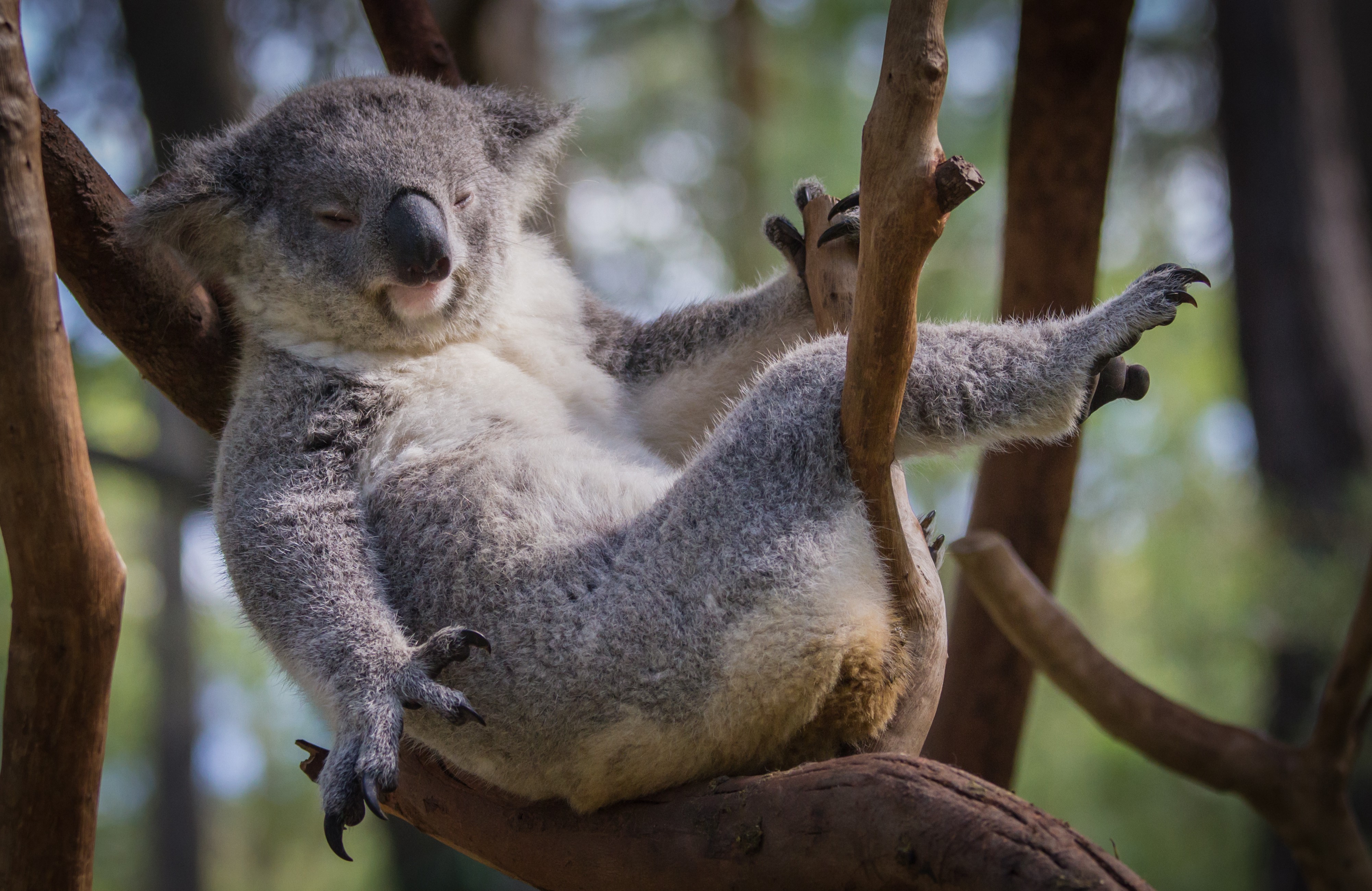
This simply means not loading images on load of the page but just on demand. We will utilize it on scroll with the help of intersection observer.
这只是意味着不在页面加载时加载图像,而只是按需加载。 我们将在相交观察器的帮助下滚动使用它。
Initially the HTML will have data attribute as data-src instead of src on all img tags as we don’t want to load them in the beginning.
最初,HTML的data属性为data-src,而不是所有img标签上的src,因为我们不想在一开始就加载它们。
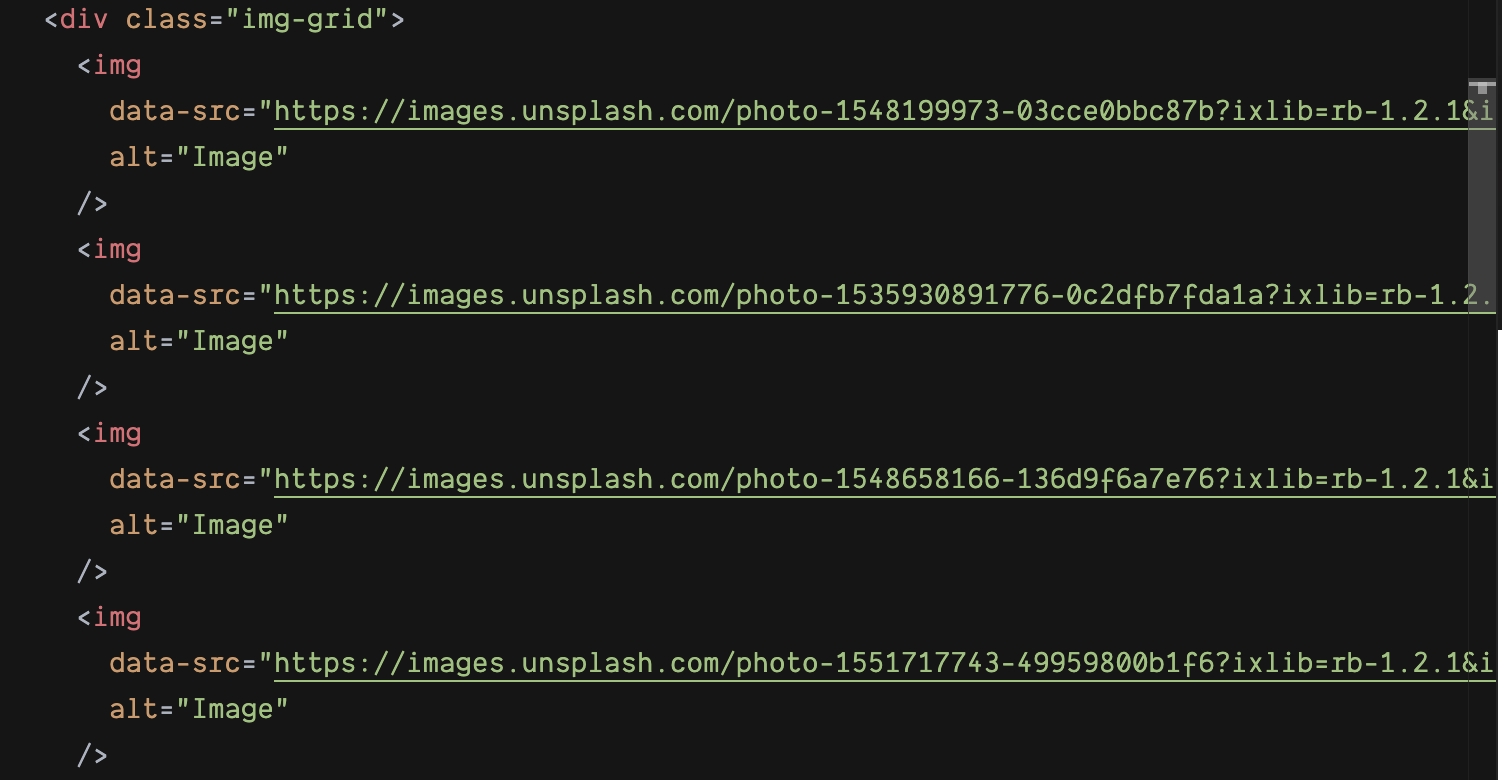
Here’s how the script will look like:
脚本如下所示:
const images = document.querySelectorAll(“.gallery img”);const imagesObeserver = new IntersectionObserver(function(entries) {
entries.forEach(entry => {
if (entry.isIntersecting) {
showImages(entry.target);
imagesObeserver.unobserve(entry.target);
}
});
},
{});
If the entry object isIntersecting
is true, we simply load the images in showImages function defined below and unobserve the target thereafter as we don’t need to observe it once its loaded.
如果入口对象isIntersecting
为true,则只需在下面定义的showImages函数中加载图像,然后取消观察目标,因为一旦加载目标就无需观察它。
Feeding in the data-src to src attribute of image tags:
将图像标签的data-src馈入src属性:
function showImages(el) {
el.src = el.dataset.src;
}
Since there are multiple images to observe, we need to loop over them:
由于要观察多个图像,因此我们需要遍历它们:
for (let image of images) {
imagesObserver.observe(image);
}
Here’s lazy load in action:
这是实际的延迟加载:
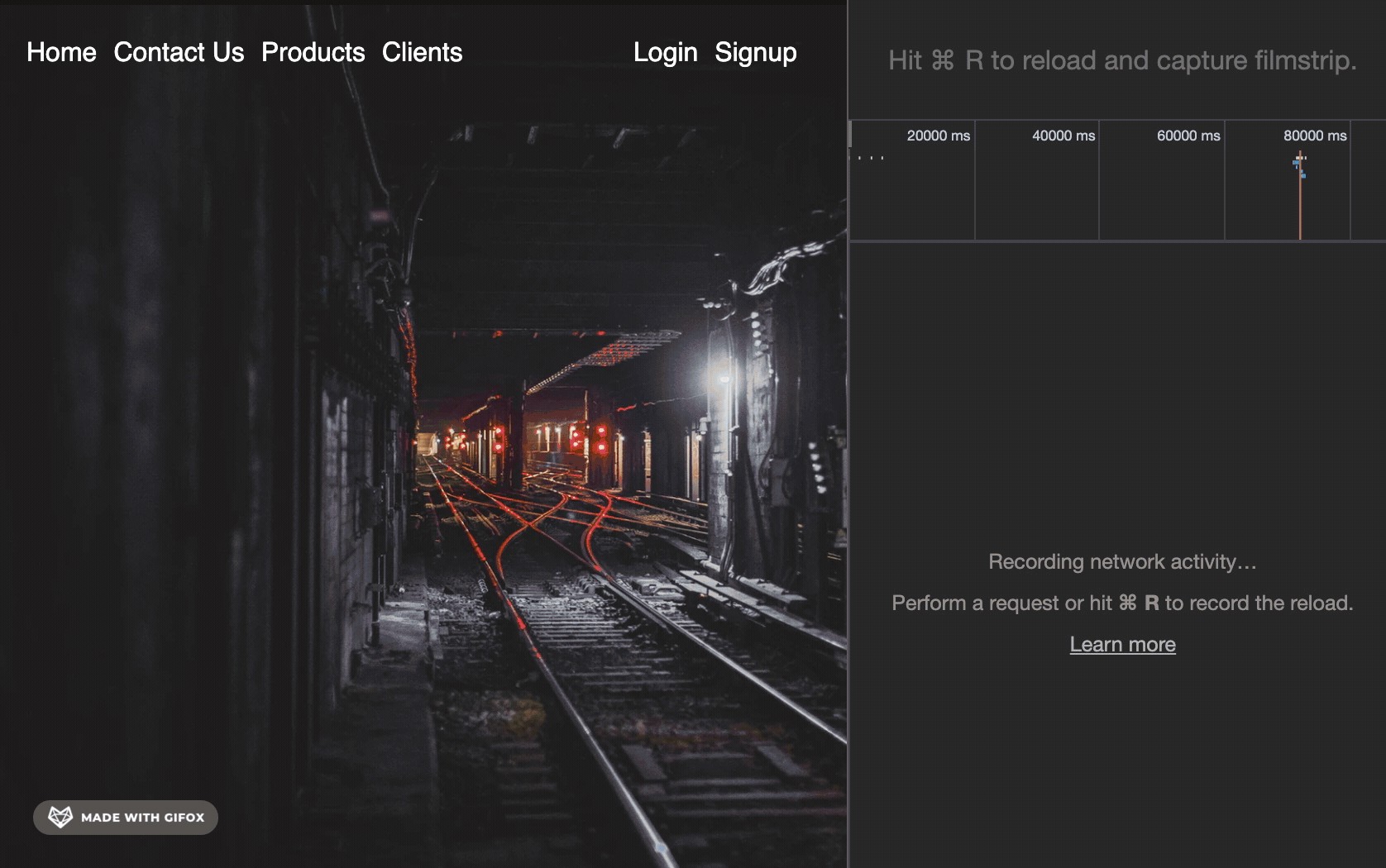
Here’s the code sandbox:
这是代码沙箱:
Intersection Observer API的其他一些用例 (Some other use cases of Intersection Observer API)
- Implementing “infinite scrolling” web sites, where more and more content is loaded and rendered as you scroll, so that the user doesn’t have to flip through pages. 实施“无限滚动”网站,在您滚动时会加载和呈现越来越多的内容,从而使用户不必翻阅页面。
- Reporting of visibility of advertisements in order to calculate ad revenues. 报告广告可见度,以计算广告收入。
- Deciding whether or not to perform tasks or animation processes based on whether or not the user will see the result. 根据用户是否会看到结果来决定是否执行任务或动画处理。
翻译自: https://medium.com/javascript-in-plain-english/intersection-observer-928a0ce309
matlab 交叉口仿真