uuid uuid4
获取AWS Honeycode表UUID (Get AWS Honeycode Table UUIDs)
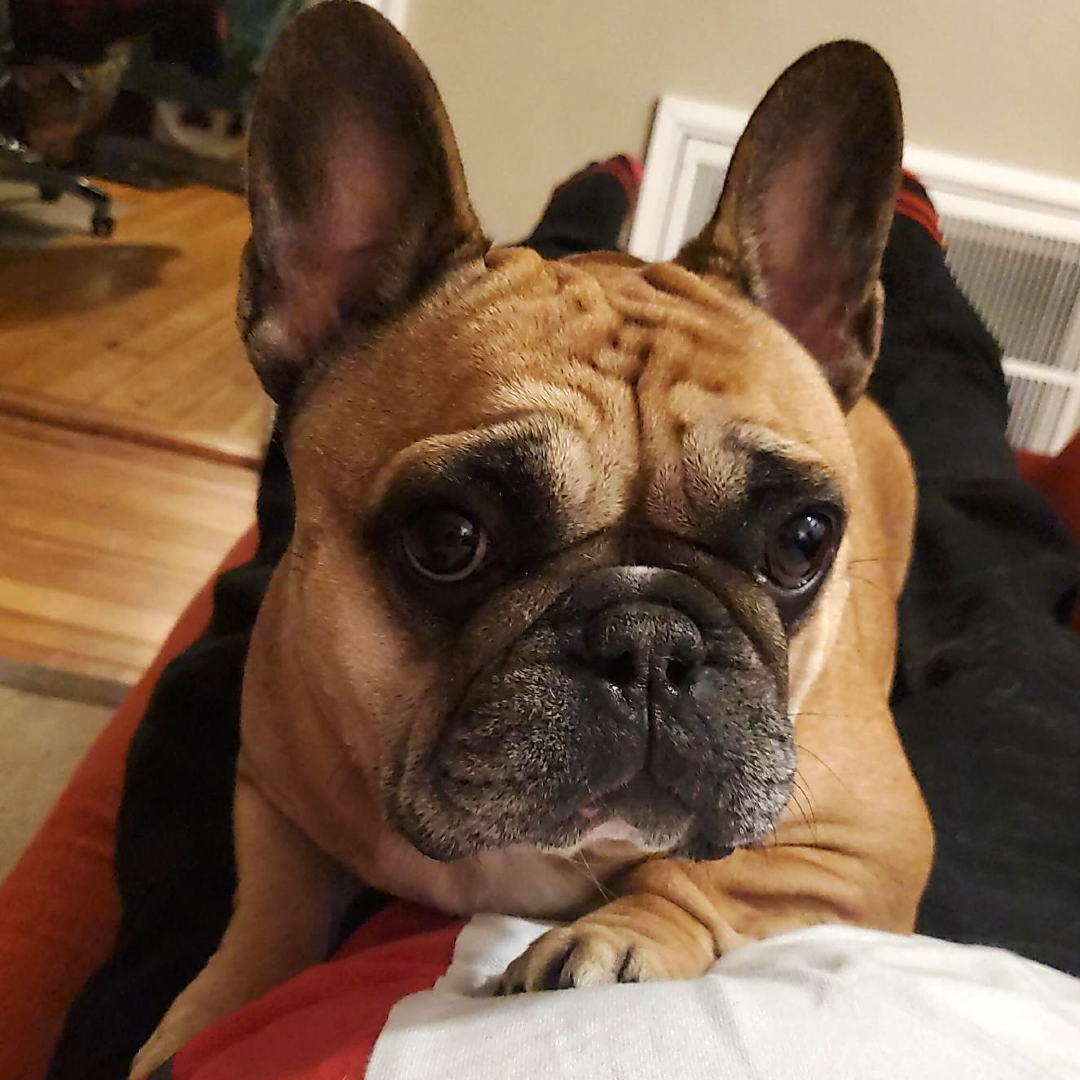
While looking to keeping Amazon Honeycode in sync with database changes, I was fortunate enough to come across Ian Mckay’s project honeycode-appflow-integration where he demonstrated the calls he makes.
在希望使Amazon Honeycode与数据库更改保持同步的同时,我很幸运地遇到了Ian Mckay的项目honeycode-appflow-integration ,他在其中演示了他所做的调用。
The Honeycode API is pretty locked down, and primarily based around ARNs and UUIDs. Fun stuff! Also their.. terminology.. between the UI and API appears to be pretty out of sync?
Honeycode API已被锁定,并且主要基于ARN和UUID。 好玩的东西! UI和API之间的术语也似乎很不同步吗?
So for a user to get their table UUID, they would also need to know their workbook UUID, and rather then trying to explain how to parse this all out, I wrote a very ugly bit of code to query the API and provide it to you.
因此,对于用户而言,获取表UUID的同时,他们还需要知道工作簿的UUID,然后尝试解释如何解析所有内容,我编写了非常难看的代码来查询API并将其提供给您。
This has resulted in query-honeycode.
这导致了query-honeycode 。
工作簿UUID (Workbook UUIDs)
To get the Workbook UUIDs we need to accomplish the following:
要获取工作簿UUID,我们需要完成以下任务:
- Login to Honeycode and get an api token 登录到Honeycode并获取api令牌
- Find out the Honeycode User ID 找出Honeycode用户ID
- Retrieve our Drive ARN 检索我们的云端硬盘ARN
- Retrieve the list of Workbooks 检索工作簿列表
API令牌 (API Token)
Using Ian’s method of retrieving the API token, bluesky-api-token
:
使用Ian的方法获取API令牌bluesky-api-token
:
const loginReq = await fetch("https://bhauthngateway.us-east-1.honeycode.aws/v2/login", {
"headers": {
"accept": "application/json, text/plain, */*",
"content-type": "application/json;charset=UTF-8",
"origin": "https://builder.honeycode.aws"
},
"body": JSON.stringify({
"emailAddress": parameterLogin,
"password": parameterPassword
}),
"method": "POST",
"mode": "cors"
});
let apitoken = '';
for (let cookie of loginReq.headers.raw()['set-cookie']) {
if (cookie.startsWith("bluesky-api-token=")) {
apitoken = cookie.split("=")[1].split(";")[0];
}
}
Honeycode用户ID (Honeycode User ID)
To get the list of workbooks, we need to include our userId in the call — odd when we have an API token. To do this we hit the logged-in-user-profile
endpoint:
要获取工作簿列表,我们需要在调用中包含userId-当我们拥有API令牌时为奇数。 为此,我们命中了logged-in-user-profile
端点:
const loggedInUserReq = await fetch("https://bhauthngateway.us-east-1.honeycode.aws/v2/logged-in-user-profile", {
"headers": {
"accept": "*/*",
"content-encoding": "amz-1.0",
"content-type": "application/json",
"cookie": "bluesky-api-token=" + apitoken,
"origin": "https://builder.honeycode.aws"
},
"method": "GET",
"mode": "cors",
"credentials": "include"
});
const loggedInUserData = await loggedInUserReq.json();
const ownerId = loggedInUserData.userProfile.userId;
驱动器ARN (Drive ARN)
So this is where we seem to start running into the terminology strangeness? We will pull the workbook ARN which appears to be our Drive ARN, i.e. our main dashboard.
因此,这似乎是我们开始遇到术语怪异之处的地方? 我们将拉出工作簿ARN,该工作簿似乎是我们的Drive ARN,即我们的主仪表板。
const controlReq = await fetch("https://control.us-west-2.honeycode.aws/", {
"headers": {
"accept": "*/*",
"content-encoding": "amz-1.0",
"content-type": "application/json",
"x-amz-target": "com.amazon.sheets.control.api.SheetsControlServiceAPI_20170701.DescribeAttacheWorkbook",
"x-client-id": "clientRegion|BeehiveSDSJSUtils||||",
"cookie": "bluesky-api-token=" + apitoken,
"origin": "https://builder.honeycode.aws"
},
"body": JSON.stringify({"attacheWorkbookType": "user_attache", "ownerId": ownerId}),
"method": "POST",
"mode": "cors",
"credentials": "include"
});
const controlData = await controlReq.json();
const workbookArn = controlData.workbook;
工作簿ARN (Workbook ARN)
So… we have the dashboard it appears, and it is made up of multiple tables. We need to get the list of tables, and identify the table which contains our workbooks. Then we need to query THAT table to get the list of workbooks and their associated ARNs.
所以……我们有一个显示板,它由多个表组成。 我们需要获取表列表,并标识包含我们的工作簿的表。 然后,我们需要查询THAT表以获取工作簿及其关联的ARN的列表。
The first step, lets get the list of tables:
第一步,让我们获取表列表:
const tableReq = await fetch("https://pod11.dp.us-west-2.honeycode.aws/external/", {
"headers": {
"accept": "*/*",
"content-encoding": "amz-1.0",
"content-type": "application/json",
"x-amz-target": "com.amazon.sheets.data.external.SheetsDataService.ListTables",
"x-client-id": "clientRegion|BeehiveSDSJSUtils||||",
"cookie": "bluesky-api-token=" + apitoken,
"origin": "https://builder.honeycode.aws"
},
"body": JSON.stringify({"workbookArn":workbookArn}),
"method": "POST",
"mode": "cors",
"credentials": "include"
});
const tableData = await tableReq.json();
Now the table we are interested in, that holds our workbooks, is AttacheAssetsTable
:
现在,我们感兴趣的包含工作簿的表是AttacheAssetsTable
:
var tableArn = "";
var rowCount = tableData.items.length;
for (var i=0; i< rowCount; i++) {
if(tableData.items[i].tableName === "AttacheAssetsTable") {
tableArn = tableData.items[i].tableArn;
}
}
Now with the appropriate table arn, we have to query it to find the contents:
现在使用适当的表arn,我们必须对其进行查询以查找内容:
const workbookReq = await fetch("https://pod11.dp.us-west-2.honeycode.aws/external/", {
"headers": {
"accept": "*/*",
"content-encoding": "amz-1.0",
"content-type": "application/json",
"x-amz-target": "com.amazon.sheets.data.external.SheetsDataService.QueryTableRowsByFilter",
"x-client-id": "clientRegion|BeehiveSDSJSUtils||||",
"cookie": "bluesky-api-token=" + apitoken,
"origin": "https://builder.honeycode.aws"
},
"body": JSON.stringify({"tableArn":tableArn}),
"method": "POST",
"mode": "cors",
"credentials": "include"
});
const workbookData = await workbookReq.json();
And now there is simply the joy of parsing this block of JSON. It appears that the name of the workbooks are in column 3, while the arns are in column 4… I couldn’t see any nice attribute that would simplify this process :(
现在,解析此JSON块简直就是一件乐事。 看来工作簿的名称在第3列中,而arns在第4列中……我看不到任何可以简化此过程的好属性:(
表ARN (Table ARNs)
That was all the hard part, now that we have the Workbook ARN, we can pull the tables associated with it via:
这就是所有困难的部分,现在有了Workbook ARN,我们可以通过以下方式提取与其关联的表:
const tableReq = await fetch("https://pod17.dp.us-west-2.honeycode.aws/external/", {
"headers": {
"accept": "*/*",
"content-encoding": "amz-1.0",
"content-type": "application/json",
"x-amz-target": "com.amazon.sheets.data.external.SheetsDataService.ListSheets",
"x-client-id": "clientRegion|BeehiveSDSJSUtils||||",
"cookie": "bluesky-api-token=" + apitoken,
"origin": "https://builder.honeycode.aws"
},
"body": JSON.stringify({includeSheetDetail: true, workbookArn: parameterWorkbookArn}),
"method": "POST",
"mode": "cors",
"credentials": "include"
});const tableData = await tableReq.json();
运行脚本 (Running the Script)
Step one is to pull the workbooks:
第一步是提取工作簿:
$ npm install $ node index.js workbooks -u USERNAME -p "PASSWORD" Workbook Name Workbook UUID
------------- -------------
Workbook1 arn:aws:sheets:us-west-2:1234567890:workbook:a132hkhk-i7yugy-ihijk--3f409c8fc35a
And then step two is to get the corresponding tables:
然后第二步是获取相应的表:
$ node index.js tables -u USERNAME -p "PASSWORD" -w "arn:aws:sheets:us-west-2:1234567890:workbook:a132hkhk-i7yugy-ihijk-3f409c8fc35a" Table Name Table UUID
------------- -------------
Table1 arn:aws:sheets:us-west-2:1234567890:sheet:a132hkhk-i7yugy-ihijk-3f409c8fc35a/b2cdc524-4085-352f-86b9-c7c956eb9da2
Table2 arn:aws:sheets:us-west-2:1234567890:sheet:a132hkhk-i7yugy-ihijk-3f409c8fc35a/aa71e742-1e32-4882-9f18-e45a7bb555dc
Table3 arn:aws:sheets:us-west-2:1234567890:sheet:a132hkhk-i7yugy-ihijk-3f409c8fc35a/a39790d1-e09b-48a8-9e97-5cf4c671f5e9
Note that there is zero error handling etc.. I’d throw it onto a To-Do list somewhere, but really hope they open the API up before then.
请注意,错误处理等为零。我会将其放在某个地方的待办事项列表上,但真的希望他们在此之前打开API。
Originally published at https://gizmo.codes.
最初发布在 https://gizmo.codes上 。
翻译自: https://medium.com/swlh/get-aws-honeycode-table-uuids-bbfdbded57dc
uuid uuid4