技术 (Technical)
介绍 (Introduction)
The main content of this article will present how the AlexNet Convolutional Neural Network(CNN) architecture is implemented using TensorFlow and Keras.
本文的主要内容将介绍如何使用TensorFlow和Keras实现AlexNet卷积神经网络(CNN)架构。
But first, allow me to provide a brief background behind the AlexNet CNN architecture.
但是首先,请允许我简要介绍AlexNet CNN架构的背景知识。
AlexNet was first utilized in the public setting when it won the ImageNet Large Scale Visual Recognition Challenge(ILSSVRC 2012 contest). It was at this contest that AlexNet showed that deep convolutional neural network can be used for solving image classification.
AlexNet赢得ImageNet大规模视觉识别挑战赛(ILSSVRC 2012竞赛)时首次在公共场合使用。 正是在这次竞赛中,AlexNet证明了深度卷积神经网络可用于解决图像分类。
AlexNet won the ILSVRC 2012 contest by a margin.
AlexNet以微弱优势赢得了2012年ILSVRC比赛。
The research paper that detailed the internal components of the CNN architecture also introduced some novel techniques and methods such as efficient computing resource utilization; data augmentation, GPU training, and multiple strategies to prevent overfitting within neural networks.
该研究论文详细介绍了CNN体系结构的内部组件,还介绍了一些新颖的技术和方法,例如有效的计算资源利用; 数据扩充,GPU训练和多种策略来防止神经网络内的过度拟合。
I have written an article that presents key ideas and techniques that AlexNet brought to the world of computer vision and deep learning.
我写了一篇文章 ,介绍AlexNet带给计算机视觉和深度学习领域的关键思想和技术。
以下是本文的一些主要学习目标: (Here are some of the key learning objectives from this article:)
Introduction to neural network implementation with Keras and TensorFlow
用Keras和TensorFlow实现神经网络的简介
Data preprocessing with TensorFlow
使用TensorFlow进行数据预处理
Training visualization with TensorBoard
使用TensorBoard训练可视化
Description of standard machine learning terms and terminologies
标准机器学习术语和术语的描述
AlexNet实施 (AlexNet Implementation)
AlexNet CNN is probably one of the simplest methods to approach understanding deep learning concepts and techniques.
AlexNet CNN可能是了解深度学习概念和技术的最简单方法之一。
AlexNet is not a complicated architecture when it is compared with some state of the art CNN architectures that have emerged in the more recent years.
与最近几年出现的一些最新的CNN架构相比,AlexNet并不是一个复杂的架构。
AlexNet is simple enough for beginners and intermediate deep learning practitioners to pick up some good practices on model implementation techniques.
AlexNet非常简单,对于初学者和中级深度学习从业人员而言,他们可以学习一些有关模型实现技术的良好实践。
All code presented in this article is written using Jupyter Lab. At the end of this article is a GitHub link to the notebook that includes all code in the implementation section.
本文介绍的所有代码都是使用Jupyter Lab编写的。 本文结尾处是指向笔记本的GitHub链接,其中包括实现部分中的所有代码。
So let’s begin.
因此,让我们开始吧。
1.工具和库 (1. Tools And Libraries)
We begin implementation by importing the following libraries:
我们通过导入以下库开始实施:
TensorFlow: An open-source platform for the implementation, training, and deployment of machine learning models.
TensorFlow :一个用于实施,培训和部署机器学习模型的开源平台。
Keras: An open-source library used for the implementation of neural network architectures that run on both CPUs and GPUs.
Keras :一个开放源代码库,用于实现在CPU和GPU上运行的神经网络体系结构。
Matplotlib: A visualization python tool used for illustrating interactive charts and images.
Matplotlib :一种可视化的python工具,用于说明交互式图表和图像。
import tensorflow as tf
from tensorflow import keras
import matplotlib.pyplot as plt
import os
import time
2.数据集 (2. Dataset)
The CIFAR-10 dataset contains 60,000 colour images, each with dimensions 32x32px. The content of the images within the dataset is sampled from 10 classes.
CIFAR-10数据集包含60,000个彩色图像,每个彩色图像的尺寸为32x32px 。 数据集中的图像内容是从10个类别中采样的。
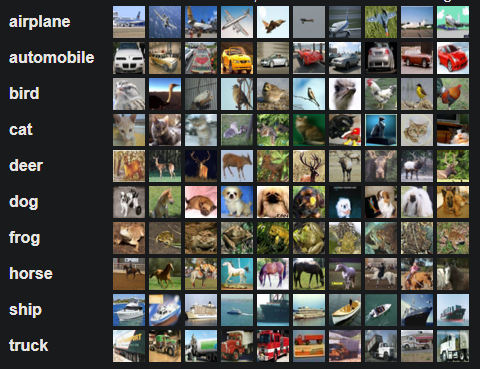
CIFAR-10 images were aggregated by some of the creators of the AlexNet network, Alex Krizhevsky and Geoffrey Hinton.
CIFAR-10图像由AlexNet网络的一些创建者Alex Krizhevsky和Geoffrey Hinton聚合而成。
The deep learning Keras library provides direct access to the CIFAR10 dataset with relative ease, through its dataset module. Accessing common datasets such as CIFAR10 or MNIST, becomes a trivial task with Keras.
深度学习Keras库通过其数据集模块相对轻松地直接访问CIFAR10数据集 。 使用Keras ,访问常见的数据集(例如CIFAR10或MNIST )成为一项琐碎的任务。
(train_images, train_labels), (test_images, test_labels) = keras.datasets.cifar10.load_data()
In order to reference the class names of the images during the visualization stage, a python list containing the classes is initialized with the variable name CLASS_NAMES.
为了在可视化阶段引用图像的类名,使用变量名CLASS_NAMES .
初始化了包含这些类的python列表CLASS_NAMES .
CLASS_NAMES= ['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck']
The CIFAR dataset is partitioned into 50,000 training data and 10,000 test data by default. The last partition of the dataset we require is the validation data.
默认情况下,CIFAR数据集分为50,000个训练数据和10,000个测试数据。 我们需要的数据集的最后一个分区是验证数据。
The validation data is obtained by taking the last 5000 images within the training data.
通过拍摄训练数据中的最后5000张图像获得验证数据。
validation_images, validation_labels = train_images[:5000], train_labels[:5000]
train_images, train_labels = train_images[5000:], train_labels[5000:]
Training Dataset: This is the group of our dataset used to train the neural network directly. Training data refers to the dataset partition exposed to the neural network during training.
训练数据集 :这是我们的数据集,用于直接训练神经网络。 训练数据是指训练期间暴露于神经网络的数据集分区。
Validation Dataset: This group of the dataset is utilized during training to assess the performance of the network at various iterations.
验证数据集 :在训练期间利用该组数据集来评估网络在各种迭代中的性能。
Test Dataset: This partition of the dataset evaluates the performance of our network after the completion of the training phase.
测试数据集 :在训练阶段完成后, 数据集的此分区评估了我们网络的性能。
TensorFlow provides a suite of functions and operations that enables easy data manipulation and modification through a defined input pipeline.
TensorFlow提供了一组功能和操作,可通过定义的输入管道轻松进行数据操作和修改。
To be able to access these methods and procedures, it is required that we transform our dataset into an efficient data representation TensorFlow is familiar with. This is achieved using the tf.data.Dataset API.
为了能够访问这些方法和过程,需要将数据集转换为TensorFlow熟悉的高效数据表示形式。 这是使用tf.data.Dataset实现的 API。
More specifically, tf.data.Dataset.from_tensor_slices method takes the train, test, and validation dataset partitions and returns a corresponding TensorFlow Dataset representation.
更具体地说, tf.data.Dataset.from_tensor_slices方法采用训练,测试和验证数据集分区,并返回相应的TensorFlow数据集表示形式。
train_ds = tf.data.Dataset.from_tensor_slices((train_images, train_labels))
test_ds = tf.data.Dataset.from_tensor_slices((test_images, test_labels))
validation_ds = tf.data.Dataset.from_tensor_slices((validation_images, validation_labels))