老孟导读:此篇文章是 Flutter 动画系列文章第四篇,本文介绍动画序列、共享动画、路由动画。
动画序列
Flutter中组合动画使用Interval
,Interval
继承自Curve
,用法如下:
Animation _sizeAnimation = Tween(begin: 100.0, end: 300.0).animate(CurvedAnimation( parent: _animationController, curve: Interval(0.5, 1.0)));
表示_sizeAnimation
动画从0.5(一半)开始到结束,如果动画时长为6秒,_sizeAnimation
则从第3秒开始。
Interval
中begin
和end
参数值的范围是0.0到1.0。
下面实现一个先执行颜色变化,在执行大小变化,代码如下:
class AnimationDemo extends StatefulWidget { @override State createState() => _AnimationDemo();}class _AnimationDemo extends State with SingleTickerProviderStateMixin { AnimationController _animationController; Animation _colorAnimation; Animation _sizeAnimation; @override void initState() { _animationController = AnimationController(duration: Duration(seconds: 5), vsync: this) ..addListener((){setState(() { });}); _colorAnimation = ColorTween(begin: Colors.red, end: Colors.blue).animate( CurvedAnimation( parent: _animationController, curve: Interval(0.0, 0.5))); _sizeAnimation = Tween(begin: 100.0, end: 300.0).animate(CurvedAnimation( parent: _animationController, curve: Interval(0.5, 1.0))); //开始动画 _animationController.forward(); super.initState(); } @override Widget build(BuildContext context) { return Center( child: Column( mainAxisSize: MainAxisSize.min, children: [ Container( height: _sizeAnimation.value, width: _sizeAnimation.value, color: _colorAnimation.value), ], ), ); } @override void dispose() { _animationController.dispose(); super.dispose(); }}
效果如下:
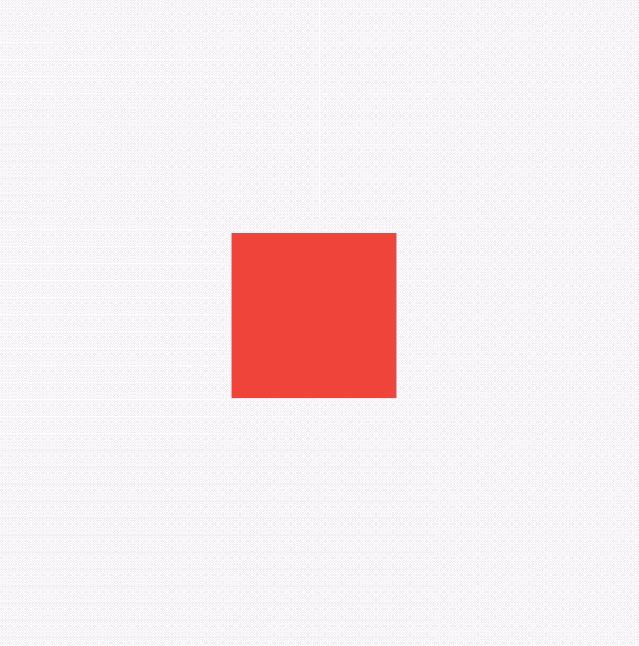
我们也可以设置同时动画,只需将2个Interval
的值都改为Interval(0.0, 1.0)
。
想象下面的场景,一个红色的盒子,动画时长为6秒,前40%的时间大小从100->200,然后保持200不变20%的时间,最后40%的时间大小从200->300,这种效果通过TweenSequence实现,代码如下:
_animation = TweenSequence([ TweenSequenceItem( tween: Tween(begin: 100.0, end: 200.0) .chain(CurveTween(curve: Curves.easeIn)), weight: 40), TweenSequenceItem(tween: ConstantTween(200.0), weight: 20), TweenSequenceItem(tween: Tween(begin: 200.0, end: 300.0), weight: 40),]).animate(_animationController);
weight
表示每一个Tween的权重。
最终效果如下:
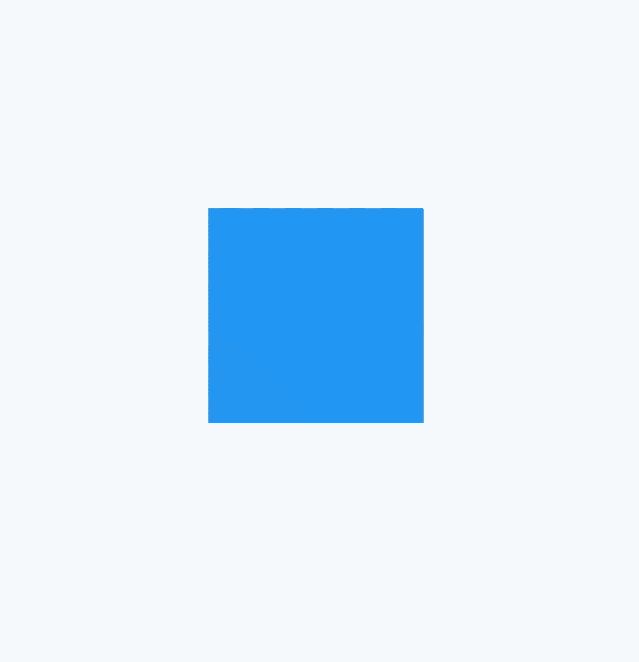
共享动画
Hero是我们常用的过渡动画,当用户点击一张图片,切换到另一个页面时,这个页面也有此图,那么使用Hero组件就在合适不过了,先看下Hero的效果图:
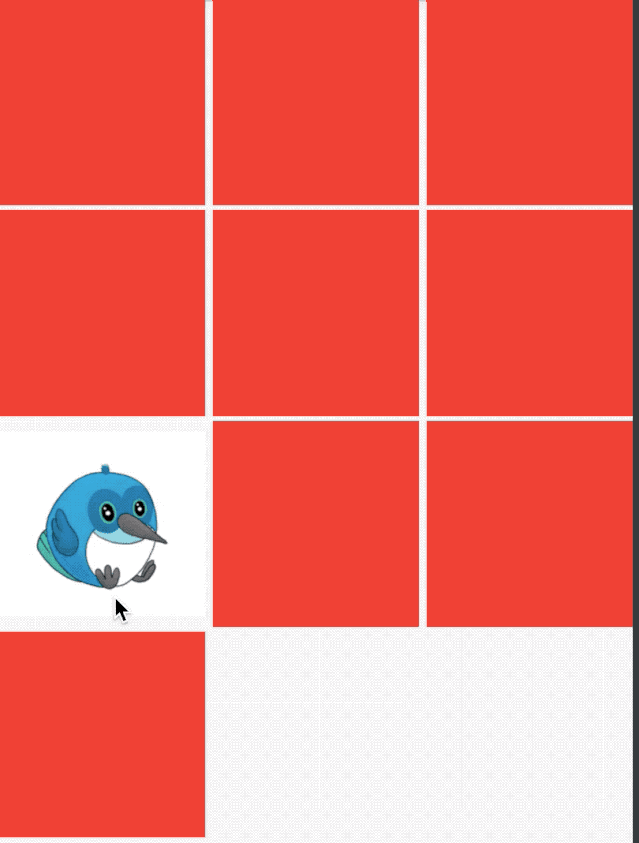
上面效果实现的列表页面代码如下:
class HeroDemo extends StatefulWidget { @override State createState() => _HeroDemo();}class _HeroDemo extends State { @override Widget build(BuildContext context) { return Scaffold( body: GridView( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 3, crossAxisSpacing: 5, mainAxisSpacing: 3), children: List.generate(10, (index) { if (index == 6) { return InkWell( onTap: () { Navigator.push( context, new MaterialPageRoute( builder: (context) => new _Hero1Demo())); }, child: Hero( tag: 'hero', child: Container( child: Image.asset( 'images/bird.png', fit: BoxFit.fitWidth, ), ), ), ); } return Container( color: Colors.red, ); }), ), ); }}
第二个页面代码如下:
class _Hero1Demo extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(), body: Container( alignment: Alignment.topCenter, child: Hero( tag: 'hero', child: Container( child: Image.asset( 'images/bird.png', ), ), )), ); }}
2个页面都有Hero控件,且tag
参数一致。
路由动画
转场 就是从当前页面跳转到另一个页面,跳转页面在 Flutter 中通过 Navigator,跳转到新页面如下:
Navigator.push(context, MaterialPageRoute(builder: (context) { return _TwoPage();}));
回退到前一个页面:
Navigator.pop(context);
Flutter 提供了两个转场动画,分别为 MaterialPageRoute 和 CupertinoPageRoute,MaterialPageRoute 根据不同的平台显示不同的效果,Android效果为从下到上,iOS效果为从左到右。CupertinoPageRoute 不分平台,都是从左到右。
使用 MaterialPageRoute 案例如下:
class NavigationAnimation extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(), body: Center( child: OutlineButton( child: Text('跳转'), onPressed: () { Navigator.push(context, CupertinoPageRoute(builder: (context) { return _TwoPage(); })); }, ), ), ); }}class _TwoPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(), body: Container( color: Colors.blue, ), ); }}
iOS效果:
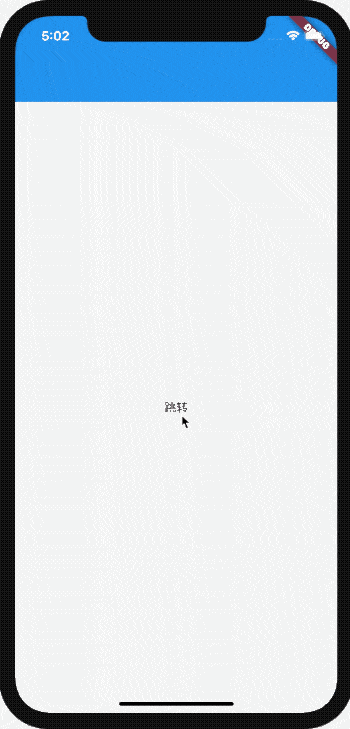
如果要自定义转场动画如何做?
自定义任何组件都是一样的,如果系统有类似的,直接看源代码是如何实现的,然后按照它的模版自定义组件。
回到正题,看 MaterialPageRoute 的继承关系:
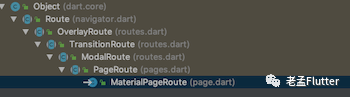
PageRoute 的继承关系:
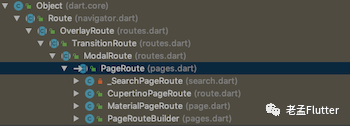
MaterialPageRoute 和 CupertinoPageRoute 都是继承PageRoute,所以重点是 PageRoute,PageRoute 是一个抽象类,其子类还有一个 PageRouteBuilder,看其名字就知道这是一个可以自定义动画效果,PageRouteBuilder源代码:
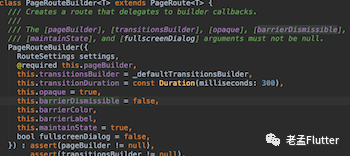
pageBuilder
表示跳转的页面。
transitionsBuilder
表示页面的动画效果,默认值代码:
Widget _defaultTransitionsBuilder(BuildContext context, Animation animation, Animation secondaryAnimation, Widget child) { return child;}
通过源代码发现,默认情况下没有动画效果。
自定义转场动画只需修改transitionsBuilder
即可:
Navigator.push( context, PageRouteBuilder(pageBuilder: ( BuildContext context, Animation animation, Animation secondaryAnimation, ) { return _TwoPage(); }, transitionsBuilder: (BuildContext context, Animation animation, Animation secondaryAnimation, Widget child) { return SlideTransition( position: Tween(begin: Offset(-1, 0), end: Offset(0, 0)) .animate(animation), child: child, ); }));
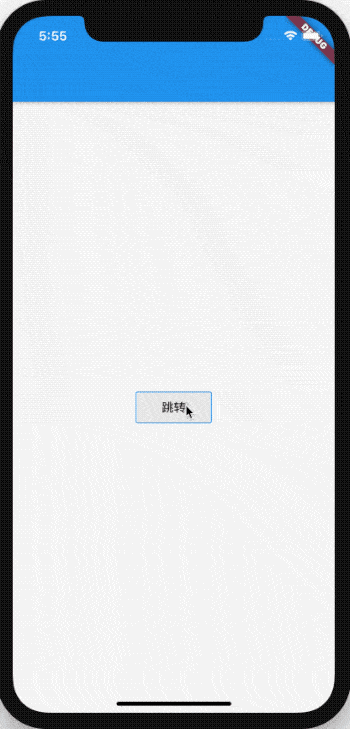
将其封装,方便使用:
class LeftToRightPageRoute extends PageRouteBuilder { final Widget newPage; LeftToRightPageRoute(this.newPage) : super( pageBuilder: ( BuildContext context, Animation animation, Animation secondaryAnimation, ) => newPage, transitionsBuilder: ( BuildContext context, Animation animation, Animation secondaryAnimation, Widget child, ) => SlideTransition( position: Tween(begin: Offset(-1, 0), end: Offset(0, 0)) .animate(animation), child: child, ), );}
使用:
Navigator.push(context, LeftToRightPageRoute(_TwoPage()));
不仅是这些平移动画,前面所学的旋转、缩放等动画直接替换 SlideTransition 即可。
上面的动画只对新的页面进行了动画,如果想实现当前页面被新页面从顶部顶出的效果,实现方式如下:
class CustomPageRoute extends PageRouteBuilder { final Widget currentPage; final Widget newPage; CustomPageRoute(this.currentPage, this.newPage) : super( pageBuilder: ( BuildContext context, Animation animation, Animation secondaryAnimation, ) => currentPage, transitionsBuilder: ( BuildContext context, Animation animation, Animation secondaryAnimation, Widget child, ) => Stack( children: [ SlideTransition( position: new Tween( begin: const Offset(0, 0), end: const Offset(0, -1), ).animate(animation), child: currentPage, ), SlideTransition( position: new Tween( begin: const Offset(0, 1), end: Offset(0, 0), ).animate(animation), child: newPage, ) ], ), );}
本质就是对两个页面做动画处理,使用:
Navigator.push(context, CustomPageRoute(this, _TwoPage()));
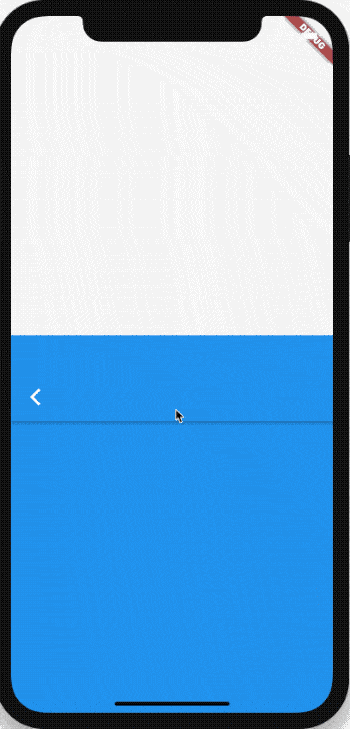
除了自定义路由动画,在 Flutter 1.17 发布大会上,Flutter 团队还发布了新的 Animations 软件包,该软件包提供了实现新的 Material motion 规范的预构建动画。
里面提供了一系列动画,部分效果:
详情:Flutter 1.17 新 Material motion 规范的预构建动画

【Flutter组件终结篇】332个组件 658页PDF
【Flutter 实战】一文学会20多个动画组件
【Flutter实战】动画核心(2/2)
【Flutter实战】动画核心(1/2)
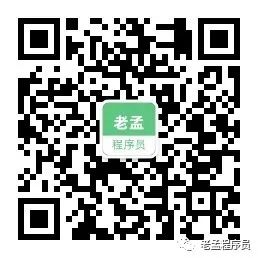