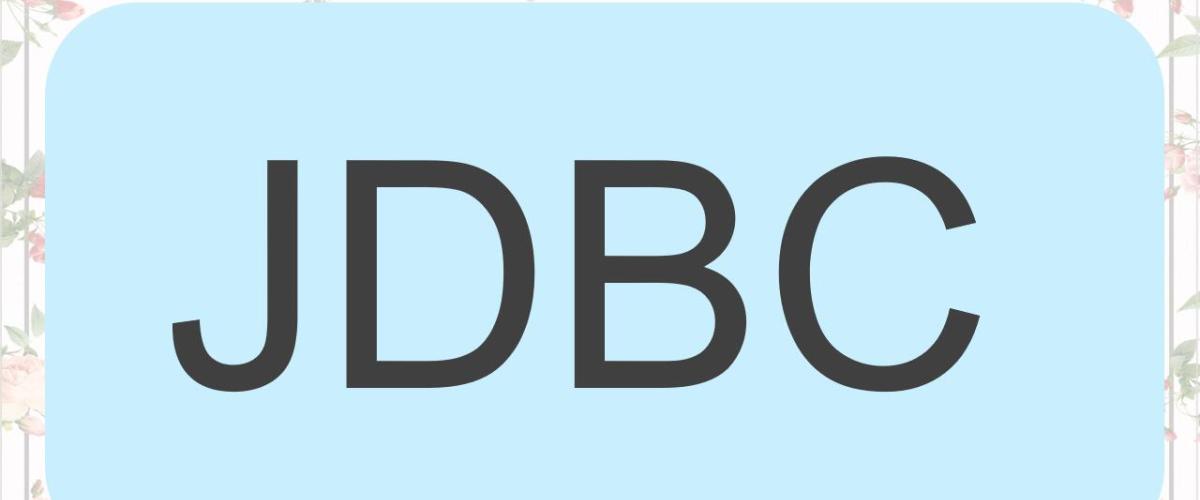
上一篇文章说了,JDBC可以做三件事:
与数据库建立连接、发送、操作数据库的语句并处理结果;
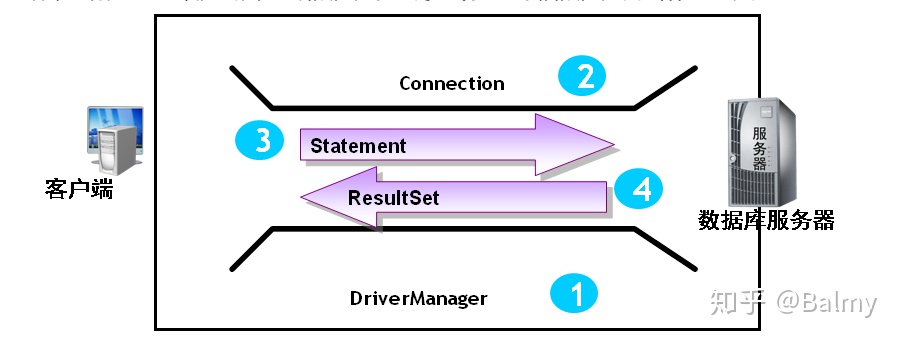
JDBC访问数据的步骤分为6步
1:加载一个Driver驱动(需要添加mysql或者oralce的驱动包)
反射 Class.forName (驱动地址)
Class.forName("com.mysql.jdbc.Driver");
2:创建数据库连接(Connection)
// 2:创建数据库连接(Connection)
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/slayer","root","root");
路径解释 "jdbc:数据库名称://ip地址:端口号/数据库名称","用户名","密码"
"jdbc:mysql://localhost:3306/slayer","root","root"
3:创建SQL命令发送器Statement (prepareStatement)
Statement statement = connection.createStatement();
4:通过Statement发送SQL命令并得到结果(insert/delete/update/select/语句)
// 4:通过Statement发送SQL命令并得到结果(insert/delete/update/select/语句)
String sql = "insert into emp values(8888,'张三','CLERK','7788','2018-08-08','1000','2000',10)";
int n = statement.executeUpdate(sql);
5:处理SQL结果
if (n>0){
System.out.println("添加成功");
}else {
System.out.println("添加失败");
}
6:关闭数据库资源(ResultSet)
connection.close();
statement.close();
完整代码
package com.lin;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class jdbcTest {
//向Departments 表中添加一个数据
public void insertDepartments(String department_name,int location_id) {
Connection connection = null;
Statement statement = null;
try {
//1.注册驱动
Class.forName("com.mysql.jdbc.Driver");
//创建链接 useUnicode=true 开启字符编码集 characterEncoding=utf-8 使用utf-8编码 目的:防止乱码
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/learn?useUnicode=true&characterEncoding=utf-8&useSSL=false",
"root", "chaoge");
//编辑sql语句
String sql ="insert into departments values(default,'"+department_name+"','"+location_id+"')";
//创建Statement对象用于发送数据
statement = connection.createStatement();
int flag = statement.executeUpdate(sql);
if (falg ==1){
System.out.println("修改成功");
}else {
System.out.println("修改失败");
}
} catch (Exception e) {
e.printStackTrace();
}finally {
if (statement !=null){
try {
statement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (connection!=null){
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
//更新departments 表中的department_di 为6 的数据 部门名称修改为java研发部 location_id改为6
public void updateDepartments( String department_id,String department_name, int location_id){
Connection connection = null;
Statement statement = null;
try {
//注册驱动
Class.forName("com.mysql.jdbc.Driver");
//创建链接对象
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/learn?useUnicode=true&characterEncoding=utf-8&useSSL=false",
"root", "chaoge");
//创建Statement对象用于发送数据
statement =connection.createStatement();
//创建SQL语句
String sql = "update departments d set d.department_name = '"+department_name+"',d.location_id = "+location_id+" where d.department_id ="+department_id;
int falg = statement.executeUpdate(sql);
if (falg ==1){
System.out.println("修改成功");
}else {
System.out.println("修改失败");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
if (statement !=null){
try {
statement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (connection!=null){
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
jdbcTest jdbcTest = new jdbcTest();
// jdbcTest.insertDepartments("销售部",10);
jdbcTest.updateDepartments("6","java研发部",6);
}
}