本技巧里,我演示如何创建两个 HTML Helper,并在 ASP.NET MVC 视图中调用。您可以使用扩展方法(extension method)创建新 HTML Helper,以显示有序列表和无序列表(bulleted and numbered lists)。
给 ASP.NET MVC 应用程序创建视图时,您可以利用 HTML Helpers 呈现标准 HTML 标签。例如,除了这样写代码:
<input name="inpSubmit" type="submit" value="Click Here!" />
您还可以这样写:
<%= Html.SubmitButton("inpSubmit", "Click Here!") %>
长远来看,HTML Helpers 可以给您节省很多时间。但是,若您希望呈现的标签没有 HTML Helper 怎么办?例如,想象一下,您希望在视图上把数据库记录显示成无序列表(<ul>),而 HtmlHelper 类不包含可让您呈现无序列表的方法。要是 HTML Helper 缺少您需要的方法,扩展它吧!
通过创建新的扩展方法,您可以给 HtmlHelper 增加新的行为。扩展方法看起来像极了普通的实例方法。然而,不同于实例方法,您在一个完全不同的类中给另一个类添加扩展方法。
在 Visual Basic.Net 里,您创建扩展的方法是,创建一个模块(module),然后用一个特殊的属性类(ExtensionAttribute)来修饰扩展方法。在 C# 中,您在静态类(static class)中定义扩展方法,使用 this 关键字标识其为扩展的。
您可以添加扩展方法到 HtmlHelper 类,以将数据库记录显示为有序列表(ordered list )或无序列表(unordered list)。
Listing 1 – ListExtensions.vb (VB.NET)


































































Listing 1 – ListExtensions.cs (C#)





























































ListExtensions 类有两个公有方法:OrderedList() 与 UnorderedList()。您传递一个集合到其中一个方法以显示有序(numbered)或无序(bulleted)列表。注意到两个方法都返回字符串类型。真是的,HTML Helper 方法不过就是呈现格式化字符串到浏览器而已。
创建好扩展方法后,您就可以在视图中使用它们:
Listing 2 – Index.aspx


<%@ Page="" Language="VB" MasterPageFile="~/Views/Shared/Site.Master" AutoEventWireup="false" CodeBehind="Index.aspx.vb" Inherits="BulletedListHelper.Index" %>
<%@ Import="" Namespace="BulletedListHelper.HtmlHelpers" %>
<asp:Content ID="indexContent" ContentPlaceHolderID="MainContent" runat="server">
<h1>Movies (Ordered)</h1>
<%= Html.OrderedList(ViewData.Model) %>
<h1>Movies (Unordered)</h1>
<%= Html.UnorderedList(ViewData.Model) %>
</asp:Content>
请注意,视图文件中导入了 BulletedList.HtmlHelpers 这个域名空间(namespace)。方法 Html.OrderedList() 用以呈现有序列表,而 Html.UnorderedList() 用以呈现无序列表。这些方法通过 HtmlHelper 类被调用,正如其他扩展方法,HtmlHelper 由视图(System.Web.Mvc.ViewPage)的 Html 属性公开出来。当您在浏览器打开这个视图,您得到结果如下图所示 :
Figure 1 – 以自定义 HTML Helper 的方式呈现视图 Index.aspx
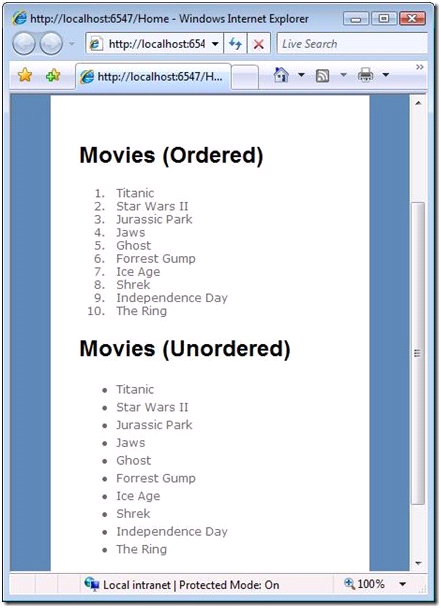
最后,控制器 HomeController 公开 Index() 方法(VB 代码省略了 ActionResult),说明您可以传递一个电影记录集合到 Index.aspx 视图。电影记录使用 Linq to SQL 方式查询出来。
Listing 3 – HomeController.vb (VB.NET)


Public Class HomeController
Inherits System.Web.Mvc.Controller
Private db As New MoviesDataContext()
Function Index()
Dim movies = From m In db.Movies Select m.Title
Return View(movies)
End Function
End Class
Listing 3 – HomeController.cs (C#)


using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using BulletedListHelper.Models;
namespace BulletedListHelper.Controllers
{
public class HomeController : Controller
{
private MoviesDataContext db = new MoviesDataContext();
public ActionResult Index()
{
var movies = from m in db.Movies select m.Title;
return View(movies);
}
}
}
您可以在 ASP.NET MVC 视图中使用这种方法呈现任何内容,例如您可以使用相同方法呈现 TreeViews,Menus,tabstrips,或者其它任何东西。
原文:ASP.NET MVC Tip #1 - Create New HTML Helpers with Extension Methods