描述:使用QtDesignner设计界面,pyQt5+python3实现主体方法制作的猜数字游戏。
游戏规则:先选择游戏等级:初级、中级、高级、魔鬼级,选择完游戏等级后点击“确定”,然后后台会自动生成一个与游戏等级匹配的“神秘数字”,游戏玩家在文本框内输入数字,再点击文本框旁边的“确定”,即可比较玩家所猜数字是否就是“神秘数字”。
游戏界面:
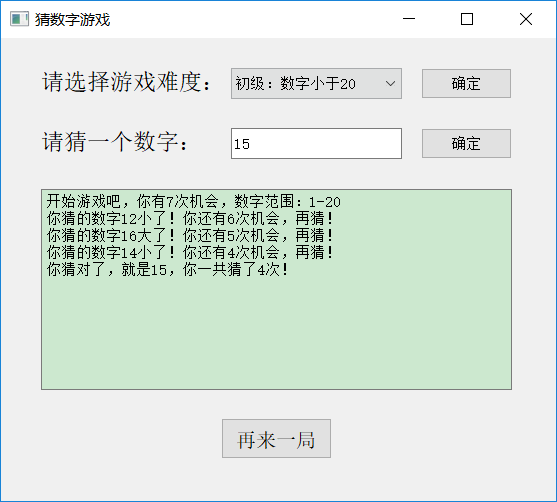
源代码:
代码1:guessNumberGame.py (界面代码)


1 # -*- coding: utf-8 -*- 2 3 # Form implementation generated from reading ui file 'guessNumberGame.ui' 4 # 5 # Created by: PyQt5 UI code generator 5.11.3 6 # 7 # WARNING! All changes made in this file will be lost! 8 9 from PyQt5 import QtCore, QtGui, QtWidgets 10 11 class Ui_Form(object): 12 def setupUi(self, Form): 13 Form.setObjectName("Form") 14 Form.resize(555, 463) 15 self.label = QtWidgets.QLabel(Form) 16 self.label.setGeometry(QtCore.QRect(40, 90, 181, 31)) 17 self.label.setObjectName("label") 18 self.comboBox = QtWidgets.QComboBox(Form) 19 self.comboBox.setGeometry(QtCore.QRect(230, 30, 171, 31)) 20 self.comboBox.setObjectName("comboBox") 21 self.comboBox.addItem("") 22 self.comboBox.addItem("") 23 self.comboBox.addItem("") 24 self.comboBox.addItem("") 25 self.pushButton_2 = QtWidgets.QPushButton(Form) 26 self.pushButton_2.setGeometry(QtCore.QRect(420, 30, 91, 31)) 27 self.pushButton_2.setObjectName("pushButton_2") 28 self.pushButton = QtWidgets.QPushButton(Form) 29 self.pushButton.setGeometry(QtCore.QRect(420, 90, 91, 31)) 30 self.pushButton.setObjectName("pushButton") 31 self.textBrowser = QtWidgets.QTextBrowser(Form) 32 self.textBrowser.setGeometry(QtCore.QRect(40, 151, 471, 201)) 33 self.textBrowser.setObjectName("textBrowser") 34 self.lineEdit = QtWidgets.QLineEdit(Form) 35 self.lineEdit.setGeometry(QtCore.QRect(230, 90, 171, 31)) 36 self.lineEdit.setObjectName("lineEdit") 37 self.label_3 = QtWidgets.QLabel(Form) 38 self.label_3.setGeometry(QtCore.QRect(40, 30, 181, 31)) 39 self.label_3.setObjectName("label_3") 40 self.pushButton_3 = QtWidgets.QPushButton(Form) 41 self.pushButton_3.setGeometry(QtCore.QRect(220, 380, 111, 41)) 42 font = QtGui.QFont() 43 font.setFamily("Agency FB") 44 font.setPointSize(12) 45 self.pushButton_3.setFont(font) 46 self.pushButton_3.setObjectName("pushButton_3") 47 48 self.retranslateUi(Form) 49 QtCore.QMetaObject.connectSlotsByName(Form) 50 51 def retranslateUi(self, Form): 52 _translate = QtCore.QCoreApplication.translate 53 Form.setWindowTitle(_translate("Form", "猜数字游戏")) 54 self.label.setText(_translate("Form", "<html><head/><body><p><span style=\" font-size:14pt;\">请猜一个数字:</span></p></body></html>")) 55 self.comboBox.setItemText(0, _translate("Form", "初级:数字小于20")) 56 self.comboBox.setItemText(1, _translate("Form", "中级:数字小于30")) 57 self.comboBox.setItemText(2, _translate("Form", "高级:数字小于50")) 58 self.comboBox.setItemText(3, _translate("Form", "魔鬼级:数字小于100")) 59 self.pushButton_2.setText(_translate("Form", "确定")) 60 self.pushButton.setText(_translate("Form", "确定")) 61 self.label_3.setText(_translate("Form", "<html><head/><body><p><span style=\" font-size:14pt;\">请选择游戏难度:</span></p></body></html>")) 62 self.pushButton_3.setText(_translate("Form", "再来一局"))
代码2:runGuess.py (方法主体代码)
1 # -*- coding: utf-8 -*- 2 import sys,random,time 3 from PyQt5.QtWidgets import QApplication, QWidget, QMainWindow 4 from guessNumberGame import Ui_Form 5 6 times=1 #声明一个模块内的全局变量;用于记录猜数字的次数 7 rand=20#声明一个模块内的全局变量;神秘数字的最大范围 8 allTimes=7#声明一个模块内的全局变量;游戏最大次数 9 class mwindow(QWidget, Ui_Form): 10 def __init__(self): #初始化 11 super(mwindow, self).__init__() #这是对继承自父类的属性进行初始化。而且是用父类的初始化方法来初始化继承的属性。 12 self.setupUi(self) 13 #定义一个方法:从下拉框选择游戏难度 14 def gameLevel(self): 15 times=1 16 global rand,allTimes 17 level=self.comboBox.currentIndex() 18 if level==0: 19 rand=20 20 allTimes=7 21 if level==1: 22 rand=30 23 allTimes=10 24 if level==2: 25 rand=50 26 allTimes = 15 27 if level==3: 28 rand=100 29 allTimes = 20 30 31 #定义一个方法:选择游戏难度后生成一个随机的神秘数字 32 def getRandNum(self): 33 global theNum,times 34 times=1 #每次选择游戏难度并点击“确定”后,已猜数字次数都重新归为1 35 w.pushButton.setEnabled(True) #设置pushButton可点击(即选择了游戏难度之后,pushButton才可点击) 36 theNum=random.randint(1,rand) 37 self.textBrowser.append('开始游戏吧,你有%d次机会,数字范围:1-%d' %(allTimes,rand)) 38 # self.textBrowser.append(str(theNum)) #直接显示神秘数字,用于调试时使用 39 40 #定义一个方法:点击“确定”按钮的事件,用于比较所猜数字和神秘数字 41 def guess(self): 42 global allTimes,times #使用全局变量times 43 yourNum = int(self.lineEdit.text()) #从文本框获取到输入的数字,并转化为int型 44 if yourNum < theNum and times < allTimes: 45 text = "你猜的数字%d小了!你还有%d次机会,再猜!" %(yourNum,allTimes-times) 46 self.textBrowser.append(text) #把提示信息写入textBrowser 47 times += 1 48 elif yourNum > theNum and times <allTimes: 49 text = "你猜的数字%d大了!你还有%d次机会,再猜!" %(yourNum,allTimes-times) 50 self.textBrowser.append(text) 51 times += 1 52 elif yourNum == theNum and times <allTimes: 53 text = '你猜对了,就是%d,你一共猜了%s次!' % (theNum,times) 54 self.textBrowser.append(text) 55 else: 56 text = '%d次机会用完了你也没猜对!神秘数字其实是:%d' %(allTimes,theNum) 57 self.textBrowser.append(text) 58 59 #定义一个方法:点击“再来一局”时触发的事件 60 def reStart(self): 61 self.textBrowser.clear() #清除textBrowser内的内容 62 self.lineEdit.clear() #清除lineEdit内的内容 63 w.pushButton.setEnabled(False) #设置pushButton不可点击(即在选择游戏难度之前,pushButton不可点击) 64 65 if __name__ == '__main__': 66 app = QApplication(sys.argv) 67 w = mwindow() 68 w.pushButton.setEnabled(False) #设置pushButton不可点击(即在选择游戏难度之前,pushButton不可点击) 69 w.pushButton.clicked.connect(w.guess) #绑定guess方法 70 w.pushButton_2.clicked.connect(w.getRandNum) 71 w.comboBox.currentIndexChanged.connect(w.gameLevel) 72 w.pushButton_3.clicked.connect(w.reStart) 73 w.show() 74 sys.exit(app.exec_()) #使程序一直循环运行直到主窗口被关闭终止进程(如果没有这句话,程序运行时会一闪而过)