bitcode是什么
Bitcode is an intermediate representation of a compiled program. Apps you upload to iTunes Connect that contain bitcode will be compiled and linked on the App Store. Including bitcode will allow Apple to re-optimize your app binary in the future without the need to submit a new version of your app to the store.
根据官方文档的解释,bitcode是被编译程序的一种中间形式的代码。包含bitcode配置的程序将会在App store上被编译和链接。bitcode允许苹果在后期重新优化程序的二进制文件,而不需要重新提交一个新的版本到App store上。
我们知道,iOS编译使用的是Clang/LLVM编译工具链。LLVM是编译器和工具链技术的集合,Clang 是LLVM的前端。C,C++ 和 Objective-C代码通过Clang编译生成中间代码LLVM IR,再经由后端生成目标平台上的本地字节码。
LLVM比较特别的地方在于LLVM IR,它把编译过程分为了三层。第一层支持多种语言作为输入,无论是OC,C++,Swift代码,最终转化为中间代码LLVM IR。第二层是共享优化器,专门对中间代码优化。第三层是不同目标平台字节码生成。这样的好处在于:想要增加增加一门新语言,只需要增加前端;想要支持新的CPU架构,只需要增加后端。
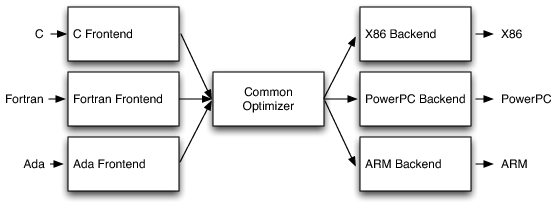
bitcode本质上就是LLVM IR的字节流格式,我们可以尝试生成IR代码,看一看庐山真面目。首先,创建一个test.c:
#include <stdio.h>
int main(int argc, char **argv)
{
printf("hello world\n");
return 0;
}
调用clang指令-emit-llvm
:
clang test.c -S -emit-llvm -o test.bc
生成的test.bc代码如下:
; ModuleID = 'test.c'
source_filename = "test.c"
target datalayout = "e-m:o-i64:64-f80:128-n8:16:32:64-S128"
target triple = "x86_64-apple-macosx10.14.0"
@.str = private unnamed_addr constant [13 x i8] c"hello world\0A\00", align 1
; Function Attrs: noinline nounwind optnone ssp uwtable
define i32 @main(i32, i8**) #0 {
%3 = alloca i32, align 4
%4 = alloca i32, align 4
%5 = alloca i8**, align 8
store i32 0, i32* %3, align 4
store i32 %0, i32* %4, align 4
store i8** %1, i8*** %5, align 8
%6 = call i32 (i8*, ...) @printf(i8* getelementptr inbounds ([13 x i8], [13 x i8]* @.str, i32 0, i32 0))
ret i32 0
}
declare i32 @printf(i8*, ...) #1
attributes #0 = { noinline nounwind optnone ssp uwtable "correctly-rounded-divide-sqrt-fp-math"="false" "disable-tail-calls"="false" "less-precise-fpmad"="false" "no-frame-pointer-elim"="true" "no-frame-pointer-elim-non-leaf" "no-infs-fp-math"="false" "no-jump-tables"="false" "no-nans-fp-math"="false" "no-signed-zeros-fp-math"="false" "no-trapping-math"="false" "stack-protector-buffer-size"="8" "target-cpu"="penryn" "target-features"="+cx16,+fxsr,+mmx,+sahf,+sse,+sse2,+sse3,+sse4.1,+ssse3,+x87" "unsafe-fp-math"="false" "use-soft-float"="false" }
attributes #1 = { "correctly-rounded-divide-sqrt-fp-math"="false" "disable-tail-calls"="false" "less-precise-fpmad"="false" "no-frame-pointer-elim"="true" "no-frame-pointer-elim-non-leaf" "no-infs-fp-math"="false" "no-nans-fp-math"="false" "no-signed-zeros-fp-math"="false" "no-trapping-math"="false" "stack-protector-buffer-size"="8" "target-cpu"="penryn" "target-features"="+cx16,+fxsr,+mmx,+sahf,+sse,+sse2,+sse3,+sse4.1,+ssse3,+x87" "unsafe-fp-math"="false" "use-soft-float"="false" }
!llvm.module.flags = !{!0, !1}
!llvm.ident = !{!2}
!0 = !{i32 1, !"wchar_size", i32 4}
!1 = !{i32 7, !"PIC Level", i32 2}
!2 = !{!"Apple LLVM version 10.0.0 (clang-1000.11.45.5)"}
可以看到,生成的中间代码IR相比汇编代码更为直观易懂。下面简单总结一下bitcode的优缺点:
优点:Apple可以根据目标机型编译生成性能最优,包大小最小的可执行文件。当编译器更新时,Apple可以对提交包含bitcode的应用进行优化而不需要我们重新编译提交。
缺点:bitcode本质上是LLVM IR,相比汇编代码更容易被分析,安全性会下降。
如何支持bitcode
Framework支持bitcode就是在编译好的目标文件中增加一个__LLVM
段用来存放编译生成的中间代码IR。应用支持bitcode的前提是项目中所有第三方库都支持bitcode,否则就只能关闭bitcode选项。
bitcode选项在xcode工程配置中默认是打开的,如果Framework是纯OC代码,只需要在工程选项Other C flags
中增加-fembed-bitcode
即可。如果Framework依赖了其他第三方库,需要所有第三方库也需要支持bitcode。假如第三方库使用clang编译,需要增加编译选项-fembed-bitcode
。
编译完成后,通过otool
命令查看目标文件是否包含存放bitcode的__LLVM
段:
otool -l xxx | grep __LLVM
如果已经包含bitcode,会有如下结果:
segname __LLVM
segname __LLVM
如何剥离bitcode
如果想要剥离掉bitcode,通过xcode提供的bitcode_strip
命令也可以很方便的做到:
xcrun bitcode_strip input [ -r | -m | -l ] -o output