一,使用自带的NSJSONSerialization
苹果从IOS5.0后推出了SDK自带的JSON解决方案NSJSONSerialization,这是一个非常好用的JSON生成和解析工具,效率也比其他第三方开源项目高。
NSJSONSerialization能将JSON转换成Foundation对象,也能将Foundation对象转换成JSON,但转换成JSON的对象必须具有如下属性:
1,顶层对象必须是NSArray或者NSDictionary
2,所有的对象必须是NSString、NSNumber、NSArray、NSDictionary、NSNull的实例
3,所有NSDictionary的key必须是NSString类型
4,数字对象不能是非数值或无穷
注意:尽量使用NSJSONSerialization.isValidJSONObject先判断能否转换成功。
样例如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
import
UIKit
class
ViewController
:
UIViewController
{
override
func
viewDidLoad() {
super
.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
var
label:
UILabel
=
UILabel
(frame:
CGRectMake
(100, 100,300,100));
label.text =
"输出结果在控制台"
self
.view.addSubview(label)
//测试结果在output终端输入,也可以建个命令行应用测试就可以了
testJson()
}
override
func
didReceiveMemoryWarning() {
super
.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
//测试json
func
testJson() {
//Swift对象
let
user = [
"uname"
:
"张三"
,
"tel"
: [
"mobile"
:
"138"
,
"home"
:
"010"
]
]
//首先判断能不能转换
if
(!
NSJSONSerialization
.isValidJSONObject(user)) {
println
(
"is not a valid json object"
)
return
}
//利用OC的json库转换成OC的NSData,
//如果设置options为NSJSONWritingOptions.PrettyPrinted,则打印格式更好阅读
let
data :
NSData
! =
NSJSONSerialization
.dataWithJSONObject(user, options:
nil
, error:
nil
)
//NSData转换成NSString打印输出
let
str =
NSString
(data:data, encoding:
NSUTF8StringEncoding
)
//输出json字符串
println
(
"Json Str:"
);
println
(str)
//把NSData对象转换回JSON对象
let
json :
AnyObject
! =
NSJSONSerialization
.
JSONObjectWithData
(data, options:
NSJSONReadingOptions
.
AllowFragments
, error:
nil
)
println
(
"Json Object:"
);
println
(json)
//验证JSON对象可用性
let
uname :
AnyObject
= json.objectForKey(
"uname"
)!
let
mobile :
AnyObject
= json.objectForKey(
"tel"
)!.objectForKey(
"mobile"
)!
println
(
"get Json Object:"
);
println
(
"uname: \(uname), mobile: \(mobile)"
)
}
}
|
输出结果如下:
1
2
3
4
5
6
7
8
9
10
11
12
|
Json Str:
Optional({
"uname"
:
"张三"
,
"tel"
:{
"home"
:
"010"
,
"mobile"
:
"138"
}})
Json
Object
:
{
tel = {
home =
010
;
mobile =
138
;
};
uname =
"\U5f20\U4e09"
;
}
get
Json
Object
:
uname: 张三, mobile:
138
|
二,使用第三方库 - JSONKit
1,新建桥街头文件Bridging-Header.h,并设置到编译参数里
1
|
#
include
"JSONKit.h"
|
2,将JSONKit的库文件导入到项目中来(JSONKit.h和JSONKit.m)
3,这时编译会发现报错,这是由于JSONKit库不支持Objective-C的自动引用计数功能导致。
需要在Build Phases -> Compile Sources -> JSONKit.m,双击添加Comipler Flag:-fno-objc-arc 。这样就完成了不支持自动引用计数的配置。
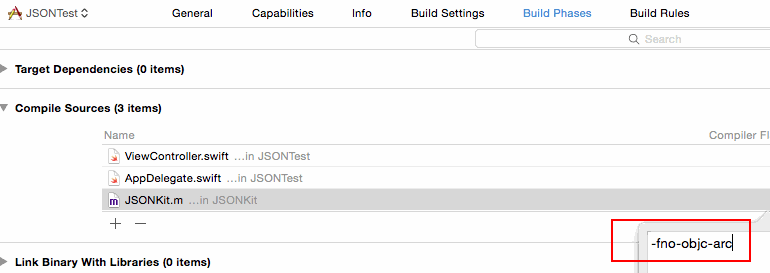
测试代码:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
import
UIKit
class
ViewController
:
UIViewController
{
override
func
viewDidLoad() {
super
.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
testJson()
}
override
func
didReceiveMemoryWarning() {
super
.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func
testJson() {
//Swift 字典对象
let
user = [
"uname"
:
"user1"
,
"tel"
: [
"mobile"
:
"138"
,
"home"
:
"010"
]
]
//使用 JSONKit 转换成为 JSON 字符串
var
jsonstring = (user
as
NSDictionary
).
JSONString
()
println
(jsonstring);
//由字符串反解析回字典
println
(jsonstring.objectFromJSONString()
as
NSDictionary
)
//使用 JSONKit 转换成为 NSData 类型的 JSON 数据
var
jsondata = (user
as
NSDictionary
).
JSONData
()
println
(jsondata);
//由NSData 反解析回为字典
println
(jsondata.objectFromJSONData()
as
NSDictionary
)
}
}
|
输出结果:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
{
"uname"
:
"user1"
,
"tel"
:{
"home"
:
"010"
,
"mobile"
:
"138"
}}
{
tel = {
home =
010
;
mobile =
138
;
};
uname = user1;
}
<7b22756e 616d6522 3a227573
65723122
2c227465 6c223a7b 22686f6d 65223a22
30313022
2c226d6f 62696c65 223a2231 3338227d 7d>
{
tel = {
home =
010
;
mobile =
138
;
};
uname = user1;
}
|